As a C programmer, you will often need to execute or run a set of instructions repeatedly based on specific conditions. More specifically, while
and do..while
loops provide you with the tools to accomplish this task with ease, which makes your code more efficient and effective.
This blog will guide you through creating while
and do..while
loops in C, by discussing their definitions, workflow, syntax, and the relevant example. So keep reading to take your programming skills to the next level!
What is a while loop in C?
In C, a while loop
is a control flow statement that permits the execution of a code block repeatedly as long as the defined condition is evaluated as true.
It can be utilized in scenarios where it is required to repeatedly run until a specific condition is met.
How to Create/Define a while loop in C?
You can easily create an while loop
in C by following the below-given syntax.
while (condition) { // Code that needs to be executed repeatedly }
The while loop
is defined using the while
keyword. Then, before moving to the execution of the added statements, the condition is verified and as long as the condition stated in the parentheses is true, the code specified in the curly braces will run repeatedly.
If it is false, the while loop
will terminate, and the execution control moves to the next statement that is defined after the loop.
Working of while loop in C
As discussed earlier, when the condition specified in the parentheses is true, the while loop
keeps running the code inside the loop. Before starting each iteration of the loop, the condition is checked or verified, and if it is evaluated as true, the added code statements will execute.
The condition is rechecked after each iteration of the loop, and if it is still true, the loop is continued. However, if the condition is false, the loop gets terminated, and the execution control is redirected to the statement that follows the loop.
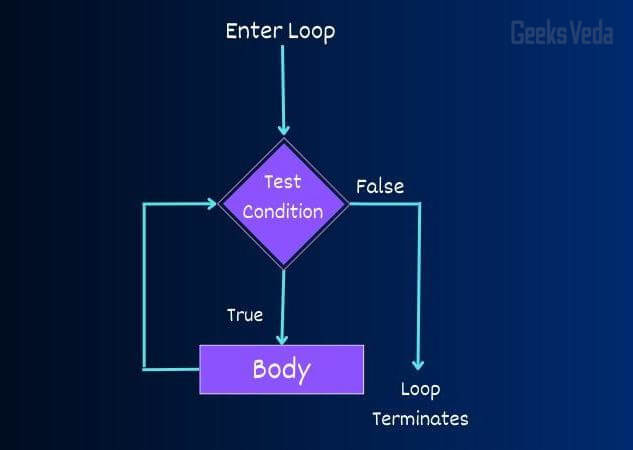
Moreover, in order to prevent the creation of the infinite loop, it is crucial to make sure that the condition defined in the while loop
will somehow evaluate as false. Now, let’s head toward the following examples.
Example 1: Create a while loop to Print the Numbers From 1 to 10
In the following example, we will utilize a while loop
for printing numbers from 1 to 10. To do so, first include the standard input/output library stdio.h
, which offers functions for input/output operations.
Within the main()
function, create a variable i as the starting point of the while loop
and assign it the value 1. Then, define the while loop
to iterate over the number from 1 to 10.
According to the specified condition, this loop
will execute until the variable’s value remains less than or equal to 10. On each iteration, the while loop
prints the value of i variable using the printf()
function.
After doing so, the variable value will be incremented by 1 by utilizing the post-increment operator i++. Moreover, when the value of i becomes greater than 10, the loop gets terminated, and the program ends.
#include <stdio.h> int main() { int i = 1; while (i <= 10) { printf("%d ", i); i++; } }
It can be observed that the while loop has successfully printed out the number from 1 to 10 as the output.
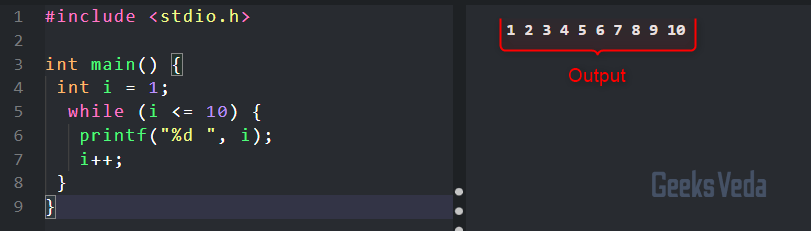
Example 2: Create a while loop to Find the Largest Number in the Array
Now, we will write a C program for finding the largest number in an array. For the corresponding purpose, firstly, we have initialized an array arr of size 6 having the integers 88, 99, 22, 11, 33, and 44.
