Python Numpy or Numerical Python is considered a fundamental library for numerical computing. It supports high-performance array operations and several mathematical functions, which makes it invaluable for data manipulation, machine learning tasks, and scientific computing,
By providing optimized algorithms and efficient data structures, NumPy allows you to work with large datasets and perform complex operations without any hassle.
What is NumPy in Python
In Python, NumPy or Numerical Python is a robust library that offers support for large, multi-dimensional matrices and arrays, including the collection of mathematical functions that can be used for operating on these arrays in an efficient manner.
It is considered a crucial tool for data analysis and scientific computing.
How to Install Python NumPy Library
For the purpose of installing NumPy, follow these steps.
1. Usingpip
– First, open your Command Prompt and write out the following command.
pip install numpy
As a result, the latest version of the NumPy will be downloaded and installed from the Python Package Index (PyPI).
2. Using Anaconda
– In case, if you want to install NumPy using Anaconda, first create a new environment or you can activate the existing one. Then, execute this command.
conda install numpy
NumPy Arrays in Python
NumPy Arrays are the cornerstone of the NumPy library and offer manipulations and the storage of large numerical datasets. You can utilize them to create.
- One-Dimensional Array
- Two-Dimensional Array
- Three-Dimensional Array
Check out the given sub-sections for practical demonstration.
Create One-Dimensional NumPy Array
For instance, we will now create a one-dimensional NumPy array named “arr
” from a Python list. After that, the “np.array()
” method is called for converting the list into an array. The resultant array will be then shown with the “print() function”.
import numpy as np # Creating a 1-dimensional array from a Python list arr = np.array([11, 22, 33, 44, 55]) print("1-dimensional array:", arr)
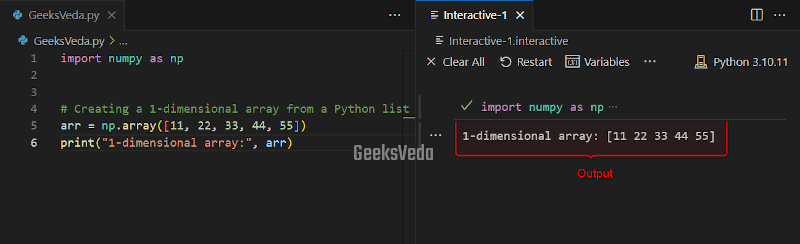
Create Two-Dimensional NumPy Array
Now, we will create a two-dimensional NumPy array called “arr_2d
” from a nested list. This nested list indicates the rows of the array and each inner list corresponds to a specified row.
Then, the “np.array()
” method converts this nested list into a two-dimensional array.
import numpy as np # Creating a 2-dimensional array from a nested list nested_list = [[11, 22, 33], [44, 55, 66]] arr_2d = np.array(nested_list) print("2-dimensional array:", arr_2d)
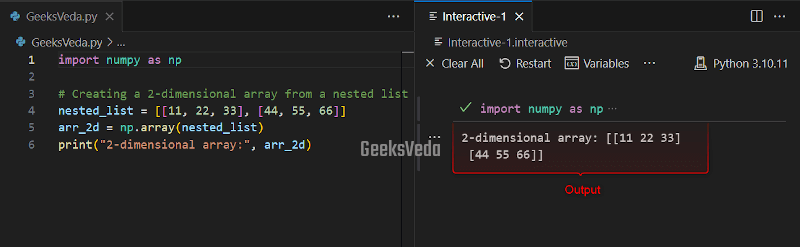
Create Three-Dimensional NumPy Array
The last scenario is to create a three-dimensional NumPy array named “arr_3d
” from a nested list. This list indicates the three dimensions of the array.
Each innermost list corresponds to the third dimension elements, where the middle lists represent the second dimension, and the outermost list represents the first dimension.
