JavaScript array manipulation with our in-depth guide on the forEach()
method. As a fundamental tool for iterating through array elements, forEach()
offers a simple syntax and versatile functionality.
In today’s blog, we will explore the syntax, basic usage, and practical applications of forEach
. So stay tuned and discover how to perform different operations on array elements, modify values, extract specific elements, and iterate 2d arrays as well.
Understanding forEach() Method in JavaScript
In JavaScript, the “forEach()
” method permits you to iterate over the array’s elements and perform any particular operation on each element. It offers an expressive and concise way to work with arrays, which makes the code more efficient and readable.
1. Syntax of forEach() Method in JavaScript
To utilize the forEach()
method, follow the provided syntax.
array.forEach(callback(currentVal, index, array), thisArg);
Here:
- The “
callback
” parameter is a function that will be executed on each array item or element. It function accepts three arguments: “currentVal
“, “index
“, and “array
“. - The “
currentVal
” refers to the current element value that is being processed. - The “
index
” is the current element index. - The “
array
” signifies the array object being traversed. - The “
thisArg
” is an optional parameter that uses “this
” value within the callback function.
2. Basic Use of forEach() Method in JavaScript
First of all, we have created a “numbers
” array having the given five elements. After that, the forEach()
method is called on this array and it takes a callback function as an argument.
More specifically, the callback function is defined by utilizing the array function syntax, which accepts two arguments, the current element as a “number
” and its “index
“.
Within the callback function, invoke the console.log() method to print a message for each element.
const numbers = [11, 22, 33, 44, 55]; numbers.forEach((number, index) => { console.log(`Element at index ${index} is: ${number}`); });
It can be observed that the forEach()
method successfully iterates over the given array and the corresponding callback function is executed for each array element.
Resultantly, the current element index of the number array and its respective value has been displayed on the console.
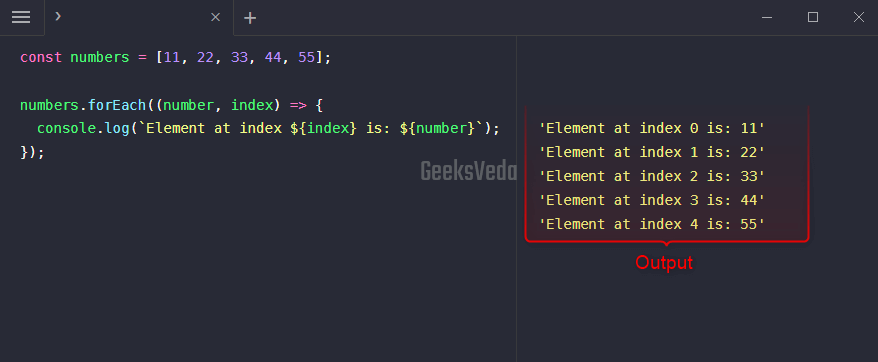
3. Role of the Callback Function in forEach() Method
As discussed earlier, a callback function is specified in the forEach()
method to invoke it for each array element. It accepts three arguments, the currentValue, index, and the array. Additionally, the callback
function handles the operations or actions that are required to perform on each element during the iteration.
In the provided example, the callback is defined by passing three arguments, “number
“, “index
“, and “array
“, which represent the current element, its index, and the created array.
So, according to the function body, the first “console.log()
” method will display a message comprising the current index and the element value and the second statement will show the enter array.
const numbers = [11, 22, 33, 44, 55]; numbers.forEach((number, index, array) => { console.log(`Element at index ${index} is: ${number}`); console.log(`Current array is: ${array}`); });
The forEach()
method will be iterated over the numbers array and the added callback function is executed for each element. In the end, the index of the current element, value, and the whole array will be displayed on the console.
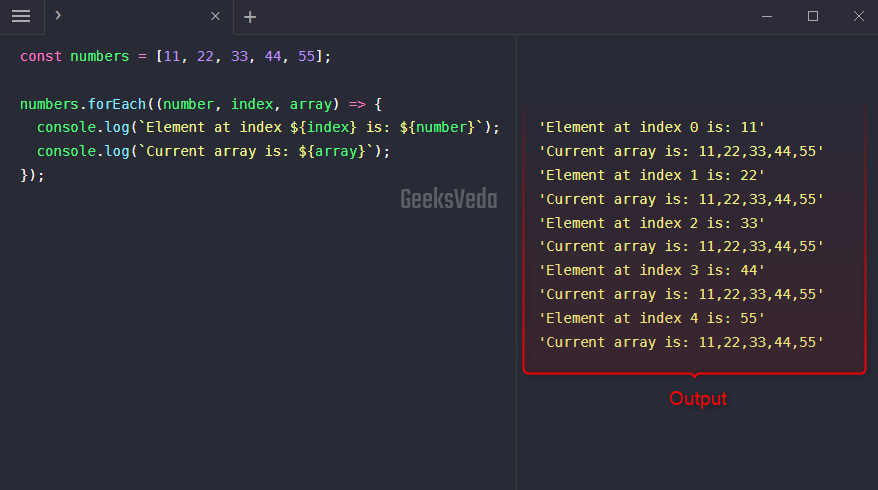
4. Use of thisArg Parameter in forEach() Method
The “thisArg
” is considered as an optional argument in forEach()
method that enables you to define the value that will be utilized as “this
” when the callback function gets executed. By adding this argument, you can control the callback function context. This can assist in maintaining a particular scope when you are working with object methods.
