In today’s guide, let’s discover the Python string formatting techniques for flexible and concise code. We will primarily discuss the old-style formatting (“%” operator
), new-style formatting (str.format()
), f-strings, template strings, and other advanced string formatting techniques.
Moreover, by following our guide, you will be able to format strings, numbers, dates, and time, align and pad strings, handle precision, and utilize other features, such as format specifiers, dictionaries, and keyword arguments.
Old Style Formatting String Using % Operator Method
In Python, the “%
” operator permits you to format string with the help of the placeholders, such as.
- “
%s
” for strings. - “
%f
” for floats. - “
%d
” for integers.
It enables you to substitute the placeholders with the respective values.
For instance, in the provided example, the “%
” operator has been utilized for formatting a string with placeholders. Here, the values of the “name
” and “age
” strings are substituted into the placeholders “%s
” and “%d
“.
name = "Sharqa Hameed" age = 25 formatted_string = "My name is %s and I'm %d years old." % (name, age) print(formatted_string)
It can be observed that the formatted string has been successfully displayed on the console.
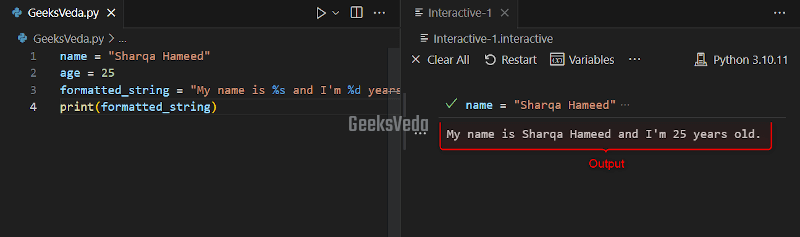
New Style Formatting String Using str.format() Method
The “str.format()
” Python method offers a more improved readability, flexibility, and better support for complex string formatting scenarios. It enables you to design placeholders by utilizing the “{}
” curly braces, which can be then replaced with the variables or values.
Here, we have invoked the “str.format()
” method for formatting a string. The placeholders “{}
” are used and the “name
” and “age
” values have been passed as arguments to the format()
method.
The placeholders are then replaced with the respective value, ultimately displaying the formatting string.
name = "Sharqa Hameed" age = 25 formatted_string = "My name is {} and I'm {} years old.".format(name, age) print(formatted_string)
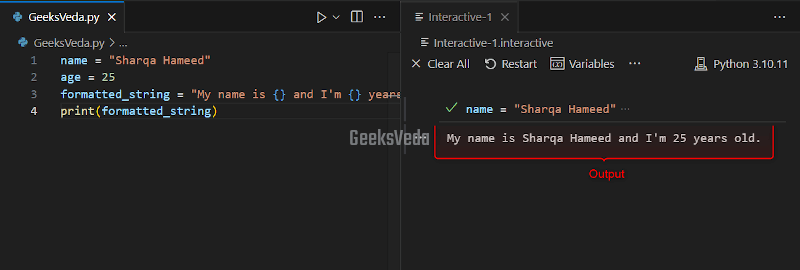
String Interpolation Using f-strings
“f-strings
” or formatted string literals were introduced in Python 3.6. They offer an intuitive and concise way of performing string interpolation, permitting you to embed expressions directly inside string literals, and minimizing the need for explicit concatenations or conversions.
For instance, a “f-string
” is now utilized for formatting a string. Then, the expressions inside the curly braces “{}
” and their values have been inserted directly into the strings.
Lastly, the print() function will be displayed on the console.
name = "Sharqa Hameed" age = 25 formatted_string = f"My name is {name} and I'm {age} years old." print(formatted_string)
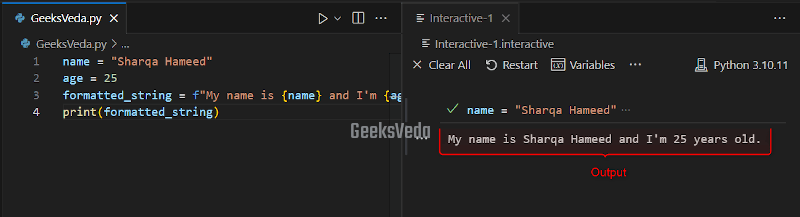
String Formatting Using Template Strings
The “Template Strings
” in Python provides a simple form of string substitution. They can be utilized in scenarios where it is required to perform basic variable substitutions without the requirement of complex manipulation or formatting.
