So far, in this Python control flow series, we have discussed for loop
, while loop
, if…else statements
.
Now, it’s time for unlocking other secrets with the dynamic duo of “break
” and “continue
” statements.
In this blog, we will demonstrate what break and continue statements are in Python and their usage with for loop, while loop, if…else statements, and exception handling with practical examples.
Moreover, the differences between the functionalities of break and continue statements will be compared for your better understanding!
What is break Statement in Python
In Python, a “break
” is a control flow statement that is utilized to terminate a loop prematurely. When this statement is added within a loop, it immediately exits the loop and resumes the execution control at the next statement that is present outside of the loop.
The break statement is primarily used in scenarios where it is required to stop the loop based on a particular condition, search for a certain element, or perform a task until a condition is met.
More specifically, when the condition is satisfied, the break statement permits you to exit the loop and avoid unnecessary iterations.
How to Use break Statement in Python
For instance, in the provided program, the for loop iterates through the elements of the “numbers
” list.
num
” iterable becomes “44
“, the break statement will be triggered which terminates the loops.Resultantly, only the first three elements will be printed on the console.
numbers = [11, 22, 33, 44, 55] for num in numbers: if num == 44: break print(num)
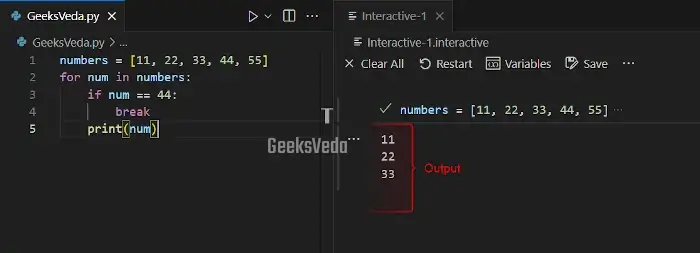
What is continue Statement in Python
The “continue
” is another control flow statement that can be utilized for skipping the rest of the current loop iteration and moving to the next iteration.
Additionally, when it is found within a loop, the remaining code part within that specific iteration is skipped, and the loop proceeds or move toward the next iteration.
This statement is mainly used when it is needed to exclude certain elements from processing or to skip specific iterations based on the defined criteria. It enables you to bypass particular code within the loop according to the defined conditions.
How to Use continue Statement in Python
In this example, the added “for loop
” iterates through the “numbers
” list. So, in this process, when an even number is encountered according to the given condition which is “(num % 2 == 0)” the continue statement will run.
As a result, the code within that iteration will be then skipped and the for loop will move to the next iteration.
numbers = [11, 22, 33, 44, 55] for num in numbers: if num % 2 == 0: continue print(num)
In this case, only the odd numbers will be displayed on the console.
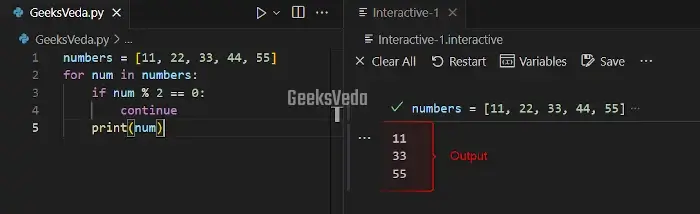
Difference Between “break” and “continue” in Python
Have a look at the given table to compare break and continue statements based on their functionalities.
break Statement | continue Statement | |
Purpose | Terminates the current or ongoing loop. | Skips the remaining code. |
Usage | Exits the loop completely. | Proceeds to the next iteration. |
Effect | Prevents further loop iterations. | Skips remaining code in the iteration. |
Control | Entirely changes the loop execution. | Continues with the next iteration. |
Condition | Utilized to meet a specific condition. | Utilized to skip specific iterations. |
Flow of Loop | Exits the loop prematurely. | Skips code within the iteration. |
Python break and continue Statement Examples
This section will demonstrate the advanced techniques which comprise break and continue statements. We will discuss how to utilize these statements with conditional statements, such as if…else, for loop, while loop, etc.
