Welcome to the world of Python numbers. From integers to floats and complex numbers, Python provides powerful functionalities for numerical computations.
So, let’s dive into this guide to understand numeric data types in Python, numeric operations and expression, type casting, built-in number functions, random number generation, and number formatting!
What is Numeric Data Type in Python
A numeric data type is a category of data types that represent numbers in a programming language. Numeric data types are used to store and manipulate numerical values such as integers, floating-point numbers, and complex numbers.
In most programming languages, including Python, numeric data types are built-in and provide different levels of precision, range, and functionality. These data types are designed to handle numerical calculations and operations efficiently.
Different Numeric Data Types Used in Python
Python offers multiple numeric data types that can be utilized for working with different types of numbers. In this section, we will explore some of the majorly utilized data types, such as int, float, and complex.
1. Python Integer (int) Data Type
Integers are whole numbers that do not comprise a fractional component. These numbers can be zero, negative, or positive.
The integer or int data type permits you to perform several types of operations and manipulation on integers, like subtraction, addition, division, and multiplication.
For instance, in the provided code, we have created two variables “x
” and “y
“. Then, we used the addition operator “+
” for calculating their “sum
” which is stored in the respective variable.
Lastly, the print() function displays the value of the sum variable on the terminal.
x = 11 y = 55 sum = x + y print("Sum:", sum)
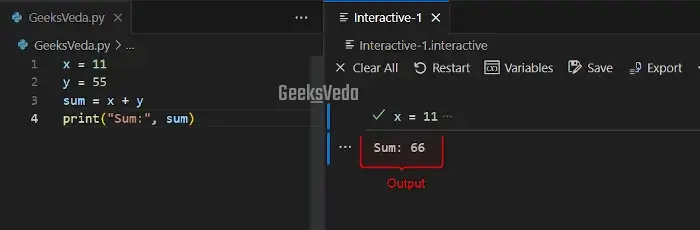
2. Python Float Data Type
Floating-point numbers refer to decimal values having fractional parts. These numbers offer more precision than integers. However, they also have limitations because of their finite representation.
Additionally, this data type supports different mathematical functions and operations.
Here, we have defined the “radius
” as a float value. After that, we specified the formula to calculate the area of the circle which will be displayed as output.
radius = 6.9 area = 3.14 * radius ** 2 print("Area: ", area)
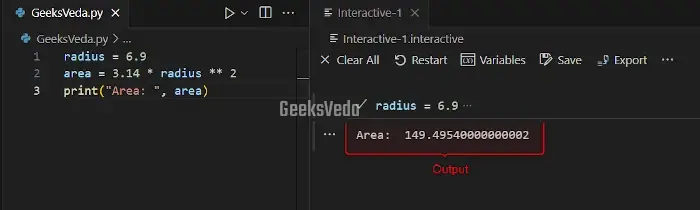
3. Python Complex Data Type
Complex numbers indicate the quantities that can be both imaginary and real components. They are written in the form of “a + bj
”, where “a
” and “b
” are the real numbers, and “j
” signifies the imaginary part. This data type also supports arithmetic functions and operations.
In the given example, two complex numbers have been defined named “z1
” and “z2
“. Then, we performed the addition operation on them and stored their respective value in the “sum
” variable, which will be ultimately displayed on the console.
z1 = 2 + 3j z2 = 1 - 2j sum = z1 + z2 print("Sum: ", sum)
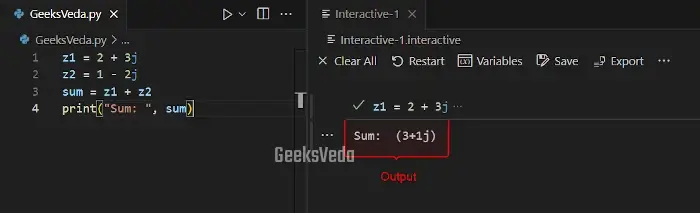
Numeric Operations and Expression in Python
Python allows you to perform different types of numeric operations, such as.
- Arithmetic Operations: Addition, Subtractions, Multiplications, Division, Exponentiation, Modulus
- Comparison and Logical Operations: Equal to, Not Equal to, Greater than, Less than, Greater than equal to, Less than equal to, AND, OR, and NOT.