In the given program, a template string is defined using the “Template” class from the “string” module. Next, the placeholder variables have been defined in the form of “$name
” and “$age
“.
After that, the “substitute()
” has been invoked for substituting the placeholder with the given values.
from string import Template name = "Sharqa Hameed" age = 25 template = Template("My name is $name and I'm $age years old.") formatted_string = template.substitute(name=name, age=age) print(formatted_string)
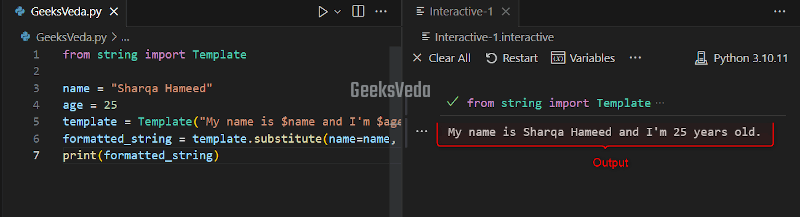
Common String Formatting Techniques in Python
Now, let’s discuss some common string formatting techniques and their practical usage.
Formatting Numbers, Dates, and Times
Python String formatting permits you to format numbers, dates, and times with respect to particular conventions or patterns. You can utilize format specifiers to control the decimal places, precision, time, and date formats as well.
Here, it can be seen that the “{:.2f}
” format specifier has been utilized for formatting the “num
” variable with two decimal places and the resultant formatted number will be shown using the print()
function.
num = 123.456789 formatted_num = "{:.2f}".format(num) print(formatted_num)
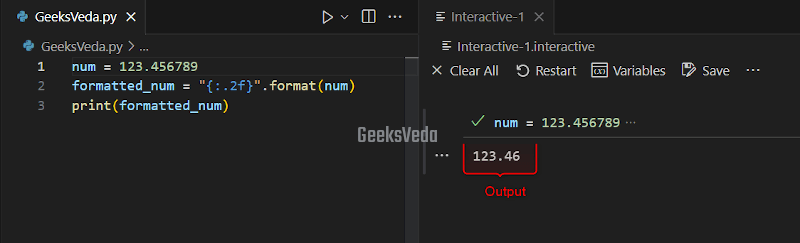
Alignment and Padding String in Python
You can also pad and align strings for achieving visually appealing output in Python. For this, utilize the alignment specifiers, like “>
“, “<
“, and “^
“, along with the padding characters for the alignment of the string to the right, left, or center.
This approach is useful for creating well-structured output or aligning columns.
For instance, we have now used the “>
” alignment specified and “10
” as the width specifier for aligning the “name
” string to the right having a field width of 10.
As a result, the padded string will be displayed on the console.
name = "Sharqa Hameed" formatted_name = "{:>10}".format(name) print(formatted_name)
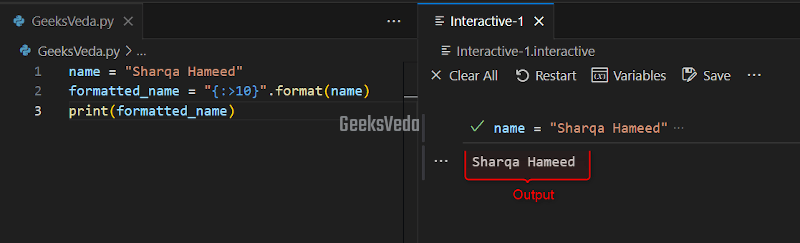
Handling Precision and Rounding in Python
In Python, you can also control rounding and precision with string formatting when you deal with floating-point numbers. To do so, specify the precision by utilizing the format specifier and apply rounding as required.
