Are you looking to learn one of the most crucial Python data structures? Look no further than Python lists! Whether you’re a beginner Python programmer or a professional, understanding how to work with lists is crucial.
In the earlier articles, we discussed Python Operators, Python Functions, Python Comments, and the usage of Python for loop.
This blog will explore many powerful operations you can perform with Python lists, from creating and manipulating lists to searching and sorting them.
So get ready to master one of the most important tools in Python programming language!
1. How to Create Lists in Python
In Python, a list can be created by utilizing the square brackets []
in which the added elements are separated by commas.
Moreover, a Python list can also hold any combination of data types such as numbers, floats, strings, etc.
Follow the provided syntax for creating a list in Python.
list_name = [element1, element2, ..., elementN]
According to the given syntax, list_name refers to the list name, and element1, element2,…, elementN are the required elements that you want to add to the list. Separate them by utilizing commas and enclose them within square brackets.
Now, we will create a list named my_list
that comprises integers and strings. Then, the print()
function is used for displaying the elements on the console.
my_list = [1, 2, 3, 'Hi', 'GeeksVeda', 'User'] print(my_list)
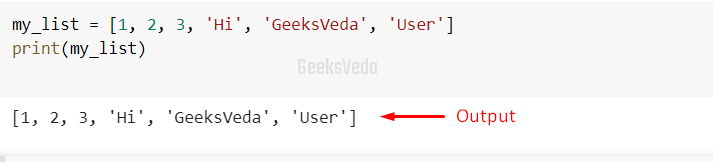
2. How to Initialize Empty Lists in Python
In Python, there is a common practice of initializing an empty list so that you can add elements to it later. Then, methods such as append()
can be invoked for adding elements to the list dynamically.
More specifically, initializing an empty list can be useful in those cases where it is required to declare a list variable without specifying any initial value.
For instance, the given example code initializes an empty list and then prints it.
my_list = [] print(my_list)
In this scenario, the output will be an empty list which signifies that my_list has been initialized with no elements.
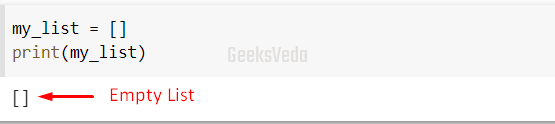
3. How to Add Elements to a List in Python
Adding elements to a Python list is one of the most common tasks that can be performed. For instance, when you want to add new data to the list or when a new element is discovered during the execution of the program.
For this purpose, Python built-in append()
method can be utilized for adding the elements at the list end.
To use the append()
method, follow the given syntax.
append(new_element)
Here, we have initially created a list named my_list that contains three integers 2, 4, 6. Then, the append()
method is invoked and 8 is passed as an argument to it.
my_list = [2, 4, 6] my_list.append(8) print(my_list)
As a result, 8 will be added at the end of the list and the updated list will be displayed using the print()
function.
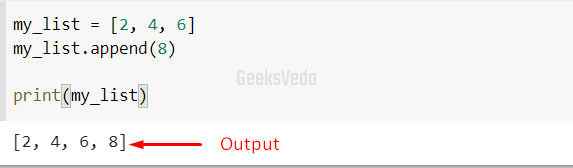
4. How to Access Elements of a List in Python
Another essential operation you may want to perform is accessing elements of a Python list. In many programs, you may need to modify a specific element or fetch the list of elements based on their index values.
To do so, add the index number of the element inside the []
brackets while accessing it with the list name
.
list_name[index]
Using the above-given syntax, we will now access the element that is present at the 1st
index of the created my_list of the fruits name.
my_list = ['mango', 'plum', 'cherry', 'date'] print(my_list[1])
The print()
function will display plum as the element found at the first index of the my_list.

5. How to Slice or Create Subsets From a List in Python
While programming in Python, sometimes you may need to work on a specific portion rather than the whole list. This is where the Python slicing or the operation of creating a subset from a list comes into the picture.
Slicing assists in extracting or fetching a specific range of elements from the list.
For the corresponding purpose, utilize the colon operator “:
” as follows.
list_name[start_index:end_index]
Here, start_index
and end_index
refer to the starting and ending indexes of the substring.
start_index
will be inclusive and end_index
will be exclusive.For example, we want to slice the given my_list of strings and create a substring starting from 1 to 2 index.
To do so, the slice
operator will be used as my_list[1:3]
, where the ending index 3 is exclusive.
my_list = ['mango', 'plum', 'cherry', 'date'] print(my_list[1:3])
As a result, the subset [‘plum’, ‘cherry’] will be printed out on the console.

6. How to Concatenate Lists in Python
Concatenating lists in Python refers to the process of joining multiple lists together to create a new list. It can be used when you need to combine or merge lists to perform a certain operation on them at once.
new_list = list1 + list2
According to the given syntax, new_list is the resultant concatenated list that comprises the elements of both list1 and list2.
