While programming in Python, you may encounter use cases, where it is required to combine and process data from different sources. This can be done by iterating over two lists in parallel.
There are multiple methods to perform this operation, including using for loop, list comprehension, zip() function, enumerate() function, custom function, itertools module, map function and lambda function. All of these techniques will be discussed in today’s guide!
Understanding Lists in Python
A Python list represents a mutable sequence data type that comprises a collection of values of multiple data types, which includes floats, integers, strings, and other lists too.
More specifically, you can create lists by utilizing the square brackets [ ]
, and added elements can be separated using commas.
Properties of Python List
Here, we have enlisted some of the properties of the Python List.
- A Python list can comprise duplicate elements.
- The added elements can be accessed using slicing or indexing.
- You can add lists within other lists.
- Being a mutable data structure, you can add, update, or delete list elements.
Iterating Through Single List in Python
Iterating a list is a process of accessing each element of the list and performing some certain operation on it. One of the most common approaches for iterating over the list element is to use a for loop as follows.
list1 = [11, 33, 55, 77, 99] for element in list1: print(element)
corresponding element on the console using the print() function.
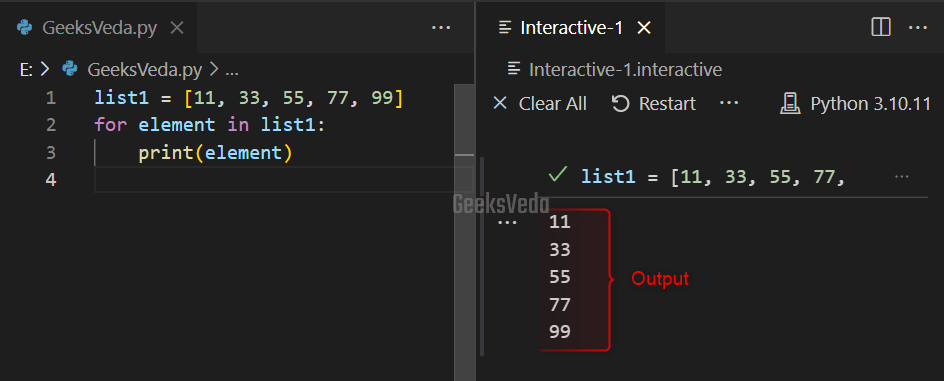
Iterating Through Two Lists in Parallel
Iterating through two lists in parallel means fetching each element of both of the specified lists at the same time and performing the required operation on them.
Python permits you to use multiple approaches for iterating over two lists in parallel, such as.
for loop
zip()
function- Custom function
enumerate()
function- List comprehension
- itertools module
- map and lambda function
1. Using for loop Method
You can utilize for loop for iterating over the indexes of both lists at the same time.
For instance, in the provided example, we first created two Python lists named list1
and list2
, one having integers and the other containing string values.
Then, we added a for loop
that iterates through the list1
and displays the respective element from the list on the basis of the current index of the first list.
list1 = [11, 33, 55, 77, 99] list2 = ['a', 'e', 'i', 'o', 'u'] for i in range(len(list1)): print(list1[i], list2[I])
As a result, the values of both lists will be shown side by side.
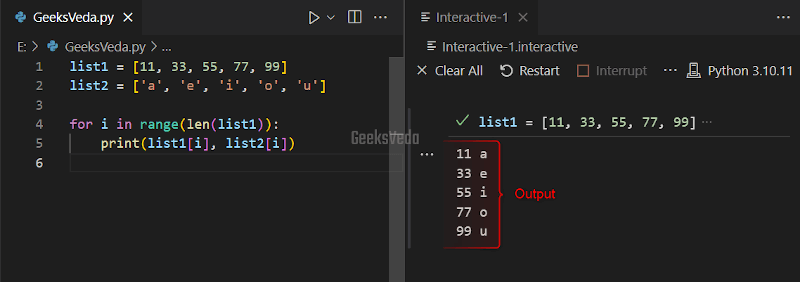
2. Using zip() Function
In Python, zip()
is a built-in function that enables you to iterate through multiple iterables which can be tuples or lists in parallel, and output a new iterator.
