Till now, we have discussed, if…else statements, for loop, while loop, and break and continue statements.
So, as the last blog of our Python control flow series, we will explore the “pass
” statement.
In this article, we will guide you on how the pass
statement can be used as a placeholder and assist you in controlling the flow of your code.
So, learn its syntax, and usage in multiple concepts like class definitions, function definitions, conditional statements, placeholder functions, and exception handling.
What is pass Statement in Python
In Python, “pass
” is a null statement that does not perform any kind of action or operation. It can be utilized as a placeholder when a statement is syntactically needed, but you do not want any code at that point.
Additionally, the pass
statement enables you to skip over code sections without encountering any syntax errors.
How to Use pass Statement in Python
In order to use a pass
statement in code, you have to follow specific syntax which is pretty straightforward. For this add the keyword “pass
” followed by the colon “:
“.
Now, let’s look at some use cases of the pass statement such as, in function definitions, loops, and conditional statements.
1. Using pass Statement in Function Definitions
The “pass
” statement is primarily utilized as a placeholder in the function definitions. It permits you to create empty functions that can be implemented later with the required actual code.
This approach can be helpful when you want to define a function structure but have not thought about its implementation.
In the provided example, “my_function()
” has been defined with the pass
statement which signifies that this function does not comprise any code yet and serves as a placeholder only.
On the other hand, the “calculate_average()
” function shows the method to use a pass
statement within the if condition of a function definition.
In this scenario, if the “numbers
” list has been found empty, the pass statement will be utilized as a placeholder for handling the case later. Else, the code calculates and prints the average of the numbers list.
def my_function(): pass def calculate_average(numbers): if len(numbers) == 0: pass # Placeholder for handling empty list case else: total = sum(numbers) average = total / len(numbers) return average # Example usage my_function() number_list = [53, 15, 32, 29] result = calculate_average(number_list) print("Average:", result)
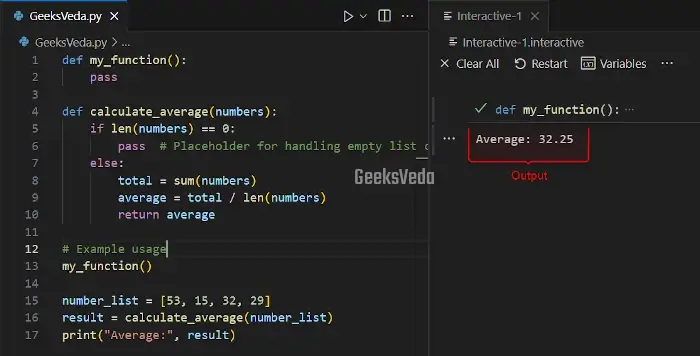
2. Using pass Statement in for loop
You can also utilize the pass
statements within loops
, like for loops
and while loops
for creating empty loops without any code execution.
It can be found useful in situations where you want to define the loop structure but do not have the code to be executed as a whole or for a particular section.
Now, in the provided example, we have utilized the “pass
” statement for skipping the “blue
” element processing. The for loop
would still continue to the next iteration without executing any code.
colors = ["pink", "blue", "black"] for color in colors: if color == "blue": pass # Skipping the processing of the "blue" element else: print(color)
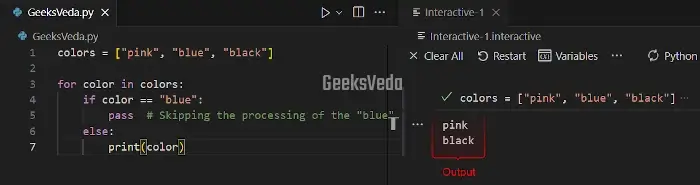
3. Using pass Statement in while loop
Here, the “pass
” statement skips the processing when the value of the “counter
” variable becomes “2
“. The loop still continues the execution to the next iteration without running any code.
