Python is a powerful programming language that supports multiple operators to assist you in carrying out different tasks.
Using these operators, you can change variable values, perform mathematical operations, and compare values among other things. More specifically, any developer who wants to write out effective and efficient code must be familiar with Python operators.
In our earlier article, we discussed Python Functions, in this blog will discuss different types of Python operators, such as Arithmetic operators, Assignment operators, Logical operators, Comparison operators, Bitwise operators, Membership operators, and Identity operators. Moreover, we will demonstrate their practical usage with the help of examples.
1. Arithmetic Operators in Python
In Python, Arithmetic operators can be utilized for performing mathematical operations. More specifically, Python supports all of the basic operations that can be found in other programming languages.
Here, we have enlisted the Python Arithmetic operators with their relevant descriptions:
- Addition Operator – This operator is represented by the
“+”
symbol and it adds two or more numbers. - Subtraction Operator – This operator is represented by the
“-”
symbol and it subtracts two numbers. - Multiplication Operator – The Multiplication operator multiplies two or more numbers and it is represented by the asterisk “
*
” symbol. - Division Operator – The Division operator divides the given numbers or operands and it is represented by the forward slash “
/
” symbol. - Modulus Operator – In case you want to find the remainder of a division, then use the Modulus operator in your Python code. It is represented by the percent “
%
” symbol. - Exponentiation Operator – Want to raise a number to a power? Utilize the Exponentiation operator. It is represented with the help of a double asterisk “
**
” symbol.
How to Use Arithmetic Operators in Python
Now, check the given program that applies each of the mentioned Arithmetic operators to the variables x and y.
x = 3 y = 7 print("Addition:", x + y) print("Subtraction:", x - y) print("Multiplication:", x * y) print("Division:", x / y) print("Modulus:", x % y) print("Exponentiation:", x ** y)
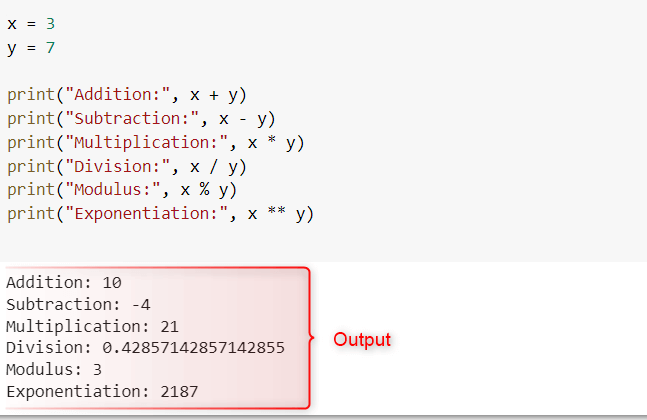
2. Comparison Operators in Python
The Python Comparison operators can be utilized for comparing two operands or simple values. These types of operators output a boolean value (which can be True or False) depending upon the evaluation of the comparison.
You can utilize the following Comparison operators in Python:
- Equal to – The Equal to Operator “
==
” in Python can be utilized for checking if the specified operands are equal or not. If both values are equal, this operator outputs True, otherwise, it displays False. - Not Equal to – The Not Equal Operator “
!=
” verifies whether two values are equal or not. If both values or operands are not equal, it outputs True, otherwise, it returns False. - Greater than – This operator is represented by the
">"
symbol and it verifies if the first operand is greater than the second one. More specifically, if the first value is greater than the second one, it outputs True, otherwise, it displays false. - Less than – This operator is represented by the “
<
” symbol. It verifies whether the first operand is less than the second one, if yes it outputs True, otherwise, it returns False. - Greater than or equal to – This comparison operator “
>=
” compares if the first operand is greater than or equal to the second one. If this condition is satisfied, True will be returned, otherwise, False will be set as the return case. - Less than or equal to – Last but not least, this operator “
<=
” verifies if the first value is less than or equal to the second one. If yes, it returns True, otherwise, it outputs False.
How to Use Comparison Operators in Python
Execute the given program to know the practical usage of the comparison operators in Python code.