+
” performs the major role in the mentioned operation.As you can see in the given code, we have two separate lists named list1
and list2
, where the first list contains strings and the second has three integers.
Then, we created a concatenated list named new_list
by combining the elements of list1
and list2
with the help of the “+
” concatenation operator.
list1 = ['mango', 'plum', 'cherry', 'date'] list2 = [2, 4, 6] new_list = list1 + list2 print(new_list)
It can be observed that the new_list
contains the elements of list1
followed by list2
elements.
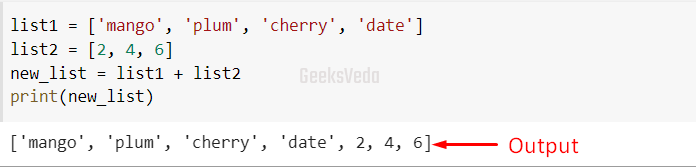
7. How to Perform List Repetition in Python
Want to repeat a list multiple times? If that’s the case, use the list repetition operator “*
” in Python.
Check out the provided syntax for performing the list repetition in Python.
new_list = my_list * n
In the above code, n
is the number of times my_list
needs to be repeated and will be stored in the new_list
.
In the example, we have initialized a list named my_list
that comprises 4 elements. Then, the repetition operator “*
” is used for repeating the list two times.
The result will be stirred in the new_list and printed on the console when the print()
function will be invoked.
my_list = [2, 4, 6, 8] new_list = my_list * 2 print(new_list)
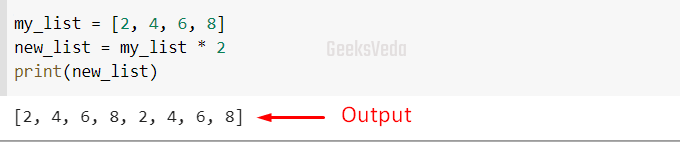
8. What are List Comprehensions in Python
In Python, List Comprehension is a concise way of creating lists based on iterable objects or existing lists. It is a powerful tool and elegant to create a list quickly.
The syntax for creating list comprehensions consists of an expression that is followed by a for loop and an if condition that is optional.
new_list = [expression for variable in iterable if condition]
This expression is evaluated for each item that exists in the iterable and the resulting values will populate the new list.
Now, we want to create a list of squares of numbers from 2 to 7 using list comprehension. Here the expression is defined as “num ** 2
“, num is variable, and “range(2, 8)
” is iterable.
The resultant list will be stored in the squares variable and displayed on the console by utilizing the print()
function.
squares = [num ** 2 for num in range(2, 8)] print(squares)
Note that “8” is exclusive while defining the range()
function.

9. How to Update Elements in a Python List
First, let’s discuss why we need to update elements in a Python list. Sometimes, you may need to change an element’s value in a list after it has been initialized, which can be done by updating the list.
Here, we are initializing a list named fruits with four elements. Then, the original list is printed with the print()
function. Next, update the second element “fruits[1]” of the list, i.e., change ‘plum‘ to ‘apple‘.
Finally, print the updated list again by utilizing the print()
function.
# Initializing a list fruits = ['mango', 'plum', 'cherry', 'date'] # Printing the original list print("Original List:", fruits) # Updating the second element fruits[1] = 'apple' # Printing the updated list print("Updated List:", fruits)
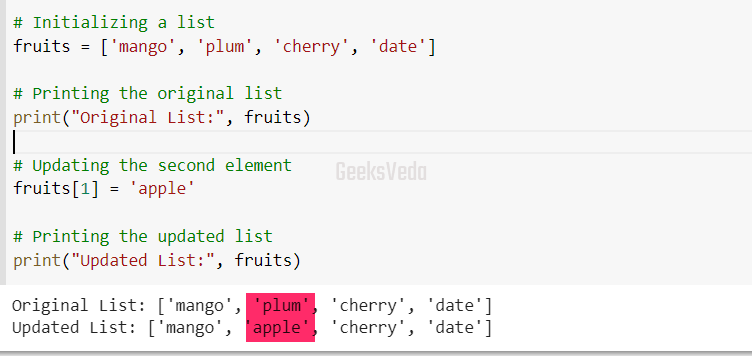
10. How to Delete Elements From a Python List
Deleting elements from a list is a common operation in Python utilized for removing redundant or unwanted elements from a list. There exist several ways to do so. One way is to use the ‘del‘ keyword followed by the index of the element to be deleted.
del my_list[index]
According to the given syntax, the element will be deleted from the my_list found at the given index.