For example, we have used the “{:.2f}
” format specifier for formatting the defined variable with two decimal places.
num = 3.14159 formatted_num = "{:.2f}".format(num) print(formatted_num)
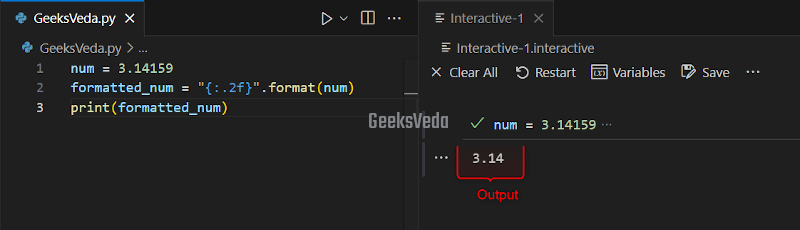
Advanced String Formatting in Python
To go one step further, you can check out the advanced string formatting technique, such as using format specifiers and conversion flags and working with dictionaries and keyword arguments. Let’s observe each of them practically!
Using Format Specifier and Conversion Flags
This advanced technique of string formatting enables you to customize the output by using the format specifier such as “%
“, “#
“, and “0
” and the conversion flags. Additionally, it also provides other formatting options and behavior.
Let’s format the “value
” variable by specifying “{:#x}
” as the format specifier which represents a hexadecimal number with the “0x
” prefix.
value = 42 formatted_value = "{:#x}".format(value) print(formatted_value)
This technique gives you full control over the presentation and formatting of the output.
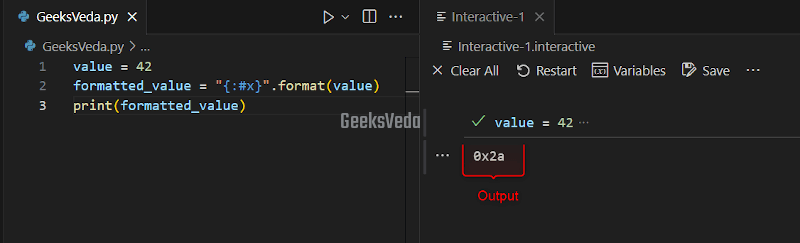
Working with Dictionaries and Keyword Arguments
Using this approach, you can pass the key-value pair to the “format()
” method and the keys can be utilized as placeholders in the string.
For instance, in the provided code, the “format()
” method has been invoked with the keyword arguments. The keys of the “person
” dictionary, like “name
” and “age
” have been utilized as the placeholders in the string, which are then substituted in the placeholders.
person = {"name": "Sharqa", "age": 25} formatted_string = "My name is {name} and I'm {age} years old.".format(**person) print(formatted_string)
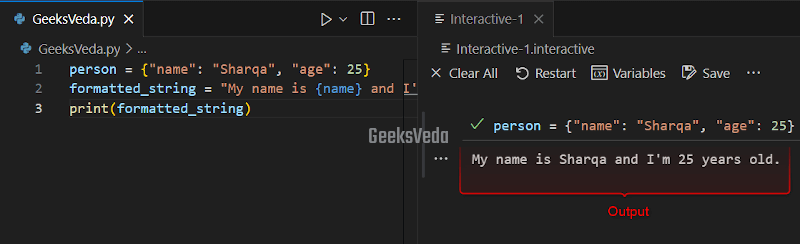
Best Practices for String Formatting in Python
Have a look at the given best practices for formatting strings in Python.
- Utilize new-style string formatting “
f-strings
” or “str.format()
“. - Avoid old-style string formatting “
% operator
” for complex tasks. - Select suitable format specifiers for alignment, precision, and padding.
- Use conversion flags and format options for organized formatting.
- Consider data type and use corresponding format specifiers.
- Use keyword arguments or dictionaries for organized formatting.
- Maintain code organization and readability.
That’s all from today’s essential guide related to Python string formatting.
Conclusion
Mastering the art of string formatting in Python is important for efficient and clean code. Through this guide, you have learned about old-style formatting, new-style formatting, f-strings, template strings, and other advanced string formatting approaches that can enhance the effectiveness and readability of your Python programs.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!