This iterator can further comprise the tuple of respective elements of each iterable.
Here, in the given code, we will utilize the zip()
function for combining both lists into a single iterable of tuples.
list1 = [11, 33, 55, 77, 99] list2 = ['a', 'e', 'i', 'o', 'u'] for a, b in zip(list1, list2): print(a, b)
The returned iterable is used for iterating through the corresponding elements of both list1
and list2
simultaneously.
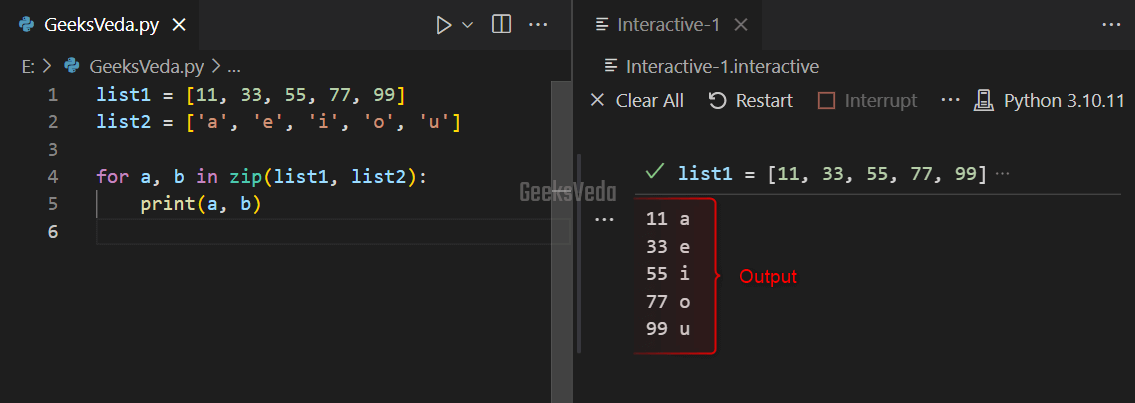
3. Using Custom Function
Another approach is to define a custom function that accepts two lists as parameters and iterates over them simultaneously using the for loop.
For instance, we will now define “custom_function()
” that accepts two lists l1 and l2 as parameters. This function further uses a for loop
for iterating over the l1 and l2 indices.
More specifically, the range()
function with the len()
method can assist in getting the list’s length.
Inside the for loop
, elements of both lists will be printed by utilizing the current index.
list1 = [11, 33, 55, 77, 99] list2 = ['a', 'e', 'i', 'o', 'u'] def custom_function(l1, l2): for i in range(len(l1)): print(l1[i], l2[i]) custom_function(list1, list2)
Lastly, the custom_function()
will be invoked by passing list1 and list2 as arguments.
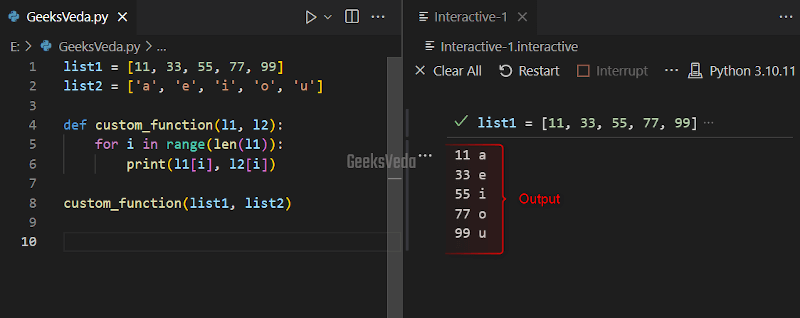
4. Using enumerate() Function
The built-in enumerate()
function enables you to iterate over other iterable objects or lists and also maintain track of the current index item that is under process.
This function accepts an iterable object as a parameter which can be a string, tuple, or list, and outputs a new object that produces a pair of values.
