In today’s guide, we will talk about the Python map()
function and its usage in programming. You can explore how the map()
function simplifies element-wise operations on iterables, which ultimately permits you to apply a function on each item effortlessly.
So, improve your programming skills and optimize your code with the map()
function.
How Does the map() Function Work in Python
In Python, the “map()
” function is called for applying or implementing it as a particular function to each iterable element and outputs the resultant iterable. This function accepts two arguments, the “function
” that needs to be applied and the “iterable
” to process.
This function is invoked for each iterable element and the results are collected for each iterable element and stored in an iterator.
Syntax of map() Function
Have a look at the syntax for utilizing the map()
function.
map(function, iterable)
Here:
- The function can be a custom, built-in, or user-defined function.
- The iterable can be a list of numbers, a tuple, a dictionary, or a set.
How to Use map() With Built-in Functions in Python
The Python map()
function enables you to implement a built-in function to each item of an iterable and output an iterator of the results.
For instance, we have utilized the map()
function for converting each number defined in the “numbers
” list to a string with the help of the “str
” built-in function.
Resultantly, the iterator will be converted to a list and printed on the terminal.
numbers = [21, 42, 63, 94, 25] result = map(str, numbers) print(list(result))
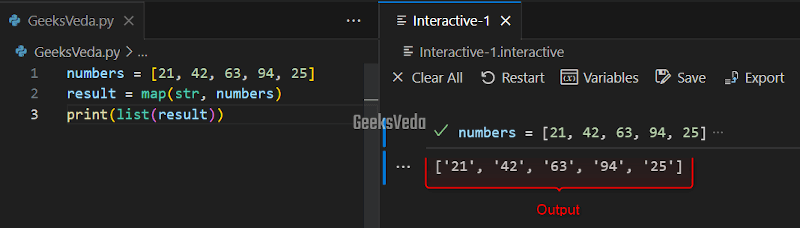
Python map() Function With Different Iterables
You can use the map() function with different iterable in Python, such as with.
- A
string
as an iterator - A
list
of numbers - A
tuple
- A
dictionary
- A
set
Using Python map() Function With String
In Python, a string is a sequence of characters that are enclosed in either single ''
or double ""
quotes. More specifically, they are Unicode-based, permitting the representation of characters from different character sets and languages.
The map()
function can be invoked with a string as an iterator for applying a function to each character of the string.
For instance, in the provided program, the map()
function is called with the “ord
” built-in function for converting each character of the string to its respective ASCII value.
As a result, the iterator will be converted to a list and shown on the terminal.
string = "Hi GeeksVeda User" result = map(ord, string) print(list(result))
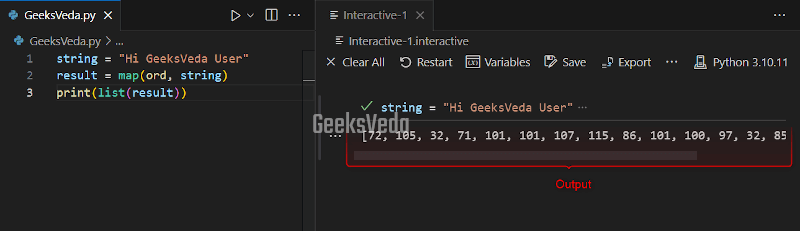
Using Python map() Function With List of Numbers
A Python list refers to an ordered collection of elements embedded in square brackets []
. They can comprise elements that belong to different data types and permit the addition of duplicated values.
Moreover, the map()
function can also be utilized with the specified list of numbers for performing different transformations or operations on each element.
Here, we have used the map()
function to double each number in the created “numbers
” list. The corresponding iterator will be converted to a list and shown on the console.
numbers = [3, 2, 1, 8, 9] result = map(lambda x: x * 2, numbers) print(list(result))
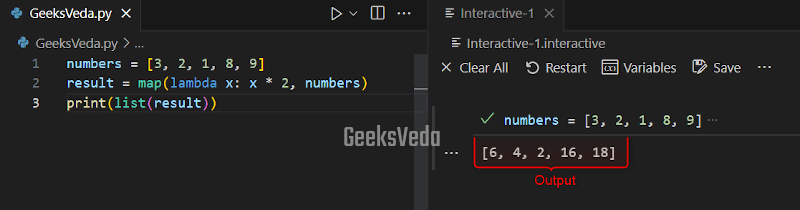
Using Python map() Function With Tuple
In Python, a tuple is an ordered list of collections of elements that are defined inside the parentheses. This structure comprises elements of different types and allows you to access individual elements with their respective index.