Then, the “np.array()
” method has been invoked to convert the nested list into a 3-dimensional array.
import numpy as np # Creating a 3-dimensional array from a nested list nested_list_3d = [[[11, 22], [33, 44]], [[55, 66], [77, 88]]] arr_3d = np.array(nested_list_3d) print("3-dimensional array:", arr_3d)
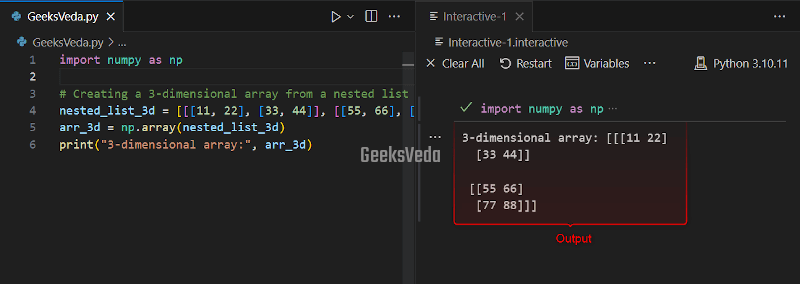
Perform Python NumPy Array Operations
To perform operations on arrays, you may need NumPy as it supports multidimensional arrays, offers a wide range of mathematical functions, and efficient computations, enables broadcasting for element-wise operations, and it also integrates with other scientific computing libraries.
Additionally, it improves the performance of array operations, which makes it a crucial tool for tasks, like data manipulation, numerical computations, and scientific analysis.
Let’s check out some useful methods of the NumPy library.
Numpy arange() Method in Python
The “arange()
” method of the NumPy library generates an array having a sequence of numbers based on the defined range.
Here, we will create an array that starts from “1
” and ends at “10
” (excluding 11). To do so, pass 1 and 11 as the arguments to the “arange()
” method. Lastly, display the resultant array on the console.
import numpy as np # Using np.arange() arr_arange = np.arange(1, 11) print("arr_arange:", arr_arange)
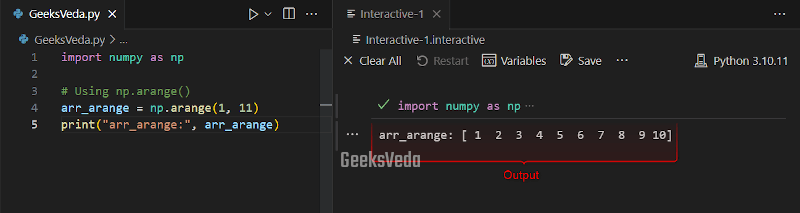
NumPy zeros() Method in Python
The “np.zeros()
” method creates an array filled with zeros. For instance, we will now generate an array of size 5 having zeros as follows.
import numpy as np # Using np.zeros() arr_zeros = np.zeros(5) print("arr_zeros:", arr_zeros)
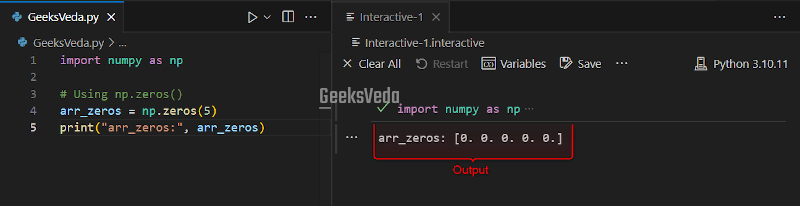
NumPy ones() Method in Python
“np.ones()
” method can be utilized for creating an array filled with ones. For instance, we will now create a 2×3 array filled with ones by invoking the “np.ones(2, 3)
” method.
