In today’s guide, we will dive into the usage of Python tuples which are versatile data structures with unique characters. Whether you are an intermediate Python programmer or a beginner, understanding tuples is like a key to optimizing your program.
So, let’s start this guide and discover the immutable nature, usage of Python tuples, and several operations that you can perform for manipulating and accessing data.
What is Tuples in Python
Python tuples are known as immutable ordered collections of elements. Some Python programmers think they are completely similar to Python lists but that’s not the case. The key difference is that a tuple
cannot be updated or modified after its creation.
Additionally, tuples
can be defined with the help of parentheses and can comprise elements of multiple data types.
How to Create and Access Tuples in Python
In Python, the process of creating a tuple
is simple. You can define a tuple by enclosing or embedding the relevant elements in parentheses “()
“.
However, for accessing the elements of a tuple, utilize slicing or indexing.
Check out the given examples as practical demonstrations.
1. How to Create a Tuple in Python
First of all, let’s create a Python tuple named “my_tuple
” that comprises three integers and three string elements. Then, print the tuple elements on the console.
my_tuple = (1, 2, 3, 'a', 'b', 'c') print(my_tuple)
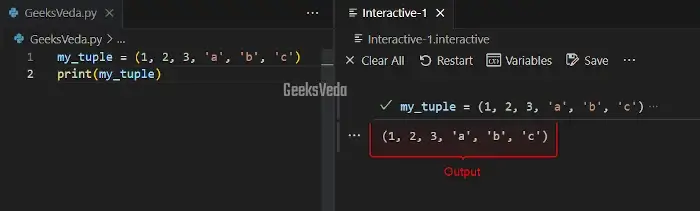
2. How to Access Elements of a Tuple in Python
Accessing Elements of a Tuple
Now, use the indexing technique for accessing the elements of the tuple. For instance, here, we will access the first and last element of the “my_tuple
” by specifying the index as “0
” and the negative index is “-1
“, respectively.
my_tuple = (1, 2, 3, 'a', 'b', 'c') print("First Element:", my_tuple[0]) print("Last Element:", my_tuple[-1])
It can be observed that the first and the last element of the “my_tuple
” have been accessed successfully.
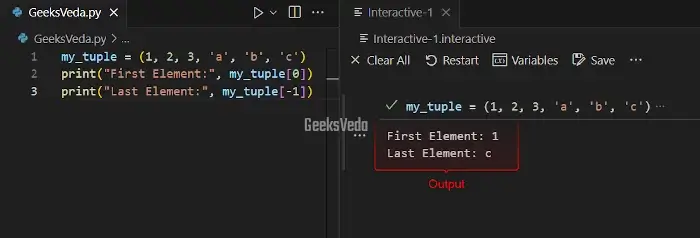
Tuple Operations and Tuple Manipulations in Python
In Python, you can perform different types of tuple operations and manipulations on the tuple, such as concatenation, repetition, and slicing. These operations can assist you in creating new tuples or modifying existing ones.
1. Add/Concatenate Elements to Tuple
Now, we have created a tuple named “my_tuple
” and added two new elements to it. In other words, we have concatenated it with another tuple with “+
” operator.
Resultantly, a new tuple named “new_tuple
” comprises all elements from both tuples.
my_tuple = (1, 2, 3) new_tuple = my_tuple + ('a', 'b') print(new_tuple)
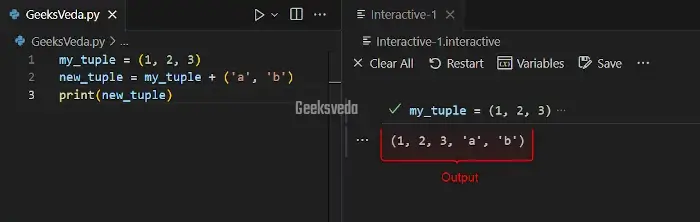
2. Update Elements of a Tuple
Let’s slice the “my_tuple
” for extracting or fetching the first three elements “[:3]
” and the element at index 4 is”[4:]
“.
Then, create a new tuple named “updated_tuple
” by concatenating all of these slices with the “x
” element.
