In the previous part of this file handling series, we demonstrated the approaches for reading files in Python. Now, in this guide, let’s discuss the fundamentals of file writing in Python.
Writing data to file is an essential skill for the management of information. Today, we will explore several techniques for writing to files and cover methods like the “write()
“, “writelines()
“, and “write_text()
” methods for writing or appending data and writing multiple lines to a file accordingly.
How to Open a File in Write Mode in Python
In order to open a file in write mode, utilize the “open()
” function with the “w
” parameter that represents the write mode. Follow the provided syntax to do so.
file_object = open(file_path, 'w')
Here:
- “
file_path
” represents the file path that you want to open in the write mode. - “
w
” represents the write mode which signifies that the file should be opened in the write mode.
How to Write a File in Python
After opening the mentioned file, you can perform write operations on it by using any of the enlisted methods.
write()
Methodwritelines()
Methodwrite_text()
Method
Let’s check out each of the given methods individually!
GeeksVeda.txt
” file.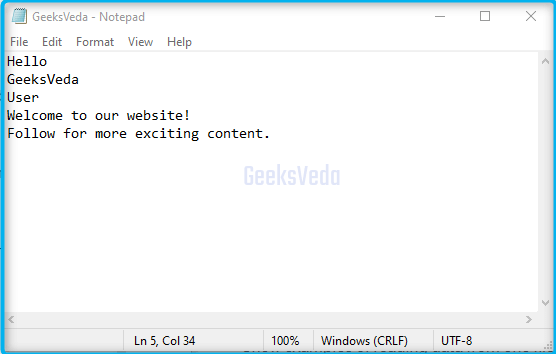
Using Python “write()” Method
Python “write()
” method can be utilized for writing data to the given file. It takes a string as a parameter that comprises the data that needs to be written.
More specifically, this method overwrites the existing file content with the new one. You can call this method for writing configuration files, log files, or generating reports.
For instance, we have defined “GeeksVeda.txt
” as the file path as it is present in the same directory. Then, we opened the file in the write mode with the “w
” parameter.
Next, the “write()
” method has been invoked and the string is passed that needs to be written. Lastly, the file is closed with the “close()
” method for ensuring the proper handling and release of the resources.
file_path = 'GeeksVeda.txt' file_object = open(file_path, 'w') file_object.write("write() method is used") file_object.close()
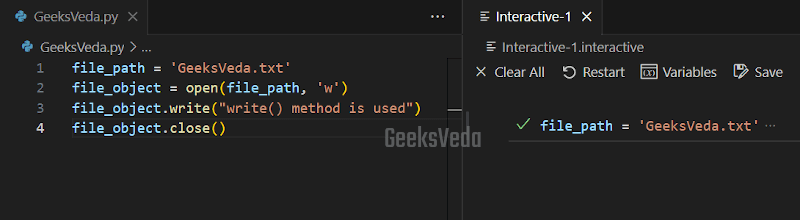
It can be observed that the added string has been written in the “GeeksVeda.txt
” file and the existing content truncated.
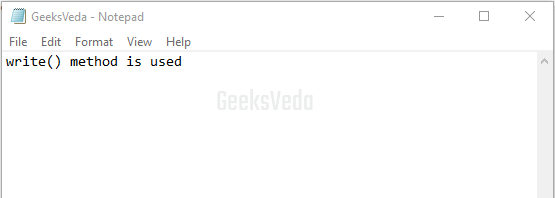
Append Text to an Existing File in Python
You can utilize the “a
” or append mode in the open()
function for appending data to an existing file. This mode permits adding new content at the end of the mentioned file without overwriting the existing content. Moreover, appending data is useful in the case of writing new information at the end of an existing file, such as for updating a data file or writing logs.
Now, in the same example, we have only changed the file mode parameter of the open()
function to “a
“. Then, the write()
method is called with the string that needs to be added at the end of the “GeeksVeda.txt
” file.
file_path = 'GeeksVeda.txt' file_object = open(file_path, 'a') file_object.write("New content appended!") file_object.close()
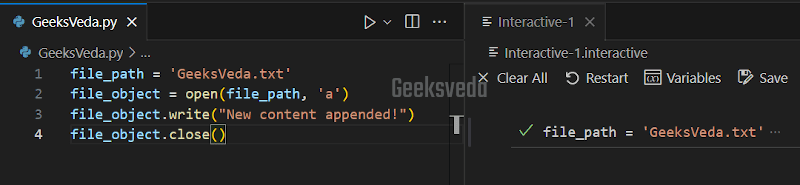
In this scenario, the specified data has been added at the end of the file.
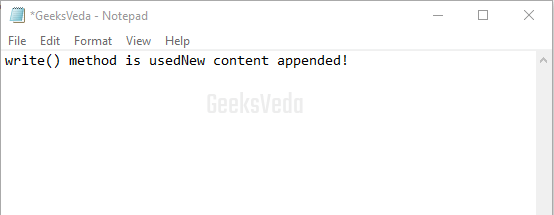
Using Python “writelines()” Method
writelines()
method is utilized for writing multiple lines of data/text to a file. It accepts a list of strings as input, where each string represents a line of data. Additionally, it does not add the newline character by default, so you have to include them explicitly.
Now, we have created a list of lines in the “lines
” variable. Then, the specified file is opened in the write mode with the open()
statement. Within the “with
” block, a generator expression is added for iterating over each line of the lines list and writing it to the “GeeksVeda.txt
” with the help of the “writelines()
” method.