import numpy as np # Using np.ones() arr_ones = np.ones((2, 3)) print("arr_ones:", arr_ones)
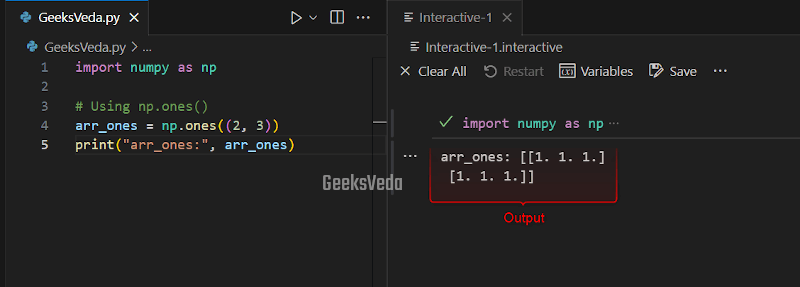
NumPy linspace() Method in Python
The “linspace()
” method can be used for generating an array with evenly spaced values within the given range. Like a harem we will create an array of size 10 with values ranging from 0 to 1, evenly spaced.
np.linspace(0, 1, 10)
“.import numpy as np # Using np.linspace() arr_linspace = np.linspace(0, 1, 10) print("arr_linspace:", arr_linspace)
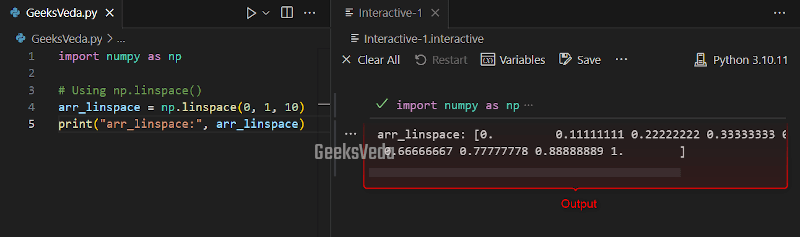
NumPy reshape() Method in Python
“reshape()
” method changes or updates the array shape without modifying its data.
For example, now, we will reshape the created 1-dimensional array named “arr
” of 2×3 dimension. The corresponding array will be displayed on the console.
import numpy as np # Using np.reshape() arr1 = np.array([1, 2, 3, 4, 5, 6]) reshaped_arr = np.reshape(arr1, (2, 3)) print("reshaped_arr:") print(reshaped_arr)
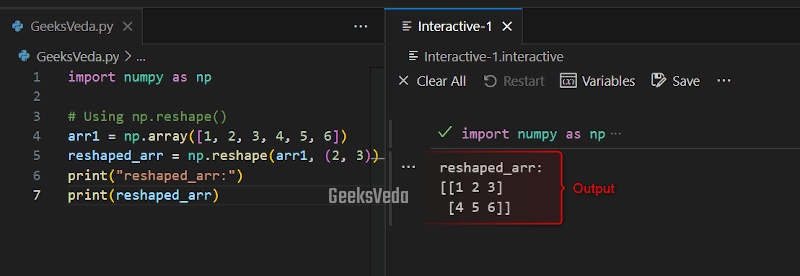
NumPy concatenate() Method in Python
The “np.concatenate()
” combines the defined multiple arrays into a single one. For instance, in this scenario, we will concatenate “arr1
” and “arr2
” along with the first axis (row-wise).
import numpy as np arr1 = np.array([1, 2, 3, 4, 5, 6]) # Using np.concatenate() arr2 = np.array([7, 8, 9]) concatenated_arr = np.concatenate((arr1, arr2)) print("concatenated_arr:") print(concatenated_arr)
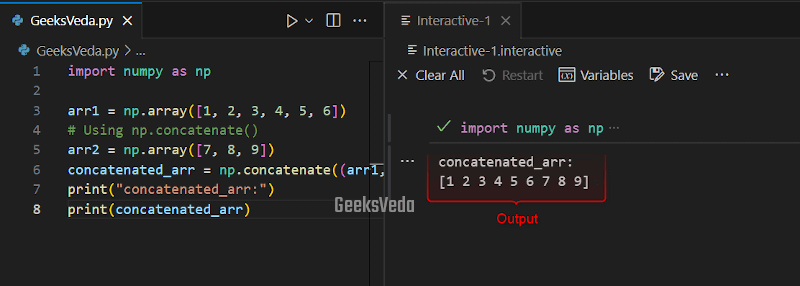
NumPy random.rand() Method in Python
The “random.rand()
” method creates an array of random numbers between 0 and 1. In this case, the “np.random.rand()
” method generates a 3×4 array filled with random numbers between 0 and 1.