The given code initializes a list named fruits having the given four elements. It then prints the original list with the print()
function. Then, the “del
” keyword is added to delete the second element of the list (which has an index of 1) using the syntax “del fruits[1]
“.
Finally, the updated list is printed by calling the print()
function again.
] # Initializing a list fruits = ['mango', 'plum', 'cherry', 'date'] # Printing the original list print("Original List:", fruits) # Delete the second element del fruits[1] # Printing the updated list print("Updated List:", fruits)
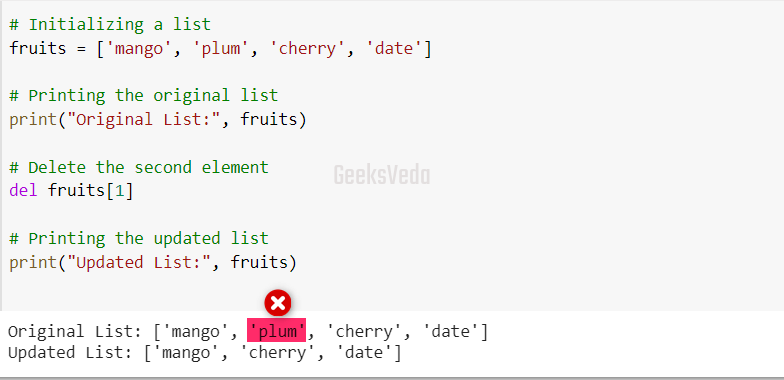
11. How to Insert Elements to a Python List
The built-in insert()
method in Python can be utilized for adding a new element to a list. This method permits you to specify the position or index where the elements should be inserted.
You can use it in case scenarios where it is required to add an element at a particular position rather than spending it at the end of the list.
Follow the below-given syntax for utilizing the insert()
method in Python.
my_list.insert(index, element)
Here, the index is the position where the specified element will be inserted.
Firstly, we have created a list of strings. Then, print the original list. Next, insert a new element “apple” at the 1 index with the help of the insert()
method. Lastly, print the updated list for the verification of the added element.
# Initializing a list fruits = ['mango', 'plum', 'cherry', 'date'] # Printing the original list print("Original List:", fruits) # Inserting a new element at index 1 fruits.insert(1, 'apple') # Printing the updated list print("Updated List:", fruits)
It can be observed that the element apple has been added at the first index successfully.
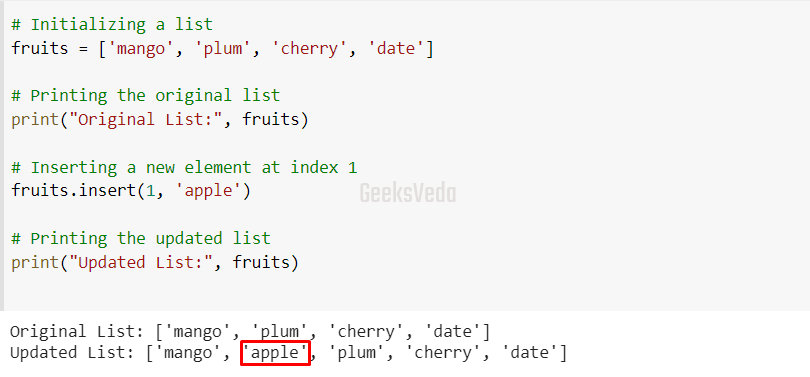
12. How to Sort a List in Python
While dealing with data, sorting is considered another important operation in Python. More specifically, Python offers the sort()
method for sorting a list in either ascending or descending order as per your requirements.
To sort the list in ascending order, use the given syntax.
my_list.sort()
Or utilize “reverse” as the optional argument of the sort()
method and set its value as “True” for sorting the list in descending order.
my_list.sort(reverse=True)
According to the given code block, firstly, we created a list of numbers. Then, use the print()
function for printing the original list on the console.
As the next step, we sorted the list with the sort()
method and again printed the sorted list. Last but the least, the sort()
method is again invoked with the “reverse” argument whose value is set as “True“. This will, resultantly, sort the list in descending order.
