In Python, lambda functions provide a robust and concise approach for defining the anonymous function without the requirements for a formal function definition. With their compact versatility and syntax, lambda functions can be primarily utilized for quick and simple operations.
Through today’s guide, we will be able to understand the lambda function, its syntax, use cases, and alternatives.
What are Lambda functions in Python
Python lambda or anonymous functions can be defined without a name. These functions are specifically utilized for concise and on-the-fly function definitions.
Here is the syntax you should follow for creating lambda functions in Python.
lambda arguments: expressions
The syntax of lambda functions comprises.
- The “
lambda
” keyword. - Followed by a list of “
arguments
“. - A colon
- Lastly, the “
expression
” indicates the function’s body.
How to Use Lambda Functions in Python
In Python, lambda functions are considered for several reasons such as.
- Provides a concise way for defining small, throwaway functions.
- Works with higher-order functions like
filter()
,map()
, andreduce()
. - Simplifies code by avoiding the requirements for defining a separate named function.
- Implements callback functions or event handlers.
For instance, in the provided program, we have defined a lambda function named “square
” for calculating the square of a number. This function accepts “x
” as an argument and outputs its square.
Then, we called this lambda function by passing the value “6
“. Resultantly, it’s square “36
” will be displayed as output.
# Defining a lambda function to calculate the square of a number square = lambda x: x ** 2 # Calling the lambda function result = square(6) print(result)
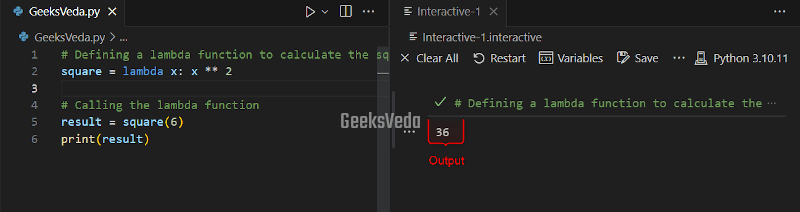
Lambda functions are concise, enabling you to define functions using a single line of code. More specifically, these types of functions can be utilized for performing simple operations, which ultimately minimizes the need for defining separate functions.
Working with Python Lambda Functions
You can also use lambda functions with the Python built-in functions like “map()
“, “filter()
“, and “reduce()
” for performing operations on different iterable objects.
Working with lambda functions in such a way enables you to perform efficient data filtration and transformation operations with minimal code.
Using Lambda Function with map()
In the given code, the map() function applied the specified lambda function to each of the “numbers
” list elements. This resultantly generates a new list of squared values.
# Using lambda function with map() numbers = [1, 2, 3, 4, 5] squared = list(map(lambda x: x ** 2, numbers)) print(squared)
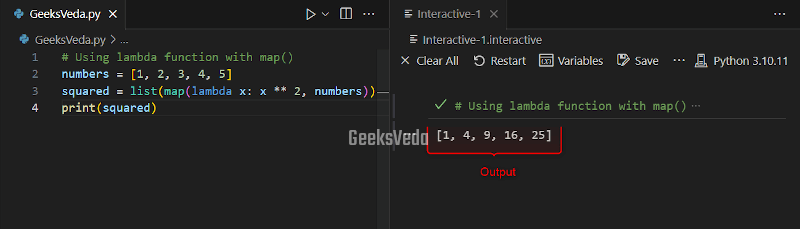
Using Lambda Function with filter()
In this case, the “filter()
” function utilizes the lambda function for filtering out the even numbers from the same “numbers
” list.
numbers = [1, 2, 3, 4, 5] # Using lambda function with filter() even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers)
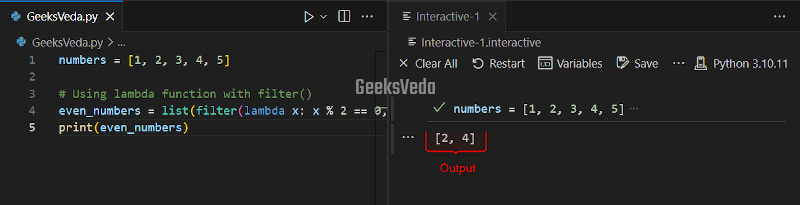
Using Lambda Function with reduce()
Now, we will invoke the “reduce()
” function for applying the lambda function for performing the product of all elements in the “numbers
” list.
from functools import reduce numbers = [1, 2, 3, 4, 5] # Using lambda function with reduce() product = reduce(lambda x, y: x * y, numbers) print(product)
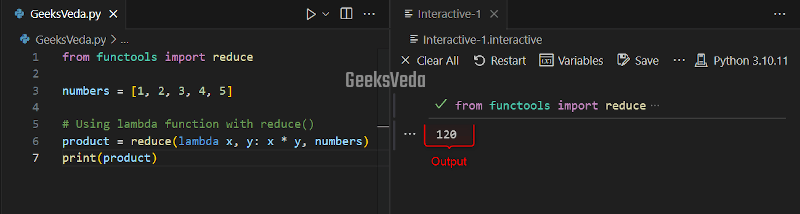
Variable Scope and Closure in Python Lambda Function
The lambda function in Python can access variables from the enclosing scope. This approach is referred to as variable scope and closure.