import numpy as np # Using np.random.rand() random_arr = np.random.rand(3, 4) print("random_arr:") print(random_arr)
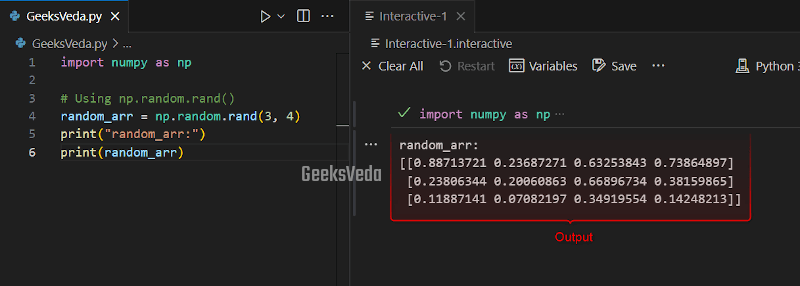
NumPy random.randint() Method in Python
“random.randint()
” method, on the other hand, has the facility of generating random numbers according to the specified range.
For instance, “np.random.randint(1, 10, size=5)
” produces a new array of size 5 having integers between 1 and 10.
import numpy as np # Using np.random.randint() random_int = np.random.randint(1, 10, size=5) print("random_int:") print(random_int)
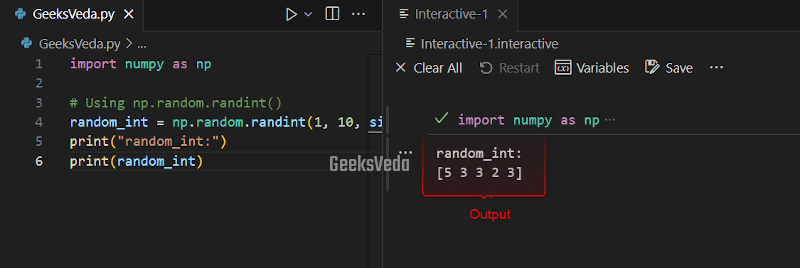
NumPy max() Method in Python
“max()
” outputs the maximum value from an array. Here, the np.max()
method will find out the maximum value in the “arr
” array.
import numpy as np arr = np.array([4, 8, 2, 6, 1, 9]) # Using np.max() max_val = np.max(arr) print("Maximum value:", max_val)
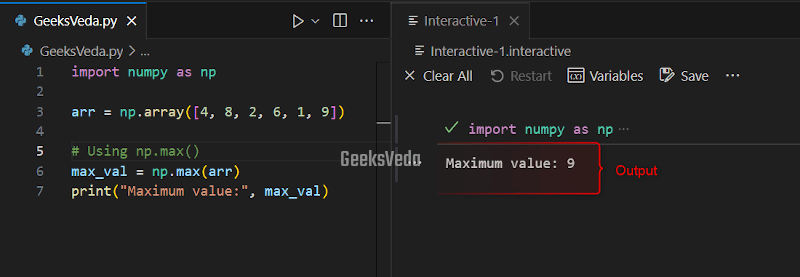
NumPy min() Method in Python
Likewise, the “min()
” method of the NumPy library outputs the minimum value that is present in the given array.
import numpy as np arr = np.array([4, 8, 2, 6, 1, 9]) # Using np.min() min_val = np.min(arr) print("Minimum value:", min_val)
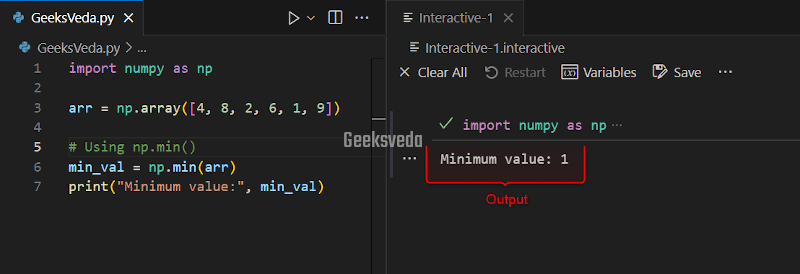
NumPy mean() Method in Python
“mean()
” evaluates the mean or average of the array. Here, in this case, the np.mean()
method will return “5.0” as the mean value of the “arr
” array.