In the below program, firstly, we have two lists list1 and list2. Within the for loop
, the zip()
function is invoked for combining these lists into a single iterable, which is resultantly iterated using the enumerate()
function.
list1 = [11, 33, 55, 77, 99] list2 = ['a', 'e', 'i', 'o', 'u'] for i, (a, b) in enumerate(zip(list1, list2)): print(i, a, b)
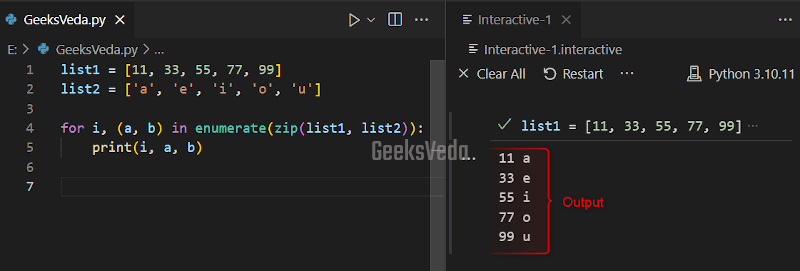
5. Using List Compression
List compression is considered a concise way of creating Python lists. It permits you to define a list using a single line of code by applying the given expression for each element of the iterable object or already created list.
This approach can be used for performing a specific operation of each element of both lists.
Now, we will utilize list compression for creating a new list named result
. The specified zip()
function combines list1 and list2 elements into pairs.
Then, the defined expression a + b
will be applied to each pair for creating a new element of the result list.
list1 = ['11', '33', '55', '77', '99'] list2 = ['a', 'e', 'i', 'o', 'u'] result = [a + b for a, b in zip(list1, list2)] print(result)
It can be observed that the corresponding elements of the list1
and list2
are iterated and then concatenated to create a new list of strings.
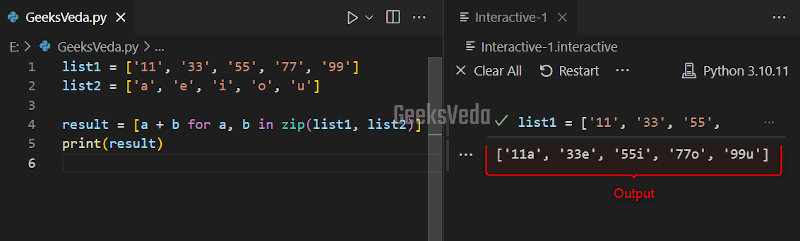
6. Using itertools Module
This Python module offers several functions that are used for iterating over multiple iterables. You can use itertools module when it is required for performing complex operations on multiple iterables.
For example, the zip_longest()
method of the itertools returns an iterator that aggregates from two or more iterables. More specifically, if any of the iterables is shorter than the other, missing values can be filled with a defined fill value.
import itertools list1 = [11, 33, 55, 77, 99] list2 = ['a', 'e', 'i', 'o', 'u'] result = itertools.zip_longest(list1, list2, fillvalue='NA') for a, b in result: print(a, b)
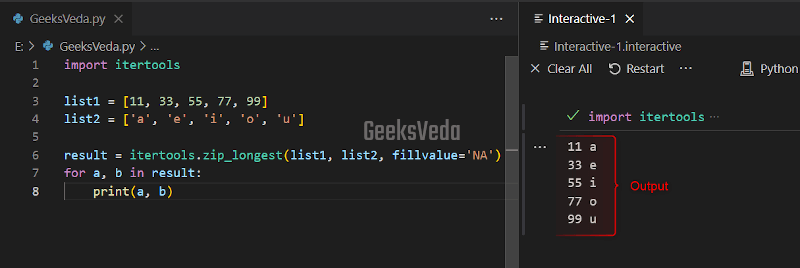
Another case would be to use the product()
method of the itertools module for getting the cartesian product of two or more iterables, which are list1
and list2
in this scenario.
import itertools list1 = [11, 33, 55, 77, 99] list2 = ['a', 'e', 'i', 'o', 'u'] result = itertools.product(list1, list2) for a, b in result: print(a, b)
This method will output a set of tuples that contains all the possible combinations of the elements from the list1
and list2
.
