Do you want to effectively handle decision-making in your Python code? If yes, the “if…else
” statement can assist you in this regard. This robust construct permits your program to respond and adapt according to the specified conditions, which ultimately opens up endless possibilities.
In today’s guide, we will explore if…else
statements, their syntax, usage, the need for elif statements, the concept of conditional branching, and multiple or nested if statements. So, keep reading!
What is Python if…else Statement
In Python, the “if…else
” statement is a fundamental control flow statement that permits the program to make decisions according to certain conditions.
With the help of the if…else
statement, you can define a code block that needs to run if the condition is met or is evaluated as true. Alternatively, the code added in the else block will run.
How Does Python if…else Statement Work
Here is the syntax you need to follow for utilizing the Python if…else statement.
if condition: # statements to run if the condition is true else: # statements to run if the condition is false
As stated above, the if…else statement
works by evaluating a condition and running the suitable code block based on the evaluation results. This condition is defined within the parentheses after adding the “if
” keyword.
Then, if the added condition is true, the indented code block of the “if
” statement will run. Otherwise, the “else
” code block will run.
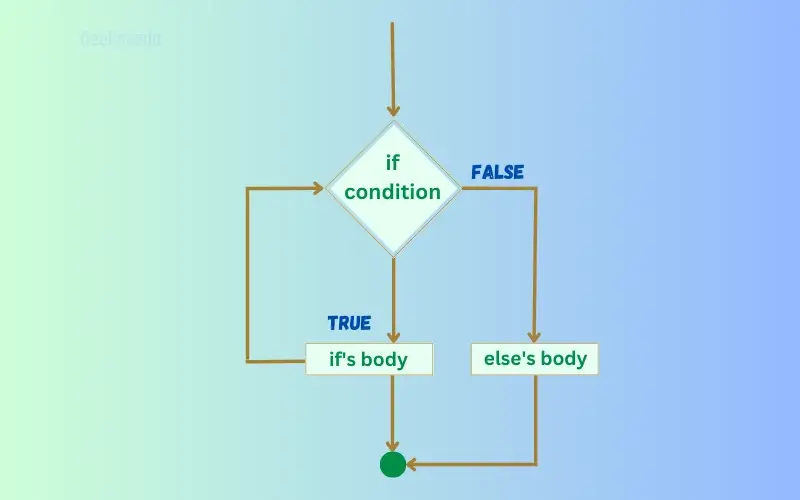
Have a look at some practical examples of utilizing the if…else statement in Python.
Check Age Eligibility
For instance, we have used the “if…else
” statement in the provided program for checking the age variable value. The condition is defined as “age >= 18
“. If it is evaluated as true, then, the if code block will print the added message. Otherwise, the else block will execute.
age = 18 if age >= 18: print("You are an Adult.") else: print("You are a Teenager")
As the value of the age variable is “18
“, the if code block performed the added functionality.
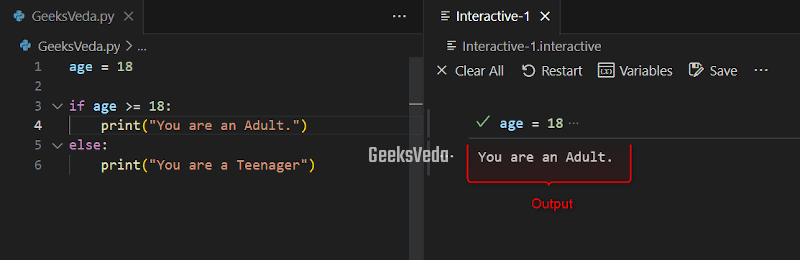
Check if the Number is Odd or Even
In the second example, we have defined the condition as “number % 2 == 0
“, which verifies if the remainder of the “number
” divided by 2 is equal to zero or we can say that the number is divisible by 2.
If yes, the number will be considered as even as given in the if code block. In the other case, it is evaluated as an odd number.
number = 9 if number % 2 == 0: print("It is an even number.") else: print("It is an odd number.")
