Today’s guide is all about Python “datetime.strptime()
” method and its commendable usages! So, if you want to convert date and time strings into datetime objects in Python, you have come to the right place.
This detailed guide provides you with the ins and outs of the Python datetime.strptime()
method which makes date and time handling a breeze.
So, let’s start the guide and learn how to utilize this method in the Python project for the best results.
What is Python datetime.strptime() Function
The Python “datetime.strptime()
” method acts as the counterpart of the datetime.strftime() method. As the datetime.strftime()
converts the datetime objects into formatted strings, the Python datetime.strptime()
method performs the reverse operations. It converts the formatted strings into the corresponding datetime objects.
This method permits you to parse strings representing dates and times into the respective datetime objects. This resultantly allows the easier analysis and manipulation of the time-related data.
What is the Use of datetime.strptime() Function
The “datetime.strptime()
” method in Python can be found useful when you are dealing with data from external sources or with user input represented as strings.
Using this method you can convert input strings into datetime objects effectively. This enables you to perform various date and time-related operations, such as comparisons, arithmetic operations, and data filtering.
2. How Does datetime.strptime() Module Work in Python
In Python, the datetime.strptime()
works by parsing a string representing the time and then converting it into a “datetime
” object.
This method accepts two arguments, one is the input string comprising the date and time information, and the second is the format string that specifies how the date components will be represented in the input string.
Here is the syntax for using the datetime.strptime() Function.
datetime.strptime(date_string, format)
According to the given syntax:
- The “date_string” is the string that refers to the date and time in the specified format that you want for the conversion.
- The “
format
” is the string specifying the date_string format with the help of format code. These codes signify the positions of the year, month, day, hour, minutes, etc.
1. Format Codes Used in datetime.strptime()
Format codes that can be utilized in the datetime.strptime()
are similar to those that can be added in the datetime.strptime() method
. These codes permit you to represent particular components of the datetime object in the input string.
Below-given table contains a description of some of the commonly used format codes:
Directive/Format Code | Description | Example |
%Y | 4-digit year | 2023 |
%y | 2-digit year | 23 |
%m | 2-digit month | 07 (for July) |
%B | Full month name | July |
%b or %h | Abbreviated month name | Jul |
%d | 2-digit day | 18 |
%A | Full weekday name | Friday |
%a | Abbreviated weekday name | Fri |
%H | 2-digit hour in 24-hour format | 15 (for 3 PM) |
%I | 2-digit hour in 12-hour format | 03 (for 3 AM/PM) |
%p | AM/PM indicator | AM or PM |
%M | 2-digit minute | 30 |
%S | 2-digit second | 45 |
%f | Microseconds | 567890 |
%Z | Timezone name | (e.g., “UTC”, “PST”) |
%z | UTC offset represented as -HHMM or +HHMM | (e.g., -0800, +0530) |
%j | Day of the year (Which can be 1 to 366) | 199 |
%U | Week number of the year (It assumes Sunday as the first day) | 28 |
%W | Week number of the year (It assumes Monday as the first day) | 28 |
%c | Locale’s suitable date and time format | Mon Jul 18 15:30:45 2023 |
%x | Locale’s suitable date format | 07/18/23 (for some locales) |
%X | Locale’s suitable time format | 15:30:45 (for some locales) |
%w | Weekday as a decimal number (0 for Sunday, 1 for Monday, etc.) | 6 |
%-y | A year without century as a decimal number | 99 |
%-d | Day of the month as a decimal number | 10 |
%-m | Month as a decimal number | 11 |
%-H | Hour (24-hour clock) as a decimal number | 20 |
%-I | Hour (12-hour clock) as a decimal number | 7 |
%-M | Minute is a decimal number | 4 |
%-S | Second as a decimal number | 50 |
2. Using the Python datetime.strptime() Method
For example, we have a string representing a date in the format “2023-07-18
” and it is required to convert it into a datetime object.
For the given requirements, we will invoke the “datetime.strptime()
” method and pass “date_string
” and the defined “format
” as arguments.
Lastly, the print() function will display the resultant datetime object on the console.
from datetime import datetime date_string = "2023-07-18" format = "%Y-%m-%d" date_time_obj = datetime.strptime(date_string, format) print(date_time_obj)
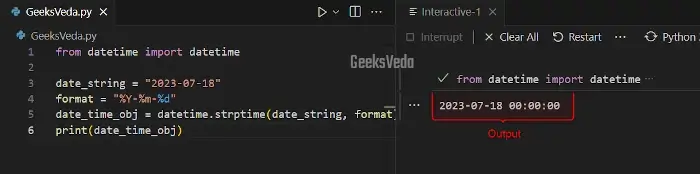
Convert Strings to Datetime Objects
The “datetime.strptime()
” method is primarily used when you need to convert date and time information (represented as strings in several formats) into datetime objects.
