Being one of the most popular programming languages in this modern technological world, Python offers a wide range of tools and features for programmers. One of its most essential features is its support for functions, which permits the developers to write reusable and efficient code.
In an earlier article, we discussed Python Operators, in this blog, we will explore the different types of Python functions, such as built-in, user-defined, lambda, recursive, and decorator functions, and their importance in creating robust and effective programs.
What are Python Functions
Python functions are defining or organize code in a modular or reusable way. These are referred to as the code blocks that perform a set of tasks or a specific operation. You can call functions from other parts of the function whenever required.
Moreover, functions in Python can accept input, or arguments, and return outputs or values. This also makes them robust and flexible tools for manipulating and organizing data in a Python program.
How to Define a Python Function
In Python, the “def” keyword is used for defining functions. This keyword is followed by the function name and the parameters that must be enclosed in the parenthesis.
Next comes the body of the function that will be indented and comprises the code that needs to be executed when the function is invoked or called with the arguments.
Follow the provided syntax for defining a function in Python.
def functionName(param1, param2): # function body return result functionName(value1, value2)
According to the given syntax:-
- “functionName” represents the name of the Python function.
- “param1” and “param2” indicates the function parameters that will store the values “value1” and “value2” as the passed arguments.
- “function body” refers to the statement that needs to be executed.
- The “return” statement will return or output the resultant value after performing the required operation added in the function body.
- Lastly, “functionName(value1, value2)” is the call to the defined function while passing the desired values as arguments.
Advantages of Using Python Functions
Check out the enlisted advantages of using functions in Python.
- Functions are the reusable part of the code that can be invoked from any part of the program.
- They also assist in improving code efficiency by minimizing the need for redundant or repetitive code.
- Functions make code more readable and easier to use by hiding complex operations behind a simple interface.
- They help in breaking down the large program into smaller, easy-to-understand chunks or code blocks.
- Moreover, functions can be easily tested in isolation, which plays a major role in identifying and fixing code bugs.
What are the Different Types of Python Functions
Here are some of the major types of functions in Python:
- Built-in Functions
- User-defined Functions
- Lambda Functions
- Recursion or Recursive Functions
- Decoration or Decorative Function
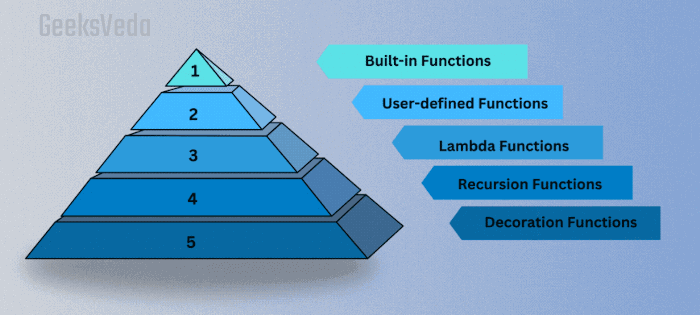
Let’s check out each of the mentioned types with the help of practical examples.
1. Built-in Python Functions
Built-in functions are part of the core Python languages. These functions can be used freely without the requirements for additional import of installation. More specifically, the Python standard library includes these functions.
Some of the commonly utilized built-in Python functions are:-
print()
– This function prints the specified values to the console. It accepts single or multiple arguments separated by spaces.len()
– This function determines the length of the added sequence which can be a list, tuple, or string.range()
– This function generates a sequence of numbers according to the given range.type()
– This function outputs the data type of the passed variable or a value.input()
– You can invoke this function to prompt the user to get input from the console.
Example – Using Built-in Functions in Python
In this example, firstly we will define a string named “str
“. Then, call the “print()
” function to display the length of the specified string.
str = "Hello GeeksVeda User" print(len(str))
The print()
function accepts the len()
function as an argument, calls it, and returns the resultant length on the console.
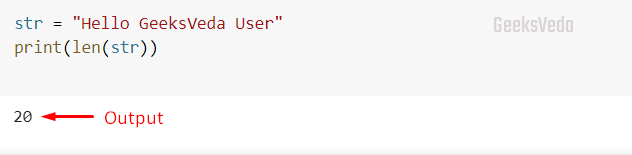
2. User-Defined Python Functions
In Python, User-defined functions are created by the users in their programs using the “def” keyword. These functions permit programmers to define their custom functionality and utilize it repeatedly throughout the program.
Example: Using User-defined Function in Python
For the demonstration, we have created a user-defined function named “calculate_area
” that accepts two parameters “length” and “width“.
Within the function body, the product of both parameters will be calculated and stored in another variable named “area“. Lastly, this function outputs the values of the area when it is called.
def calculate_area(length, width): area = length * width return area print("Area is:", calculate_area(3, 9))
It can be observed that the print()
function is invoked to display the calculated area. It further calls the calculate_area function with the arguments “3” and “9” and outputs “27” as the area.
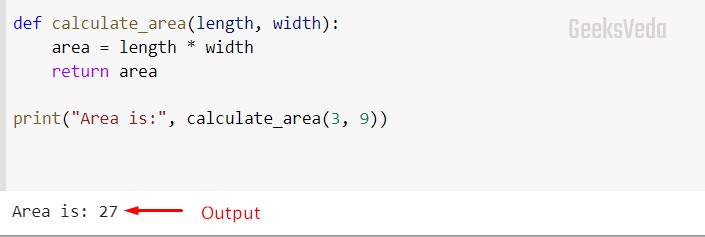
3. Lambda Python Functions
Lambda or Anonymous functions in Python can have multiple arguments but only one expression. These functions do not have a specific name. However, they are defined using the “lambda” keyword.
