In the previous blogs of this Python flow control series, we discussed the if…else statements and the “for loop“. Now, step up and explore the functionality of the while loop in Python.
Python while loop
is a robust construct that permits repetitive code execution based on the added condition. It provides flexibility when the iteration is not known.
So, in this article, we will check out the syntax, execution flow, techniques, patterns, and nesting of the while loops.
Moreover, we will compare the while loop with the for loop in terms of its functionality.
What is while loop in Python
In Python, while loop
permits you to execute a code block repeatedly until the particular condition remains true. It offers an efficient way for creating loops that continues until a certain condition is no longer satisfied. Moreover, the loop body runs until the condition is met.
How is while loop Used in Python
While programming in Python, you can utilize a while loop
for repeating a code block until the particular condition has been evaluated as true.
These loops offer flexibility in handling specific or dynamic situations where you have no idea about the number of iterations. More specifically, a while loop can be used for iterative computations, user input validation, and interaction with data structures.
In order to use Python while loop, follow this syntax.
while condition: # code block
Here, the added “condition
” or “test expression
” has been evaluated before each iteration. If it is evaluated as “true
“, the defined code block will run.
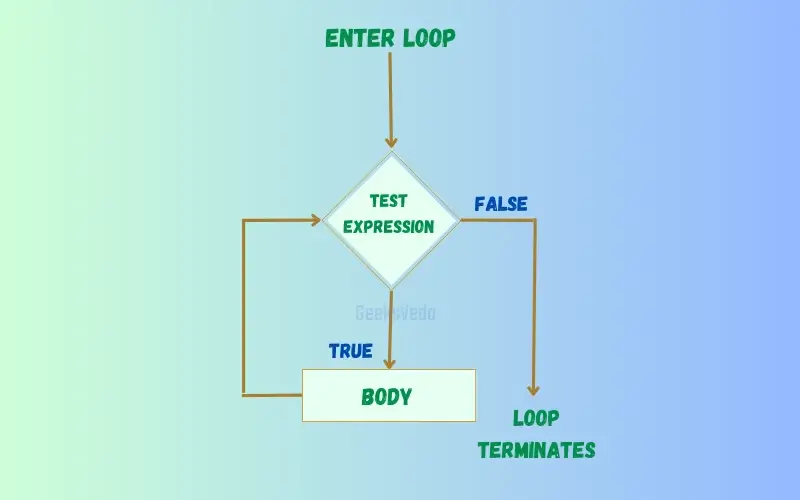
Let’s observe some practical examples of using the while loop.
1. Create a Countdown Using while loop
In this example, we have added the while loop to count down from 10 to 1. This loop continues as long as the value of the “counter
” variable is greater than 0.
counter = 10 while counter > 0: print(counter) counter -= 1
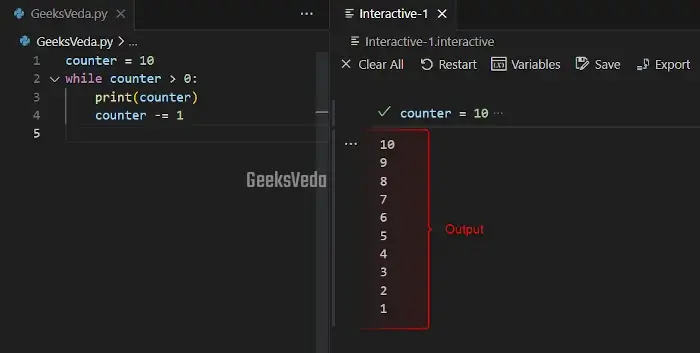
2. How to Validate User Input Using while loop
Now, we will demonstrate the usage of the while loop for validating the user input. The added while loop asks the user to input the enter or type a password and it continues until the correct password has been entered.
password = "" while password != "geeksvedauser": password = input("Please, Enter the password: ") if password == "geeksvedauser": print("Access has been granted!") else: print("You have entered an Invalid password! Try again.")
In our case, we will type out “geeksvedauser
” which is the correct password that terminates the while loop and prints the added message.
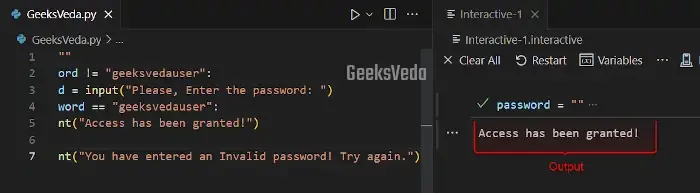
Control Flow and Execution in Python while loop
Now, let’s talk about the control flow and execution of the while loop in detail. The execution flow within the while loop begins by validating the condition.