x = 3 y = 7 print("Using Equal to:", x == y) print("Using Not equal to:", x != y) print("Using Greater than:", x > y) print("Using Less than:", x < y) print("Using Greater than or equal to:", x >= y) print("Using Less than or equal to:", x <= y)
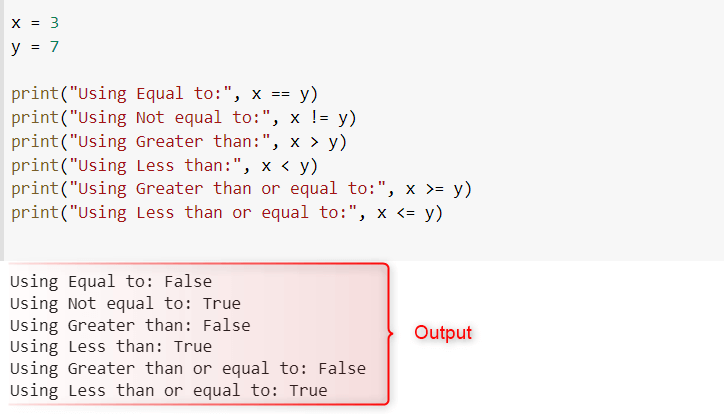
3. Assignment Operators in Python
The Assignment operators in Python can be utilized for assigning values to variables in a program. These operators include these enlisted operators:
- Assignment Operator – It is represented by the equals “
=
” symbol and it assigns the value placed on the right side to the variable present on the left side. - Addition Assignment Operator – It is indicated by the “
+=
” symbol. This operator adds and assigns the value placed on the right side to the variable present on the left side. - Subtraction Assignment Operator – This operator is represented by the “
-=
” symbol and it subtracts the given right-side values from the variable and assigns the result to the left-sided variable. - Multiplication Assignment Operator – It is represented by the “
*=
” symbol and it multiplies the right side value to the left side operand and performs the variable assignment operation. - Division Assignment Operator – You can use this operator for dividing the value placed on the right side with the value of the left side operand. It is represented as “
/=
”. - Modulus Assignment Operator – It is represented by the “
%=
” symbol. You can utilize this operator to calculate the modulus of the left-side operand with the right-side value. Resultantly, assigns the value to the left side operand. - Exponentiation Assignment Operator – It is represented by the “
**==
” symbol” and raises the left side variable value to the power of the right side operand. After that, it stores the result in the left-side variable. - Bitwise AND Assignment Operator – It is symbolized by “
&=
”. It evaluates the bitwiseAND
of the left side value with the right side variable and assigns the value to it. - Bitwise OR Assignment Operator – This operator is represented by the “
|=
” symbol and it evaluates the bitwise OR of the left-side value with the right-side variable and assigns the value to it.
How to Use Assignment Operators in Python
Have a look at the given program which uses the first five discussed Assignment operators.
x = 10 print("Assignment operator:", x) x += 5 print("Addition assignment operator:", x) x -= 3 print("Subtraction assignment operator:", x) x *= 2 print("Multiplication assignment operator:", x) x /= 3 print("Division assignment operator:", x)
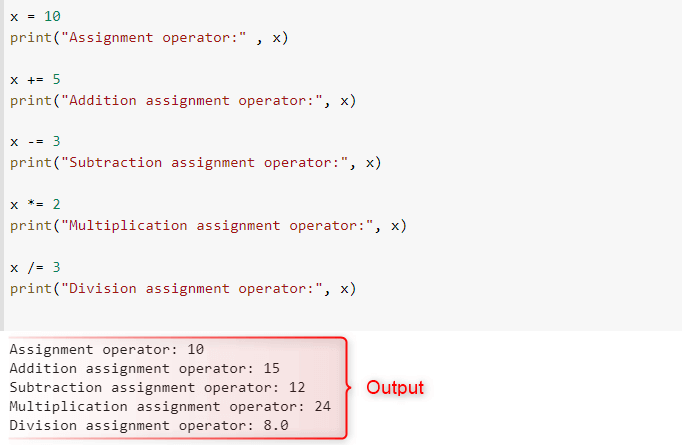
The second program demonstrates the use of the remaining four Python assignment operators.
x = 10 x %= 5 print("Modulus assignment operator:", x) x **= 2 print("Exponentiation assignment operator:", x) x &= 1 print("Bitwise AND assignment operator:", x) x |= 2 print("Bitwise OR assignment operator:", x)
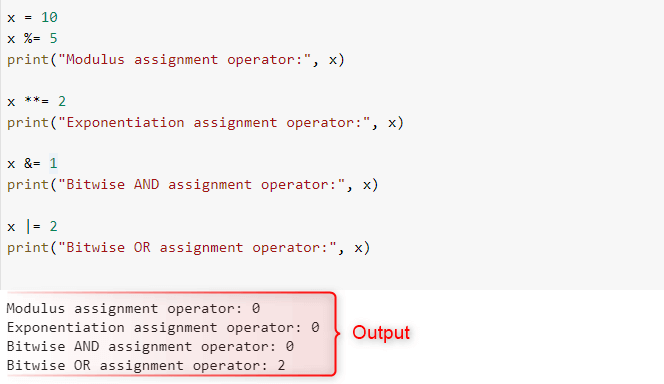
4. Logical Operators in Python
While programming in Python, the Logical operators are used for combining multiple conditions and returning a boolean value, based on the evaluation. Logical Operators have three types:
- and – This logical operator outputs True if both of the added conditions are evaluated as True.
- or – This logical operator outputs True if at least one of the added conditions is evaluated as True.
- not – This logical operator outputs the opposite of the resultant boolean value of the condition.