- Bitwise Operators: Bitwise AND, OR, XOR, NOT, Left shift, and Right shift.
- Membership Operators: in, not in.
- Identity Operators: is, is not.
Numeric Data Types Conversion in Python
In Python, you can easily convert different data types and perform typecasting when required. More specifically, one data type can be converted into another by using implicit or explicit conversion or typecasting.
1. Implicit Type Conversion
The process in which Python automatically converts numbers between compatible types when needed is known as Implicit conversion. For instance, when you perform any arithmetic operation that involves different data types, the interpreter then coerces the operands into a common type, like the below-given example.
x = 11 y = 4.5 sum = x + y print("Sum:", sum)
As you can see, we have tried to add the int type variable “x
” and a float type variable “y
“. So, the interpreter will perform the implicit conversion and convert the integer to float even before addition and also display the result in floating numbers.
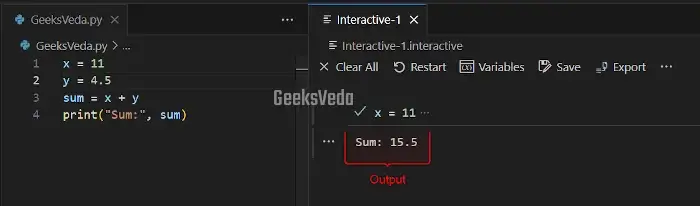
2. Explicit Type Conversion
Python also offers built-in functions that can be invoked for converting values to integers, float, or complex data types as per requirements. Therefore, in case you want to explicitly convert numbers from one type to another, use type casting.
Here, we have converted the “x
” float value to an integer with the “int()
” function. Resultantly, the values will be stored in the “y
” variable.
x = 12.56 y = int(x) print(y)
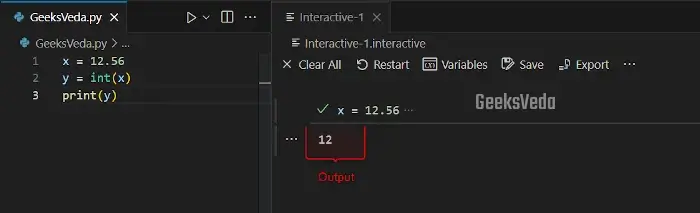
Type Casting Functions in Python
Now, let’s discuss the three main type casting functions that are.
int()
Functionfloat()
Functioncomplex()
FunctionBelow-given sub-sections will define each of the mentioned functions with their relevant examples.
1. Using int() Function
The “int()
” Python function converts the given value into an integer data type. It accepts a string or numeric argument and outputs an integer. However, in case, if the argument is a float, it deletes or truncates its decimal part.
For instance, in the provided code, the “int()
” function will convert the float value of the “x
” variable into an integer.
x = 10.5 y = int(x) print(y)
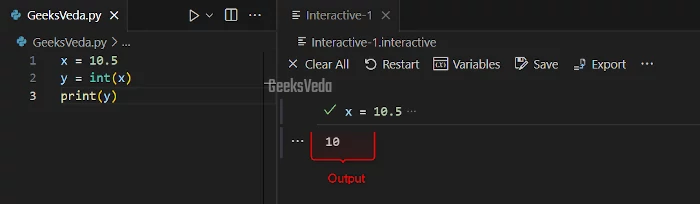
2. Using float() Function
Likewise, the “float()” function can be invoked for converting a value to a floating-point number or data type. It accepts a string or numeric argument and outputs a float.
Here, the “float()
” function converts the given string value of the “x
” variable into the float data type and stores the value in the “y
” variable.
x = "3.14" y = float(x) print(y)
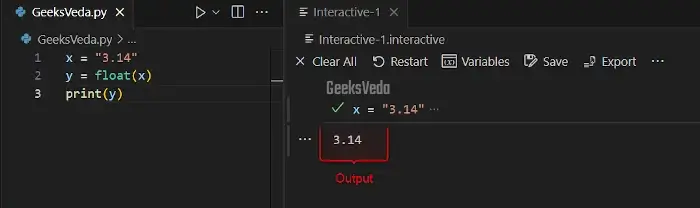
3. Using complex() Function
In case, if it is required to create a complex number object, use the “complex()
” Python function. This function accepts two optional arguments: the first one represents the real part and the second argument indicates the imaginary part.