If the condition is met, the defined code block runs. After that, the specified condition is checked again. If it is still true, two discussed operational flows are repeated.
Additionally, it continues until the condition becomes false. At this point, the while loop terminates and the program continues to run the rest of the code.
Python Techniques Using while loop
In this section, we will discover common techniques and patterns utilized in the while loop in Python, such as using loop counters, sentinel values, increment, and decrement variables. These patterns have the ability to improve the ability to use the while loop.
1. Create Loop Until the Condition is Met
In this example, we have defined the while loop for iterating over the “colors
” list until the condition “index < len(colors)
” is met.
This loop starts with the index value as 0 and continues until the value of the index variable is less than the length of the “colors
” list.
After each iteration, the value of the index variable will be incremented by 1 and move forward toward the next element. This pattern enables looping through the defined list until all list elements have been iterated.
After each iteration, the value of the index variable will be incremented by 1 and move forward toward the next element. This pattern enables looping through the defined list until all list elements have been iterated.
colors = ["pink", "blue", "orange"] index = 0 while index < len(colors): print(colors[index]) index += 1
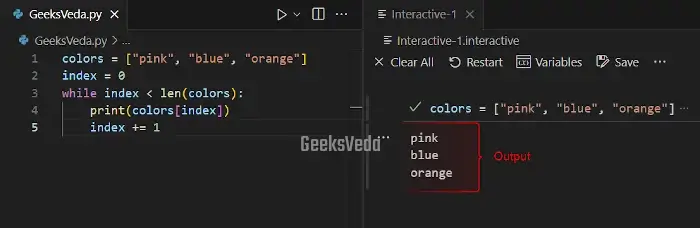
2. Counter with while loop
Here, we have defined a counter variable named “count” that will be utilized for keeping the record of the iteration number. The added while loop will continue until the counter reaches 6.
count = 0 while count < 6: print("Iteration no:", count) count += 1
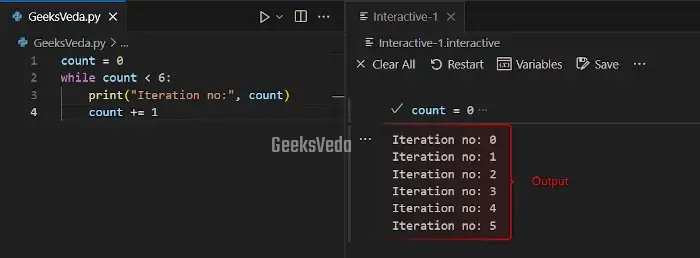
3. Sentinel Value With while loop
In this example, the while loop asks the user to enter numbers until the sentinel value, which is “-1
” has been entered. This loop will end when the sentinel value has been encountered.
number = 0 while number != -1: number = int(input("Enter a number (-1 to exit): ")) print("You have entered:", number)
Firstly, we have entered “2
“.
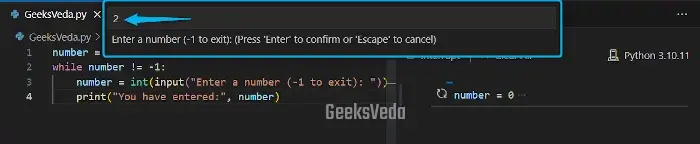
In the output section, note that the entered numbers have been displayed and the loop continues to execute till we have input “-1
“. As a result, the while loop will be terminated.
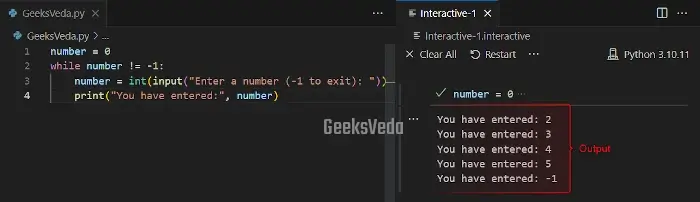
4. Increment Variable in while loop
This example shows the method of using an increment variable within a while loop. This loop will display the odd numbers from one to 10 by incrementing the value of the “start
” variable by 2 in each iteration.
start = 1 while start <= 10: print(start) start += 2
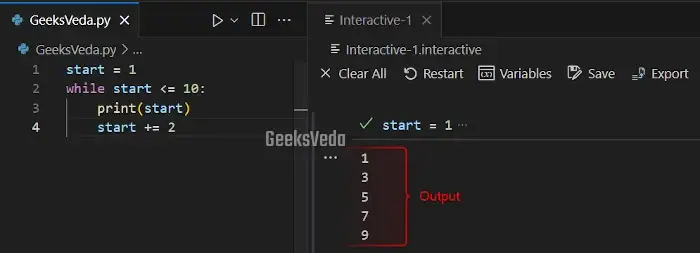
5. Decrement Variable in while loop
Now, we have changed the value of the “start
” variable to 10 and the while loop condition to “start >= 0
“. This loop will print the even numbers from 10 to 0 by decrementing the “start” variable by 2 in each iteration.