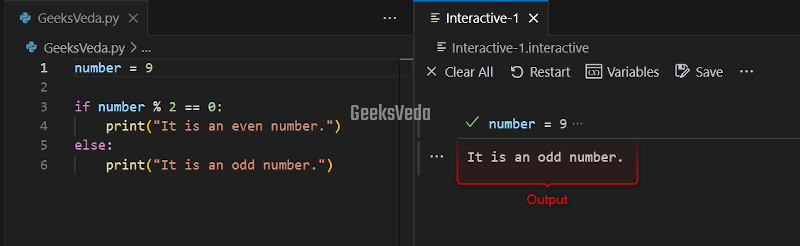
Check if the Number is Positive
Check out the provided example, in which the condition “x > 0
” will be evaluated. If the value of the given “x
” variable is greater than zero, the print() function will display the message that the number is positive.
Alternatively, the else code block will run, and the value of the “x
” variable will be declared as non-positive.
x = 4 if x > 0: print("It is a positive number.") else: print("It is a negative number.")
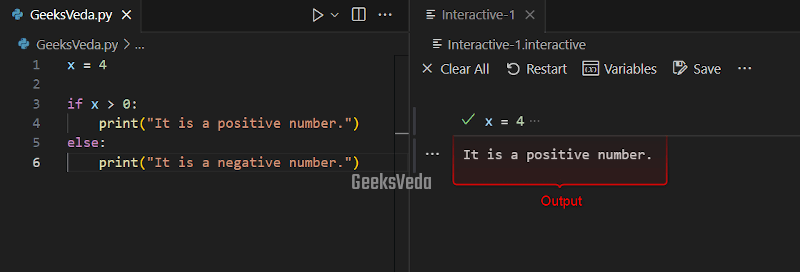
Importance of Indentation in if…else Statement in Python
In Python, indentation is essential for determining the hierarchy and grouping of the statement within the program. More specifically, in the if…else
statement, the proper indentation should be followed for the correct code execution.
Here, in the given code, we have utilized the indentation for distinguishing between the “if
” and “else
” code blocks.
Carefully observe the print() functions that have been involved in the respective code blocks. These functions will be executed according to the evaluation of the condition.
x = 5 if x > 0: print("The number is positive.") print("It's greater than 0.") else: print("The number is non-positive.") print("It's less than or equal to 0.")
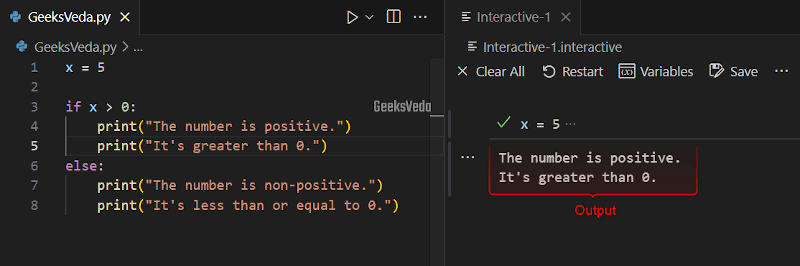
Conditional Branching with Python if…else Statement
Conditional branching enables a program to make decisions and run different code blocks based on the defined conditions. It allows the program to modify its behavior dynamically, create complex decision-making structures, and respond to varying inputs.
More specifically, you can use the if…else statements, ternary operators, and switch statements in your program.
For instance, here, we have used the if…else
statements for applying conditional branching. This program takes a “y
” as a year value from the user as input and determines whether it is a leap year or not.
It utilizes multiple if…else
statements to check the divisibility of the “y
” variable value by 4, 100, and 400. After that, based on the evaluation, the program displays the defined message on the terminal.
y= int(input("Enter a year: ")) if y % 4 == 0: if y % 100 == 0: if y % 400 == 0: print(y, "is a leap year.") else: print(y, "is not a leap year.") else: print(y, "is a leap year.") else: print(y, "is not a leap year.")
In this scenario, we have entered “1998
” which is not a leap year.
