Have you ever wondered how to optimize memory usage and simplify your code while making the most of the flexibility of custom iterable objects? Now, look no further!
In today’s guide, we will explore the working of the “yield
” keyword in Python through practical examples. Moreover, we will also discuss its relationship with generator functions, iterators, and iterable and enlist the advantages and disadvantages of using it in your program.
What is the “yield” Keyword in Python
The “yield
” keyword is utilized in the context of generator functions for creating iterators. It enables a function to generate or produce a sequence of values that are allowed for further iteration one at a time.
When a generator function finds out the “yield
” keyword, it pauses the execution and yields a value to its respective caller. Moreover, the function state will be saved, and it can resume when the next value is required.
Benefits of Using “yield” Keyword in Python
Look at the provided benefits of utilizing the yield keyword.
- Improved Performance – Avoids unnecessary computations which lead to faster code execution.
- Efficient Memory Usage – The yield keyword permits on-demand value generation which minimizes the memory requirements.
- Simplified Code Structure – Permits writing iterative algorithms in a sequence.
- Infinite Sequence Generation – Helps generate infinite sequences without storing all values.
- Resource management – Enables efficient handling of resources.
- Integration With Iterators – Offers support for the creation of custom iterable objects.
- Framework Compatibility – Widely utilized in several Python libraries and frameworks.
Difference Between “yield” and “return” Statements
Now, check out the given table to understand the differences between “yield
” and “return
” statements.
yield | return | |
Purpose | Suspends function execution, enabling multiple values to generate over multiple calls. | Terminates the function execution and outputs a single value. |
Usage | Used in generator functions. | Used in regular functions. |
Generator object | Returns a generator object. | Outputs a single value. |
Multiple values | Yield multiple values. | Returns a single value. |
Stateful | Maintains the state of the function. | Does not maintain the function state. |
Control flow | Permits iterative and lazy evaluation of values. | Run linearly, control flows toward the caller. |
Resumption | Can resume execution. | Execution does not resume. |
Now, let’s dig deeper into the yield keyword usage in Python.
Brief on Generator Functions in Python
A special kind of function that utilizes the “yield
” keyword for creating an iterator is called a Generator function. It is defined as a regular function, however, it utilizes “yield
” instead of “return
” to emit values. More specifically, it outputs a generator object that can be iterated further when invoked.
Generator Function With the “yield” Keyword in Python
To use the “yield
” keyword, first, define a generator function with the “def
” keyword followed by the function name. Then, within the body of the function, specify the “yield
” keyword to yield a value.
In this example, we will generate Even numbers with “yield
” Keyword. We have defined a generator function named “even_num()
“. This function will produce an infinite sequence of even numbers. This function utilized a “while
” loop for producing numbers continuously.
Each number is yielded with the help of the “yield
” keyword, including the next even number in the sequence.
def even_num(): num = 0 while True: yield num num += 2 new_even = even_num() for i in range(10): print(next(new_even))
By invoking the created generator function and using a “for
” loop with “range(10)
“, the first ten even numbers will be printed on the console, one at a time.
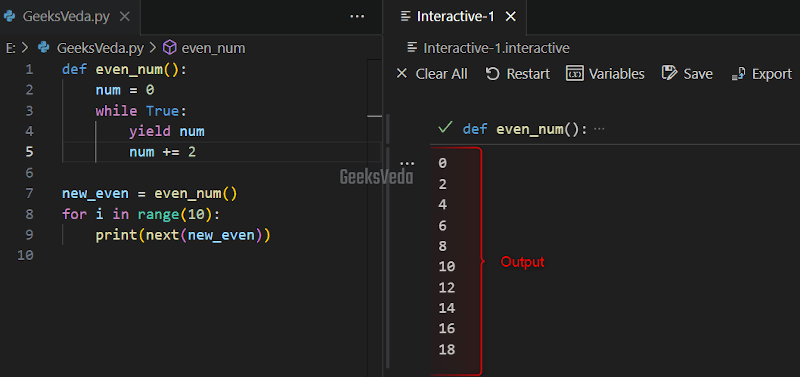
That’s how you can generate values on-the-fly without storing all of them in memory.