import numpy as np arr = np.array([4, 8, 2, 6, 1, 9]) # Using np.mean() mean_val = np.mean(arr) print("Mean value:", mean_val)
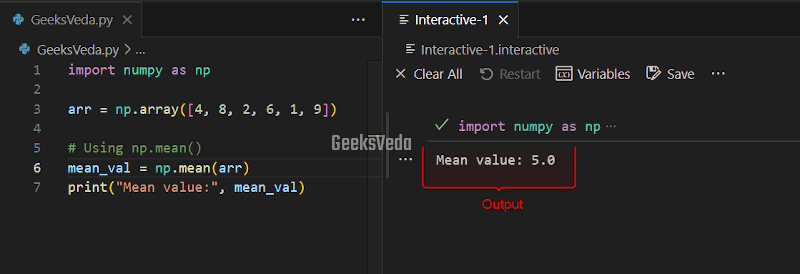
NumPy median() Method in Python
In this example, “np.median()
” calculates the median of the “arr
” array which is “5.0
“.
import numpy as np arr = np.array([4, 8, 2, 6, 1, 9]) # Using np.median() median_val = np.median(arr) print("Median value:", median_val)
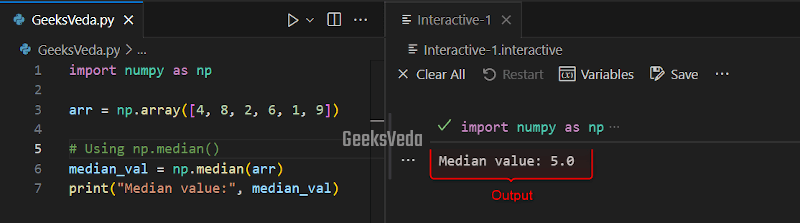
NumPy sum() Method in Python
Let’s calculate the sum of all array “arr
” elements with the “sum()
” NumPy method.
import numpy as np arr = np.array([4, 8, 2, 6, 1, 9]) # Using np.sum() sum_val = np.sum(arr) print("Sum value:", sum_val)
It can be observed that the sum has been displayed as “30
“.
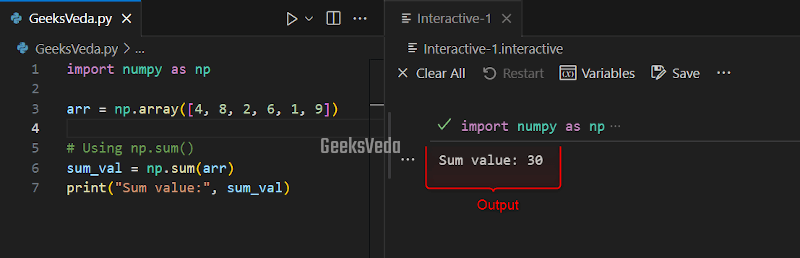
NumPy std() Method in Python
The “np.std()
” method can be invoked for calculating the standard deviation of an array. For instance, here, “np.std(arr)
” evaluates the standard deviation of the “arr
” array.
