Are you looking to understand how slicing works in Python? Slicing is a robust feature that permits you to extract or retrieve specific subsets of data from strings, lists, or tuples.
In today’s blog, we will explore the ins and outs of slicing in Python, from extracting substrings to accessing elements with precision.
So, discover the versatility of slicing and learn best practices to optimize your code for efficient data manipulation and analysis through this guide.
What is Slicing in Python
In Python, slicing offers a robust way of manipulating strings. It enables you to reverse strings, retrieve substrings, and perform several other operations. Using this feature, you can access a subset of elements from a sequence based on their indices.
More specifically, slicing can be achieved using the slicing operator “:
” which can be placed inside the square brackets.
How Does Slicing Work in Python
To perform slicing, you have to define the “start
” and “stop
” indices within the square brackets as follows.
text[start:stop]
In this scenario:
- The
start
index refers to the first element that is required to be included in the slice. - The “
:
” is the slicing operator. - The stop index signifies the index of the element just after the last one that will be included.
As a result, the slice will comprise elements from the start index up to the last index. Note that the last index will be exclusive (not included).
How to Slice Strings in Python
Extracting substrings is another common use case of the slicing string in Python. This operation permits you to fetch a particular substring or portion from a string.
For instance, in the following program, we have created a string named “str
” having the given value. Now, we want to extract a substring that will start from the “7
” index and ends at “16
“. To do so, the string slicing operation will be given as “str[7:16]
“.
str = "Hello, GeeksVeda User" substring = str[7:16] print(substring)
The start index is “7
” which represents the character “G
” and the stop index is “16
“, which represents the space before the “U
” character. So, in this case, the resulting substring will be “GeeksVeda
“.
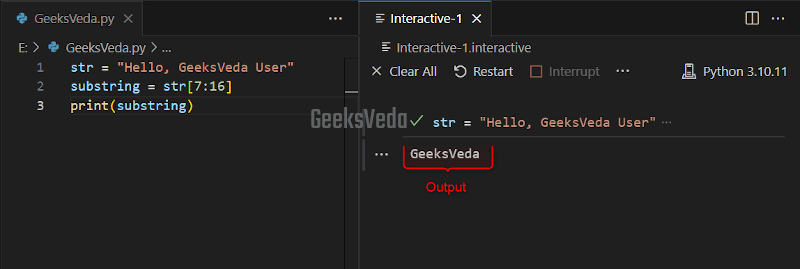
To reverse the given string, you can pass the negative step value as “-1
” without specifying the start index.
str = "Hello, GeeksVeda User" substring = str[::-1] print(substring)
As you can see, the value of the “str
” string has been displayed in reverse order.
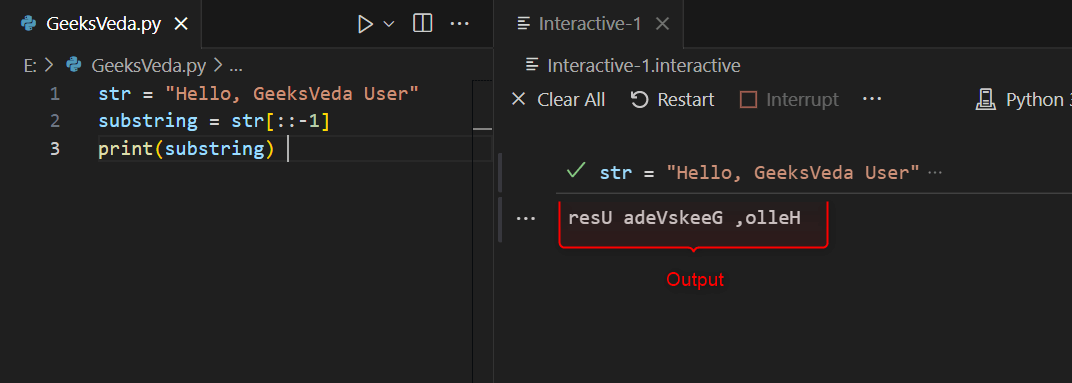
How to Slice Lists and Tuples in Python
You can also apply the slicing technique to lists and tuples. Slicing these data structures provides different benefits like retrieving particular elements from a tuple or extracting sublists.
Let’s check out some relevant examples.
In the first example, we have created a list named “numbers
” that has 5 elements. Then, we applied the slicing technique to slice it using the index “2
” to access the respective element which is 33. Lastly, this element will be displayed on the console using the print() function.
numbers = [11, 22, 33, 44, 55] element = numbers[2] print(element)
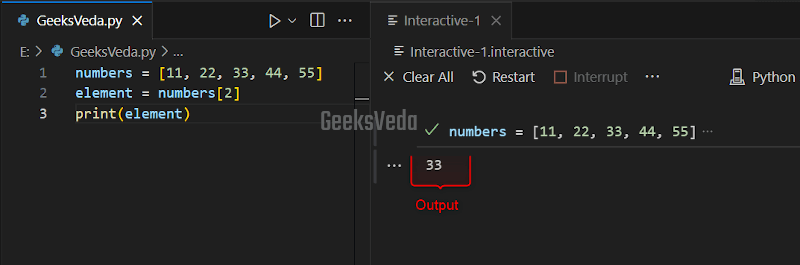
As the second example, we have a “colors” list having five elements. Then, we will apply to slice to extract a sublist which will start from the index “1
” till “4
“. However, the element located at the fourth index will be exclusive.
colors = ['pink', 'blue', 'orange', 'yellow', 'black'] sublist = colors[1:4] print(sublist)
Here, it can be seen that the resultant slice contains three elements.
