Welcome to our today’s guide related to the Python strftime()
method.
This crucial method enables you to transform date and time data into customized, user-friendly formats.
So, in this post, we will explore, datetime.strftime()
method, its supported directives, customized date, and time representations, additional formatting options, and the approaches to using datetime.strftime()
method in Python.
What is strftime () Method in Python
Python’s “strftime()
” method is a robust tool that is offered by the “datetime
” module. It permits the developers to convert datetime objects into formatted strings.
This method basically represents “string format time
” and is a crucial function that can be used when it is required to represent date and time information in a human-readable format.
Additionally, with the help of format codes, you can control how each component of the datetime object is represented in the output string.
What is the Use of strftime() in Python
These are some of the key features of the datetime.strftime()
Method:
- It enables customization of date and time representations with respect to particular requirements.
- It offers the option to format dates in different regions and languages, enhancing internationalization capabilities.
- Properly formatted date can make the sorting the filtering operation more efficient.
How Does strftime() Work in Python
The datetime.strftime()
method works by accepting a “datetime
” object and a “format
” string as inputs or arguments. This format string comprises a combination of format codes and literal text.
Then, the method replaces the format codes in the format string according to the values from the “datetime
” object and generates the final formatted string.
Here is the syntax of the datetime.strftime()
method.
datetime_object.strftime(format)
According to the given syntax:
- “
datetime_object
” is the “datetime
” object that is required to format as a string. - “
format
” is the format that refers to the desired format for the datetime object.
Now, let’s check out a simple example of converting a datetime object into a formatted string with datetime.strftime() method.
Convert a datetime Object to a String
First of all, we will create a “datetime
” object that represents “2023-07-18 15:30:45
” as the date and time. After that, the datetime.strftime()
method is utilized for formatting this datetime object into a string representation.
More specifically, the format string is given as “%Y-%m-%d %H:%M:%S
” comprising the format codes that refer to the required format for each component of the datetime object.
from datetime import datetime # Create a datetime object date_time_obj = datetime(2023, 7, 18, 15, 30, 45) # Format the datetime object formatted_string = date_time_obj.strftime("%Y-%m-%d %H:%M:%S") # Output the formatted string print(formatted_string)
So, when the program executes, the datetime.strftime()
method scans the given format string for format codes. It then fetches the respective value from the datetime object and substitutes it into the suitable position in the format string.
Remember that any non-format code characters in the format string will remain unchanged in the formatted string.
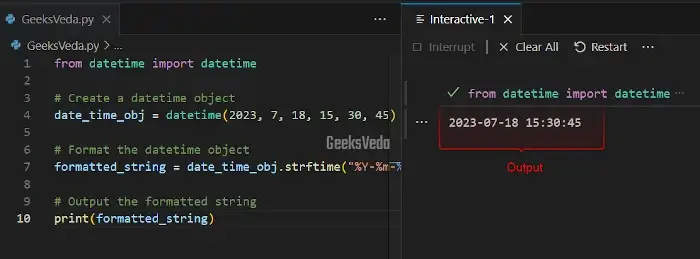
Format Code/Directives for strftime() Method
Directives or format code for the “strftime()
” method is the special format code that permits you to represent the particular components of a datetime object when converting it into a formatted string.
Moreover, “%
” is added as a prefix with these directives and utilized within the format string.
Check out the provided list of directives/format code used in the strftime()
method or Function.
Directive/Format Code | Description | Example |
%Y | 4-digit year | 2023 |
%y | 2-digit year | 23 |
%m | 2-digit month | 07 (for July) |
%B | Full month name | July |
%b or %h | Abbreviated month name | Jul |
%d | 2-digit day | 18 |
%A | Full weekday name | Friday |
%a | Abbreviated weekday name | Fri |
%H | 2-digit hour in 24-hour format | 15 (for 3 PM) |
%I | 2-digit hour in 12-hour format | 03 (for 3 AM/PM) |
%p | AM/PM indicator | AM or PM |
%M | 2-digit minute | 30 |
%S | 2-digit second | 45 |
%f | Microseconds | 567890 |
%Z | Timezone name | (e.g., “UTC”, “PST”) |
%z | UTC offset represented as -HHMM or +HHMM | (e.g., -0800, +0530) |
%j | Day of the year (Which can be 1 to 366) | 199 |
%U | Week number of the year (It assumes Sunday as the first day) | 28 |
%W | Week number of the year (It assumes Monday as the first day) | 28 |
%c | Locale’s suitable date and time format | Mon Jul 18 15:30:45 2023 |
%x | Locale’s suitable date format | 07/18/23 (for some locales) |
%X | Locale’s suitable time format | 15:30:45 (for some locales) |
%w | Weekday as a decimal number (0 for Sunday, 1 for Monday, etc.) | 6 |
%-y | A year without century as a decimal number | 99 |
%-d | Day of the month as a decimal number | 10 |
%-m | Month as a decimal number | 11 |
%-H | Hour (24-hour clock) as a decimal number | 20 |
%-I | Hour (12-hour clock) as a decimal number | 7 |
%-M | Minute is a decimal number | 4 |
%-S | Second as a decimal number | 50 |
1. Custom Date Representations
With the help of the directives that are discussed in the previous section, you can create custom date presentations like these:
- %Y-%m-%d: Indicates the date in the format “
YYYY-MM-DD
” (e.g., 2023-07-18). - %H:%M:%S: Refers to the time in the format “
HH:MM:SS
” (e.g., 15:30:45). - %B %d, %Y: Indicates the date in the format “Month Day, Year” (e.g., July 18, 2023).