Then, two integer variables max and i are declared and initialized to the first element [0] of the arr array and 1, respectively.
Next, a while loop
is defined for iterating over the remaining elements of the arr array. Note that this loop continues as long as i variable value is less than 6, which is the size of the array.
Within the while loop
, an if
statement is defined to compare the current element of the array (arr[i])
with the current maximum value (max). If the current element is greater than the value stored in max, its value will be updated accordingly.
Then, the post-increment operator i++
will increment the value of i
. The while loop
will terminate when it finds the largest number in the array and outputs it to the console with the help of the printf()
function.
#include <stdio.h> int main() { int arr[6] = {88, 99, 22, 11, 33, 44}; int max = arr[0], i = 1; while (i < 6) { if (arr[i] > max) { max = arr[i]; } i++; } printf("Largest number is: %d", max); }
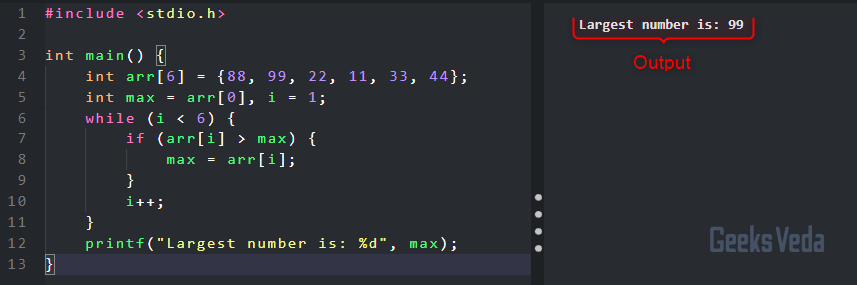
What is do..while loop in C?
The do..while
loop in C is a loop that runs or executes the added statements at least once before verifying the defined condition.
It can be differentiated from the while loop
in a way that do..while
loop allows the code block to run at least once even if the added condition is initially evaluated as false.
How to Create/Define a do..while loop in C?
Check out the provided syntax for creating a do..while
loop in C:
do { // Code that needs to be executed repeatedly } while (condition);
Here, the do keyword represents the starting of the loop, followed by the code block or the statements that need to be executed repeatedly. Then, a condition is defined after adding the while keyword.
This condition will be verified after each iteration, except for the first one. Moreover, if the defined condition is evaluated as true, the loop execution continues, otherwise, the do..while loop gets terminated.
Working of do..while loop in C
In C programming, a do..while
loop runs the added statements at least once before verifying the condition. Then, the loop will carry on running the body if the condition is true and check again at the end of each iteration. The loop will end and control will be passed to the statement after it if the condition is evaluated as false.
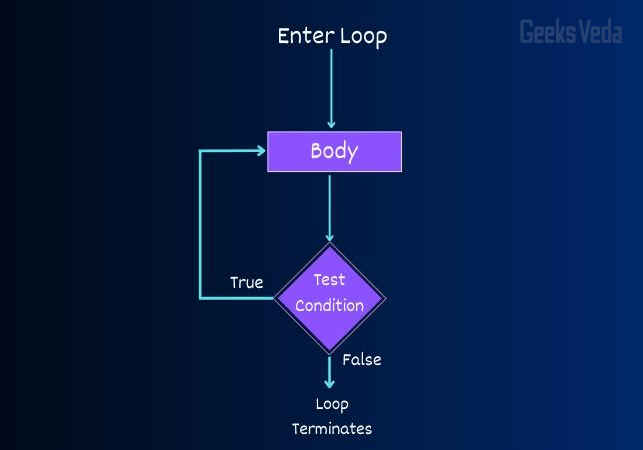
Moreover, it is the specialty of the do..while
loop that it runs code at least once before verifying the condition.
