Are you having trouble getting your JavaScript code to iterate over large datasets? If yes, then the JavaScript for loop is the only thing you need. By utilizing the JavaScript for loop, you can iterate over each array element or the key-value pair of an object.
So, follow our post to get to know about the working of for loop in JavaScript programming language. Moreover, you can learn how to use the break and continue statements to control the for loop’s execution and look at practical applications like filtering out odd numbers or locating the first even number in an array and printing it on the console.
JavaScript for Loop
A JavaScript for loop permits the execution of a code block repeatedly for a specified number of a time or until the defined condition is evaluated as true. It is one of the most commonly utilized loop statements used in combination with arrays for iterating over each element.
Follow the provided syntax for using the JavaScript for loop:
for (expression 1; expression 2; expression 3) { // code block to be executed }
Here:
- The expression 1 is utilized for initializing variables and executed before the loop starts.
- The expression 2 refers to the condition that is checked or verified before each iteration of the loop. If the defined condition is met, the loop will continue to execute. In the other case, the loop will terminate.
- Lastly, expression 3 runs after each iteration and updates the values of the variables.
How JavaScript for Loop Works
Now, let’s discuss the working of for loop in JavaScript with respect to the added flowchart:
- The expression or the initializer executes before the loop starts. It can be utilized for initializing the loop variable. You can add any valid JavaScript expression as an initializer variable. However, it should initialize a loop counter variable.
- Then, the condition is evaluated or checked before each iteration of the loop. As stated earlier, if the condition is met, the loop continues, otherwise, it ends.
- The iterator is executed after each iteration of the loop and it updates the variable. This iterator can be any valid expression utilized to increment or decrement the loop counter variable.
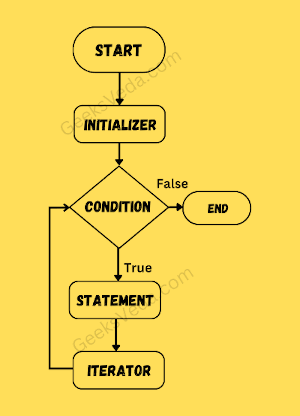
Now, have a look at the given practical examples of using for loop in JavaScript.
1. How to Loop with Increment Iterator in JavaScript
In this example, we have initialized a for loop
with the variable “i”
set to 0. This loop will continue to run until the value of i
is less than 6. After each iteration of the loop, the variable’s value will be incremented by 1 because of the added iterator “i++”
.
for (let i = 0; i < 6; i++) { console.log(i); }
Note that, for each iteration, the console.log()
method will print out the current value of i
variable on the console. This signifies that the numbers starting from 0 to 5 will be displayed, one at a time.
However, when the value of i
variable becomes 6, the specified condition “i < 6”
is no longer true and the loop will terminate.
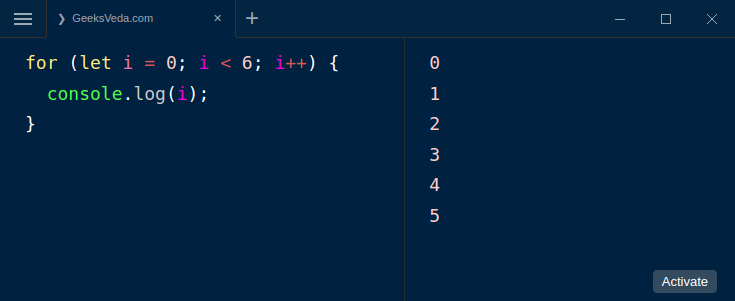
2. How to Loop with Decrement Iterator in JavaScript
At the first glance, this example may seem like the previous one. However, if you carefully check it out, all of the three added expressions are different.
Here, the for loop
starts from 6 and iterates backward because of the added decrement iterator "i--"
. For each iteration, the console.log()
prints out the value of the variable i
like 6, 5, 4, 3, 2, 1, to the console, until it reaches 0, i.e., "i > 0"
. At this point, the for loop will get terminated.
for (let i = 6; i > 0; i--) { console.log(i); }
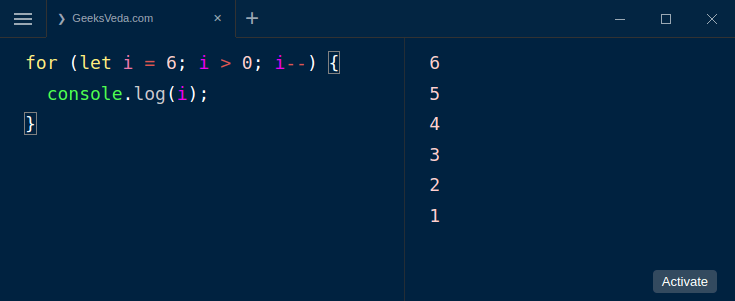
3. How to Loop Through an Array in JavaScript
In JavaScript, the for loop
can be also utilized for iterating over arrays and performing the required operations on their elements. In this section, we will explore how to use JavaScript for loop to iterate over an array of integers and calculate their sum.