More specifically, when a lambda function utilizes a variable that is not defined within its own body, it looks for the variable in the enclosing scope where it was defined.
In the provided example, the outer function “outer_function()
” defines a variable named “x
” and outputs a lambda function that adds “x
” to the corresponding argument “y
“.
As the next step, the lambda function, assigned to “inner_function
“, captures the “x
” value from the enclosing scope.
So, when the “inner_function
” is invoked with the argument “15
“, it adds the captured value of “x
“, and displays “25
” as the output of the program.
def outer_function(): x = 10 return lambda y: x + y inner_function = outer_function() result = inner_function(15) print(result)
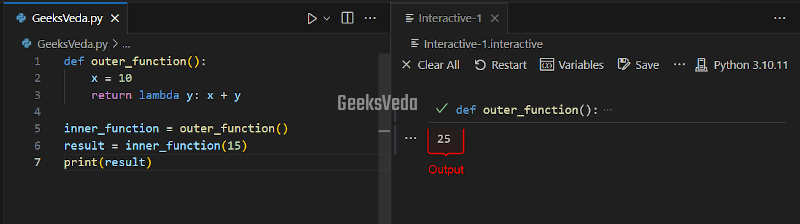
Use Cases for Lambda Functions in Python
While programming in Python, lambda functions can be utilized in practical scenarios where an anonymous function is required. These functions are often employed for filtering, sorting, and transforming data, specifically with built-in functions, such as “filter ()
“, “sorted()
“, and “map()
“.
Let’s explore the above-discussed use cases practically individually.
Sort List Using Lambda Function
In the given program, we have a list of dictionaries named “authors
“.
Then, within the sorted()
function, we have passed the created list as the first argument and the lambda function being the second argument defines the sorting key as the “age
” value of each dictionary.
Resultantly, the “Authors
” list will be sorted with respect to the age key values.
authors = [ {"name": "Sharqa", "age": 25}, {"name": "Ravi", "age": 32}, {"name": "Tulsi", "age": 26} ] # Sorting a list of dictionaries based on a specific key sorted_authors = sorted(authors, key=lambda person: person["age"]) print(sorted_authors)
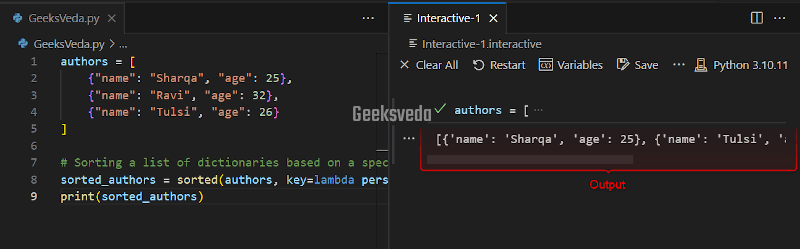
Filter List Using Lambda Function
Here, we have a list named “numbers
” having ten numbers. By using the lambda function, we will filter even numbers from the created list with the filter()
method.
# Filtering a list of numbers to get even numbers numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers)
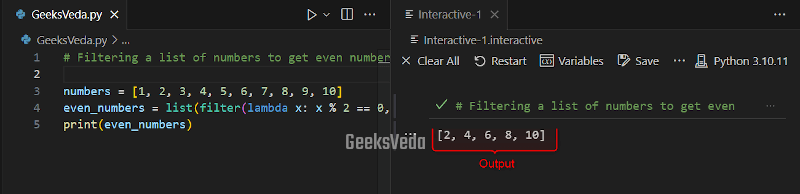
Transform List Using Lambda Function
Now, let’s transform a list of “strings
” to uppercase by invoking the lambda function with the map()
function. Note that the “upper()
” method will assist in performing the required functionality.
# Transforming a list of strings to uppercase strings = ["hello", "geeksveda", "user"] uppercase_strings = list(map(lambda s: s.upper(), strings)) print(uppercase_strings)
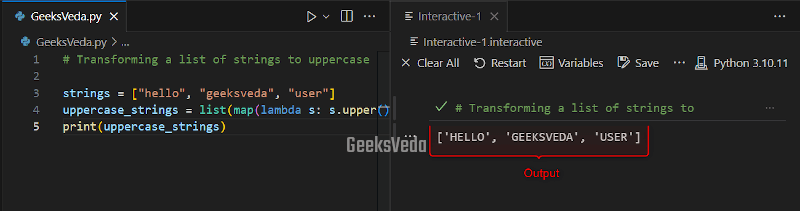
Limitations of Lambda Functions in Python
Have a look at some of the limitations of lambda functions in Python.
- Limited to single expressions, restructuring complex language.
- Lack of extensive exception management and error handling.
- Debugging can be challenging due to its anonymous nature.
- Limited code reusability and maintainability compared to named functions.
- Readability can be compromised with nested or complex lambda functions.
Alternatives to Lambda Function in Pythons
As mentioned earlier that while dealing with complex scenarios, the lambda function may not be the best fit. So there are other alternative techniques or approaches that can be considered.