1. continue Statement with if…else
For instance, here, the for loop iterates over the given “numbers” list having ten elements.
continue
” statement is added in the if…else
statement to skip the even numbers. This permits only printing the odd numbers on the terminal.numbers = [11, 22, 33, 44, 55, 66, 77, 88, 99, 110] for num in numbers: if num % 2 == 0: continue print(num)
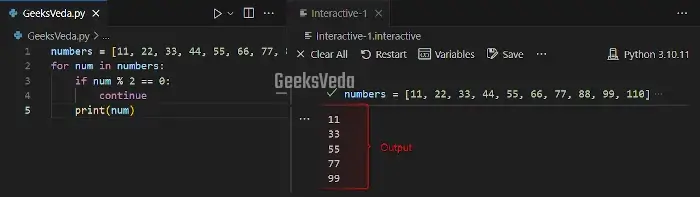
2. break Statement with for loop
This program iterates over the list of “colors
” and displays each color until it encounters a “blue
” element. Once the mentioned element is found, the break statement will run and the loop gets terminated prematurely.
colors = ['black', 'pink', 'yellow', 'blue', 'red'] for color in colors: if color == 'blue': break print(color)
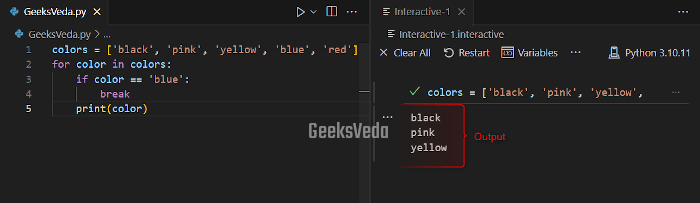
3. continue Statement with a while loop
Here, the added while loop increments the “num
” variable from one to 20. Moreover, the added “continue
” statement is utilized for skipping the even numbers and only showing the odd numbers on the console.
num = 0 while num < 20: num += 1 if num % 2 == 0: continue print(num)
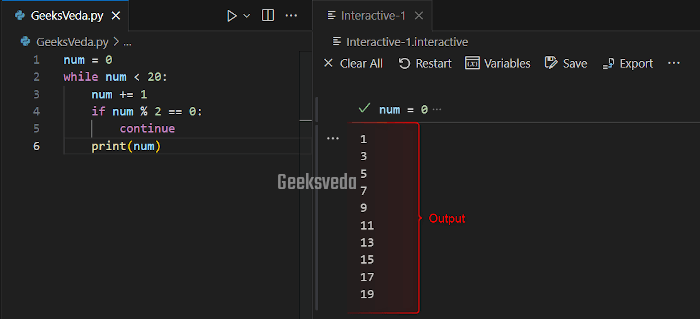
4. break Statement with a while loop
There is no built-in do-while loop in Python. However, the same functionality can be embedded by adding the break statement in the while statement.
For instance, in the given code, we have implemented the do-while loop functionality using the while True condition. The added loop displayed the “num
” variable value, which starts from 1 and gets doubled in each iteration.
This loop will terminate because of the break statement, when “num
” exceeds the “200
” value.
num = 1 while True: print(num) num *= 2 if num > 200: break
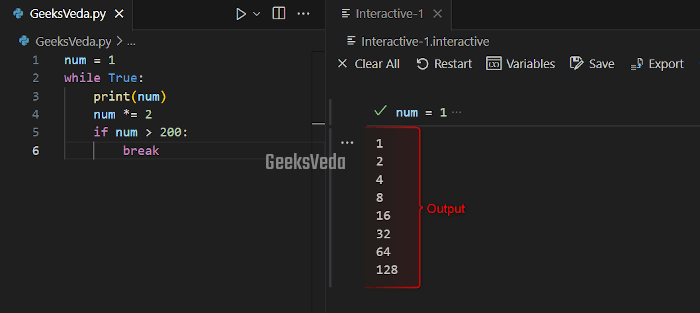
5. while loop with break and continue Statements
Now, we have an infinite while loop
that asks the user to type a number. In this scenario, if “q
” is entered, the “break
” statement will run and it terminates the loop.