import numpy as np arr = np.array([1, 2, 3, 4, 5, 6]) std_val = np.std(arr) print("Standard deviation:", std_val)
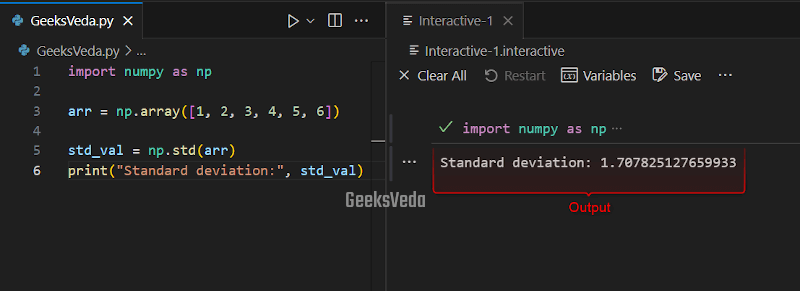
Numpy var() Method in Python
“np.var()
” evaluates the variance of the array which has been invoked in the below-provided example.
import numpy as np arr = np.array([1, 2, 3, 4, 5, 6]) var_val = np.var(arr) print("Variance:", var_val)
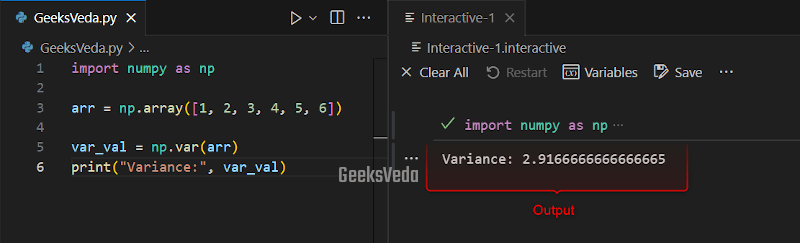
Numpy unique() Method in Python
“unique()
” method of the NumPy method finds out the unique array elements.
In this example, the “np.unique()
” method has been invoked for identifying the unique elements in the “arr
” array.
import numpy as np arr = np.array([1, 2, 3, 2, 4, 3, 5]) unique_vals = np.unique(arr) print("Unique values:", unique_vals)
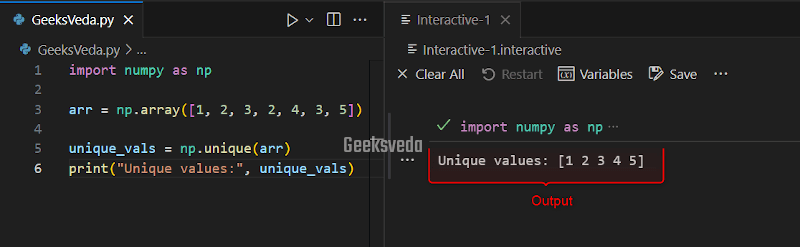
NumPy dot() Method in Python
NumPy “dot()
” method performs matrix multiplication between two arrays. For instance, we have invoked “np.dot(arr1, arr2)
” for performing the matrix multiplication between “arr1
” and “arr2
“.
import numpy as np arr1 = np.array([[1, 2], [3, 4]]) arr2 = np.array([[5, 6], [7, 8]]) dot_product = np.dot(arr1, arr2) print("Dot product:") print(dot_product)
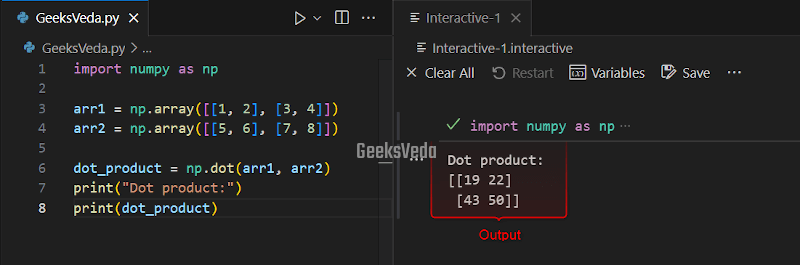
NumPy transpose() Method in Python
In order to swap the axes of an array, utilize the “transpose()
” method as follows.