The newline character “\n
” is added at the end of each line for the line separation. In the end, the file will be automatically closed when the “with
” block is exited.
file_path = 'GeeksVeda.txt' lines = ["New Line 1", "New Line 2", "New Line 3"] with open(file_path, 'w') as file_object: file_object.writelines(line + '\n' for line in lines)
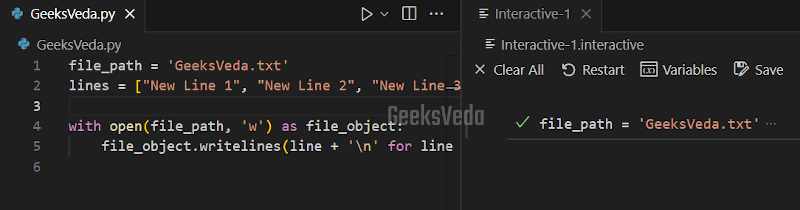
As you can see, multiple lines have been written in the “GeeksVeda.txt
” file.
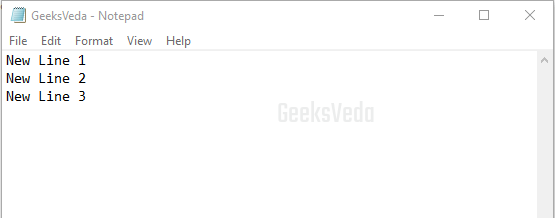
Using Python “write_text()” Method
Want to get rid of the hassle of handling the file opening and closing while writing to a file? Use the “write_text()
” method. It is a convenient method that is offered by the “pathlib
” module for writing text files.
This method accepts a string as input and writes to the given file. As stated earlier, it automatically handles the file opening and closing operations.
To utilize the “write_text()
” method, firstly import the “Path
” class from the “pathlib
” module. Then, create an object of the class named file_path having the “GeeksVeda.txt
” as the file path. Use this object to invoke the “write_text()
” method and pass the string that needs to be written in the file.
from pathlib import Path file_path = Path('GeeksVeda.txt') file_path.write_text("write_text() is used")
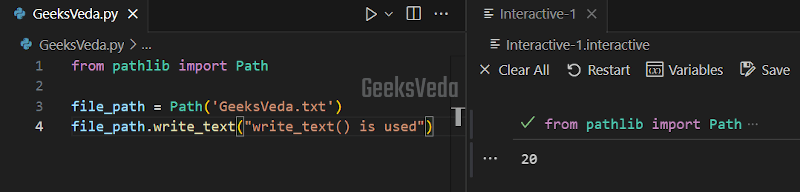
The given image signifies that the added text has been written in the “GeeksVeda
” file.
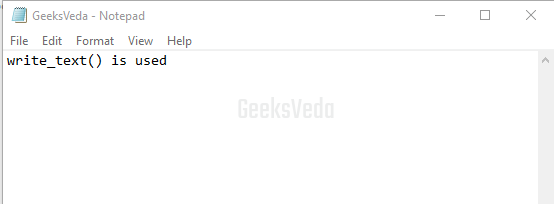
Python File Writing Errors and Their Fixes
In the upcoming sub-section, we will check out some of the common errors related to writing files in Python and their fixes.
PermissionError in Python
This error indicates that you don’t have the required permission for writing to the mentioned file or when the file is set as read-only. To fix it, ensure that you have the required write permission for the directory or file.
try: file_object = open('GeeksVeda.txt', 'w') # Perform file writing operations here except PermissionError as e: print("Permission denied:", str(e))
DiskFullError in Python
Encountering this error means that no more space has been left on the device where it is needed to write the file. To resolve this error, free up disk space by deleting unnecessary files or selecting a different location with sufficient disk space.
try: file_object = open('GeeksVeda.txt', 'w') # Perform file writing operations here except DiskFullError as e: print("No space left on device:", str(e))
IOError in Python
The IOError in Python occurs when there are problems related to input/output operations with the file. This can happen because of multiple reasons such as incorrect file paths, files not existing, or issues with the file system. To fix it, make sure that the file system is functioning properly, check the file path, and verify if the file exists.
try: file_object = open('GeeksVeda.txt', 'w') # Perform file writing operations here except DiskFullError as e: print("No space left on device:", str(e))
IsADirectoryError in Python
This error signifies that you have specified a directory instead of a file. Note that the open()
function does not allow opening a directory in write mode.
Therefore, to resolve this error, validate the file path and make sure it refers to a file rather than a directory.
TypeError in Python
While writing data to a file, the TypeError can occur if the type gets mismatched. For instance, in the given code, the “data
” variable is an integer, and it is required to be converted to a string before performing the concatenation operation. To fix this error, we have to convert the data to a string using str(data), before writing it to the “GeeksVeda.txt
” file.
try: file_object = open('GeeksVeda.txt', 'w') data = 123 file_object.write("Data: " + str(data)) # Convert data to a string before concatenation except TypeError as e: print("Type error:", str(e))
Best Practices for Writing to a File in Python
Here, we have given some of the best practices for writing to a file in Python
- Utilize the “
with
” statement for handling the file opening and closing. - Select the suitable mode which can be either “
w
” write mode, or append mode “a
“, as per your program requirements. - Handle errors beforehand with exception handling.
- Convert non-string data types to strings before writing.
- Always close the file to release system resources.
That’s all essential information regarding writing to a file in Python.
Conclusion
Mastering the art of file writing is crucial for handling data manipulation and storage in Python. Therefore, by understanding the several methods and best practices, you can effectively write data to files, optimize your code and handle errors for performing the file operations efficiently.
This was the last guide of our ongoing file handling series in Python, in which we discussed opening files, and reading files, and also checked out various approaches for writing to a file in Python.
You can also check out our dedicated Python Tutorial series!