Moreover, the second example will discuss the usage of for loop for iterating over an array of strings and changing their case on each iteration.
Example 3.1: Calculate the Sum of the Array Elements
First of all, we have defined an array of five elements. Then, the sum
variable is initialized with 0. After that, we have defined a for loop
that will iterate over the number array by utilizing the loop variable i
.
During each for loop
iteration, the current element value numbers[i]
will be added to the sum. In the end, when the for loop completes its iteration, the final value of the sum variable will be displayed on the console.
const numbers = [2, 4, 6, 8, 10]; let sum = 0; for (let i = 0; i < numbers.length; i++) { sum += numbers[i]; } console.log(sum);
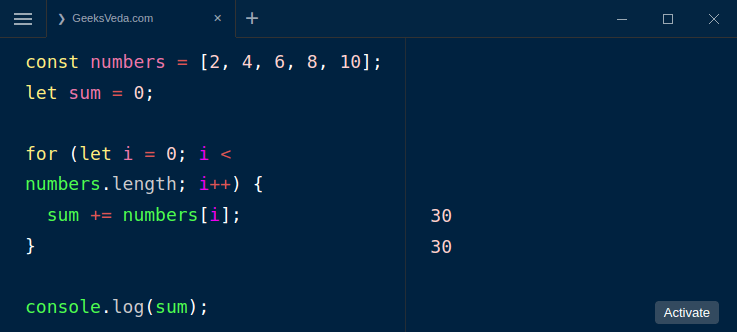
Example 3.2: Convert Array Elements to Uppercase in JavaScript
In this example, let’s iterate an array of strings and change the case of the relevant element. To do so, first, define an array named fruits having three elements. Next, add a for loop to iterate over the fruits array with the help of the variable i
.
For each iteration, the toUpperCase()
method will be invoked and it will convert the current element fruits[i]
to uppercase. Lastly, when the for loop termites, the updated fruits array will be printed out on the console having values in uppercase.
const fruits = ['Cherry', 'Mango', 'Plum']; for (let i = 0; i < fruits.length; i++) { fruits[i] = fruits[i].toUpperCase(); } console.log(fruits);
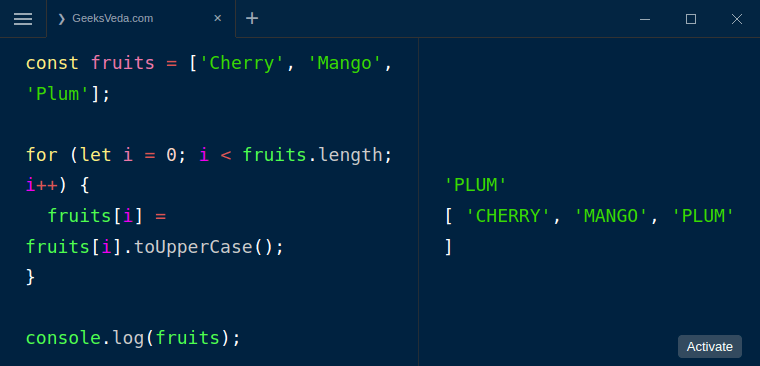
4. How to Loop Through an Object in JavaScript?
JavaScript for loop also permits you to loop through objects and modify the required properties. Want to explore this approach? Have a look at the provided examples.
Example 4.1: Loop Through Objects Keys and Values in Javascript
The example demonstrates the usage of the for loop
to print the keys and the values of the properties to the console.
First of all, an object named a person has been defined as having the given three properties. Next, a for loop is added to iterate over the created object with the help of the key variable.