Lastly, display the resulting updated tuple on the console.
my_tuple = (1, 2, 3, 'a', 'b', 'c') print("Original Tuple:", my_tuple) updated_tuple = my_tuple[:3] + ('x',) + my_tuple[4:] print("Updated Tuple:", updated_tuple)
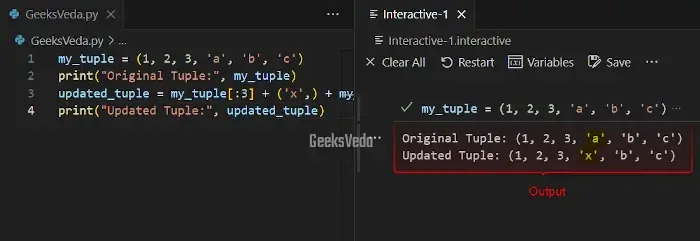
3. Delete Elements From a Tuple
Here, we are removing the element present at index 2 from the “my_tuple
” by concatenating the slices “[:2]
” and “[3:]
“.
This makes sure that the resulting tuple does not contain the third element.
my_tuple = (1, 2, 3, 'a', 'b', 'c') new_tuple = my_tuple[:2] + my_tuple[3:] print(new_tuple)
As a result, the third element from the “my_tuple
” will be removed.
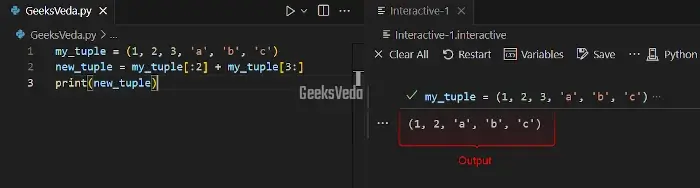
How to Pack and Unpack a Tuple in Python
Python tuple packing is referred to as the process of creating a tuple by assigning multiple values to a single variable. On the other hand, tuple unpacking permits you to assign the tuple elements to multiple variables.
These techniques can be specifically utilized when it is required to assign values from a tuple to separate variables.
In the provided example, we will create a tuple named “coordinates
” having two values. After that, assign the values of the created tuple to the variables “x
” and “y
” as tuple unpacking.
Lastly, display the corresponding values on the console.
coordinates = 3, 4 x, y = coordinates print("x =", x) print("y =", y)
Built-in Tuple Functions and Methods in Python
Python also offers multiple built-in methods and functions that can be utilized with tuples
.
These functions and methods enable you to perform multiple operations on tuples, like finding the maximum or minimum values, finding the length of the tuple, calculating the sum, and more.
Now, look at the given examples.
1. Using len() Function
The “len()
” Python function can be invoked for finding the length of the length.
For instance, we will now find the length of the “my_tuple
” and display it.
my_tuple = (1, 2, 3, 4, 5) print("Length:", len(my_tuple))
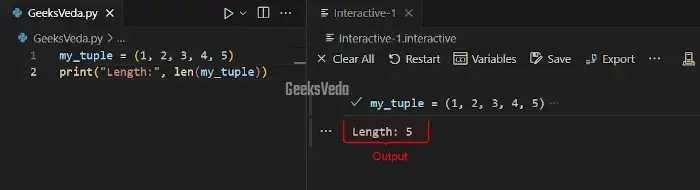
2. Using max() Function
The “max()
” function outputs the maximum values from a tuple. Let’s find the maximum value that is present in the “my_tuple
“.
my_tuple = (1, 2, 3, 4, 5) print("Maximum Value:", max(my_tuple))
It can be observed that “5
” is the maximum value of “my_tuple
“.
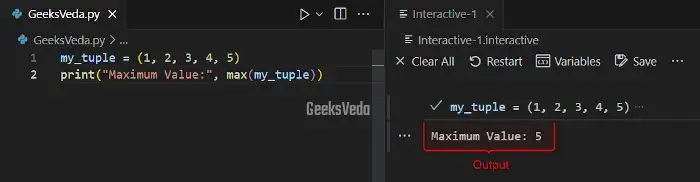
3. Using min() Function
Python “min()
” function outputs the minimum values from a tuple as follows.
my_tuple = (1, 2, 3, 4, 5) print("Minimum Value:", min(my_tuple)) As you can see, “1” is the minimum value found in “my_tuple”.