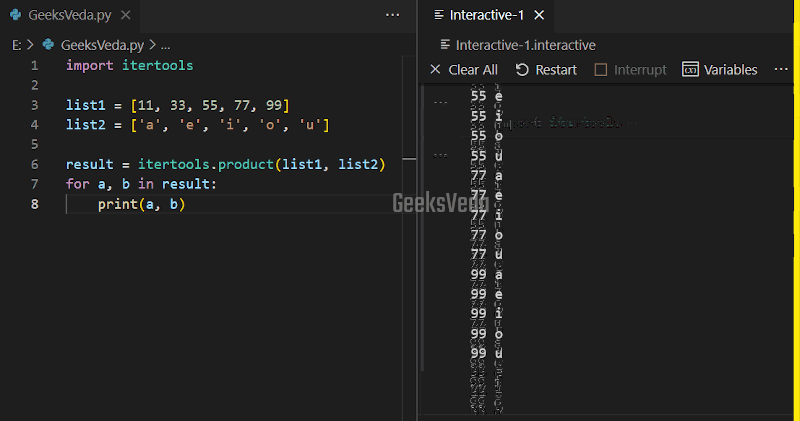
7. Using map() and lambda Function
You can also utilize Python map() function in combination with the lambda
function for iterating through two lists in parallel. To do so, first, pass the lambda
function to map along with list1 and list2.
The lambda
function takes two parameters, one from each list, and outputs a tuple of both elements. Then, the map()
function applied the specified lambda
function to each pair of the elements of both lists and resultantly returns an iterator of tuple.
list1 = [11, 33, 55, 77, 99] list2 = ['a', 'e', 'i', 'o', 'u'] result = map(lambda x, y: x * y, list1, list2) for value in result: print(value) list1 = [1, 3, 5, 7, 9] list2 = ['a', 'e', 'i', 'o', 'u'] result = map(lambda x, y: x * y, list1, list2) for value in result: print(value)
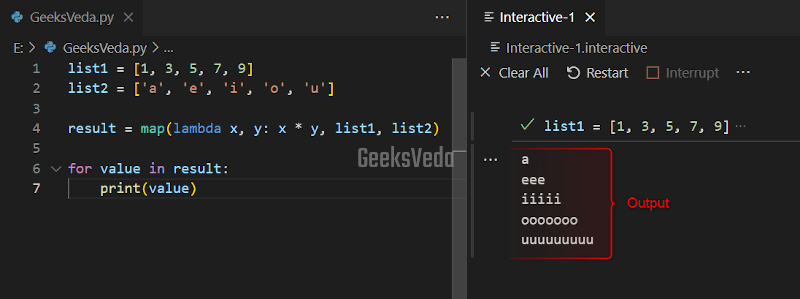
Comparison Table: Performance and Use Cases
Check out the provided comparison table which is based on the performance and use cases of the discussed approaches.
Approach | Performance | Use Cases |
For loop | Flexible, simple | Iterate over an iterable or list |
zip() function | Faster than for loop and custom function | Parallel iteration over multiple lists |
Custom function | Can be optimized for specific or certain tasks | Reusable code |
enumerate() | Somehow slower than for loop | Iterate over an iterable or list and keep track over the index |
List comprehension | Faster than custom function and for loop | Create new listers based on existing lists |
itertools module | Efficient for large datasets | Perform advanced iteration related tasks |
map and lambda function | Faster for extensive lists, but has overhead | Apply a function to each element of a list |
That’s all from this informative guide regarding iterating two lists in parallel.
Conclusion
Iterating through two lists in parallel is a common task in Python that can be performed using several approaches available to accomplish this.
You can use built-in Python functions like zip()
, enumerate()
, or list comprehension can result in more concise and readable code
On the other hand, custom functions or for loops offer more flexibility.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!