Have you ever thought about why new Python programmers start learning to program with the “Hello world!” a go-to message? The answer lies in its simplicity. Printing the mentioned string is a quick and simple approach to ensure that this is working as required.
This article will demonstrate several methods for printing the Hello World! message in Python, ranging from the standard print()
function to other approaches like f-strings and the %
operator.
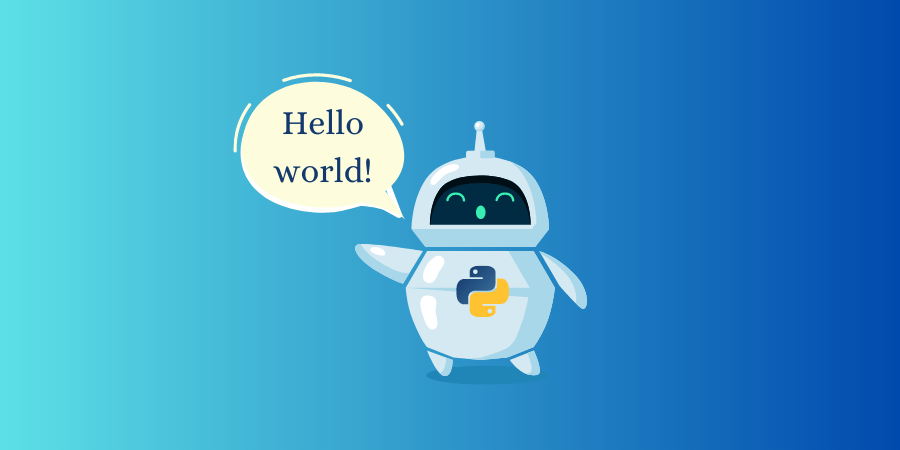
So, whether you are a beginner or a professional developer, mastering these basic methods is crucial for building more complex applications and programs.
1. Using Python print() function
In Python, the built-in print()
function displays the added message to the console. Programmers use this function for debugging purposes and to print out any information in a user interface.
To utilize the print()
function, follow the provided syntax.
print("string")
Here, the print()
function displays the added string on the console.
For instance, we will now call the print()
function for printing the string “Hello world!” on the console.
print("Hello world!")
Another approach is to store the specified string in a variable named str
and pass it as an argument to the print()
function.
str = "Hello world!" print(str)
It can be observed that the “Hello world!” string has been displayed on the console.
Moreover, you can also invoke the print()
function two times and pass the substrings one by one, as “Hello” and “World!”.
print("Hello") print("world!")
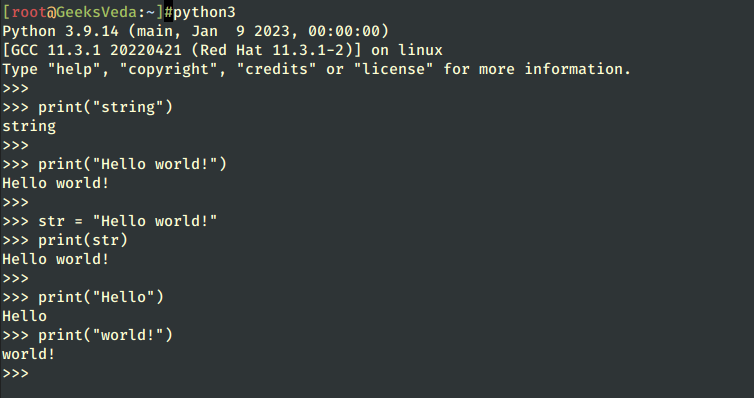
2. Using Python f-strings
f-strings, or formatted string literals, are a feature in Python that allows for the insertion of expressions inside string literals. It is created by placing the “f” character before the starting double quotes. The literal string added after that will be shown in the output.
Here is the syntax utilized for passing f-strings as an argument to the print()
function.
print(f"string")
Let’s try to print the “Hello world!” f-string using the print() function as follows:
print(f"Hello world!")
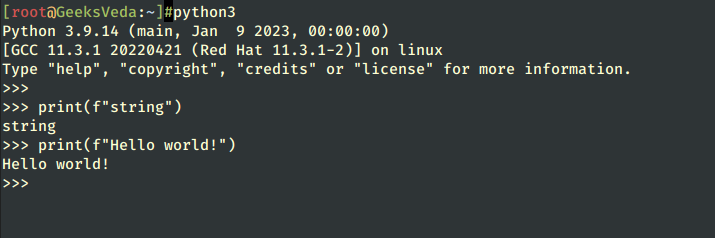
3. Using Python Concatenation
The process of joining or combining two or more substrings to form a new string is known as concatenation, which can be performed using the “+”
operator.
For instance, we will now concatenate the “Hello” and “World!” substrings and also add a space between them " "
using double quotes.
print("Hello" + " " + "World!")
Or you can store the substrings in relevant variables and then concatenate them using the “+”
operator.
str1 = "Hello" str2 = "world!" print(str1 + " " + str2)
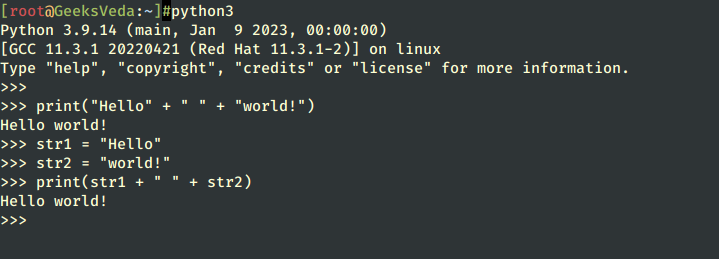
4. Using Python format() Method
The Python format()
method allows the insertion of values into a string by replacing placeholders with the values of the variables. This method is primarily utilized for generating output that contains dynamic values.