Generate a Fibonacci Sequence With “yield” Keyword
Here, in the provided code, we have defined a function named “fibonacci()
” that generates an infinite sequence of Fibonacci numbers. Within the function body, we have initialized two variables “x
” and “y
” as 0 and 1, respectively.
With the help of the while loop, the function enters an infinite loop. In each iteration, the current value of “x
” is yielded by utilizing the yield
keyword. This also represents the next Fibonacci number. Next, both of these variables are updated for calculating the next Fibonacci number.
The generator object “new_gen
” is created by invoking the generator function. Lastly, by using the “for
” loop with the range(10)
, the first ten Fibonacci numbers will be shown on the console.
def fibonacci(): x, y = 0, 1 while True: yield x x, y = y, x + y new_gen = fibonacci() for i in range(10): print(next(new_gen))
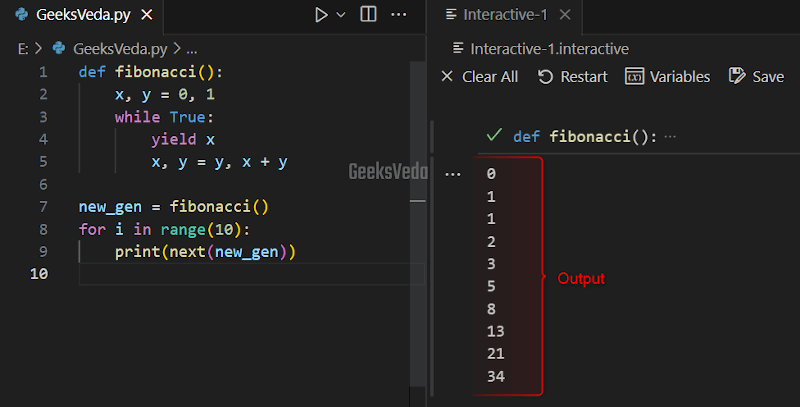
What are Iterators and Iterables in Python
An iterator is an object in Python that applies the iterator protocol, which ultimately requires the existence of “iter()
” and “next()
” methods. It indicates a stream of values that can be iterated upon.
On the other hand, an iterable is an object that outputs an iterator when the “iter()
” is invoked on it.
Create Iterators with “yield” Keyword in Python
The creation of the iterators can be simplified with the yield
keyword. When this keyword is added within the function definition, it converts the function into an iterator automatically. Moreover, each yield statement offers the next value in the ongoing sequence.
It also saves the function states which enables it to resume or continue from the last yield whenever requested.
Relationship of “yield” Keyword and Iterable Protocol
The iterable protocol needs an object for the implementation of the “iter()
” method, which returns an iterator object. By utilizing the “yield
” keyword inside a generator function, you can automatically create an iterator that follows the iterable protocol.
Moreover, the iterator can be used in “for
” loops or with other iterable-related functions.
Using Custom Range Iterator With “yield” Keyword
In the provided program, we have defined a custom iterator named “Numbers
” that produces a range of numbers from the specified “start
” and “end
” value.
This class implements the “__iter__
” method that will handle the iterator object.
Within the __iter__
method, a while
loop is added that generates the numbers incrementally with the help of the “yield
” keyword. This loop continues its execution until the current number reaches the end value.