counter = 0 while counter < 10: if counter == 2: pass # Skipping the processing when counter is 2 else: print(counter) counter += 1
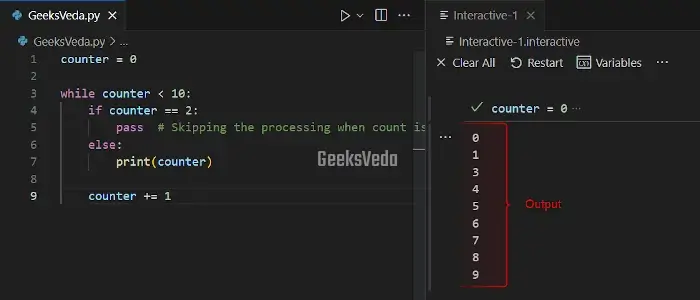
4. Using pass Statement in Conditional Statement
The “pass
” statement can be used with a conditional statement like the “if
” statement for skipping over a specific condition without running any code.
This approach can be utilized in scenarios when you are working on the code structure and want to handle the desired condition later.
For instance, in the provided code, the “pass
” statement behaves as a placeholder for handling the condition where “x
” is greater than five. It permits you to leave that specific section empty for implementation later.
x = 20 if x > 5: pass # Placeholder for handling the condition when x > 5 else: print("x is less than or equal to 5")
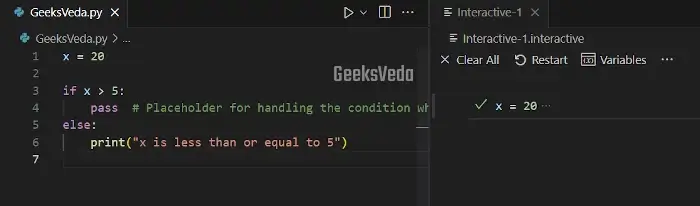
Advanced Usage of Python pass Statement
In this section, we will discuss some of the advanced usage of pass statements in Python, like placeholder functions, class definitions, and in exception handling.
1. Using pass Statement in Placeholder Functions
While programming in Python, sometimes, it is required to define a function that needs to be implemented later. In such a case, utilize the “pass
” statement as a placeholder. It enables you to define a function without writing code inside the function body.
For instance, in the “placeholder_function()
“, the pass statement is now acting as a placeholder for the function body. This signifies that the implementation will be added later on.
def placeholder_function(): pass # Placeholder function with no implementation placeholder_function()
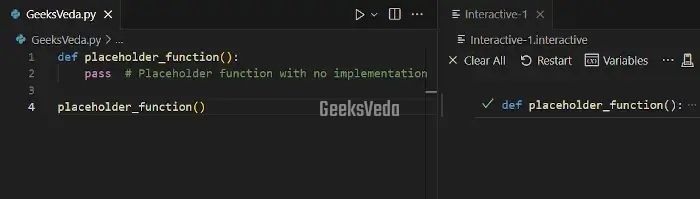
2. Using pass Statement in Class Definitions
Likewise, you can add a “pass” statement in the class definitions for creating an empty class that can be filled later with the attributes and methods.
Here, “MyClass
” has only a pass statement added as a placeholder for the class body. It permits you to only define the class structure before adding any attributes and methods.
class MyClass: pass # Empty class with no attributes or methods my_obj = MyClass()
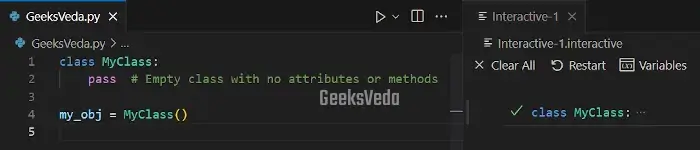
3. Using pass Statement in Exception Handling
You can also utilize a “pass” statement in exception handling when it is needed to catch and handle certain exceptions, but it is not required to run any code in the exception block.