This method permits you to parse the defined string with respect to the given format and create the respective datetime objects.
Additionally, this conversion enables you to work with the datetime objects and perform multiple operations on them as discussed earlier.
1. Convert a List of Date Strings into Datetime Objects
For instance, we have a list of dates in the “dd-mm-yy
” format and it is required to convert these strings into datetime objects with datetime.strptime()
method.
To do so, define the format as “%d-%m-%Y
” for representing the positions of the day, month, and year in the respective strings, accordingly.
Then, the datetime.strptime()
method has been invoked that performs the discussed operation and stores the datetime objects in the “date_objects
” list.
from datetime import datetime date_strings = ["18-07-2023", "25-12-2023", "03-09-2024"] format = "%d-%m-%Y" date_objects = [datetime.strptime(date_str, format) for date_str in date_strings] print(date_objects)
It can be observed that the datetime objects list has been displayed on the console.
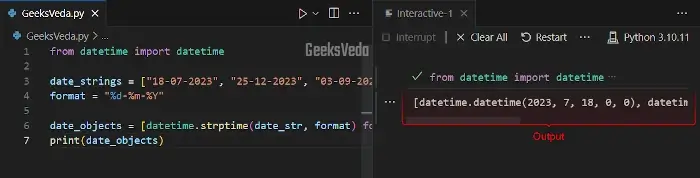
Working with Timezones and Localization in Python
In order to parse and handle the timezones in the input strings, you can utilize the datetime.strptime()
method. However, remember that it does not store time zone information in the resultant datetime objects.
Therefore, use the “pytz
” library that offers the timezone information and allows you to work with timezones and localization, effectively.
1. Convert UTC Date into Datetime Object
In the provided example, we have an input string that indicates the date and time in UTC. Then, the “pytz.timezone()
” function has been invoked for creating a UTC timezone object.
It is then applied to the datetime object with the help of the replace()
method. Resultantly, we will have a datetime object with accurate timezone information.
from datetime import datetime import pytz date_string = "2023-07-18 15:30:45" format = "%Y-%m-%d %H:%M:%S" timezone = pytz.timezone("UTC") date_time_obj = datetime.strptime(date_string, format).replace(tzinfo=timezone) print(date_time_obj)
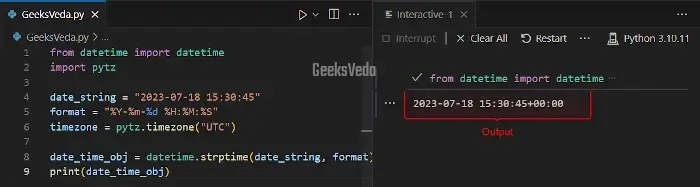
Comparison of datetime.strptime() with Other Parsing Methods
Now, let’s compare the “datetime.strptime()
” with other parsing methods such as “dateutil.parser.parse()
“, “pandas.to_datetime()
“, and “arrow.get()
” with respect to different functionalities:
Functionality | datetime.strptime() | dateutil.parser.parse() | pandas.to_datetime() | arrow.get() |
Parses Strings | Yes | Yes | Yes | Yes |
Flexible Date Formats | Limited support for formats. | Extensive support for various formats. | Limited support for formats. | Yes |
Timezone Handling | Limited support for timezones. | Limited support for timezones. | Limited support for timezones. | Supports multiple timezones. |
Locale-aware Parsing | No | Yes | No | No |
Handling Missing Values | Raises ValueError on parsing error. | Handles parsing errors and outputs NaN. | Raises ValueError on parsing error. | Raises an exception on parsing errors. |
Integration with Pandas | Can be utilized with pandas through apply() or map(). | Can be utilized with pandas through apply() or map(). | Native support in pandas through to_datetime(). | Can be utilized with pandas through apply() or map(). |
User-Friendliness | Needs explicit format codes for parsing | Needs less specific formatting. | Needs explicit format codes for parsing. | More human-readable with natural language expressions. |
That’s all from today’s guide related to the usage of the Python datetime.strptime()
method.
Conclusion
Python datetime.strptime() is a robust method that can be utilized for parsing datetime strings into datetime objects with custom format codes.
This resultantly makes the date input handling more efficient. Moreover, this method also improves data processing and overall visualization.
So, use the Python datetime.strptime()
method and optimize the time-related tasks in your Python projects.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!