But, if the entered number is even, the “continue
” statement will skip the remaining code in the current iteration and move to the next iteration.
According to the program logic, only the odd numbers will be displayed on the console which clears the concept of using break and continue for controlling the loop execution.
while True: user_input = input("Please enter a number (or 'q' to quit): ") if user_input == 'q': break number = int(user_input) if number % 2 == 0: continue print(f"Entered number {number} is odd.")
Let’s enter “35
” which is an odd number. As you can see, the print() function displayed the added message that the typed number is odd.
Now, we will enter “q
” to quit.
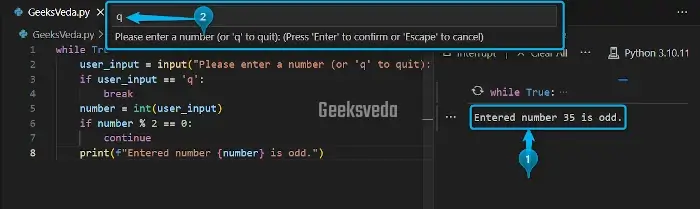
Now, the while loop
has been terminated.
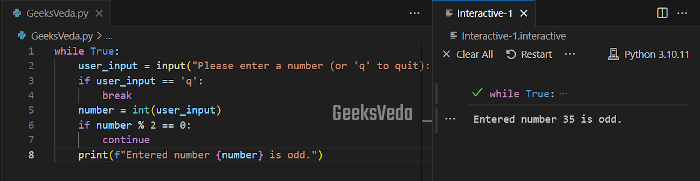
Handling Exception with break/continue Statements
The break
and continue
statements can be utilized for exception handling in Python. More specifically, when an exception has been encountered within a loop, the normal loop flow gets interrupted.
So, if a break statement has been found within a “try
” block, it terminates the loop without executing the remaining code block.
Likewise, if a continue statement is encountered within a try
block, it will skip the rest of the loop body and move to the next iteration.
For instance, here a for loop
has been defined. Note that when the loop variable equals “4
“, it will raise the “ValueError
“.
In case, if the exception occurs, the exceptions block will handle it and display the relevant message.
On the other hand, the else block will run if no exception occurs, signifying the successful completion of the loop. Lastly, the “finally
” block will always run no matter whether the exception occurs or not.
try: for i in range(6): if i == 4: raise ValueError("Invalid value has been encountered") print(i) except ValueError as e: print("Exception:", str(e)) else: print("Loop has been completed successfully") finally: print("Cleanup code (Done)")
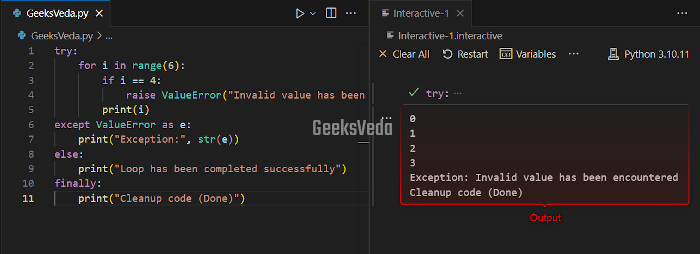
That’s all from this effective guide related to the usage of break and continue statements in Python.
Conclusion
In this blog, we discussed the use of break and continue statements for controlling the flow of the loop and terminating the loops prematurely, and skipping the iteration.
These crucial flow control statements can assist you in improving your programming skills. In the next part of this Python control flow series, we will discuss the Python pass statement.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!