Today’s guide is all about basic input and output. We will discuss the fundamentals of interacting with users by accepting input, processing and manipulating data, and handling files without any hassle. More specifically, mastering these skills will help you develop interactive and dynamic applications.
So, whether you are a beginner or someone who wants to enhance your Python programming skills, this guide will assist you in understanding the basic input and output capabilities!
What is Input and Output Function in Python
For any programming language, input and output are the fundamental operations. Additionally, Python supports robust functions for handling them.
Input permits a program for receiving data from users or external sources.
On the other hand, output
displays or shows the results or relevant information regarding the program. Moreover, the versatility of Python in input and output represents it as an ideal choice for programmers for creating interactive applications, data processing, and other operations.
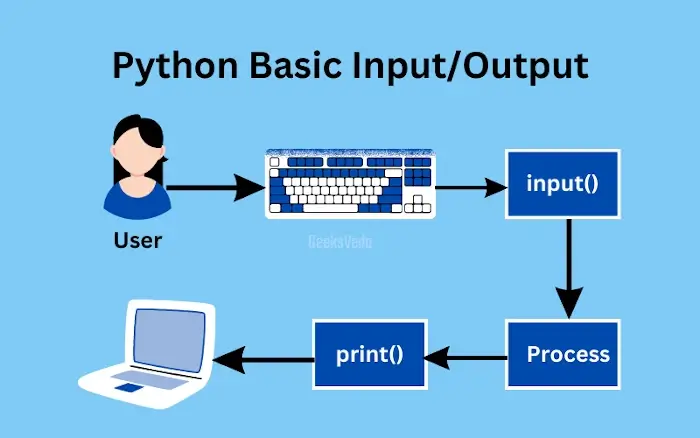
How to Write an Input Function in Python
Python’s built-in “input()
” function enables you to interact with the user by capturing the relevant input from the keyboard. When this function is invoked, the program waits for the user to enter or specify the data and hit the “Enter
” key.
The entered data will be output as a string, which can be further converted to other data types as required.
1. Get Basic Input from the User
For instance, in the provided code, we will take two values from the user, the “name
” and the “age
” with the input()
function. After that, we will print out the accepted values on the console.
# Input Methods - Using input() function name = input("Please Enter your name: ") age = input("Please Enter your age: ") print("Hello, " + name + "! You are " + age + " years old.")
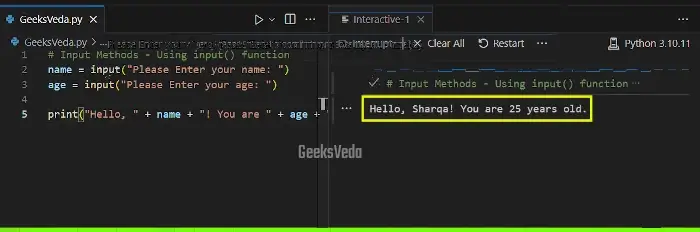
2. Numeric Input and Conversion
In this example, we will input two numeric values from the user, and calculate their sum with the “+
” operator, and store it in the “result
” variable.
# Numeric Input and Conversion num1 = int(input("Enter the first number: ")) num2 = int(input("Enter the second number: ")) result = num1 + num2 print("The sum of the numbers is:", result)
We have entered “25
” as the first number. and “10
” as the second number. Lastly, the resultant sum value has been displayed as “35
“.
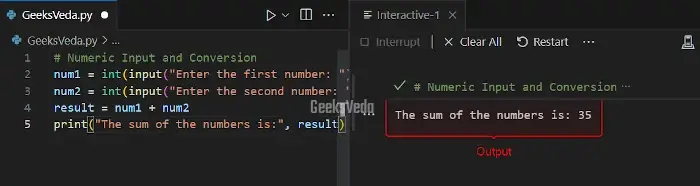
Python Output Function
Output
methods in Python signify the approaches or functions that can be utilized for displaying the results, information, or data produced by the programs.
For this purpose, the print() function is primarily used, while other methods involve writing to files, formatting output, and working with standard streams.
1. Print Output with print() Function
Python’s “print()
” function can be invoked for showing the output on the screen. It accepts one or more arguments separated by commas and displays them on the same line.
This means that the “print()
” method automatically adds a space between them. More specifically, the respective output will be displayed on the console, which permits you to view the program results.
In the below program, we will print the values of the created variables “name
” and “age
” with the “print()
” function.
# Printing Output with print() function name = "Sharqa Hameed" age = 25 print("Name:", name, "Age:", age)
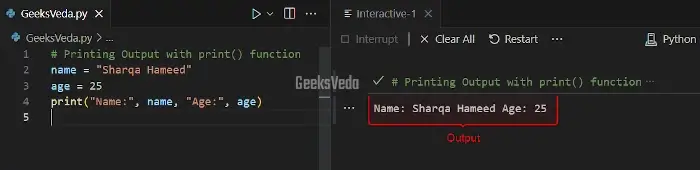
2. Format Output in Python
Python supports two powerful methods for the purpose of formatting outputs with placeholders. These methods are “f-strings
” or formatted string literals and the second one is “str.format()
“.
These methods permit you to insert variables or values into strings without any hassle.
For example, firstly, we created two variables, “name
” and “age
” and assigned them their respective values. After that, using the “print()
” function and using f-string
, we displayed the formatted output.
name = "Sharqa Hameed" age = 25 print(f"Hi, my name is {name} and I am {age} years old.")