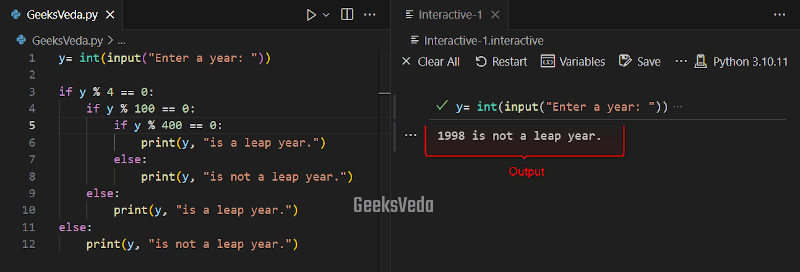
Python Nested if Statements
Continuing the flow of the previous section, multiple or nested if statements are the specific implementation of conditional branching. In this approach, an if…else
statement is placed within the code of another if…else
statement.
This enables more complex decision-making structures by defining conditions based on the outcome of the previous conditions.
Check the Sign of Input Number
In the provided code, we have utilized the nested if statement for verifying the sign of the input number. In case, if the number is non-negative, the inner if statement again checks if the number is zero or positive.
After the evaluation, the corresponding message has been displayed on the console.
num = int(input("Enter a number: ")) if num >= 0: if num == 0: print("Entered number is zero.") else: print("Entered number is positive.") else: print("Entered number is negative.")
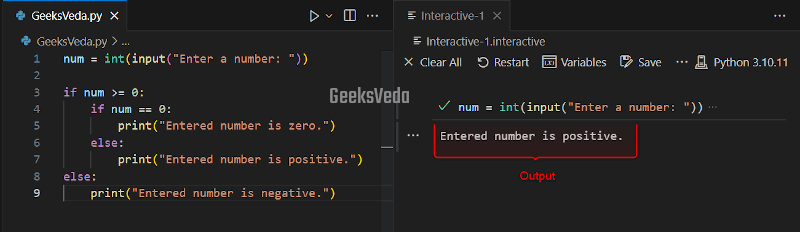
Python elif Statements
While programming in Python, you may have encountered situations where it is required to test multiple conditions and run different code blocks based on the evaluation.
In such scenarios, it is ideally not possible to cover all cases only using the if…else
statements as they can handle only two cases. So, here comes the “elif
” statement to rescue you.
The “elif
” or “else if
” statement permits you to handle or take care of multiple conditions sequentially after the starting “if
” statement.
It offers an alternative condition set for verifying if the condition specified in the “if
” statement is false.
You can chain multiple conditions together and run the respective code block of the first true condition.
Check Multiple Conditions Using elif Statements
Here, in this example, an “elif
” statement is added for checking the multiple conditions. If the “number
” is evaluated as greater than zero, it is considered positive. Else if, it is equal to zero, then the number is zero, else it is negative.
num = 10 if num > 0: print("The number is positive.") elif num == 0: print("The number is zero.") else: print("The number is negative.")
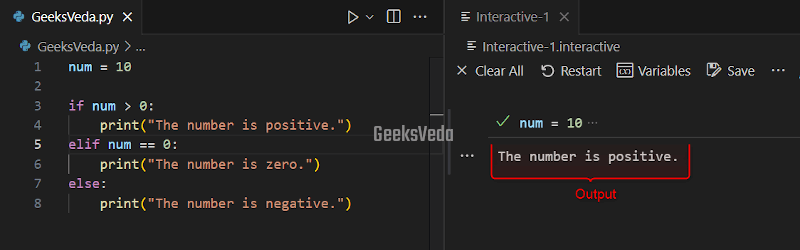
That’s all from this effective guide related to Python if…else statement.
Conclusion
Python if…else statement allows applying conditional branching, enabling programs to run different code blocks based on the added conditions.
Moreover, by utilizing the nested if statements and elif statements, you can handle multiple or several conditions and create complex-decision making structures in your Python project.
Learning these concepts will empower you to create interactive and flexible programs that have the capability to adapt according to the given scenarios.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!