- Named Functions
- Classes and Methods
- Comprehensions and Generators
- Decorators
Named Function
Defining a named function offer more readability and flexibility. These types of functions permit multiple statements, better error handling, and complex logic. The named functions are particularly utilized in those scenarios where it is required to reuse the logic or when the codebase becomes more extensive.
Here, we have defined a named function “add_numbers
” for adding two numbers. This function accepts two arguments “x
” and “y
” and outputs their sum.
def add_numbers(x, y): return x + y result = add_numbers(3, 4) print(result)
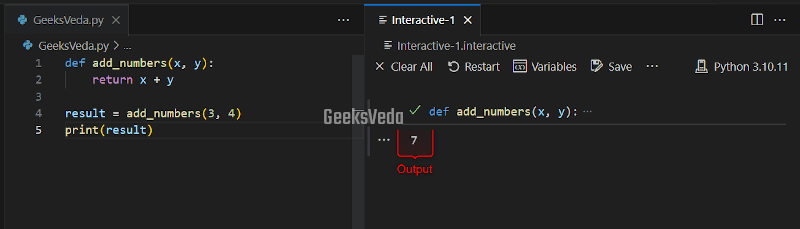
Classes and Methods in Python
In OOP, classes and methods provide a more organized and structured approach to handling complex scenarios.
More specifically, classes encapsulate the relevant functionality and permit you to implement more sophisticated logic. On the other hand, the methods within the classes can encapsulate different operations which significantly improves the readability of the code.
In the provided code, we have defined a class name “Calculator
” with the “add_numbers
” method for performing the addition operations. This class supports a way for encapsulating the related functionality and can handle more complex operations.
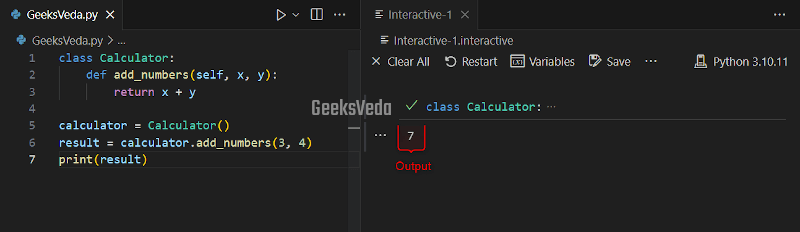
Comprehensions and Generators in Python
In case, if it is required to transform data, filter, set comprehensions, list comprehensions, or generator expression can offer a more readable and expressive solution.
Moreover, comprehension permits concise iteration over the iterable objects and provides the facility for applying complex transformations or conditions.
Here, list comprehension is utilized for creating a new list named “squared_numbers
” by squaring each element in the “numbers
” list.
numbers = [1, 2, 3, 4, 5] squared_numbers = [x ** 2 for x in numbers] print(squared_numbers)
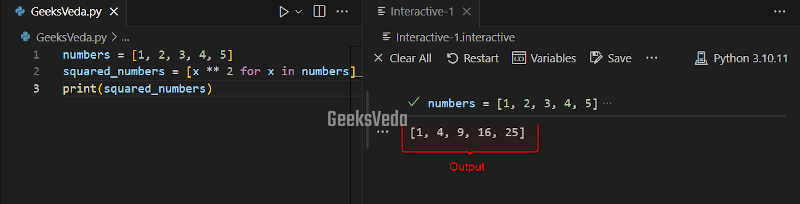
Decorators in Python
Sometimes, you need to modify the function by wrapping it with additional code. In such cases, the decorator functions can be utilized for implementing concepts like authentication, caching, or logging.
For instance, here, the “log_function_call
” function has been defined for logging the name of the invoked function. In this scenario, the “greet()
” function has been decorated with “@log_function_call
“.
When the function is invoked, the decorator adds the logging behavior before running the original function.
def log_function_call(func): def wrapper(*args, **kwargs): print(f"Calling function: {func.__name__}") return func(*args, **kwargs) return wrapper @log_function_call def greet(name): print(f"Hello, {name}!") greet("GeeksVeda User")
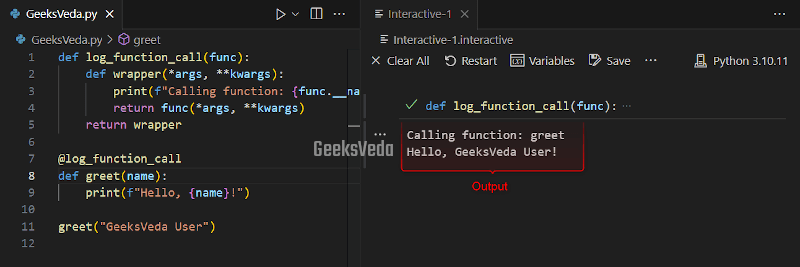
Conclusion
The lambda function in Python offers an efficient and compact way of defining anonymous functions. They are utilized for simplifying the code, working with higher-order functions, and handling simple operations.
However, their limitations in handling extensive errors and complex error, makes them appropriate for simple, single-expression tasks in Python.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!