Now, in this example, we will use the map()
function with a lambda function for calculating the square of each of the elements of the newly created “tuple_nums
” tuple.
The resultant iterator will be then converted to a tuple and displayed.
tuple_nums = (13, 12, 43, 74, 25) result = map(lambda x: x ** 2, tuple_nums) print(tuple(result))
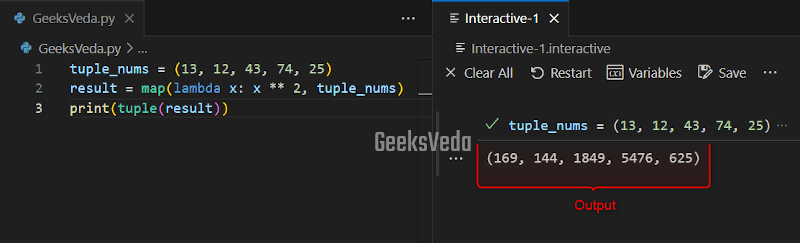
Using Python map() Function With Dictionary
A Python dictionary is an unordered collection of the relevant key-value pairs enclosed in curly braces {}
. It can be utilized for storing and fetching data based on unique keys.
Additionally, the map()
function can be called with a Dictionary for applying a function to its corresponding dictionary value.
Here, we have created a dictionary named “ages
” that contains the ages of three persons. Next, we have defined a function named “is_adult()
” that accepts an age and outputs “True
” in case the person’s age is greater than or equal to 18. Otherwise, “False
” will be returned as the boolean value.
The map()
function is used with “is_adult()
” as the function and the “ages.values()
” as the iterable for applying the function to each dictionary value.
The corresponding iterator will be then converted to a list with the “list()
” function and displayed on the console.
ages = {'Alexa': 15, 'Sharqa': 25, 'Kenny': 35} def is_adult(age): return age >= 18 adults = map(is_adult, ages.values()) print(list(adults))
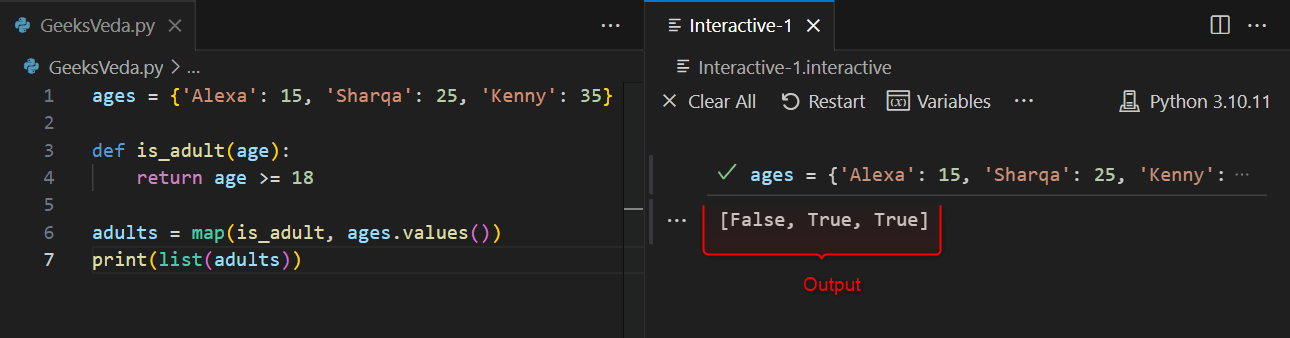
Using Python map() Function With Set
A Python set is an unordered collection of unique elements that are enclosed in the curly braces {}
. It can be utilized for storing a collection of distinct items and deletes the duplicate values automatically.
Now, let’s demonstrate the process of applying a function to each element of the set with the map()
function. For this, we have invoked the map()
function with a lambda
function to double each element value in the “my_set
“.