For each iteration, the console.log()
method will output the current key-value pair of the object to the console with the help of the template literals "${}"
.
const person = { name: 'Sharqa', age: 25, email: 'sharqa@geeksveda.com' }; for (let key in person) { console.log(`${key}: ${person[key]}`); }
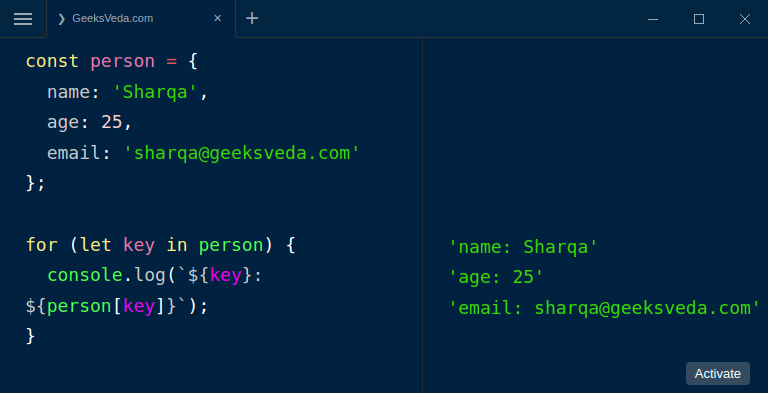
Example 4.2: Modifying Object Properties in JavaScript
Now, let’s head towards the method to modify the object properties. To do so, we have used the already created object name person. Then, a new empty object named newPerson is defined. After that, we have utilized the for loop for iterating over the person object using the key variable.
Likewise, during each iteration, the toUpperCase()
method will convert the key to uppercase and the corresponding property value will be assigned to the new object.
At the end of the iteration, the newPerson object will be output to the console.
const person = { name: 'Sharqa', age: 25, email: 'sharqa@geeksveda.com' }; const newPerson = {}; for (let key in person) { newPerson[key.toUpperCase()] = person[key]; } console.log(newPerson);
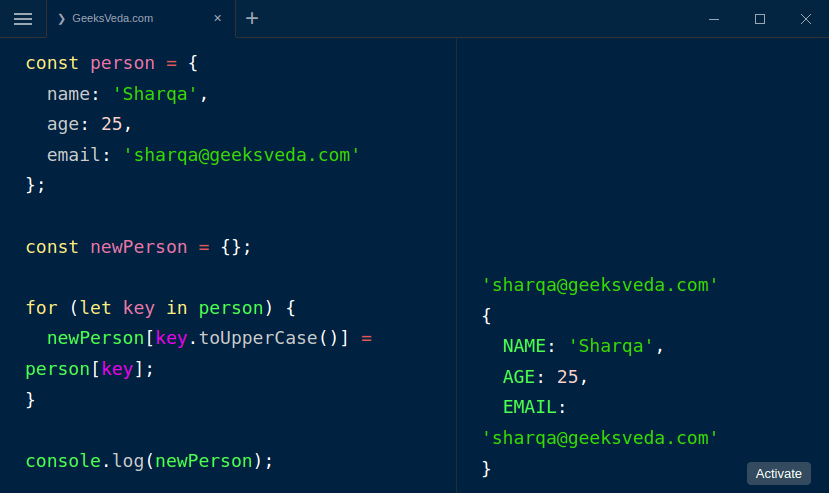
5. How to Use Nesting For Loops in JavaScript
A loop that is placed within a loop is known as a nested loop. In JavaScript, you can utilize a for loop for iterating over a two-dimensional or a matrix. More specifically, in outer for loop iteration, the inner for loop will run completely.
For instance, in the below-given code, we have defined a nested loop for printing multiplication tables from 1 to 10. For each iteration of the outer for loop, the inner for loop will execute from 1 to 10 and also multiply the current value of the outer loop with the inner loop at the current loop “${i * j}”
.
Lastly, the result will be printed out to the console by utilizing the string interpolation.
