While programming in Python, sometimes you may need to display the calendar to perform the required functionality. Python with its versatile libraries and rich ecosystems supports several methods to achieve this task.
Whether you want to display a simple text-based calendar, or a graphical user interface with interactive widgets, relevant Python diverse approaches can be applied easily. So, in today’s guide, we will discuss different techniques for displaying calendars in Python.
Why Display Calendar in Python
From personal organization to professional scheduling and beyond, the representation of calendars holds immense importance in various contexts. More specifically, Python offers several methods for displaying calendars, which makes it a robust tool for addressing different scenarios.
Here are some of the scenarios where displaying calendars in Python becomes crucial:
- Personal Time Management – Visualizing calendars helps individuals manage their daily schedules, appointments, and tasks effectively. Python’s calendar capabilities assist in creating organized plans.
- Event Scheduling and Reminders – Displaying calendars aids in scheduling and tracking events, meetings, and deadlines. Calendar displays can include reminders to ensure important activities are not overlooked.
- Data Visualization and Analysis – For data-driven projects, calendars can visualize temporal data patterns, such as sales trends, project milestones, or weather conditions over time.
- Historical Research and Analysis: Researchers and historians often need to analyze historical events within a time frame. Displaying calendars facilitates a chronological overview of historical data.
How to Display Calendar in Python
In order to display the calendar in Python, you can use:
- Calendar Module
- Datetime Module with strftime()
- Third-party Libraries
- Custom Calendar Functions
Let’s check out each of the listed approaches practically!
1. Using Calendar Module
Python built-in “calendar
” module can be utilized for showing calendars. Within this module, the “calendar.TextCalendar
” class enables you to generate textual representations of the calendars. Additionally, by specifying the year and month, you can create neatly formatted calendars.
For instance, in the below-given program, the “calendar.TextCalendar
” class is initialized with the “calendar.SUNDAY
” parameter for setting Sunday as the first day of the week.
Next, the “formatmonth(year, month)
” method is invoked to generate a textual representation of the specified month for the given year.
import calendar year = 2023 month = 8 cal = calendar.TextCalendar(calendar.SUNDAY) month_calendar = cal.formatmonth(year, month) print(month_calendar)
As a result, the formatted calendar with the selected month will be displayed on the console.
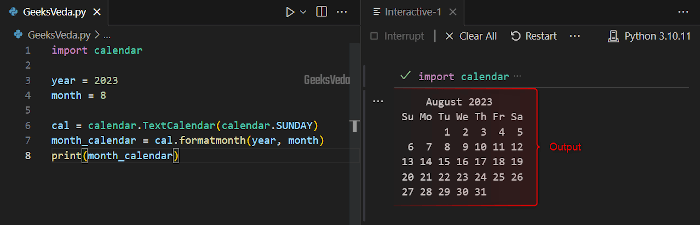
2. Using datetime Module with strftime()
The strftime() function of the datetime module also supports the functionality of the displaying calendar. For this, you can manipulate the date formatting to create a simple calendar representation.
Here, the “datetime
” module is utilized for creating the date objects for the first and the last day of the specified month. The added-for loop iterates through each day of the month and converts the date to a string format with the “strftime()
” function, where the “%a, %d” format represents the day’s name and number.
from datetime import datetime, timedelta year = 2023 month = 8 first_day = datetime(year, month, 1) last_day = datetime(year, month + 1, 1) - timedelta(days=1) for day in range(1, last_day.day + 1): current_date = datetime(year, month, day) print(current_date.strftime("%a %d"))
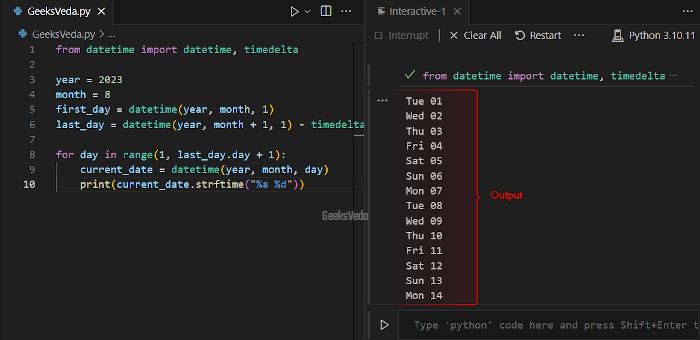
3. Using Third-party Libraries
As discussed earlier, the Python ecosystem is embedded with third-party libraries that support extended functionalities for calendar displays. For instance, libraries such as “tkinter” and “tkcalendar” enable you to create graphical user interfaces with calendar widgets.