The syntax for using the format()
method is as follows:
string.format(value1, value2, ...)
Here:
- string represents the string you want to format.
- value1, value2,…, are the values required to insert in the specified string.
In the following example, the format()
method will insert the string “Hello” into the placeholder {}
in the string as “{} world!”
. As a result, the string “Hello world!” will be printed out on the console.
print("{} world!".format("Hello"))
In the other way, the following format()
method will replace two placeholders “{} {}”
with the values of the defined string “str1” and “str2”, respectively, resulting in the string “Hello world!”.
str1 = "Hello" str2 = "world!" print("{} {}".format(str1, str2))
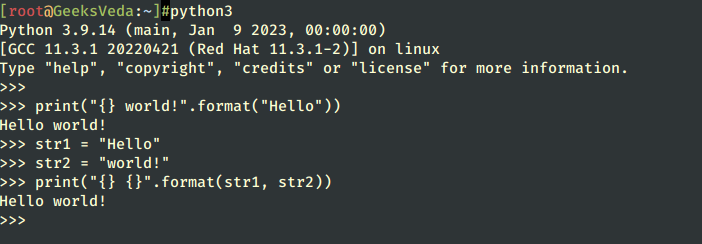
5. Using Python % Operator
The Python “%”
modulo operator outputs the remainder of the two operand’s division. However, it can be also utilized for string formatting. Similar to the format()
method, this operator permits you to insert the values into a string.
Here is the syntax for utilizing the string formatting operator.
string % values
According to the given syntax, “values” can be a single value or a tuple that you want to insert into the “string“.
In the given code, the “%s world!”
is a string that comprises a placeholder “%s”
. This placeholder tells the Python interpreter to insert a string value into that location.
Then, the “%”
operator is utilized for applying the string formatting. Next, the “Hello” string is passed as an argument to the operator.
print("%s world!" % "Hello")
Now, when the print()
function is invoked, it returns the resultant string to the console as “Hello world!”.
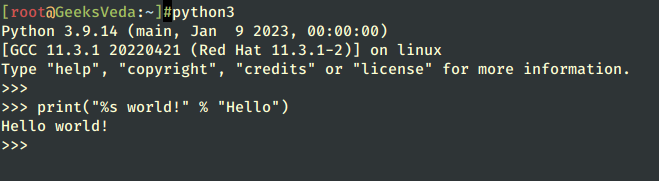
6. Using Python join() Method
Python built-in method join()
can be utilized for joining together list elements or single strings using the added delimiter.
Follow the provided syntax for using the join()
method.
delimiter.join(iterable)
Here:
- delimiter is the string that separates the elements of the sequence.
- iterable is the sequence of the strings that are required to join.
In the below-given code, the join()
method is added to join the string “Hello” and “world!” into a single string which will be separated by a space that is specified as a delimiter. Lastly, the print()
function will output the resultant string.
print(" ".join(["Hello", "world!"]))
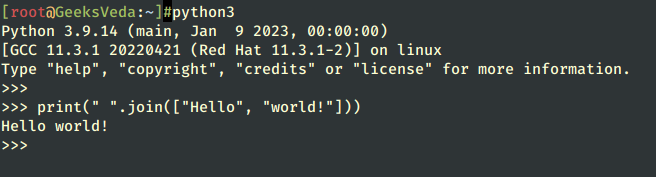
7. Using Python * Operator
The *
(multiplication) operator in Python is a versatile tool that can be used for several purposes. For instance, for multiplication, repetition, argument, keyword unpacking, or adding a prefix to a parameter name.
For example, we have defined a tuple named “greeting” as (“Hello”, “world!”). Then, the “*”
operator is defined in the print()
function. The added operator will unpack the tuple elements and pass them as separate arguments to the function.
greeting= ("Hello", "world!") print(*greeting)
As a result, the print()
function will print “Hello world!” to the console.
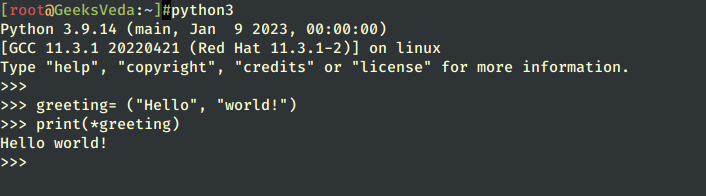
8. Using Python write() Method
The Python “sys” module included a write()
function that writes a string to the standard output stream. More specifically, the “sys.stdout.write()” accepts a string as an argument and writes it to the standard output stream without adding a new line character at the end.
Here is how to utilize the write()
method for printing the Hello world! message to the console.
import sys sys.stdout.write("Hello world!")
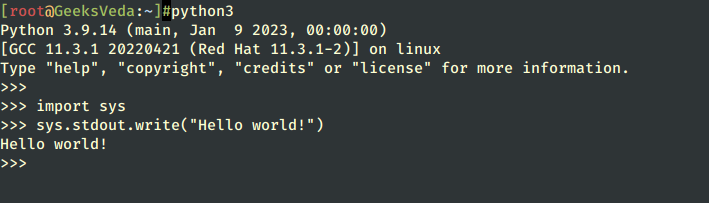
That’s how you can print out the specified message using multiple approaches in Python.
Conclusion
Every beginner should master the basic Python programming task of printing “Hello world!”. Whether you utilize, the print()
function, f-strings, str.format()
method, *
or %
operators, or write()
method, the end result will be the same.
Exploring and experimenting with different approaches and understanding their syntax will assist you in becoming a proficient Python programmer.