For example, here the two pass statements have been added in try and except block separately. This means that no particular code execution is needed for the exception handling.
try: # Code that may raise an exception pass except Exception: # Exception handling code pass
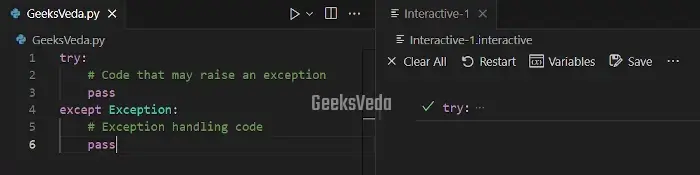
The pass Statement vs break/continue Statements
Have a look at the provided table to compare the pass
statement with the break
and continue
statement in terms of its functionalities.
pass Statement | break Statement | continue Statement | |
Use | Placeholder | Exit loop | Skip iteration |
Purpose | No action | Terminate loop | Skip Iteration |
Applicable to | Any code block | Mainly loops (for, while) | Mainly loops (while, for) |
Flow of Control | No change | Exit loop | Skip to the next iteration |
Nesting | Independent | Breaks the inner loop | Skips the current iteration |
Relationship | Standalone | Loop-dependent | loop-dependent |
Alternatives of pass Statements in Python
In some scenarios, the use of the “pass” statement may not seem the most suitable approach. So, depending on the case, you can consider its alternatives such as using comments, a function that does nothing, or raising a “NotImplementedError
“.
Each of these alternatives will be now explained individually,
1. Using a Comment as pass Statement
In this example, the pass statement is serving as a placeholder and the added comment offers a remainder for implementing the logic. This approach enables the easy identification of pending tasks.
# Alternative using a comment def placeholder_function(): # TODO: Implement function logic pass
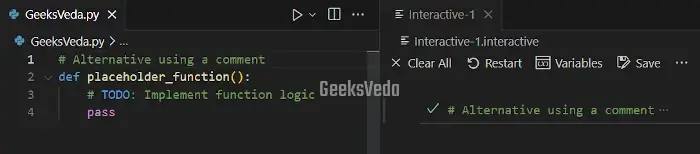
2. Using a Function as pass Statement
We have now defined a function “do_nothing()
” that does not comprise any code and simply returns without performing any operation. It serves as a placeholder function whose implementation can be added when required.
# Alternative using a function that does nothing def do_nothing(): return
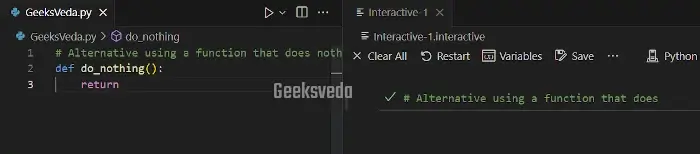
3. Using Raise as pass Statement
You can also utilize a “raise” statement for raising the “NotImplementedError
“. This exception is commonly used for indicating that method functionality has not been implemented yet.
# Alternative using a raise statement def placeholder_function(): raise NotImplementedError
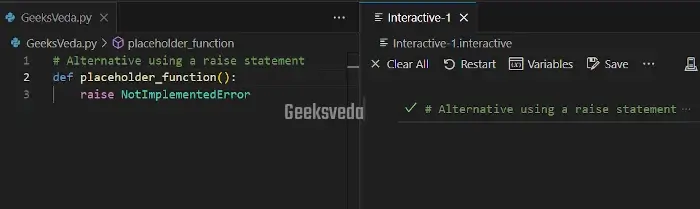
That was all from this effective guide related to the usage of Python pass statements.
Conclusion
Python’s “pass
” statement is a versatile tool that can be used for controlling code flow and handling placeholders. It permits you to skip code blocks without encountering any errors.
In today’s guide, we have explored the usage of pass statements in function definitions, loops, conditional statements, class definitions, placeholder functions, and exception handling. Moreover, we have provided alternatives for you!
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!