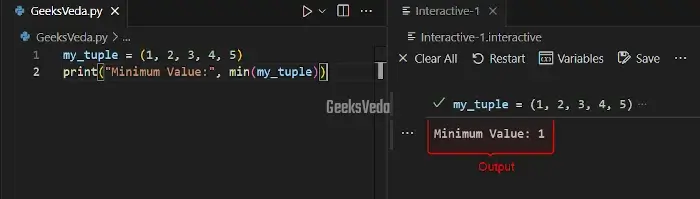
4. Using sum() Function
In case you want to calculate the sum of all of the elements of the created tuple, invoke the “sum()
” function as follows.
my_tuple = (1, 2, 3, 4, 5) print("Sum:", sum(my_tuple))
“15
” has been displayed as the sum of all elements of “my_tuple
“.
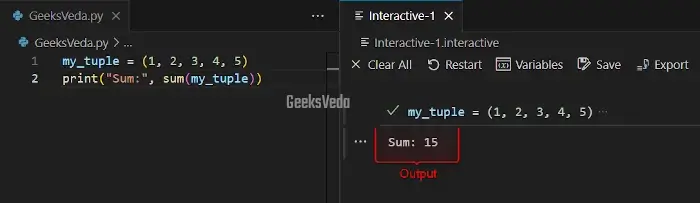
5. Using index() Method
The “index()
” method of Python can be utilized for finding the index of a certain value in the tuple. Here, in the provided example, we will try to find the index of the “3
” element in the defined “my_tuple
“.
my_tuple = (1, 2, 3, 4, 5) print("Index of 3:", my_tuple.index(3))
The given output signifies that 3 is present at the second index.
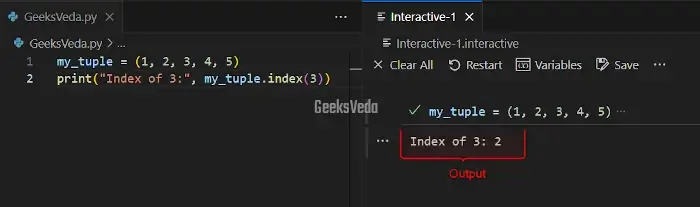
6. Using count() Method
You can also invoke the “count()
” method for displaying the number of occurrences of a particular value in a tuple.
For instance, here, we are counting the number of times “4
” is present in “my_tuple
“.
my_tuple = (4, 1, 2, 3, 4, 5, 4) print("4 is present", my_tuple.count(4), "times.")
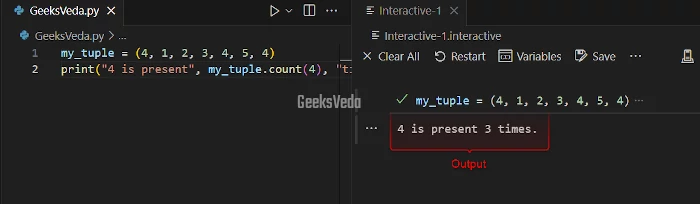
Difference Between Tuple and List in Python
Now, let’s have a look at the below-given table to know the key differences between tuples and lists in terms of their features.
Feature | Tuples | Lists |
Syntax | Parentheses “()” | Square brackets “[]” |
Mutability | Immutable | Mutable |
Creation | Faster | Slower |
Access | Faster | Slower |
Memory Usage | Less memory | More memory |
Suitability | Constant values, fixed data | Data manipulation, dynamic data |
Functionality | Limited methods and operations | Extensive methods and operations |
Typical Usage | Utilized for data integrity, hash keys | Utilized for storage, data manipulation |
Iteration | Faster | Slightly slower |
Sorting | Slower (immutable nature) | Faster |
Extensibility | Limited to concatenation and repetition | Can be appended, modified, or removed dynamically |
Create Dictionary Keys From Tuple in Python
Tuples
can be utilized as the keys in the dictionaries and the elements in the sets respectively. In the provided example, we demonstrated the usage of the tuple as the key in the dictionary.
Here, the tuples ('Sharqa', 25)
and ('Ravi', 30)
are serving as the keys, and their respective values “Pak
” and “Ind
” can be accessed using the keys.