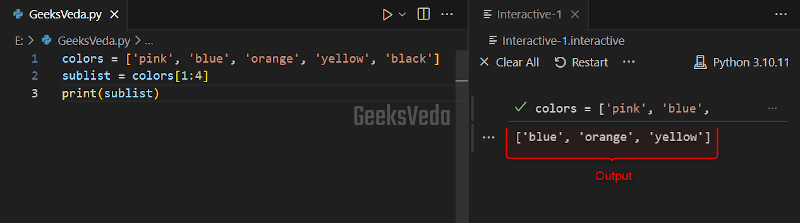
Advanced Slicing Techniques in Python
Python also offers support for advanced slicing techniques. These approaches provide more control and flexibility over the resulting subset. Some of the advanced slicing techniques in Python include skipping the start, stop, or step parameters, using negative steps for reverse slicing, or utilizing a stride.
Let’s check out each of them individually.
Skipping Start, Stop, or Step Slicing Parameters
While slicing in Python, skipping start, stop, or step parameters can assist in achieving the desired results. For instance.
- Skipping the start parameter sets the slice defaults to the sequence beginning.
- Skipping the stop parameter sets the defaults to the sequence end.
- Skipping the step parameter sets the defaults to step one.
In this program, we have practically demonstrated the above-stated concept of the slicing parameters.
num = [10, 2, 3, 6, 8, 9, 4, 5, 1, 7] print("Omit the start parameter", num[:5]) print("Omit the stop parameter", num[5:]) print("Omit the step parameter", num[::2])
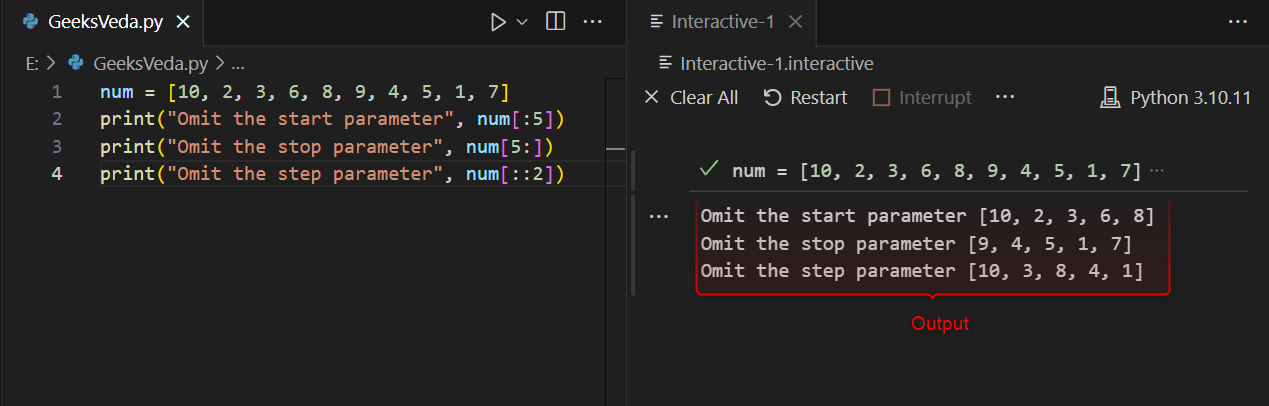
Slicing With a Stride in Python
Now, let’s discuss more about the step parameter of the slicing technique. This parameter determines the stride which defines the number of elements that will be skipped between each element in the resulting slice. Moreover, by adjusting the value of the step parameter, you can retrieve the elements at regular intervals from a sequence.
In the provided code, the stride is set to “2
“, which will make sure that every second element is included in the sliced substring.
num = [10, 2, 3, 6, 8, 9, 4, 5, 1, 7] print(num[1:8:2])
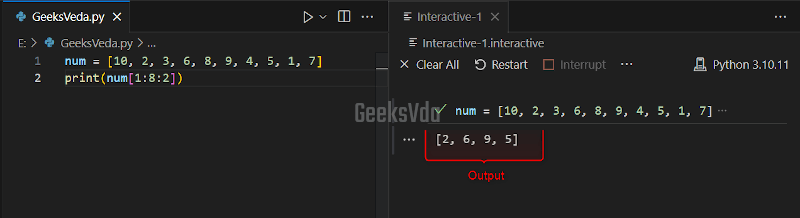
Reverse Slicing with Negative Step in Python
While slicing in Python, a negative step is specified when you particularly need to involve reverse sequences. More specifically, it permits you to reverse the order of the elements in the resultant slice as follows.
msg = "Hello, User!" print(msg[::-1])
It can be observed that the negative step value “-1
” reversed the msg string value as “!resU ,olleH
“.