import numpy as np arr1 = np.array([[1, 2], [3, 4]]) transposed_arr = np.transpose(arr1) print("Transposed array:") print(transposed_arr)
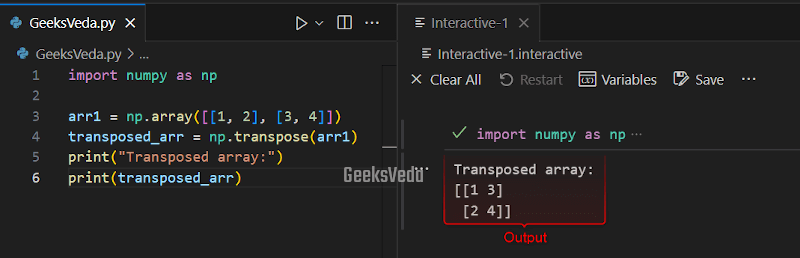
Numpy save() Method in Python
For the purpose of saving an array to a binary file, call the “save()
” method of the NumPy library. Here, we will save the “arr
” array to the binary file named “my_array.npy
“.
import numpy as np arr = np.array([1, 2, 3, 4, 5]) # Saving arr to a binary file called 'my_array.npy' np.save('my_array.npy', arr)
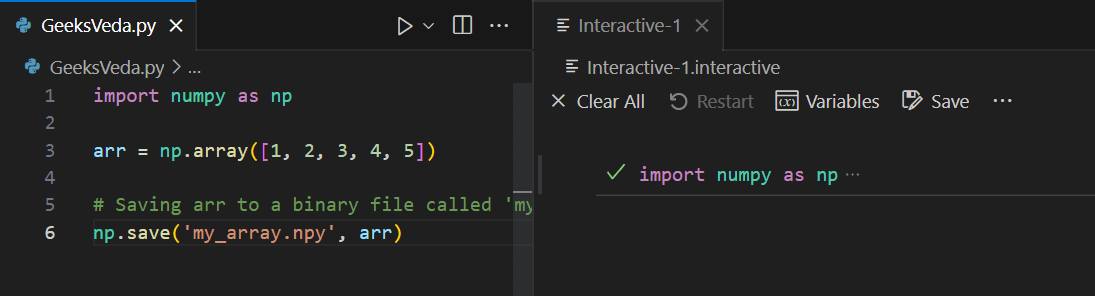
NumPy load() Method in Python
Likewise, to load()
an array from a saved binary file, call the “load()
” method. In this scenario, the “np.load()
” method loads the saved array from the “my_array.npy
” file.
import numpy as np # Loading the saved array from 'my_array.npy' loaded_arr = np.load('my_array.npy') print("Loaded array:", loaded_arr)
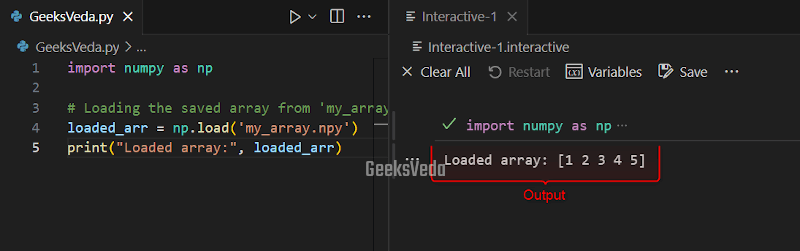
NumPy where() Method in Python
Want to find out the indices of the array elements that satisfy the given condition? Utilize the “where()
” method.
For instance, we have now invoked the “np.where()
” method for finding the indices where the “arr
” array elements are greater than 3.
import numpy as np arr = np.array([1, 2, 3, 4, 5, 6]) # Finding indices where elements are greater than 3 indices = np.where(arr > 3) print("Indices where elements are greater than 3:", indices)
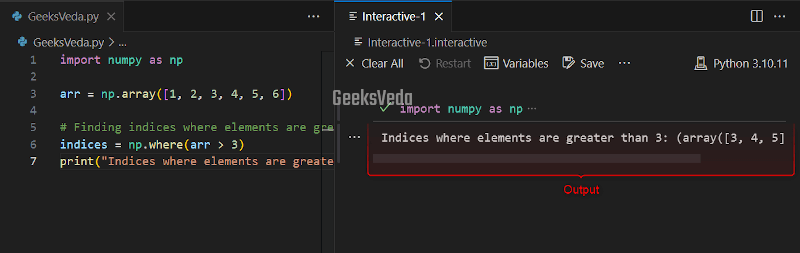
NumPy sort() Method in Python
“np.sort()
” method can be used for sorting the array elements in ascending order. For example, here the sort()
method sorts the elements of the “arr
” array in ascending order.