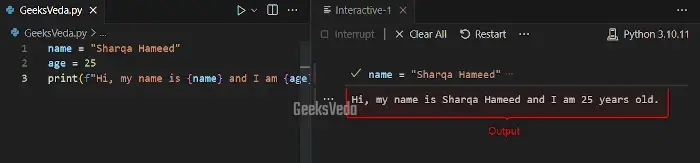
In the second example, “str.format()
” is utilized for formatting the output string. Note that the curly braces “{}
” serve as the placeholders for the variables and the “format()
” method adds or inserts the values in the corresponding positions.
# Formatting Output with str.format() item = "book" price = 30 print("I bought a {} for ${:.2f}.".format(item, price))
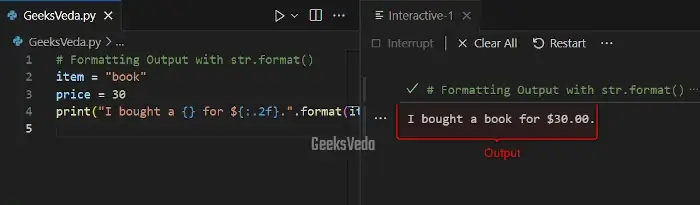
3. Write Output Function to Files
In terms of data processing and storage, writing output to files is a crucial aspect. Therefore, Python permits you to create, open, and write data files with the open() function in combination with the write() method.
Here, we will first open the “GeeksVeda.txt
” text file in write or “w
” mode and then invoke the “write()
” method for writing the added string to the mentioned file.
# Writing Output to Files with open("GeeksVeda.txt", "w") as file: file.write("We are writing to the GeeksVeda file.")
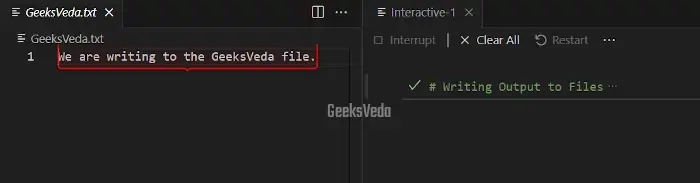
Python Input and Output: Standard Streams
Python standard streams are the default input/output channels that are linked with the running program or script. These streams are represented by:
- stdin: It handles input from the user.
- stdout: It displays output to the screen.
- stderr: It is utilized for error messages.
In the following program, the input()
function will read the user name and age from the “stdin
“. After that, we will multiply age by “2
” and write the output to the “stdout
” with the “sys.stdout.write()
” method.
Then, if the specified age is negative, the “ValueError
” will be raised and the error message will be written to “stderr
” by invoking the “sys.stderr.write()
” method.
import sys def main(): try: # Reading input from stdin name = input("Enter your name: ") age = int(input("Enter your age: ")) # Performing some operation and writing output to stdout result = age * 2 sys.stdout.write(f"Hello, {name}! Your age multiplied by 2 is {result}.\n") # Generating an error and writing error message to stderr if age < 0: raise ValueError("Age cannot be negative.") except ValueError as ve: # Writing error message to stderr sys.stderr.write(f"Error: {str(ve)}\n") if __name__ == "__main__": main()
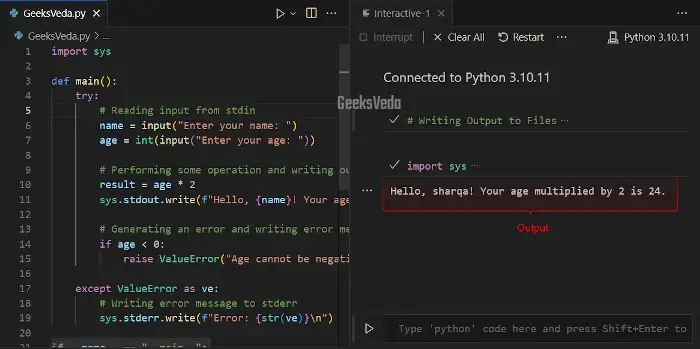
Read and Write Text Files in Python
In Python, reading and writing text files is a common operation that can be performed in file handling and data processing. Additionally, Python supports built-in functions for interacting with text files.
As discussed above, the “open()
” function enables you to read the content of the text file when its mode is specified as “r
“.
# Reading Text Files with open("GeeksVeda.txt", "r") as file: content = file.read() print(content)
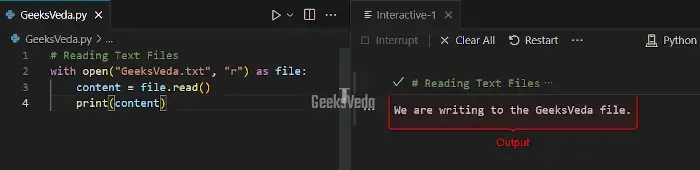
Similarly to write some data to a text file, add the “w
” mode in the open()
function as follows.
# Writing Text Files data = "New line added" with open("GeeksVeda.txt", "w") as file: file.write(data)
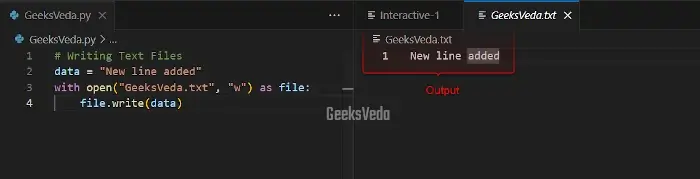
input()
” function and its usage, refer to this guide.That’s all about basic input and output in Python.
Conclusion
Handling Python’s basic input and output is considered essential for any programmer. These operations permit you to interact with the users, process and manipulate data, and work with files.
So, understand these concepts for creating robust applications and handling data with ease.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!