Have you ever been stuck in a situation where you need to find the largest of the three numbers in Python? For instance, when you are sorting data, making decisions based on values, or optimizing algorithms.
Today’s guide is all about exploring different approaches for finding the largest among three numbers. We will discuss different approaches, such as nested if-else statements
, the built-in max() function
, and conditional ternary operations with practical examples.
So, let’s start the guide!
Why Find the Largest Among Three Numbers in Python
In Python programming, determining the largest among three numbers is an essential task in multifaceted applications. It seems simple to do, however, it holds importance in decision-making and facilitating optimized algorithms.
Here are some of the key use cases where identifying the largest among the given numbers is crucial:
- Sorting and Ranking – In sorting algorithms, identifying the largest element is crucial for arranging data.
- Maximum Values – Finding the peak value in data sets aids in understanding data ranges and trends.
- Decision-Making – When choosing the best option from multiple candidates, the largest may signify superiority.
- Optimizing Algorithms – Certain algorithms depend on the maximum value to determine stopping conditions.
- Statistical Analysis – In statistical computations, the largest value can influence measures like outliers.
- Resource Allocation – In resource allocation problems, the largest value might signify the highest demand.
- Data Validation – Ensuring data integrity involves identifying unexpected outliers, often the largest values.
How to Find the Largest Among Three Numbers in Python
In order to find the largest among the three numbers in Python, you can use:
- Nested if-else Statements
- Built-in max() Function
- Conditional Ternary Operator
- List and max() Function
1. Using Nested if-else Statements
Using nested if-else statements is the traditional method for finding the largest among the three numbers. More specifically, by comparing the numbers and the nesting conditions, you can effectively determine the largest number.
For instance, in the following program, we will define a function named “find_largest()” that utilizes the nested if-else statements to compare the three numbers and determine the largest one.
def find_largest(a, b, c): if a >= b: if a >= c: return a if b >= c: return b return c num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) num3 = float(input("Enter the third number: ")) largest = find_largest(num1, num2, num3) print(f"The largest among {num1}, {num2}, and {num3} is {largest}.")
We will now input three values one and one and then check out the largest one.
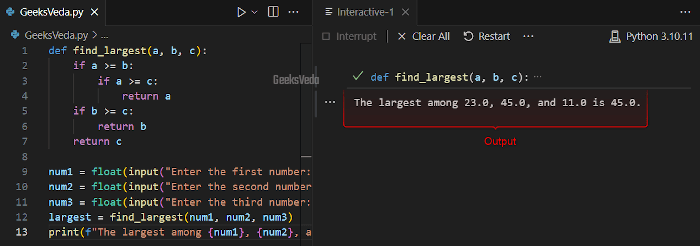
2. Using Built-in max() Function
Python’s built-in “max()
” function simplifies the task of finding the largest among three numbers. By directly passing the numbers as arguments, you can instantly obtain the maximum value.
Here, the “max()
” function will directly compare the three numbers and identify the largest one.
num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) num3 = float(input("Enter the third number: ")) largest = max(num1, num2, num3) print(f"The largest among {num1}, {num2}, and {num3} is {largest}.")
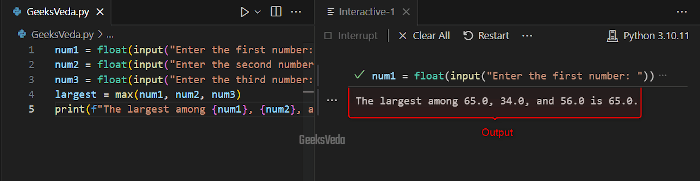
3. Using Conditional Ternary Operator
The conditional ternary operator is a concise approach for finding the largest among the three numbers. By defining a compact conditional expression, you can promptly obtain the largest value.
According to the given program, we will now add the “>” operator for comparing the numbers entered by the user and determining the largest among them.
num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) num3 = float(input("Enter the third number: ")) largest = num1 if num1 > num2 and num1 > num3 else num2 if num2 > num3 else num3 print(f"The largest among {num1}, {num2}, and {num3} is {largest}.")
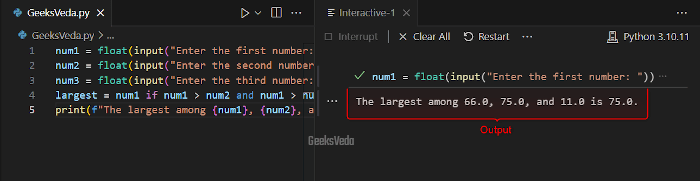
4. Using List and max() Function
Using the combination of list and the max() function can be utilized for simplifying the largest among three numbers. By constructing a list of the numbers and applying the max()
function, you can readily identify the maximum value.
In the given code, firstly, we will store the three input numbers in the “nums” list. After that, we will invoke the max() function with the list for finding the largest number.
nums = [] for i in range(3): num = float(input(f"Enter number {i+1}: ")) nums.append(num) largest = max(nums) print(f"The largest among the entered numbers is {largest}.")
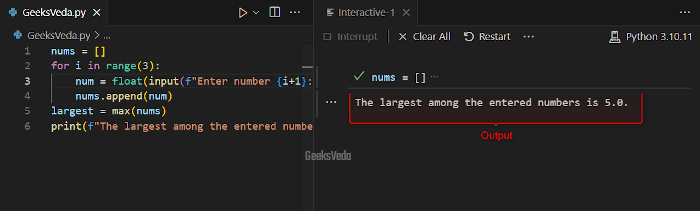
Comparative Analysis of All Methods
We will now compare all discussed methods, and their advantages, disadvantages, and use cases:
Method | Advantages | Disadvantages | Use Cases |
Nested if-else Statements |
|
|
Basic number comparison where control over each comparison is essential. |
Built-in max() Function |
|
|
Quick and straightforward comparison of numbers when readability is a priority. |
Conditional Ternary Operator |
|
|
Compact comparisons in small scripts or functions where brevity and simplicity are valued. |
List and max() Function |
|
|
Situations with larger sets of numbers where memory isn’t a concern and readability is important. |
That brought us to the end of our guide for finding the largest among three numbers in Python.
Conclusion
Finding the largest among the three in Python might seem a common one, but it is no less important. For this purpose, you can utilize nested if-else statements
, built-in max()
function, condition ternary operation, or the use of a list and the max()
function.
Depending on your project requirements, use any of the mentioned approaches and create more efficient algorithms.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!