How to Use Logical Operators in Python
Now, check out the example code.
x = 6 y = 4 z = 10 print("Result of AND operator:", x > y and z > y) print("Result of OR operator:", x > y or z < y) print("Result of NOT operator:", not x > y)
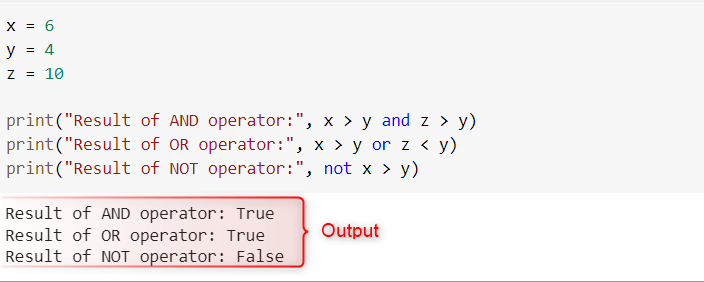
5. Bitwise Operators in Python
Bitwise operators can perform the enlisted operations on binary numbers:
- Bitwise AND Operator – This operator is represented by the “
&
” symbol and it outputs 1 if both bits are 1, otherwise displays 0. - Bitwise OR Operator – This operator is represented by the “
|
” symbol and it outputs 1 if at least one bit is 1, otherwise displays 0. - Bitwise XOR Operator – This operator is represented by the “
^
” symbol and it outputs 1 if only one bit is 1, otherwise displays 0. - Bitwise NOT Operator – This operator is represented by the “
~
” symbol and it flips the bits of the number. - Bitwise Left Shift Operator – It is represented by the “
<<
” symbol and utilized for shifting the bits to the left with respect to the specified number of places. - Bitwise Right Shift Operator – It is represented by the “
>>
” symbol and is used for shifting the bits to the right according to the specified number of places.
How to Use Bitwise Operators in Python
Here is the program that uses all of the discussed Bitwise operators in Python.
x = 4 y = 3 print("Bitwise AND operator:", x & y) print("Bitwise OR operator:", x | y) print("Bitwise XOR operator:", x ^ y) print("Bitwise NOT operator:", ~x) print("Bitwise Left Shift operator:", x << 1) print("Bitwise Right Shift operator:", x >> 1)
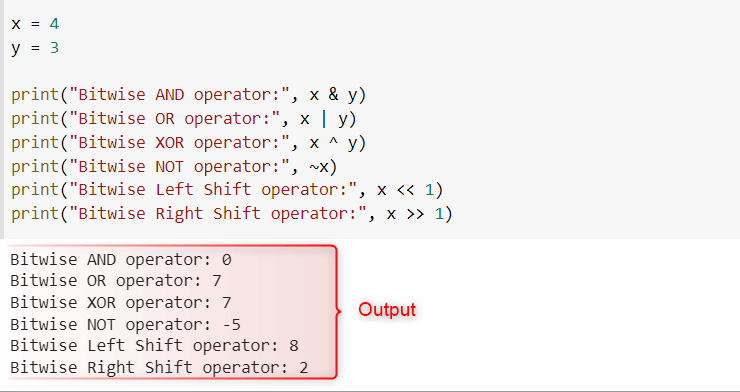
6. Membership Operators in Python
The Membership operators can be utilized for testing if a value is a member or a part of a sequence or not. These operators have two types:
- in – It outputs True in case the value is found in the sequence.
- not in – It outputs True if the given value is not found in the sequence.
How to Use Membership Operators in Python
Check out the provided program in case you want to use the Membership operators in your Python code.
x = [1, 2, 3, 4, 5] print("Membership IN operator:", 3 in x) print("Membership NOT IN operator:", 0 not in x)
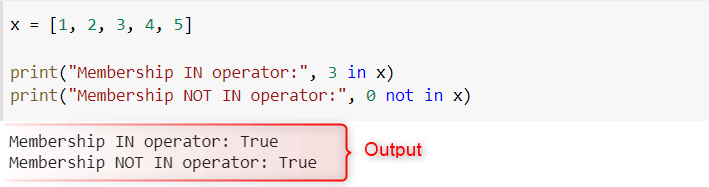
7. Identity Operators in Python
Need to compare the memory addresses of two objects in Python? Use the following Identity operators as per your requirements:
- is – This operator outputs True if both variables point to the same object.
- is not – This operator outputs True if both variables point to different objects.
How to Use Identity Operators in Python
Here is a Python program that uses both of the mentioned Identity operators:
x = 5 y = [1, 2, 3] print("Identity IS operator:", x is y) print("Identity IS NOT operator:", x is not y)
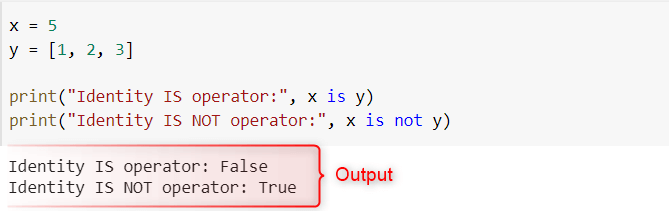
That’s how you can use Python operators in your code.
Conclusion
Every developer should understand the basic fundamentals of Python operators. More specifically, knowing how to use the different Python operators can make your code more effective and it does not matter if you are working on simple arithmetic or complex bit manipulation.
You can also enhance the quality of your projects and your programming abilities by taking the time to study and practice these operators. So keep exploring and keep learning through our Python Tutorials series!