In JavaScript programming, effective variable declaration, and scoping need a deep understanding of their key differences. “var
” introduces block scope and avoids hoisting, whereas “let
” avoids hoisting and is utilized for declaring a function-scoped variable.
So, welcome to our guide exploring the key differences between the var
and let
keywords. Moreover, we will also provide the best practices regarding their usage.
Understanding Variable Scope in JavaScript
In JavaScript, variable scope represents the visibility and accessibility of variables in a certain part of a program. It identifies where the variables should exist and how long they are present. Additionally, having knowledge about the variable scope is important for writing bug-free code.
Types of Variable Scopes
Have a look at the enlisted types of variable scopes.
- Function Scope – Variables that are declared inside a function are referred to as function-scoped variables. These variables can be accessed only within the same function.
- Block Scope – Variables that are declared within a block are referred to as block-scoped variables.
- Global Scope – Variables that are declared outside of any block or function are known as global-scoped variables. After declaring globally scoped variables, you can access them anywhere in the JavaScript program.
Importance of Variable Scope
While programming in JavaScript, properly using the variable scope ensures that variables are limited to where they are required. This helps in minimizing the chances for unintended modifications and naming conflicts.
More specifically, it also assists in identifying and fixing bugs, resultantly improving code maintainability and readability.
Introducing “let” and “var” in JavaScript
This section will discuss the method of declaring variables using let
and var
keywords.
Define “let” as a block-scoped Variable Declaration
In ES6, the keyword “let
” was introduced which permits the declaration of the block-scoped variables. More specifically, variables that are declared by utilizing the let keyword can be only accessed within the block in which they are created.
In this example, the “num
” variable is limited to the added “if
” block, and accessing it outside the block will resultantly throw a ReferenceError.
function msg() { if (true) { let num = 50; console.log(num); // Output: 50 } console.log(num); // Throws an error: } msg();
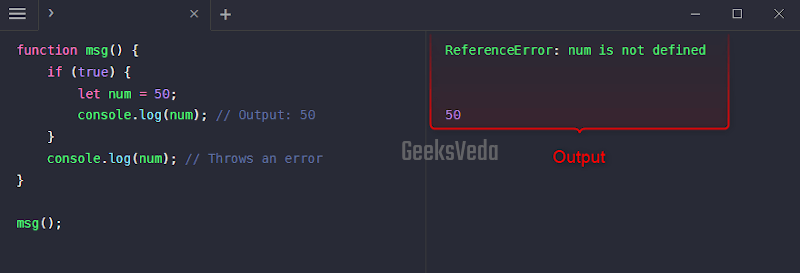
Define “var” as a Function-Scoped Variable Declaration
Prior to the introduction of let
, the “var
” is the keyword utilized for declaring the function-scoped variables.
When you declare variables using it, they have function scope and can be only accessed within the function in which they are declared.
Here, in the provided example, the declared variable “num
” is a function-scoped variable. Therefore, it can be accessed both outside and inside of the added “if
” block.
function msg() { if (true) { var num = 50; console.log(num); // Output: 50 } console.log(num); // Output: 50 } msg();
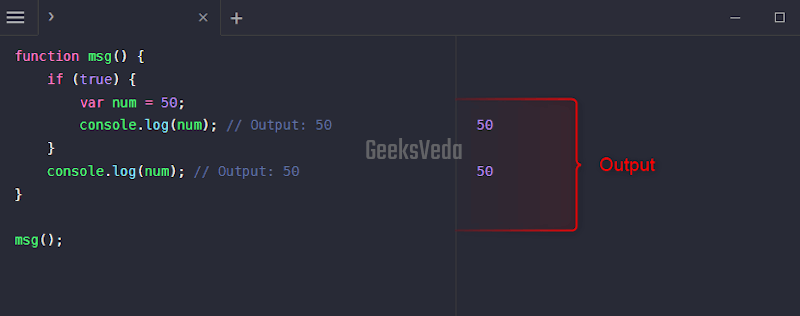
Now, let’s talk about the key differences between var and let in JavaScript, one by one.
Difference Between “let” and “var” Scope
As discussed previously, the variable has a block scope if they are declared by utilizing the “let
” keyword. This restricts them to the block in which they are declared.
More specifically, block scope signifies the representation of curly braces pair { }
, such as within a loop, or if statement.
Remember that, you cannot access the let variable outside of their block.
if (true) { let num = 40; console.log(num); // Output: 40 } console.log(num); // Throws an error
It can be observed that accessing the “num
” variable outside of the block displayed the respective ReferenceError.
