Python code is designed to be easy to understand, which makes it an excellent choice for both beginners and professional developers. More specifically, the usage of Comments is one of the ways Python achieves its readability.
Comments are the lines of code that comprise the explanation related to the code and are ignored by the interpreter. They can assist in saving time and improving the overall code quality.
In our previous articles, we discussed Python Operators and Python Functions. In this blog, we will explore the importance of adding comments in Python, their types, and syntax, and practically check out the relevant examples as well.
What are Python Comments
In Python, comments refer to the lines of code that are only meant for the readers and are ignored by the interpreter. These comments are utilized for providing the content, explaining the code, or in case you want to make notes for yourself.
Comments assist developers to get to know about the functionality and purpose of an application code. Moreover, while programming in Python, it is considered a best practice to add comments for better readability of the code.
Why Commenting Your Python Code Is So Important?
Check out the enlisted points to know why commenting is so important in Python:
- Comments assist in explaining the code functionality and its purpose for other developers who may want to work on the same project after some time.
- Adding comments minimizes the chances of confusion and also improves the code readability.
- They make it easy to debug and maintain the code later on.
- You can also identify the code areas where optimization or improvement is required while adding the comments.
- Comments can be also utilized as a reminder for yourself by associating them with certain code parts.
- Well-commented code is easier to understand and it saves a lot of time and effort for the next time when you or any other developer tries to understand it.
- Moreover, comments are sometimes also added to fulfill particular regulations of coding standards.
Types of Python Comments
There exist two major types of Python comments:-
- Single-line Comments
- Multi-line Comments
How to Use Single-line Comments in Python
Code comments that only span over or take up one line are known as Single-line comments. These types of comments are primarily utilized for adding a brief description of the code or making a note about something that is required to be done.
Here is the syntax you have to follow for adding single-line comments in Python.
# This is a sample single-line comment
#
and can be placed at the end of a line or on a separate line.Let’s check out the usage of the single-line comment with the help of some examples.
Example 1: Adding Single-Line Comments in Python
In the following example, we have added two single-line comments. The first comment is added as a note about the code. Whereas, the second comment is placed at the end of the same line as the print statement.
This comment is providing additional explanation or context about the associated line of the code. For instance, it explains that we are calling the print()
function.
# Let's start coding print("Hello GeeksVeda User!") # Calling the print() function
After executing the above code, the string added to the print()
function will be displayed on the console. Both of the added comments will be ignored by the Python interpreter.
Moreover, these comments do not affect the code output.

As seen in the previous example, to provide additional information related to the code, you can add the comment in the same line.
More specifically, the single-line comment that starts with #
and can place at the end of the code line is also known as an Inline comment.
Example 2: Adding Inline Comments in Python
For instance, we have added two inline comments in the following example. The first comment explains the purpose of variable initialization and the second one explains what the print()
function is doing.
For the given program, the Python interpreter will first store the value 50 in the total variable. Then, double its value by multiplying by 2.
Lastly, display the updated value by utilizing the print()
function.

How to Use Multiline Comments in Python
While programming in Python, you can also utilize Multi-line comments for writing a comment that spans multiple lines. These comments are used to add a more detailed explanation of the code, such as what the code does, how it works, and why it is important to perform the given operation.
Moreover, multi-line comments help in documenting modules, functions, and classes in Python.
To add a multi-line comment in Python, specify triple quotes """
at the start and end of the comment.
""" This is a sample multi-line comment It can span multiple lines """
In this section, we will check out two examples related to the usage of multi-line comments in Python.
Example 1: Adding Multiline Comments in Python
In the given code, we have added a multi-line comment that is enclosed in triple quotes at the start and the end. This comment consists of two lines that refer to the additional context and the purpose of the program.
""" This is an example of a multi-line comment The given print() function prints the added string """ print("Hello GeeksVeda User!")
Likewise, executing the given code will display the message added to the print()
function and the added multi-line comment will be ignored by the interpreter.