start = 10 while start >= 0: print(start) start -= 2
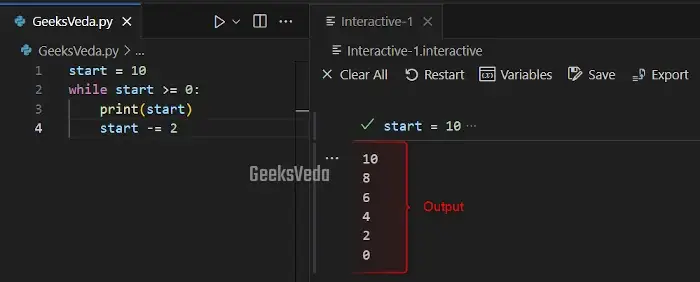
Nested while loops in Python
Now, let’s demonstrate how to use nested while loops in Python with the help of examples.
1. Using Simple Nested while loop
For instance, we have utilized the nested while loops where the inner loop will run for each outer loop iteration. Note that the “row
” variable controls the outer loop iterations while “col
” performs the same functionality for the inner while loop.
Moreover, the value of the “row
” and “col
” variables will be printed for all combinations.
row = 1 while row <= 5: col = 1 while col <= 2: print(row, col) col += 1 row += 1
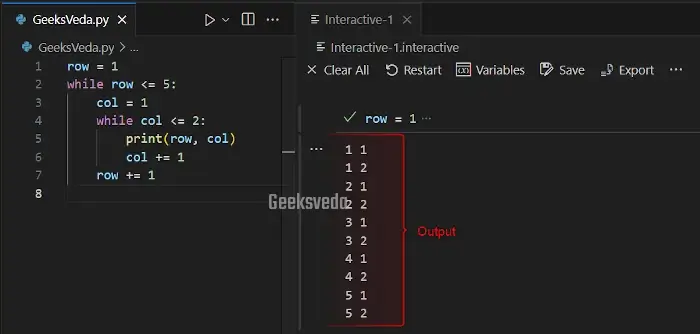
2. Create Pattern Using Nested while loop
Here, in this case, we have utilized the while loops for creating a pattern of asterisks “*
“. Note that the number of asterisks in each row increases incrementally with the help of the “row
” and “col
” variables value.
size = 8 row = 1 while row <= size: col = 1 while col <= row: print("*", end="") col += 1 print() row += 1
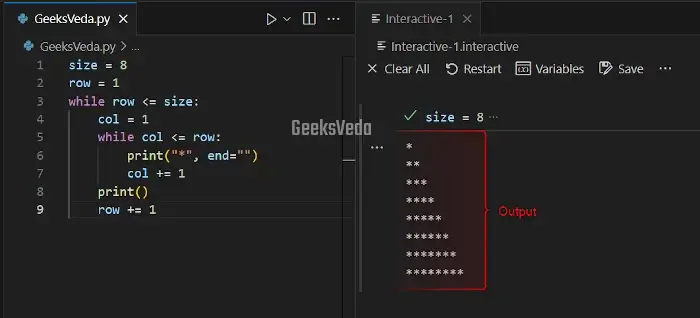
Python while loop VS for loop
Have a look at the provided table for comparing the functionalities of the while loop with for loop.
while loop | for loop | |
Use case | Unknown number of iterations. | Predictable or known number of iterations. |
Initialization | Explicit initialization. | No explicit initialization. |
Condition | Loop exits based on the defined condition. | Loop exists based on the defined iterable. |
Increment//Decrement | Manual Control. | Automatic iteration is required. |
Break | Needs explicit use of “break”. | Can utilize a “break” statement for terminating the loop. |
Nesting | Can be nested. | Can be nested. |
Common Use | Loops until the particular condition. | Iterating over ranges or sequences. |
Bonus Tip: Terminate an Infinite loop in Python
Sometimes, while programming in Python, infinite loops can occur because of logical errors on any other intentional design for particular scenarios where continuous is needed. Therefore, in order to effectively terminate the infinite loop the break and continue statements can be used.
Conclusion
Master the Python while loop for handling the program control efficiently. In today’s post, we have explored syntax, techniques, and nesting and also compared its functionality with for loop.
In the next part of this series, we will discuss Python break and continue statements. So, level up your programming skills and start iterating with confidence!
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!