Here is the syntax that you need to follow for defining the lambda function in Python.
lambda arguments: expression
In the given syntax:-
- “
lambda
” is the keyword utilized for defining the lambda function. - “
arguments
” are the input values of the created lambda function. These values must be enclosed in parentheses and should be separated by commas. - “
expression
” is a single expression that the lambda function evaluates and returns.
Example: Using Lambda Function in Python
The following code defines a lambda function called “square()
” that only accepts one argument “a” and outputs its square. The added expression “a*a
” is evaluated and returned as the function result.
square = lambda a: a * a print(square(5))
It can be observed that we have invoked the lambda function and passed “5” as an argument and it returned “25” as its square value.
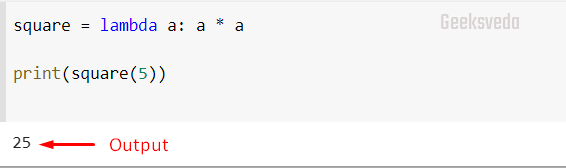
4. Recursive Python Functions
In Python, a Recursive function calls or invokes itself during its execution. This approach is known as Recursion and it is utilized for solving problems that can be further broken down into simpler and smaller versions of the same problem.
To define and use a recursive function in Python, follow the provided basic syntax.
def function_name(parameters): if base_case_condition: # return some value for the base case else: # make a recursive call to the function with updated parameters return function_name(updated_parameters)
Here:-
- “
def
” keyword defines the recursive function with the specified “function_name
“. - “
parameters
” are considered the input values for it. - “
base_case_condition
” defines the base case for the function. If this condition is evaluated as true, it returns a value without making more recursive calls. - “
updated_parameters
” represent the updated input values that are passed to the function in a recursive call.
Example: Using Recursive Function in Python
This example is about defining a recursive function related to calculating the factorial of a number in Python. For this purpose, the base case is when the “n” number equals 0. In this case, the function will output 1.
Otherwise, if n is greater than the function will call itself (performs the recursion) and pass “n-1” as the argument and multiply the result by “n” and return it.
def factorial(n): if n == 0: return 1 else: return n * factorial(n-1) print(factorial(7))
As a result, when “7” is passed as an argument, “5040” has been returned as its factorial.
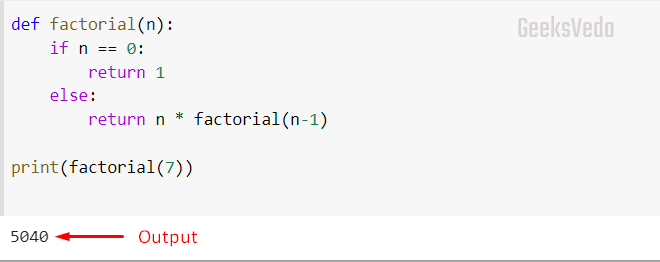
5. Decorative Python Functions
Decorative Python functions are used to enhance or modify the behavior of a function without directly updating its code.
These types of functions allow you to add any functionality to a function before or after it gets executed or modify its input/output. Moreover, they are defined by utilizing the “@decorator
” keyword.
def decorator_func(original_func): def wrapper_func(*args, **kwargs): # Code to run before the original function is invoked result = original_func(*args, **kwargs) # Code to run after the original function is called return result return wrapper_func @decorator_func def my_func(): # function body
- The “
decorator_func
” is a decorator that accepts the “original_func
” as its argument and outputs the “wrapper_func
” that assists in wrapping the original function. - This wrapper_function can accept multiple arguments as “
*args
” and keyword arguments as “**kwargs
“. - The “
result
” variable saves the resultant value that is displayed by the wrapper_func. - Note that to use the decorator, define the
@decorator_func
syntax above the function you want to decorate. In this scenario, “decorator_func
” will wrap the “my_func
“.
Example: Using the Decorator Function in Python
Define a decorator function named “my_decorator
” that accepts “func
” as its argument. This decorator function defines a new function called “wrapper
” that displays the specified message before and after executing the original function.
Then, the “@my_decorator
” syntax is utilized for decorating the “my_function
” with “my_decorator
“. When my_function is invoked, it will run the wrapper function rather than the original function.
def my_decorator(func): def wrapper(): print("Before calling the function") func() print("After calling the function") return wrapper @my_decorator def my_function(): print("This is the function body") my_function()
Lastly, the “my_function()
” is called which runs the wrapped version of the function and gives the following output.
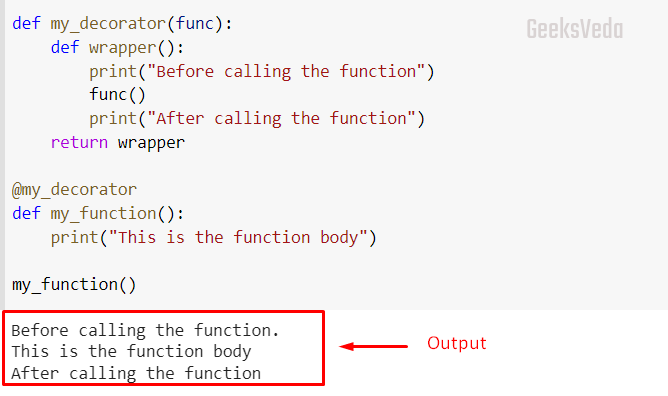
That’s how you can define different types of functions in Python.
Conclusion
Python functions are a cornerstone of any programming project. From simple built-in functions to complex user-defined and recursive functions, Python offers multiple options for developers to create modular, reusable, and efficient code.
Moreover, decorators and lambda functions also provide additional tools to modify and extend the behavior of functions, making them even more powerful and versatile.
So keep exploring and keep learning through our Python Tutorials series!