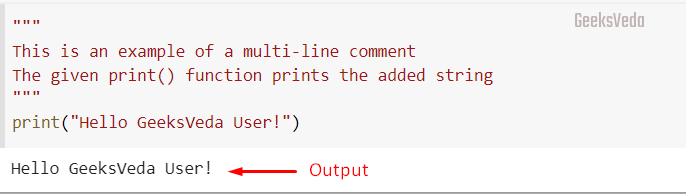
Example 2: Adding Documentation Strings
This example code contains documentation or docstring, a type of multi-line comment utilized for documentation purposes in Python.
Here, the function is defined with the help of the def keyword, followed by the function name sum and then the required parameter is added. The function body includes a docstring explaining what the function will return.
def sum(x): """ This function returns the sum of a number """ return x + 2 print(sum(5)
According to the given code, when the function is called it will return the sum of the passed argument and 2 as 7, and the added docstring will be ignored.
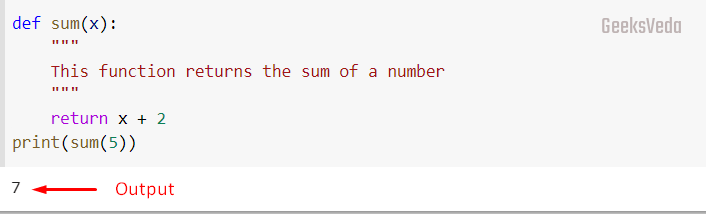
Best Practices for Using Python Comments
Here, we have enlisted some of the best practices for using comments in Python:
- Add comments in Python code to explain why, not what.
- Use a consistent Style.
- Utilize concise and clear language.
- Keep comments up-to-date.
- Reserve inline comments for specifying brief content.
- Use multi-line comments for documentation.
- Don’t over-comment everything.
That was all the generic instructions. Now, let’s practically discuss two specific use cases related to best practices for adding Python Comments.
Write Comments for Yourself in Python
In case you are only writing code for yourself in a Python program, it is easy to have this assumption that you will remember what a particular function does or why you have added a specific line of code.
However, with the passage of time, it is considered normal to forget the purpose of the context of the code. That’s the reason it is crucial to add comments even if you think your memory is too sharp!
Have a look at the below given some of the best practices that you should keep in mind while writing comments for yourself.
Utilize comments for explaining the purpose of a function or a variable.
# This variable stores the user's name username = "Sharqa Hameed" def get_user_email(username): # This function outputs the email address for the specified username. # ...
Add comments in order to explain the decision-making or the relevant thought process, especially if you are working on a complex project.
# We are using a binary search algorithm here because the data is sorted # and we need to find the value as quickly as possible def find_value(sorted_data, target_value): # ...
Describe edge cases or limitations through comments. This operation assists in improving the overall code quality by avoiding bugs.
# This function supposes that the input data is sorted and contains no duplicates def binary_search(data, target): # ...
Write Comments for Others in Python
There could be a scenario where a Python programmer writes code or develops a project that will be handed over to another developer for further operations. If that’s the situation, then it is more important to write clear and concise comments that serve both the purpose and functionality of the code.
These are some of the best practices one should follow while writing Python comments for others.
Add Python comments for explaining the overall purpose of a class, module, or function. This can assist other developers to understand the code easily.
# This module offers utility functions for working with dates and times import datetime def format_date(date): # This function formats the specified date as a string in ISO 8601 format # ...
Utilize comments to describe any limitations or assumptions related to the code. As a result, you will deliver more robust code.
def calculate_tax(income): """ This function supposes that the income is in USD and that there are no deductions or exemptions """
Python comments can be also added for explaining any complex logic or algorithm in the code, or to explain how and why the decisions were made.
# This function utilized a binary search algorithm to find the first occurrence of # the target value in the defined data. If the value is not found, it outputs -1. def binary_search(data, target): # ...
That was all about using Python Comments.
Conclusion
In Python, you can add single-line or multi-line comments using #
or triple quotes """
at the start and the end of the lines, respectively.
Adding these comments depends on your requirements. For instance, whether it is required to define single inline comments or you want to specify the doc strings for documentation purposes.
Moreover, comments in Python are essential for creating understandable, maintainable, and efficient programs.
So keep exploring and keep learning through our Python Tutorials series!