for (let i = 1; i <= 10; i++) { for (let j = 1; j <= 10; j++) { console.log(`${i} x ${j} = ${i * j}`); } }
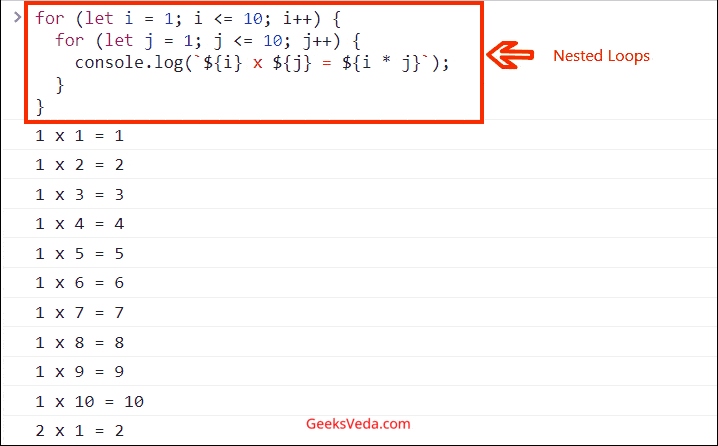
6. How to Use JavaScript with Break Statement
In JavaScript, the break statement is often utilized for terminating the loop before its natural end. More specifically, it can be used with a for loop to search for a specific value or with the defined condition within the list or array.
In the following example, the for loop will iterate through the created array of numbers. Inside this loop, an if
statement verifies if the current element is even by utilizing the modulo operator as “number[i] % 2 === 0”
.
If the number is found as even, it will be displayed on the console and the loop will terminate because of the break statement.
const numbers = [1, 3, 5, 4, 7, 8, 9, 12, 10]; for (let i = 0; i < numbers.length; i++) { if (numbers[i] % 2 === 0) { console.log(numbers[i]); break; } }
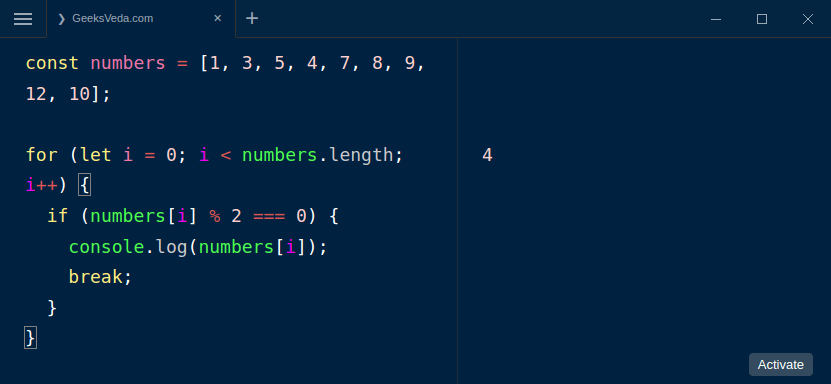
7. How to Use JavaScript with Continue Statement
The continue statement in a for loop is used for skipping the next iteration if the given condition is evaluated as true.
For instance, in the provided example, we have added a for loop that iterates through the numbers array. If the current number is odd, the for loop skips the next iteration due to the defined continue statement. However, if the current number is even, it will be shown on the console.
const numbers = [1, 3, 5, 4, 7, 8, 9, 12, 10]; for (let i = 0; i < numbers.length; i++) { if (numbers[i] % 2 !== 0) { continue; } console.log(numbers[i]); }
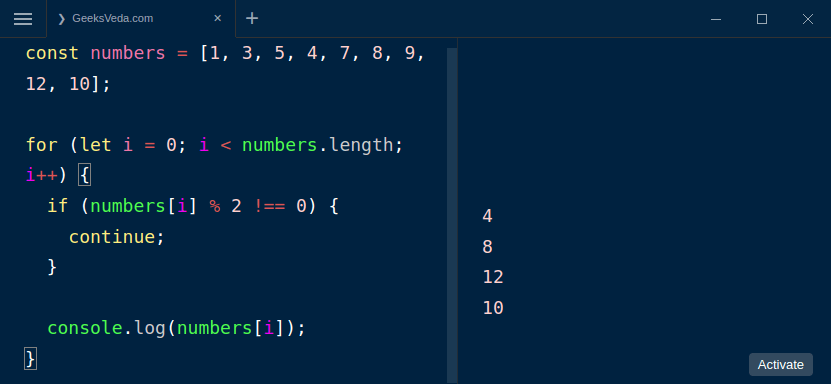
That’s how you can use a for loop in different scenarios.
Conclusion
Every developer should master the JavaScript for loop, which is a fundamental programming concept. The for loop is a powerful and flexible approach to iterate through objects, arrays, or a combination of both.
Keep exploring how you can utilize the for loop to optimize your code and address practical issues by experimenting with the examples we covered, such as filtering out odd integers or accessing object keys.
Want to learn more related to JavaScript, check out our dedicated JavaScript series!