These widgets offer interactive calendar views which makes it easy to visualize and navigate through dates.
According to the below program, the “tkcalendar” library is used for creating a graphical calendar widget with the help of the “Calendar” class.
import tkinter as tk from tkcalendar import Calendar root = tk.Tk() cal = Calendar(root, selectmode='day') cal.pack() root.mainloop()
When the given code executes, a new window will open up with an interactive calendar widget that users can utilize to select dates.
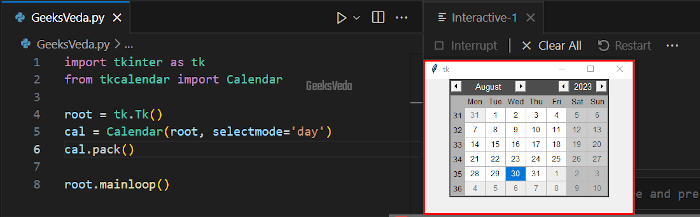
4. Using Custom Calendar Functions
Creating custom functions for displaying calendars offers flexibility in formatting and content. More specifically, by defining functions that generate calendars, you can design them with respect to your requirements.
In the given program, we will define a “display_calendar()
” custom function that accepts a year and month as arguments. Then, the “calendar.monthcalendar()
” method generates a matrix that represents the given month’s week and dates.
This function iterates through each week using a for loop and formats the days into a printable string.
import calendar def display_calendar(year, month): cal = calendar.monthcalendar(year, month) for week in cal: print(' '.join(str(day) if day != 0 else ' ' for day in week)) year = 2023 month = 8 display_calendar(year, month)
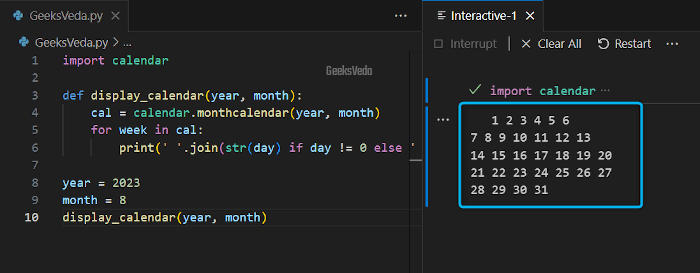
Choosing the Right Method for Displaying Calendar
The given table will assist you in selecting the most suitable method for displaying the calendar in your project:
Method | Advantages | Disadvantages | Use Cases |
Calendar Module | Simple and built-in functionality. | Limited customization options. | Displaying basic text-based calendars. |
datetime Module | Flexibility in date manipulation. | Requires manual formatting. | Generating date-based views for specific periods. |
tkcalendar Library | Graphical and interactive calendar widget. | GUI dependency. | Creating GUI applications with date selection. |
Using the Custom Calendar Functions | Customization and formatting flexibility. | Requires coding and formatting. | Designing calendars with personalized formatting. |
That brought us to the end of our guide related to displaying a calendar in Python.
Conclusion
Python supports several tools for displaying calendars in your project. From the simple “calendar
” module to the interactive graphical widgets, the approaches discussed in today’s guide cover a broad spectrum of possibilities.
So, depending on the requirements, select the method that aligns best with your goals and create intuitive calendar displays that improve the user experience.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!