numbers = [5, 9, 4, 1, 2, 8, 6, 7, 3, 0] print("Original list:", numbers) # Sorting the list in ascending order numbers.sort() # Printing the sorted list print("Sorted list in ascending order:", numbers) # Sorting the list in descending order numbers.sort(reverse=True) # Printing the sorted list print("Sorted list in descending order:", numbers)
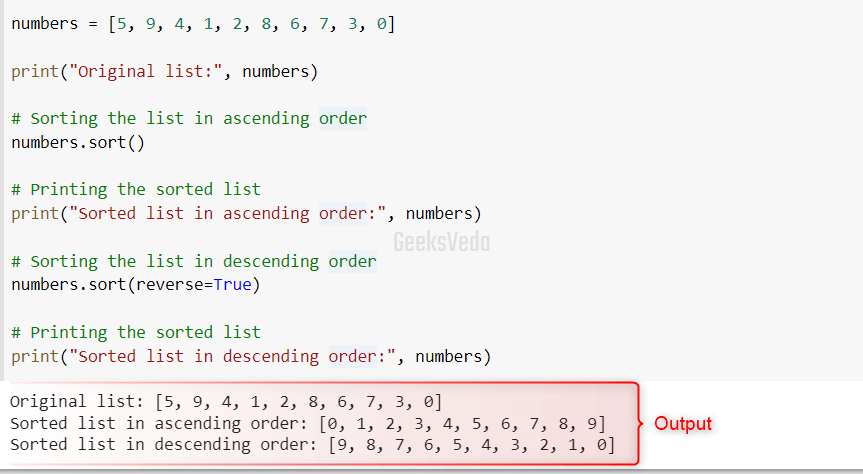
13. How to Reverse a Python List
Reversing a list in Python means modifying the order of the elements from the original list to a reversed one. This can be useful when you need to process the list of elements in a different order.
for instance, from the end to the beginning. More specifically, Python offers the reverse()
built-in method for the discussed purpose.
Check out the provided syntax for using the reverse()
method.
my_list().reverse()
The given example initializes a list called “numbers” with ten integer elements. It then prints the original list by utilizing the print()
function. The list is then reversed using the reverse()
method, and the updated list is printed using the print statement again.
Remember that the reverse()
method doesn’t return anything, it only updates or modifies the original list in place.
numbers = [5, 9, 4, 1, 2, 8, 6, 7, 3, 0] print("Original list:", numbers) # Reversing the list numbers.reverse() # Printing the updated list print("Updated list", numbers)
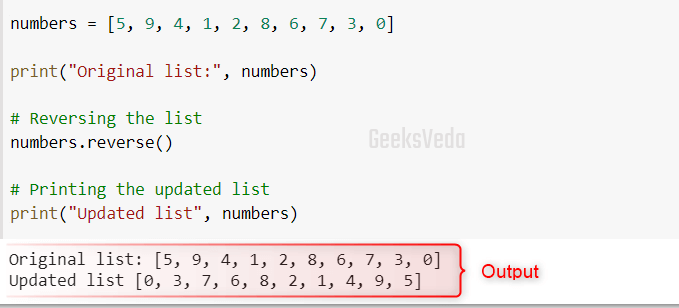
14. How to Search for an Element in a Python List
In Python, the operation of searching for an element can be done by utilizing the “in” keyword or the “index()
” method.
The in keyword outputs a boolean value indicating whether the element is a part or member of the list or not. Whereas, the index()
method outputs the index of the first occurrence of the element in the list.
For in
keyword.
element in my_list
Here, the specified element will be checked in the my_list.
For the index()
method.
my_list.index(element)
Here, my_list is the list where the element will be searched and its index will be returned.
For instance, we have defined a fruit list. Then, use the “in” keyword to verify whether the “apple” and “plum” elements are present in the list. These two statements will return the boolean value accordingly.
After that, we called the index()
method to find the first occurrence of the plum element in the list. The result value will be printed out by utilizing the print()
function.
fruits = ['mango', 'plum', 'cherry', 'date'] # Checking if an element is present in the list print("Apple found:", 'apple' in fruits) print("Plum found:", 'plum' in fruits) # Finding the index of the first occurrence of an element in the list print("Mango found at:", fruits.index('plum'))
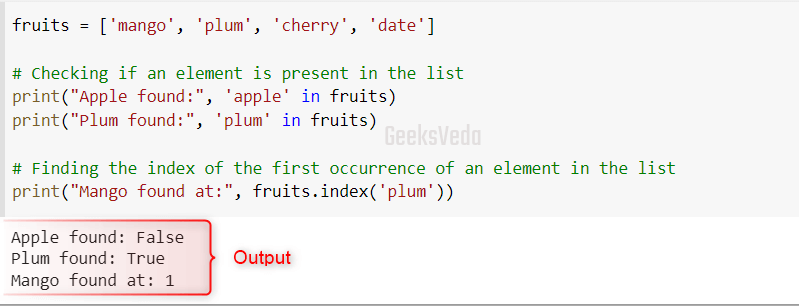
That’s how you can use Python lists in different scenarios.
Conclusion
Every Python developer should be familiar with lists because they are a fundamental data structure. Lists offer a strong approach to handling and manipulating collections of data in your code due to their wide range of functions and flexible capabilities.
More specifically, whether you’re creating a simple script or a sophisticated application, learning Python lists will be a crucial step in your development as a programmer and will level up your skills.
You can also check out our dedicated Python Tutorial series!