# Using tuples as dictionary keys my_dict = {('Sharqa', 25): 'Pak', ('Ravi', 30): 'Ind'} # Accessing values using tuple keys print(my_dict[('Sharqa', 25)]) print(my_dict[('Ravi', 30)])
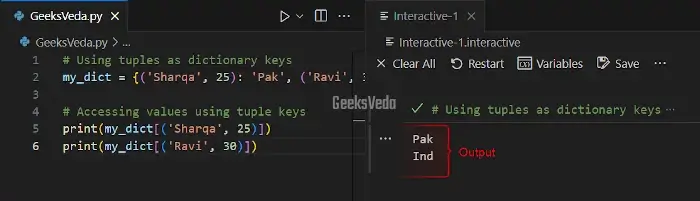
Create a Set Element of Tuple in Python
Now, let’s utilize the Python tuples as elements in a set. Here, “my_set
” comprises the tuples having fruit names and their respective colors.
in
” has been used for verifying if the mentioned tuples exist in the set or not.# Using tuples as set elements my_set = {('apple', 'red'), ('banana', 'yellow')} # Checking set membership print("Apple Found:", ('apple', 'red') in my_set) print("Orange Found:", ('orange', 'orange') in my_set)
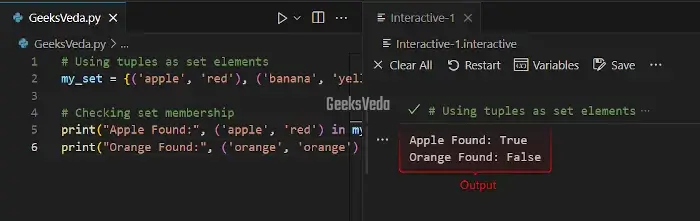
Nested Tuples in Python
Nested tuples can be created by including tuples inside other tuples. For instance, the given program demonstrates the creation of the nested tuple, where elements are going to be accessed using indexing.
More specifically, by accessing specific indexes, we will retrieve the required values within the nested tuples.
# Creating nested tuples person = ('Ravi', ('Sharqa', 25), ('Ind', 'Pak')) # Accessing elements of nested tuples print(person[0]) print(person[1][0]) print(person[1][1]) print(person[2][0]) print(person[2][1])
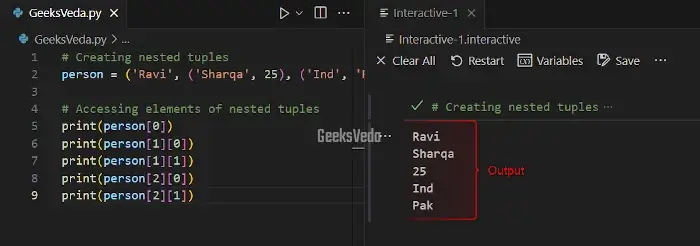
Python Tuples Comprehension
In Python, tuples comprehension enables the creation of tuples with concise syntax. For instance, the provided example can be utilized for generating a tuple of squares of numbers from 1 to 5.
# Tuple comprehension for squares of numbers squares = tuple(x ** 2 for x in range(1, 6)) print(squares)
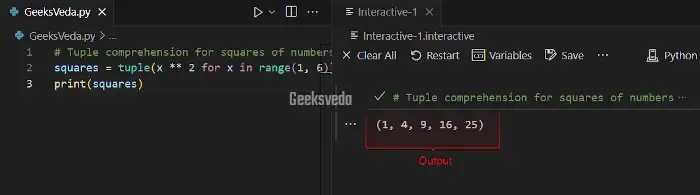
Now, in the second example, we will generate a tuple of even numbers from 1 to 10. With the help of the for loop and the range function, the numbers that are not divisible by 2 will be filtered out.
# Tuple comprehension for even numbers evens = tuple(x for x in range(1, 11) if x % 2 == 0) print(evens)
Here is the tuple comprising the even numbers from 1 to 10.
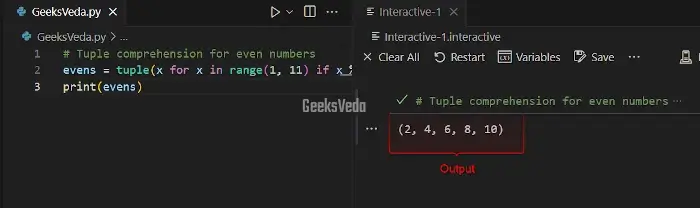
That sums up our today’s effective guide related to Python tuples and their usage.
Conclusion
Python tuples are ordered and immutable data structures with several use cases and operations. These data structures provide efficient memory usage and can also be utilized as the dictionary keys and set elements.
Therefore, understanding tuples is crucial for you to make your program more efficient.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!