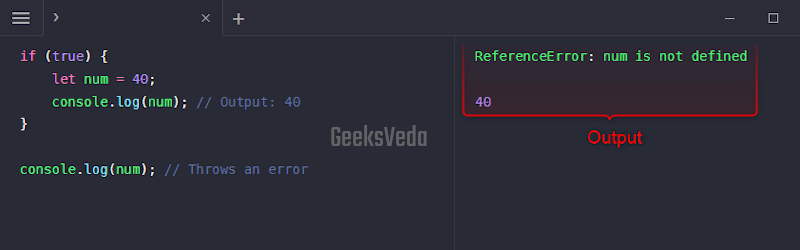
In the case of declaring a variable with the “var
” keyword, you can access this variable anywhere in the whole function.
function msg() { if (true) { var num = 40; console.log(num); // Output: 40 } console.log(num); // Output: 40 } msg();
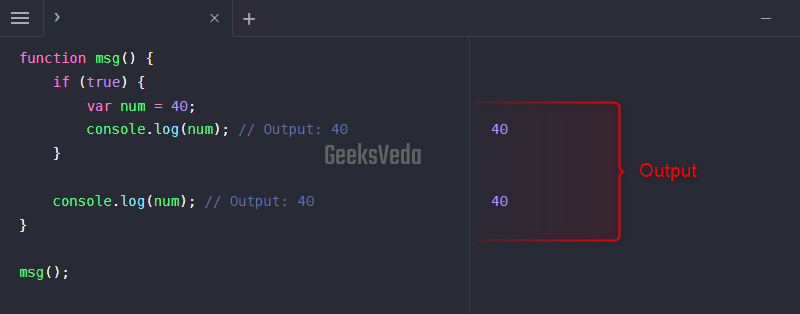
Hoisting Difference in “let” and “var” Variable
JavaScript does not allow hoisting (the behavior of shifting all declarations to the top of the current scope) the variable declared with the “let
” keyword to the top of their scope.
More specifically, these variables are placed in a temporal dead zone until the execution control reaches the point where they are declared.
So, accessing a let
variable before its declaration will throw a ReferenceError as follows.
console.log(num); // Throws an error
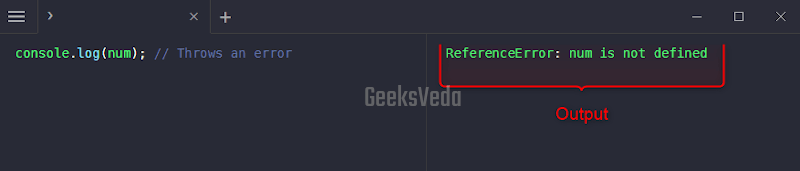
So, to display the variable value, declare it first and then access it.
let num = 30; console.log(num); // Output: 30
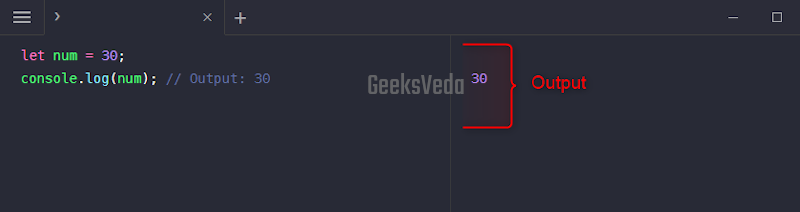
In the other case, JavaScript hoists the variable at the top of the relevant scope during the creation phase of the execution context, if they are declared with the “var
” keyword.
This indicates that it does not matter where you have declared the variable, it is going to be accessible throughout the whole scope.
console.log(num); // Output: undefined var num = 40; console.log(num); // Output: 40
In the output of the given code, the “num
” will be accessed but as it is not defined yet, therefore its value will be stated as “undefined
“.
After declaring the variable, the next statement will print its value to the console.
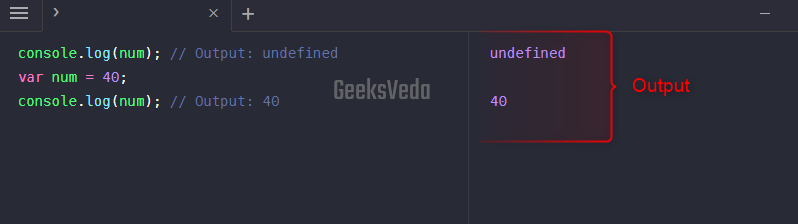
Difference Between “let” and “var” When Reassigning and Redeclaring
In JavaScript, you can reassign a new value to the variable declared with the “let
” keyword, within the same block like this.
