In the last article of this Python file handling series, we discussed how to open a file in Python. Now, in this guide, you will check out the method of reading a file in order to extract data from it.
To do so, you can utilize different methods like “read()
“, “readline()
”, or “readlines()
“.
By understanding the working of these approaches, you can efficiently retrieve and process the file content. So, let’s dig more about file reading in Python and unleash the possibilities for manipulating data.
What is File Reading in Python
The process of accessing and fetching data from a file is known as file reading. This operation enables you to retrieve the file content, which can be binary data, text, or any other type of information which is stored in the file.
Python offers multiple methods or functions that permit you to read the entire file, read line by line, or read all lines to a list, as per your preferences.
More specifically, file reading is a crucial operation in Python that allows you to work with the data stored in the defined file and perform different operations on it.
How to Read From a File in Python
In order to read from a file in Python, open the file by specifying “r
” as read mode in the “open()
” function and then utilize any of the following methods.
- Using
read()
Method - Using
readline()
Method - Using
readlines()
Method
Now, let’s check out each of these methods practically!
GeeksVeda.txt
” file in the upcoming sections.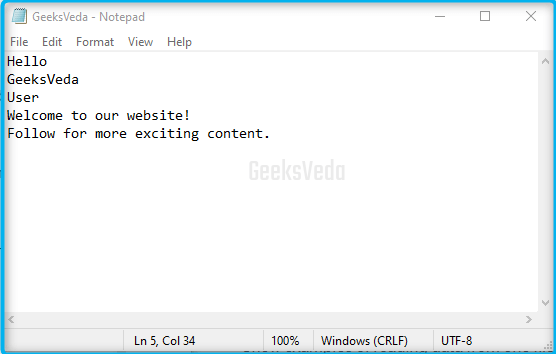
1. Using Python read() Method
The Python “read()
” method is utilized for reading the entire file content. This method outputs the content as a single string to the console. Moreover, it can be used in a scenario when it is required to fetch the entire content of a file and process it as a whole, or perform any operation at once.
For example, in the given program, we have opened the “GeeksVeda.txt
” file in a read “r
” mode. Then, the “read()
” method is invoked on the “file_object
” for reading and returning the whole file content as a string.
Resultantly, the content will be stored in the “content
” variable and displayed on the console using the print() function. Lastly, the “close()
” method is called for closing the file
file_path = 'GeeksVeda.txt' file_object = open(file_path, 'r') content = file_object.read() print(content) file_object.close()
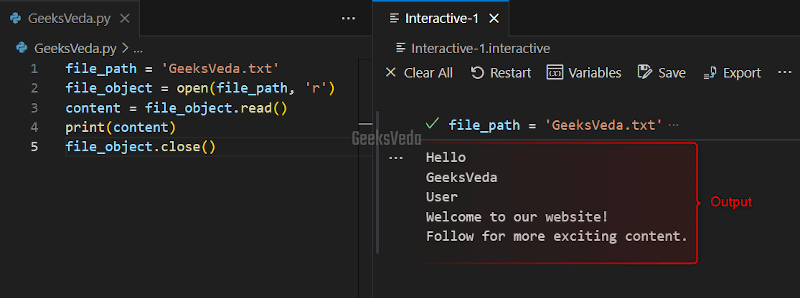
2. Using Python readline() Method
Read a File Line by Line method assists in reading the file line by line. It outputs a string presenting the current line.
However, any conditional statement such as the “while
” loop can be used for printing all file content at once. You can utilize the readline()
method when it is required to process the file line by line.
Now, in the same program, we have invoked the “readline()
” for reading the first line and storing it in the “line
” object. Then, we added a while
loop for iterating through each line. This loop will continue to execute when there exists more lines for reading.
Note that the added print()
function inside the while loop will show each line on the terminal. Lastly, the file will be closed with the “close()
” function.
file_path = 'GeeksVeda.txt' file_object = open(file_path, 'r') line = file_object.readline() while line: print(line) line = file_object.readline() file_object.close()
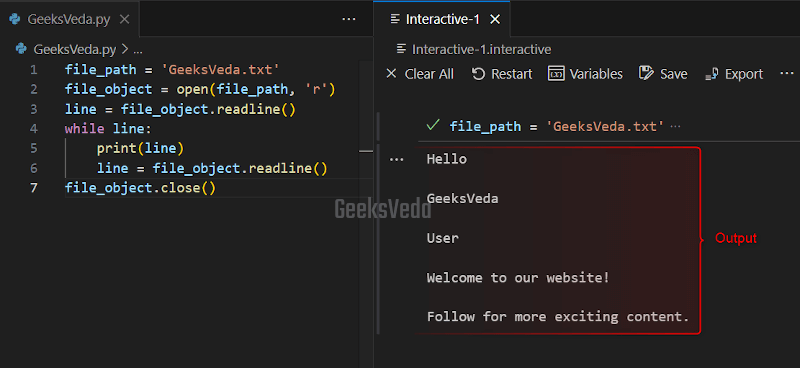
3. Using Python readlines() Method
The “readlines()
” method reads all lines of the file and outputs them as a list of strings. This method is utilized when it is needed to access particular lines of a file or when you need to perform specific batch operations on the file lines.
For instance, here, after opening the “GeeksVeda.txt” file, the “readlines()
” method is called on the “file_object
“. As a result, this method will read all of the lines and display them as a list of strings which will be stored in the “lines
” variable.