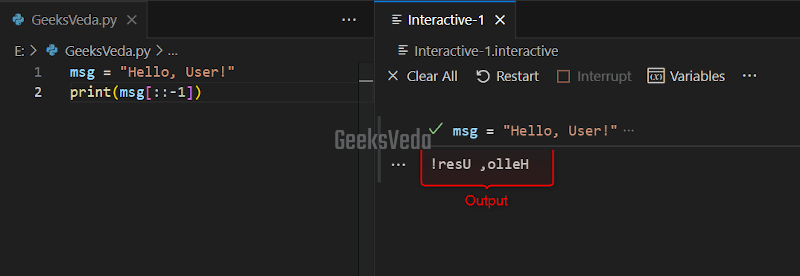
Common Use Cases of Slicing in Python
In Python, the slicing technique is widely utilized in different real-world scenarios. It provides a convenient way for extracting and manipulating data from the sequence.
So, look at some of the common uses of Slicing.
Using Slicing to Filter Data
You can use slicing for the operations related to data filtering. This use case addresses the need to fetch specific subsets from a dataset based on the given condition.
For example, from the given list, we will extract the even numbers by specifying “2
” as the step value.
num = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_num = num[1::2] print(even_num)
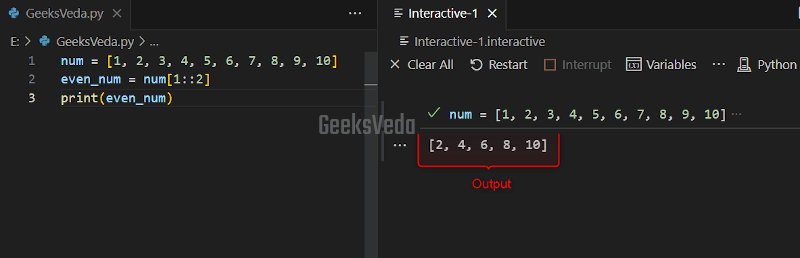
Using Slicing to Implement Pagination
Pagination in Python refers to the process of dividing a large dataset into smaller chunks for simple display, easier navigation, and retrieval.
You can consider slicing for implementing pagination in applications. For instance, in the provided example, the “dataset
” is sliced to retrieve particular items based on the “page_number
” and “page_size
“.
dataset = ["a", "b", "c", "d", "e", "f", "g"] page_size = 3 page_number = 2 start_index = (page_number - 1) * page_size end_index = start_index + page_size page_items = dataset[start_index:end_index] print(page_items)
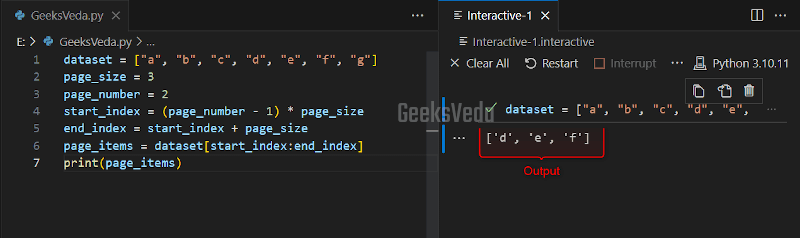
Using Slicing for Data Transformation
Data transformation is another use case of slicing in Python. It assists in manipulating and transforming data by retrieving subsets and reordering elements.
For instance, we have a string named “msg
” which will be sliced and reordered for creating a new string with the reversed words and an added comma.
msg = "Hello, User!" reordered_msg = msg[7:] + msg[5:7] + msg[:5] print(reordered_msg)
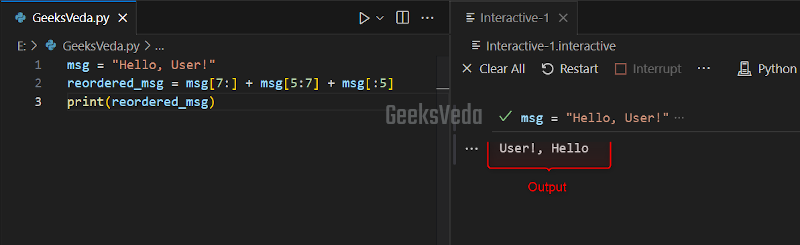
Best Practices of Using Slicing in Python
Look at the enlisted best practices for using slicing in Python.
- Use clear variable names.
- Check indices to avoid errors.
- Understand the inclusive and exclusive indices.
- Avoid excessive nested slicing.
- Utilize negative steps for reverse slicing.
- Document complex slicing.
- Create new objects instead of modifying the original ones.
- Combine slicing with other features.
- Learn more about the advanced techniques related to slicing.
That’s all from this informative guide related to slicing in Python.
Conclusion
It is essential to master Python slicing for effective data management and extraction. Slicing offers simple substring extraction, particular element retrieval, and data modification. Moreover, you can also optimize your code and fully utilize Python’s slicing features by keeping in mind the best practices and learning advanced slicing techniques.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!