Then, the resultant iterator will be converted to set and shown on the console.
my_set = {11, 22, 33, 44, 55} result = map(lambda x: x * 2, my_set) print(set(result))
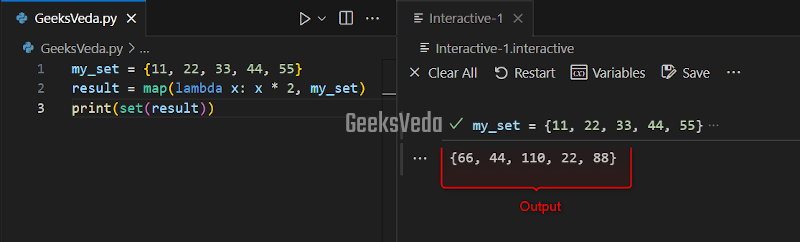
How to Use map() With Lambda Function in Python
Lambda
or anonymous function for defining small and single-use functions which do not have a particular name. These functions offer a convenient way for writing and using functions on the fly.
You can also use map()
with lambda function for performing operations on an iterable. For instance, here the lambda function for double each number in the “numbers
” list. The resultant iterator will be converted to a list and printed.
numbers = [11, 22,33, 44, 55] result = map(lambda x: x * 2, numbers) print(list(result))
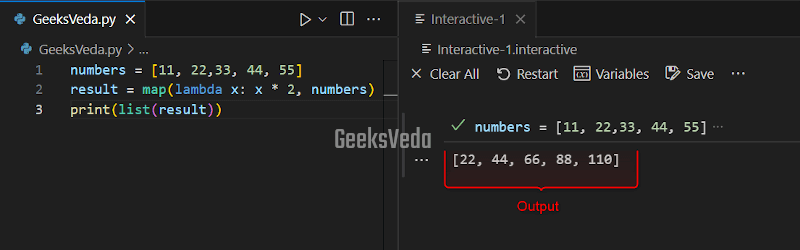
How to Use map() With User-Defined Function in Python
As a Python programmer, you can create a user-defined function for performing a particular operation or task. These functions assist in improving readability, organizing code, and prompting code reuse. More specifically, the map()
function can be used with such user-defined functions.
Let’s utilize the map()
function with our user-defined “square()
” function, which will square each number in the “numbers
” list. Then, the corresponding iterator will be converted to a list and printed out.
def square(x): return x ** 2 numbers = [3, 2, 7, 8, 1] result = map(square, numbers) print(list(result))
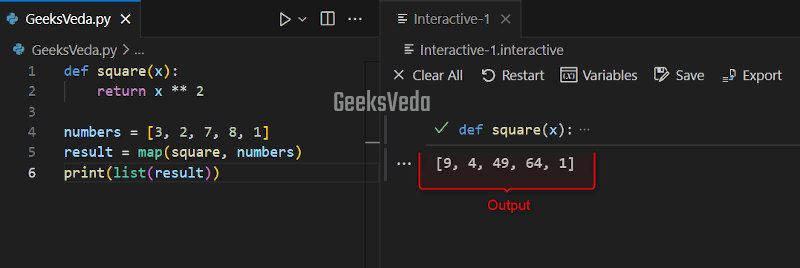
How to Use Multiple Iterators Inside map() Function in Python
Multiple iterators refer to the process of iterating over multiple iterables at the same time. They permit you to perform any operation on the respective elements from the multiple collections or sequences in a synchronized manner, which ultimately enables you to iterate them parallel and manipulate data.
Moreover, the map()
function can be utilized with multiple iterables as arguments for applying a function that accepts multiple arguments to each element of the iterables.
Here, in this scenario, the map()
function is used with a lambda function that accepts two arguments for adding the respective elements from the “numbers
” and “squares
” lists. Lastly, the resultant iterator will be converted to a list and displayed on the console.
numbers = [3, 6, 3, 8, 5] squares = [1, 2, 9, 10, 15] result = map(lambda x, y: x + y, numbers, squares) print(list(result))
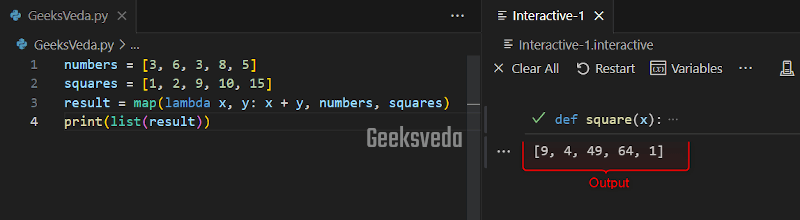
That’s all from this informative guide related to the Python map()
function.
Conclusion
Python map()
function is a robust tool utilized for transforming data in an iterable with the defined function. It permits efficient and concise code by applying the defined function to each element of the iterable.
It is versatile enough to work with lambda, built-in, or custom functions, multiple iterators, and iterable. So, use it as per your preferences.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!