Moreover, each number is yielded to the function caller, and the while
loop continues until the specified condition is met.
class Numbers: def __init__(self, start, end): self.start = start self.end = end def __iter__(self): current = self.start while current < self.end: yield current current += 1 my_range = Numbers(1, 5) for num in my_range: print(num)
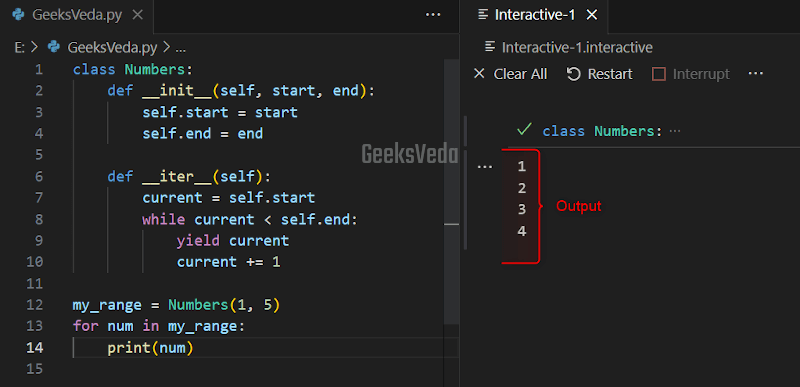
Using Custom List Iterable With “yield” Keyword
For this example, firstly, we will create a custom iterable named “Animals
” that represents a list having different animal names. Then, we will add a “add_items()
” method that can be utilized for adding items to the list.
Next comes the “__iter__
” method that uses a for loop for iterating over the list items and “yield
” each item to the caller. As a result, list items will be generated one by one.
class Animals: def __init__(self): self.items = [] def add_item(self, item): self.items.append(item) def __iter__(self): for item in self.items: yield item my_list = Animals() my_list.add_item("Cat") my_list.add_item("Lamb") my_list.add_item("Rabbit") my_list.add_item("Elephant") for animal in my_list: print(animal)
By using this custom iterable, we have added items to the list and iterated them as follows.
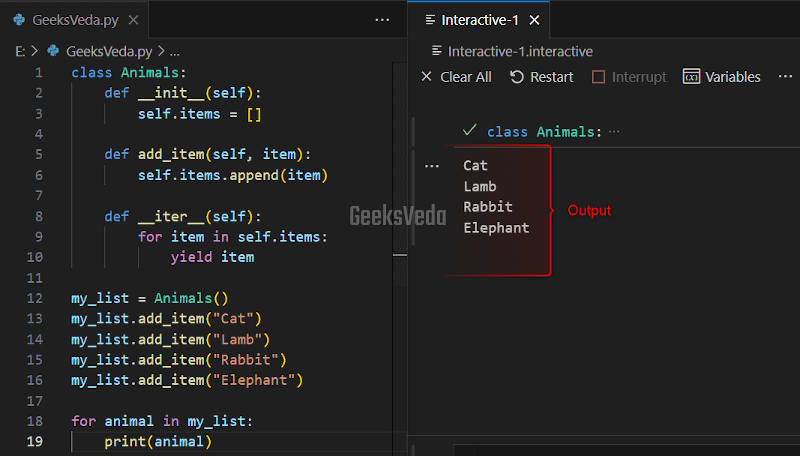
Advantages and Disadvantages of Using “yield” Keyword
Have a look at the given lists comprising the advantages and disadvantages of the yield keyword in Python.
Advantages –
- The “
yield
” keyword generates values on-demand which ultimately reduces memory usage. - It offers a concise way of writing iterative algorithms.
- It facilitates asynchronous operators with async generators.
- It permits the creation of generator functions for custom iterables.
- It enables the generation of infinite sequences without storing all values.
Disadvantages –
- This keyword can be only utilized with generator functions.
- It requires careful handling of the function state.
- The generator function does not offer support for accessing the values randomly.
- Generator functions are inherently single-threaded.
That’s all from today’s informative guide related to the usage of the “yield” keyword in Python.
Conclusion
The “yield
” keyword in Python offers memory-efficient value generation and enables the creation of custom iterable objects. It simplifies code structure for iterative algorithms and provides support for asynchronous programming.
Being limited to generator functions and lacking random access, “yield
” still remains a robust tool for enhancing code flexibility and improving performance.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!