Lastly, the lines will be printed in the form of a list and the defined file will be closed.
file_path = 'GeeksVeda.txt' file_object = open(file_path, 'r') lines = file_object.readlines() print(lines) file_object.close()
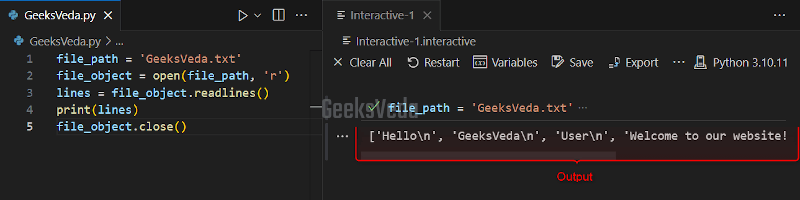
Python File Reading Errors and Their Fixes
During reading files in Python, several errors and exception situations can occur. Therefore, it is crucial for you to handle these situations beforehand to ensure error-free file reading.
Some of the potential errors and their fixes are given in the next subsections.
FileNotFoundError: in Python
This type of error can error when the mentioned file does not exist at the specified path. Therefore to handle it, use the try-except block and define a meaningful error message to your valuable user.
try: file_path = 'xyz.txt' file_object = open(file_path, 'r') # Perform file reading operations file_object.close() except FileNotFoundError: print("Sorry! Specified file does not exist.")
As you can see, in this case, the file has not been found and the corresponding message has been displayed on the console.
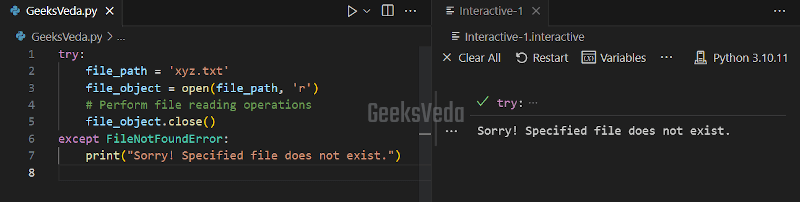
PermissionError: in Python
This type of error is encountered when the program does not have the required permission for accessing the file.
To demonstrate it, we have set “read-only
” access for our “GeeksVeda.txt
” file. Resultantly, when this file will be requested to open in “w
” or write mode, it will display the Permission denied error.
try: file_path = 'GeeksVeda.txt' file_object = open(file_path, 'w') # Perform file reading operations file_object.close() except PermissionError: print("Permission denied. File cannot be accessed.")
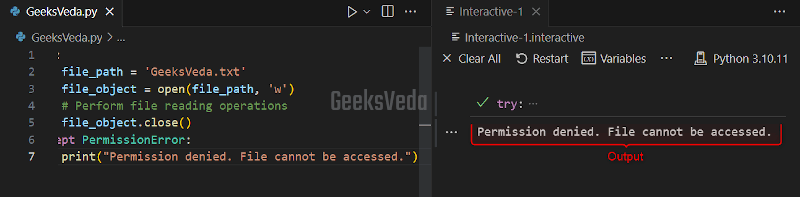
IOError: in Python
IOError occurs because of several types of Input/Output related problems, like a file being in an invalid state or facing a read error. To handle it, utilize a try-except block and define the relevant feedback in the form of a message.
try: file_path = 'xyz.txt' file_object = open(file_path, 'r') # Perform file reading operations file_object.close() except IOError: print("An error occurred while reading the file.")
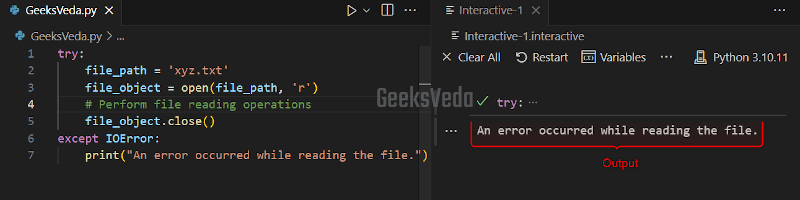
Why is it Important to Close a File After Reading
Have a look at the listed reasons to close a file after reading it in Python.
- Closing a file prevents resource leaks and enhances the system’s performance.
- It permits other processes for accessing the file.
- It also ensures data integrity and saves any changes that are made.
- It is suggested as a good programming practice.
- Moreover, closing files avoids data loss and file corruption.
Bonus Tip: Combine Read and Write Operations in Python
Yes! It is possible to combine read and write operations on a file. This technique permits you to read data from the source file and write it to the destination file. For this, you have to follow the provided steps.
- First of all, open the source file in the read mode “
r
” and the destination file in the write mode “w
“. - Next, read the source file content with the help of a suitable reading method as per your requirements.
- Write the fetched content to the destination file with the
write()
method. - Lastly, close the source and destination files.
- Remember to implement this logic inside the try-except block for handling any potential error.
- For example, we will now read the content of the “
GeeksVeda.txt
” (source file) and write its content in the “testingfile.txt
” (destination file).Here, theread()
method is used for reading the content of the source file, while the “write()
” will write it down to the specified destination file.Moreover, we have also added the relevant message to indicate if the file content has been copied or not.
try: with open('GeeksVeda.txt', 'r') as src_file, open('testingfile.txt', 'w') as destination_file: content = src_file.read() destination_file.write(content) print("File content has been copied successfully.") except FileNotFoundError: print("Source file not found.") except IOError: print("Sorry! An error occurred during file operations.")
It can be observed that the file content has been copied successfully.
Combine Read and Write Operations in Python That’s all essential information regarding reading a file in Python.
Conclusion
File reading is a crucial operation in Python that is performed for retrieving data from files. This operation can be performed using the “read()
“, “readline()
“, or “readlines()
” method as per your requirements.
Moreover, remember to close the files after reading them to prevent resource leaks and data loss.
In the next part of this file handling series, we will check out the method to write to a file in Python (in more detail).
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!