2. Other Formatting Options
In the datetime.strftime(), you can also utilize other characters in the format string as additional options given in the below table:
Character | Description | Example |
0 | Pad with zeros | %m -> 07 (July), %M -> 03 (3 minutes) |
– | Left-align | %-d -> 18 (left-aligned day), %-H -> 3 (left-aligned hour) |
: | Custom text separators | “%Y:%m:%d” -> “2023:07:18” |
/ | Custom text separators | “%Y/%m/%d” -> “2023/07/18” |
How to Use strftime() Method in Python
The strftime()
method can be used in data analytics, finance, and web development. It transforms the timestamps for trend analysis.
This ultimately improves the data visualization in a user-friendly layout. Moreover, it also standardizes the time-related data, ultimately enhancing the over user experience and efficiency across different industries.
Now, let’s check out some practical examples related to the usage of the datetime.strftime()
method.
1. Using Format Codes
In the first example, we will create a “datetime
” object and format it into two different strings. The format codes are given as “%Y-%m-%d
” for the date and “%H:%M:%S
” for the time and are then passed as arguments to the datetime.strftime()
method accordingly.
Lastly, the print() function will display the date and time in the given format.
from datetime import datetime # Create a datetime object date_time_obj = datetime(2023, 7, 18, 15, 30, 45) # Format the datetime object using format codes formatted_date = date_time_obj.strftime("%Y-%m-%d") formatted_time = date_time_obj.strftime("%H:%M:%S") # Output the formatted strings print(formatted_date) print(formatted_time)
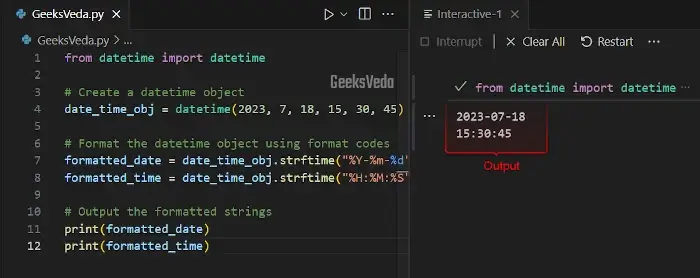
2. Specify Padding and Alignment
Now, in the datetime.strftime() method, we have specified the formatting of the “datetime
” object with padding and alignment with “%m/%d/%y
” for the date and “%H:%M:%S
” for the time.
Moreover, the “lstrip()
” method has been invoked to left-align the hour leading to zero.
from datetime import datetime # Create a datetime object date_time_obj = datetime(2023, 7, 5, 9, 5, 7) # Format the datetime object with padding and alignment formatted_date = date_time_obj.strftime("%m/%d/%Y") formatted_time = date_time_obj.strftime("%H:%M:%S") # Use str.lstrip() to left-align the hour without leading zero formatted_hour_left_aligned = date_time_obj.strftime("%I:%M %p").lstrip("0") print(formatted_date) print(formatted_time) print(formatted_hour_left_aligned)
As you can see, the formatted date, time, and hour have been displayed on the console.
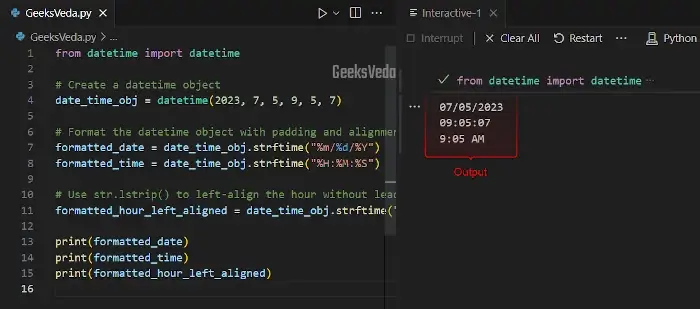
3. Generate a Timestamp
In the provided example, firstly, we will generate the current datetime with the “datetime.now
” method. Then, the fetched date and time will be formatted as a timestamp in the format “MM-DD HH:MM:SS
” and displayed as output.
from datetime import datetime # Get the current datetime current_datetime = datetime.now() # Format as a timestamp timestamp = current_datetime.strftime("%Y-%m-%d %H:%M:%S") print("Timestamp:", timestamp)
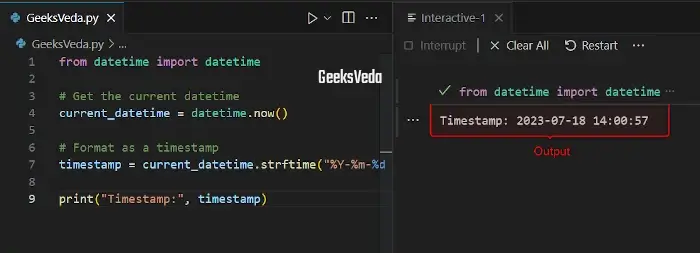
4. Customise the Date Format
Here, we have a “datetime
” object which refers to the “2023-01-01
” date. Then, we called the datetime.strftime()
method and passed the custom string format using format codes as “%B %d, %Y
“.
