In programming and mathematics, multiplication tables serve as fundamental tools that contribute to understanding, learning, and problem-solving. More specifically, displaying these tables is not only about numbers, it assists in making patterns, educating, and improving coding skills.
Today’s guide is all about displaying multiplication tables in Python. From nested loops to string formatting, list comprehension, and the use of the panda DataFrames, this guide will discuss all approaches with practical examples!
What is the Purpose of Multiplication Table in Python
As discussed earlier, displaying the multiplication table is more than a routine task and it holds its importance in problem-solving, mathematical learning, and coding operations.
Here are some of the use cases to explain the significance of showing multiplication tables in Python:
- Educational Aid – Multiplication tables are fundamental to arithmetic education, aiding students in understanding multiplication concepts.
- Mathematical Visualization – Displaying multiplication tables visually enhances mathematical comprehension and pattern recognition.
- Algorithm Development – In programming, multiplication tables can serve as building blocks for algorithm development and optimization.
- Coding Practice – Creating multiplication tables provides hands-on coding experience for beginners to sharpen their skills.
- Data Representation – Multiplication tables are often used to represent data in a tabular format, useful in various data analysis tasks.
- Interactive Learning – Interactive multiplication tables can engage learners in a dynamic and engaging manner.
- Mathematical Games – Multiplication tables are central to numerous educational games that strengthen mental math skills.
- Debugging and Testing – Multiplication tables can be used as a testing ground for coding and debugging exercises.
- Educational Software – Multiplication tables can be integrated into educational software to enhance the learning experience.
How to Display Multiplication Table in Python
In order to display the multiplication table in Python, you can use:
- Nested Loops
- String Formatting
- List Comprehension
- Pandas DataFrame
Let’s check out each of the listed approaches!
1. Using Nested Loops
Using nested loops is the traditional approach for generating multiplication tables. More specifically, by using the two for loops, one for rows, and another for columns, you can systematically calculate and show the products.
def display_multiplication_table(n): for i in range(1, n+1): for j in range(1, n+1): print(f"{i} x {j} = {i*j}") print() table_size = int(input("Enter table size: ")) display_multiplication_table(table_size)
Here, firstly, we will enter the table size. As a result, the multiplication table will be displayed on the console.
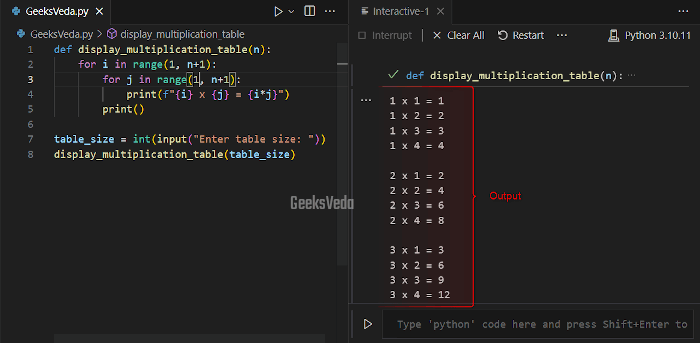
2. Using String Formatting
String formatting is a methodical approach that can be utilized for presenting multiplication tables with precision and clarity. Using this approach, you can control the alignment and spacing of the elements which help in creating an organized and visually appealing output.
In the following program, the “display_multiplication_table()
” function employs the string formatting approach to ensure a neatly arranged multiplication table. Here, the format specifier “2” aligns each product within a field with 2 characters.
def display_multiplication_table(n): for i in range(1, n+1): for j in range(1, n+1): product = i * j print(f"{i} x {j} = {product:2}", end="\t") print() table_size = int(input("Enter table size: ")) display_multiplication_table(table_size)
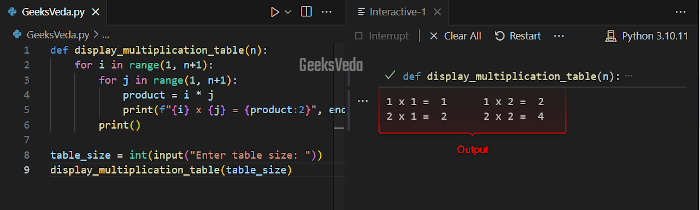
3. Using List Comprehension
List comprehension provides a concise method for creating a multiplication table by generating a list of formatted strings. This approach is primarily utilized in scenarios where it is required to generate smaller multiplication tables.
Here, the “display_multiplication_table()
” function uses the list comprehension method for generating a list of formatted strings that represents the multiplication table. Then, the list will be sliced and joined to create rows.
def display_multiplication_table(n): table = [f"{i} x {j} = {i*j:2}" for i in range(1, n+1) for j in range(1, n+1)] for i in range(0, n*n, n): print("\t".join(table[i:i+n])) table_size = int(input("Enter table size: ")) display_multiplication_table(table_size)
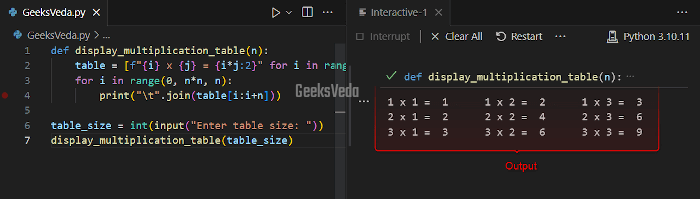
4. Using Pandas DataFrame
With the help of the pandas library, you can create multiplication tables in the form of a structured DataFrame. This method not only offers an organized presentation but also provides several opportunities for data manipulations and analysis within the DataFrame structure.
According to the given code, we have a “display_multiplication_table()
” function that creates a multiplication table using a nested list comprehension. The resultant data will be structured into a DataFrame where each row and column corresponds to the factors and products respectively.
import pandas as pd def display_multiplication_table(n): data = [[i * j for j in range(1, n+1)] for i in range(1, n+1)] df = pd.DataFrame(data, index=range(1, n+1), columns=range(1, n+1)) print(df) table_size = int(input("Enter table size: ")) display_multiplication_table(table_size)
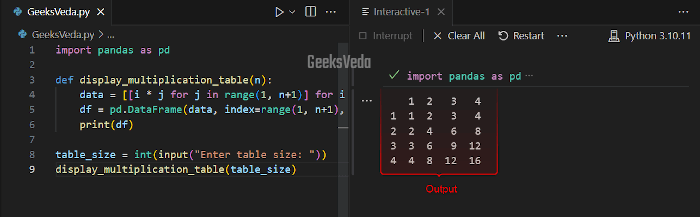
Choosing the Right Method to Display the Multiplication Table
Have a look at the table for selecting the right method for displaying the multiplication table:
Method | Advantages | Disadvantages | Use Cases |
Nested Loops |
|
|
Basic educational purposes, learning programming concepts, smaller tables. |
String Formatting |
|
|
Aesthetically pleasing presentation, visual representation, and medium-sized tables. |
List Comprehension |
|
|
Quick creation of smaller tables, practice with list comprehension, compact code. |
Pandas DataFrame |
|
|
Data analysis tasks, integration with other data manipulation, larger and structured tables. |
That’s all from today’s effective guide related to displaying multiplication tables in Python.
Conclusion
In Python, you can display multiplication tables with the help of different approaches. From the straightforward nested loops to the creative formatting of string manipulation, each method has its own pros and cons depending on the use cases.
So, whether you are aiming for educational purposes, visual appeal, or efficient data handling, these diverse options offer flexibility in achieving your goals.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!