Here, in the provided example, we have defined a msg()
function which accepts an “element
” parameter. In its function definition, the console.log()
method is utilized for concatenating “this.prefix
” and the element to the console.
Next, we have created a “numbers
” array and a “context
” object that contains a prefix property that is set to “'Number: '
“. Then comes the forEach()
method that is invoked on the numbers array and it takes the msg()
function and the context object as arguments.
function msg(element) { console.log(this.prefix + element); } const numbers = [11, 22, 33, 44, 55]; const context = { prefix: 'Number: ' }; numbers.forEach(msg, context);
The forEach()
method will iterate over the numbers array and execute the msg()
function for each element. As an output, the concatenation of the prefix property from the context object and the current element will be displayed on the console.
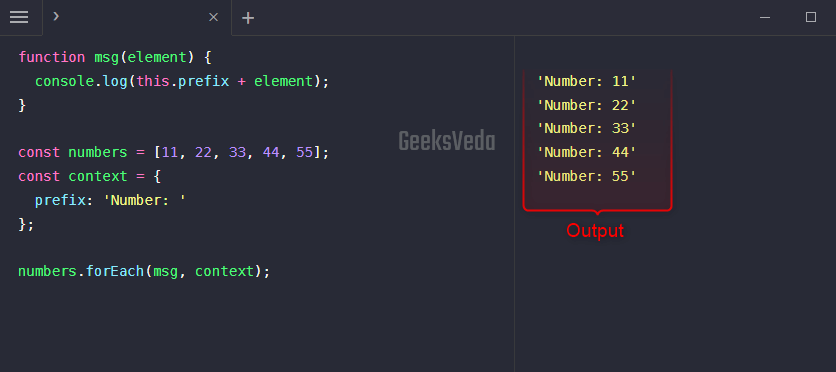
Now, let’s check out some practical applications of looping over an array using forEach()
in JavaScript.
Capitalize the First Letter of Each Word in Array Using forEach()
In this example, we will use the forEach()
method to iterate over an array of strings named “colors
” and modify its elements. The specified callback function will capitalize the first letter of each element by utilizing the “charAt()
” method to fetch the first character.
Then, the “toUpperCase()
” method converts it to uppercase. Moreover, the updated word is then assigned to the original array at the respective index.
const colors= ['blue', 'white', 'yellow']; colors.forEach((color, index, array) => { array[index] = color.charAt(0).toUpperCase() + color.slice(1); }); console.log(colors);
It can be observed that the first letter of each element has been capitalized successfully.
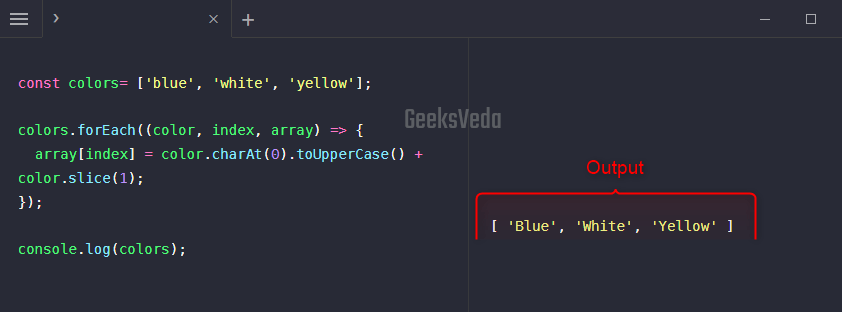
Update All Array Elements Using forEach()
Now, let’s have a look at the usage of the forEach()
method for iterating over an array of “numbers
“. The defined callback function will multiply each number by 2 and modify the value in the original array.
const numbers = [4, 9, 7, 1, 2, 5]; numbers.forEach((number, index, array) => { array[index] = number * 2; }); console.log(numbers);
As you can see, now the numbers array contains updated values.