Example 1: Create a do..while loop to Print the Numbers When the Condition is Initially False
This example will demonstrate how do..while
loop works when the condition is initially false. As the first step, the variable i has been declared and initialized with the value 10.
Then, a do..while
loop is defined that will iterate over the numbers from the 10 and greater than it. This loop will continue its execution until the value of the variable i
is less than or equal to 9.
Within the do..while
loop body, the printf()
function is invoked that prints the value of i
variable. After that, the variable’s value will be incremented by 1, likewise, using the post-increment operator (i++)
.
#include <stdio.h> int main() { int i = 10; do { printf("%d ", i); i++; } while (i <= 9); }
Even though the condition i <=9
of the do-while
loop is initially false, the added statements will be executed for once.
Therefore, the value of i
is printed to the console once, followed by a space character. After that, when the value of i
becomes greater than 9, the loop gets terminated.
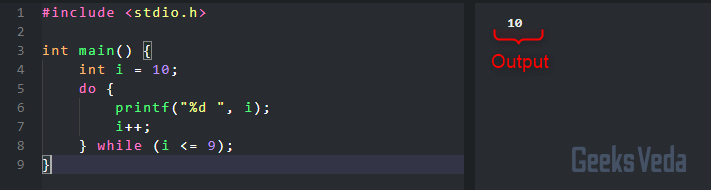
Example 2: Create a do..while loop to Get the Sum of Natural Numbers
Now, let’s check out the approach to get the sum of natural numbers from 1 to 15 with the help of the do-while
loop. For this purpose, the variables i
and sum are initialized to 1 and 0, accordingly.
The do..while
loop has to iterate until the value of i
variable is less than or equal to 15. Within each iteration of the loop, the value of i
is added or appended to the variable sum using the +=
shorthand notation.
After doing so, the i
variable value is then incremented by 1 by utilizing the post-increment operator i++
.
#include <stdio.h> int main() { int i = 1, sum = 0; do { sum += i; i++; } while (i <= 15); printf("Sum of Natural Numbers is: %d", sum); }
At the end of the iterations, the sum of the natural numbers from 1 to 15 stored in the variable sum will be printed out by invoking the printf()
function.
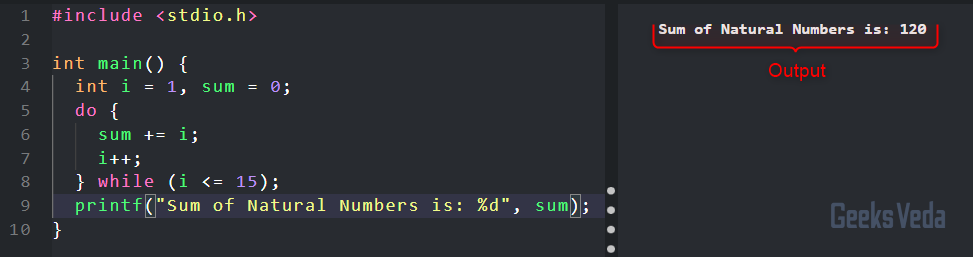
When to Use while and do..while loop in C?
Here, we have enlisted the use cases of while
and do..while
loops in C:
while loop
- When it is required to run a set of statements repeatedly based on the defined condition that is checked or verified before the loop body is executed.
- When you have no idea about the exact number of times the loop will be executed, but you know the condition under which it will terminate.
- When the loop condition is initially false, the loop will not run at all.
do..while loop
- When you need to run a set of statements repeatedly based on a condition that is evaluated after the loop body is executed at least once.
- When it is required to run the loop body at least once and it does not matter whether the loop condition is true or false.
- Moreover, the do..while loop can be utilized when you need to prompt the user for input until valid input is received.
Overall, both of the discussed loops provide different functionalities and you need to choose the most suitable one based on your program requirements.
Conclusion
Both while
and do..while
loops are essential C programming concepts that can significantly improve the effectiveness of your programs. These loops provide programmers with more control over the execution and flow of their programs, enabling them to code in a more streamlined way.
Moreover, you can improve your programming abilities by understanding how to use these loops in C with the help of the provided details in our post.