import numpy as np arr = np.array([5, 2, 8, 1, 6]) # Sorting the array in ascending order sorted_arr = np.sort(arr) print("Sorted array:", sorted_arr)
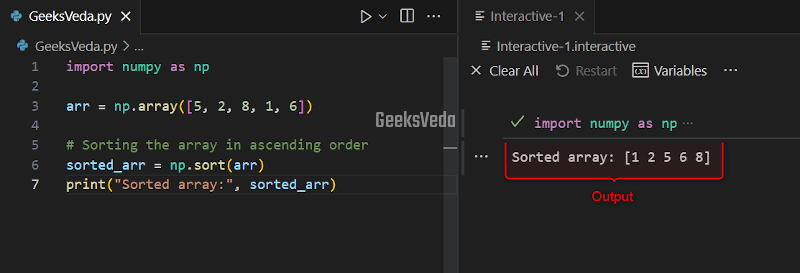
NumPy searchsorted() Method in Python
“searchsorted()
” method of the NumPy library is invoked for performing a binary search and outputs the index where an element should be inserted to maintain the sorted order.
import numpy as np arr = np.array([5, 2, 8, 1, 6]) sorted_arr = np.sort(arr) # Performing a binary search and returns the index of the element index = np.searchsorted(sorted_arr, 6) print("Index of 6:", index)
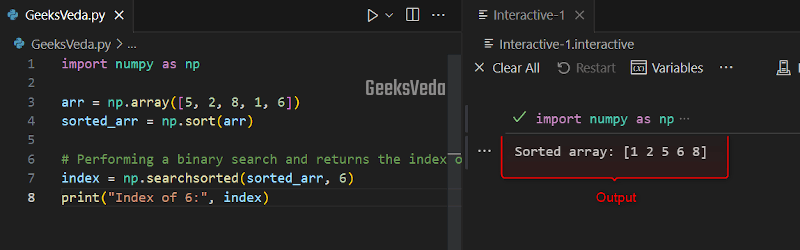
NumPy nanmean() Method in Python
“nanmean()
” method calculates the mean of the array and ignores the “NaN
” values. Here, in the provided example, the “np.nanmean(arr)
” method evaluates the mean of the “arr
” array and excludes the “NaN
” values.
import numpy as np arr = np.array([1, 2, np.nan, 4, 5]) # Calculates the mean, ignoring NaN values mean_val = np.nanmean(arr) print("Mean value:", mean_val)
As you can see that the resultant mean value has been displayed on the console.
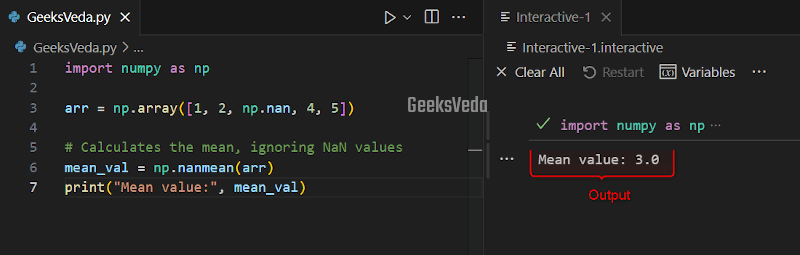
That’s all from this effective guide related to the Python NumPy library.
Conclusion
Python NumPy is a crucial library for efficient numerical computing. It offers a powerful array of manipulation functionalities handling missing data, and performing data analysis tasks.
Therefore, mastering NumPy is crucial for scientific computing, data manipulation, and machine learning in Python.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!