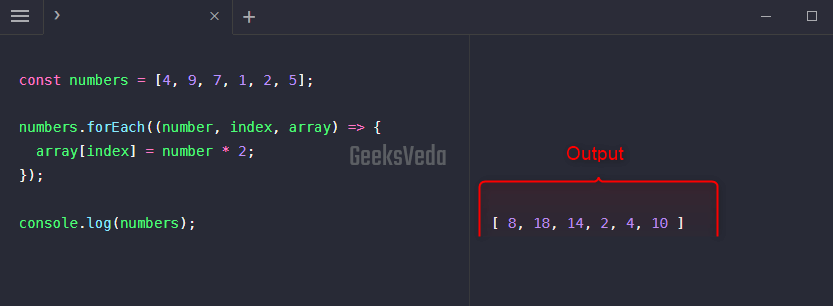
Filter and Extract Specific Array Elements With forEach()
The JavaScript forEach()
method also permits you to filter and extract particular array elements, For instance, in the provided example, the forEach()
method is used for iterating over an array of “numbers
“.
The added callback function checks if each number is divisible by 2 (which means it is even). If the specified condition is evaluated as true, the corresponding number will be added to the “evenNumbers
” array.
const numbers = [11, 12, 13, 14, 15, 16, 17, 18, 19, 20]; const evenNumbers = []; numbers.forEach((number) => { if (number % 2 === 0) { evenNumbers.push(number); } }); console.log(evenNumbers);
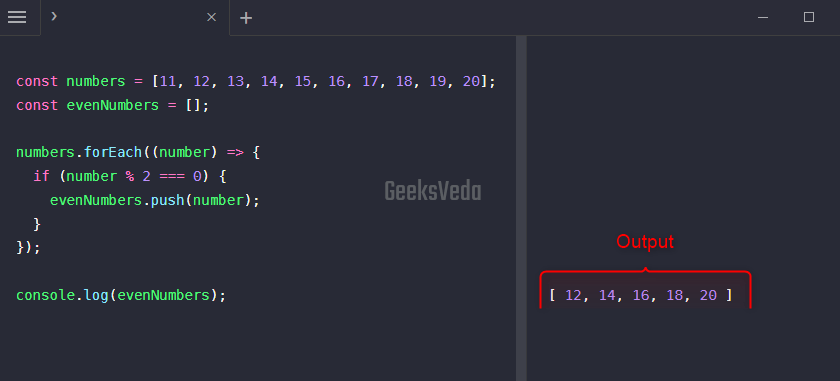
Loop Over a Two-Dimensional Array With forEach()
In JavaScript, the forEach()
method can be utilized for iterating over multidimensional arrays and enables you to perform operations on each element. For example, to iterate over a 2D array using forEach()
, you can nest multiple forEach()
methods as follows.
const matrix = [[11, 32], [23, 42], [95, 26]]; matrix.forEach((row) => { row.forEach((element) => { console.log(element); }); });
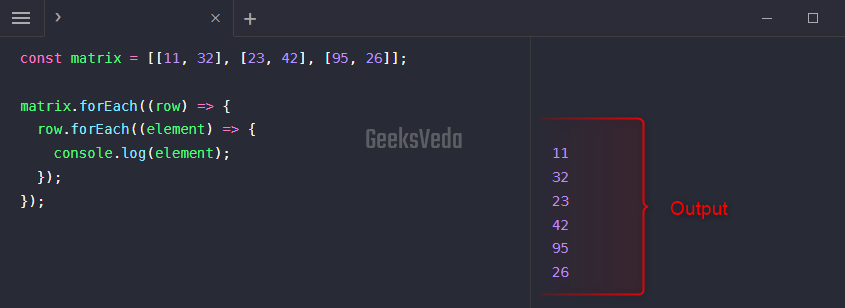
Best Practices to Loop Over an Array in JavaScript
Check out the provided best practices for looping over an array in JavaScript.
- Utilize the “
forEach()
” method for simple iteration. - During the iteration, avoid modifying the array if it is not required.
- Choose self-explanatory and meaningful parameter names for readability.
- Handle asynchronous operations correctly.
- Consider performance and select appropriate looping methods.
That’s all from this informative guide regarding the forEach()
method in JavaScript.
Conclusion
The forEach()
method in JavaScript offers a simple and effective way to iterate over array elements. It runs the provided callback function for each array element, permitting you to perform specified actions or operations on individual array elements.
By understanding the syntax and basic usage of the forEach()
method, including accessing the current element, index, and the entire array, you can master the operations related to data processing and array manipulation.