let num = 20; num = 80; // Reassignment is allowed console.log(num); // Output: 80
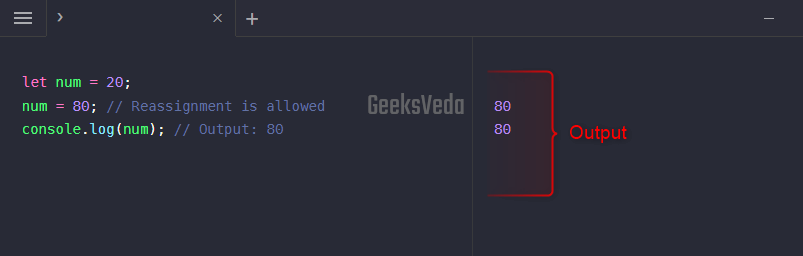
However, if you try to redeclare a new value to the variable and within the same block will the syntaxError is given in the output of the provided code.
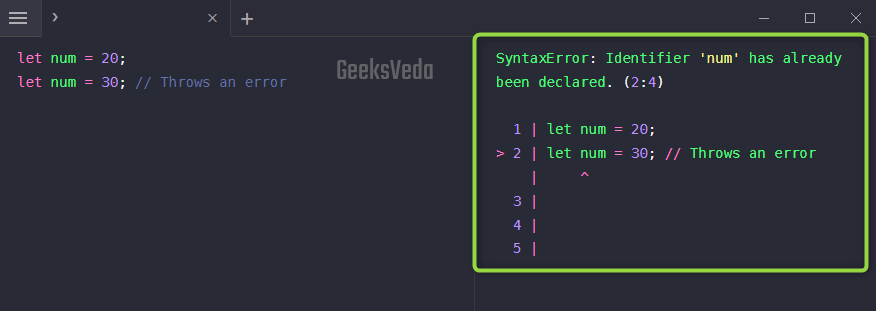
On the other hand, reassignment, and redeclaration are allowed for the variables declared with the “var
” keyword.
The mentioned operations can be performed within the same scope without any issues.
var num = 60; num = 20; // Reassignment is allowed console.log(num); // Output: 20 var num = 909; // Redeclaration is allowed console.log(num); // Output: 909
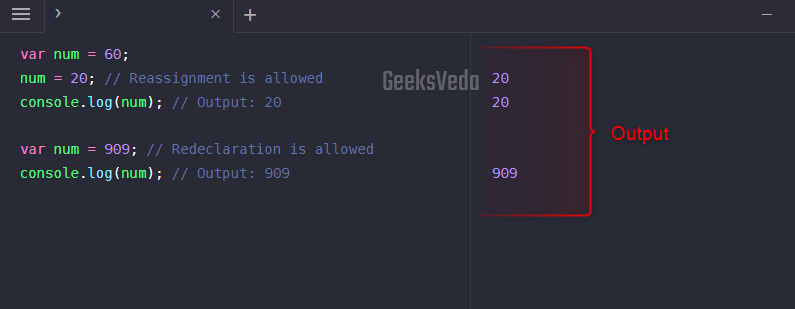
It can be seen in the given output that the value of the “num
” variable has been reassigned and this variable is also redeclared later without any issue.
Comparison Table “var” vs “let”
Let’s check out the provided table, to sum up the discussed key differences between “var
” and “let
” keywords in JavaScript.
Feature | “let” | “var” |
Compatibility | Introduced in ES6 | Available in older JavaScript older versions |
Scope | Block Scope | Function Scope |
Hoisting | No Hoisting | Hoisted at the top of its scope |
Temporal Dead Zone | Yes | No |
Redeclaration | Not allowed (within the same block) | Allowed (within the same block) |
Reassignment | Allowed (within the same block) | Allowed (within the same block) |
Best Practices for Using “var” and “let” in JavaScript
Check out the given best practices before jumping into the variable declaration in JavaScript.
-
- For block-scoped variables, prefer using “let” over “
var
“. - Utilize “
let
” for loop counters to restrict the unintended leakage of the variable. - Use “
var
” only when it is required to declare a function-scoped variable. - Get benefits from the temporal dead zone by using the let keyword. This also assists in catching potential errors.
- Follow a consistent variable declaration style.
- Select the appropriate keyword based on the scope and requirements of your JavaScript program.
- For block-scoped variables, prefer using “let” over “
Conclusion
Being a JavaScript programmer, it is essential for you to know the difference between the “var
” and “let
” keywords.
Shortly stated, “var
” declared function-scoped variable, allowed to be hoisted and is compatible with other JavaScript versions. Whereas, “let
” declares a block-scoped variable, avoids hoisting, and is introduced in ES6.
Moreover, by following this guide, you can write simple, easy-to-maintain JavaScript code with proper variable scoping.