However, if only one argument is passed, it is considered the real part, and the imaginary part is assumed zero.
In the following program, the “complex()
” function creates a complex number “5+6j
“, where 5 is the real part and 6 is the imaginary part.
x = complex(5, 6) print(x)
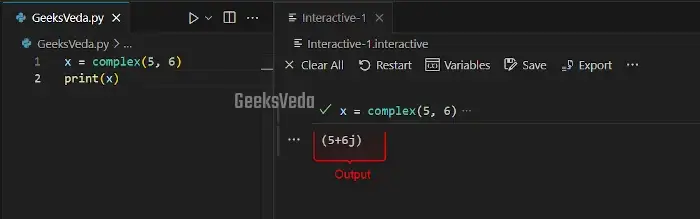
Python Built-in Number Functions and Modules
Python supports multiple built-in modules and functions that provide advanced capabilities for working with numbers.
For example, the “math
” module in Python is like an inventory of mathematical functions. It contains functions for logarithms, trigonometry, exponentiation, rounding, and more.
Let’s invoke the “sqrt()
” method. To do so, firstly import the “math” module in the program. Then, define an integer “x
” having the desired value. After that, call the “sqrt()
” method for calculating the square root of the x
variable.
import math x = 36 y = math.sqrt(x) print(y)
It can be observed that the square root of “36
” has been printed out as “6.0
“.
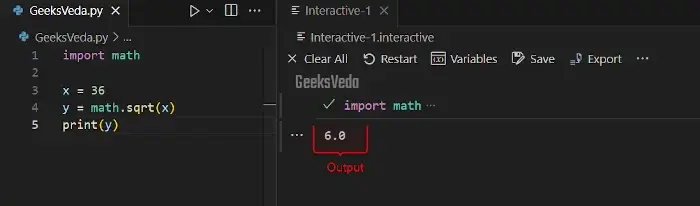
Generate random Number in Python
The “random
” module of Python enables you to generate random numbers. It offers methods that can be utilized for generating random floating-point numbers, and integers, and making random choices from the sequences.
In the following example, we have used the “randint()
” method of the random module for generating a random number between “11
” and “20
“.
import random x = random.randint(11, 20) print(x)
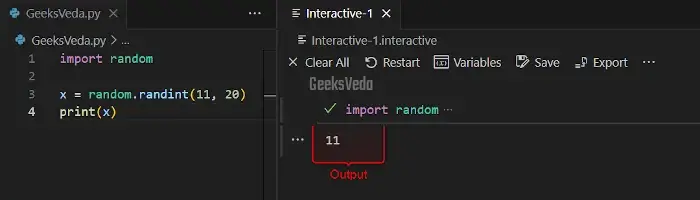
Number Formatting and Output in Python
Python also offers the facility of formatting numbers using various functions and methods. One such popular method is to use the “f-strings
” for formatting numeric values with particular padding, precision, or formatting options.
For instance, here, we have utilized the f-string for formatting the value of the “x
” variable with two decimal places and displaying them as formatted strings.
x = 3.14159 formatted_number = f"The value of pi is approximately {x:.2f}" print(formatted_number)
It can be observed that the formatting has been applied successfully.
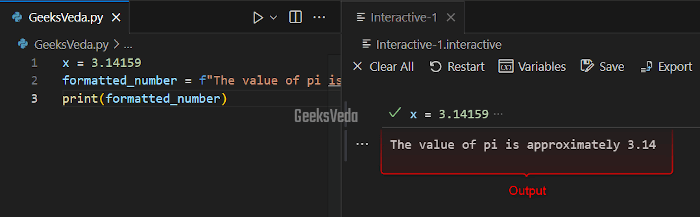
That was all related to Python numbers, their data type, conversion, and formatting.
Conclusion
Understanding the Python number system is essential for you as it is employed in multiple applications, such as financial analysis and scientific calculations. Python offers a robust and versatile set of tools for working with numbers.
From mathematical functions to typecasting, number formatting, and random number generation, Python has the ability to handle complex numeric computations with ease.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!