This resultantly formats the string as “New Year: Month Day, Year
” format.
from datetime import datetime # Create a datetime object date_time_obj = datetime(2023, 1, 1) # Format as a custom date custom_date = date_time_obj.strftime("New Year: %B %d, %Y") print(custom_date)
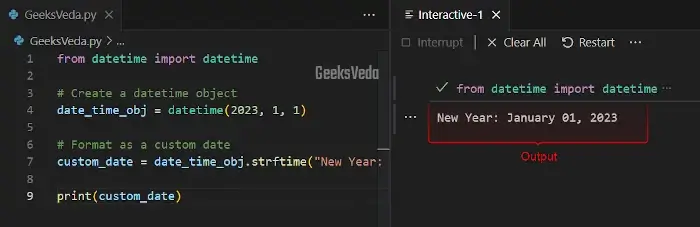
5. Format Dates for Different Time Zones
According to the given program, we will create a “datetime
” object in UTC, and then convert it into the “America/New_York
” time zone by calling the “astimezone()
” method.
Then, the datetime.strftime()
method formats the datetime objects. Lastly, the print()
function shows both UTC and New York time with respect to their timezone.
from datetime import datetime, timedelta import pytz # Create a datetime object in UTC utc_datetime = datetime(2023, 7, 18, 15, 30, 45, tzinfo=pytz.utc) # Convert the UTC datetime to a specific time zone (e.g., New York) ny_timezone = pytz.timezone('America/New_York') ny_datetime = utc_datetime.astimezone(ny_timezone) # Format the datetime objects for display formatted_utc = utc_datetime.strftime("UTC Time: %Y-%m-%d %H:%M:%S %Z") formatted_ny = ny_datetime.strftime("New York Time: %Y-%m-%d %H:%M:%S %Z") print(formatted_utc) print(formatted_ny)
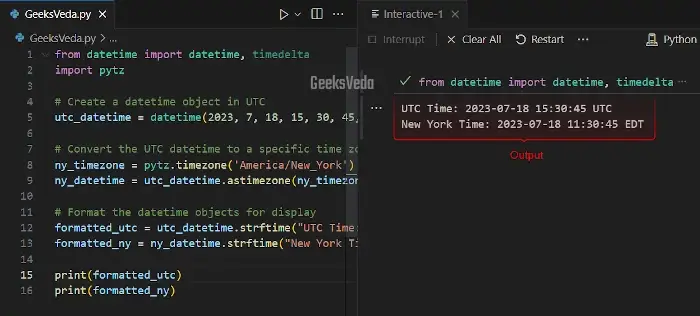
6. Create a Date Format for the Event Countdown
Here, firstly, the current date and time are retrieved by utilizing the “datetime.now()
” method. Then, we have defined “2023-12-31
” as the target and the remaining time until the event is calculated.
Then, we extracted the number of days and remaining hours from the time difference. Lastly, the countdown message has been formatted, displaying the days and hours left until New Year’s Evening.
from datetime import datetime # Get the current date and time current_datetime = datetime.now() # Define the target event date event_date = datetime(2023, 12, 31) # Calculate the time remaining until the event time_remaining = event_date - current_datetime # Extract the number of days and hours remaining days_remaining = time_remaining.days hours_remaining = time_remaining.seconds // 3600 # Format the countdown message countdown_message = "New Year's Eve Countdown:\n{} days and {} hours remaining!".format(days_remaining, hours_remaining) print(countdown_message)
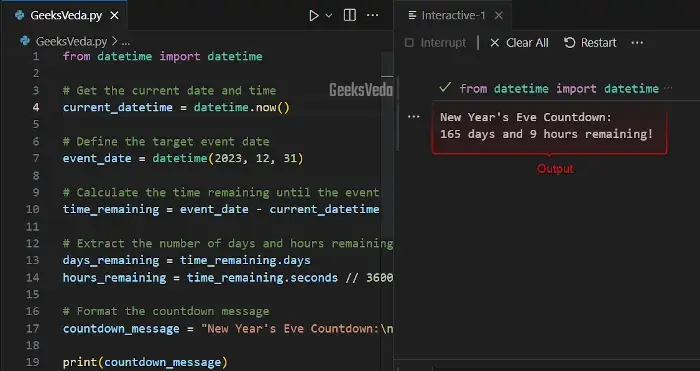
That’s all from this effective guide related to the usage of the datetime.strftime()
method.
Conclusion
The “datetime.strftime()
” method in Python permits easy conversion of the datetime object into custom string representations. Using its customized format codes, you can improve data visualization and perform time-related tasks across multiple applications.
So, understand this method deeply and then deploy it in your Python project.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!