Dictionaries in Python offer efficient data storage and retrieval with the help of key-value pairs.
So, in today’s guide, we will explore dictionary creation, the approaches to accessing and modifying its elements, essential methods, operations, and comprehension. Let’s unlock the capabilities of Python dictionaries and be ready to utilize them in your program.
What is a Dictionary in Python
A Python dictionary is referred to as an unordered collection of key-value spares. More specifically, each key is unique and it has a corresponding value in the defined collection. This data structure is called an associative array, a hash map, or a simple dict.
You can also implement dictionaries in Python using the hash tables which offer a constant-time average-case complexity for key inserts, lookups, and deletes.
Create and Initialize Dictionary in Python
Python supports different methods for creating dictionaries. However, the most common approach is to embed the key-value pairs in the curly braces “{}
“.
For instance,
my_dict = {"key1": value1, "key2": value2}
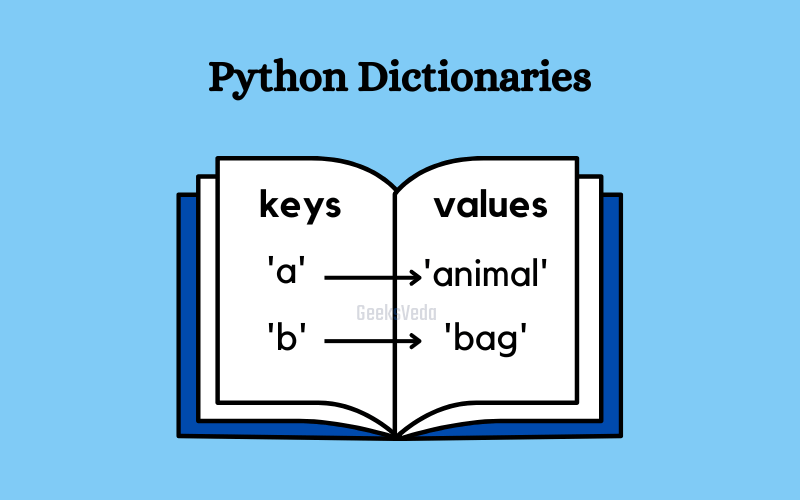
Additionally, you can also invoke the “dict()
” constructor for creating a dictionary like this.
my_dict = dict(key1=value1, key2=value2)
Another method is to create dictionaries from any other iterable objects or data structure.
For instance, you can combine two lists into a dictionary with the help of the zip() function.
keys = ["name", "age", "city"] values = ["Sharqa", 25, "Paris"] my_dict = dict(zip(keys, values)) print(my_dict)
As a result, you will have a dictionary named “my_dict
” comprising three key-value pairs.
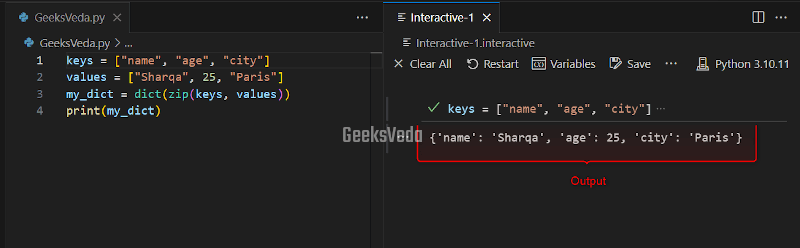
Access and Modify Dictionary Elements in Python
For the purpose of accessing values in a Python dictionary, utilize its corresponding key. For instance, we have a dictionary named “my_dict
“. Now, to fetch the associated value with the “name
” key, we will write “my_dict["name"]
“, which will output the added name.
However, if the key is not present in the created dictionary, “KeyError
” will be raised. So, in order to handle the missing keys issue without encountering an error, you can call the “get()
” method.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} name = my_dict.get("name") print ("Name: ", name) occupation = my_dict.get("occupation") print ("Occupation: ", occupation)
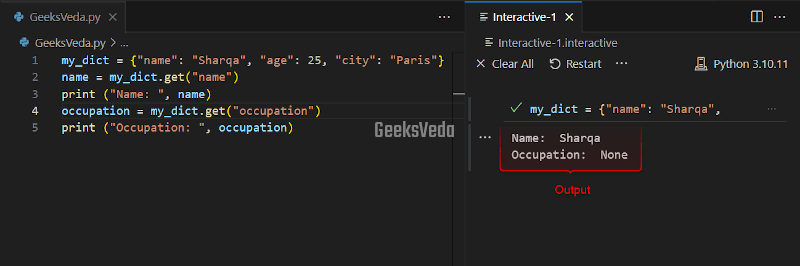
Moreover, you can also specify a default value that can be displayed in case the added key is found as we defined “Unknown
” for the “occupation
” key that does not exist in the “my_dict
“.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} name = my_dict.get("name") print ("Name: ", name) occupation = my_dict.get("occupation", "Unknown") print ("Occupation: ", occupation)
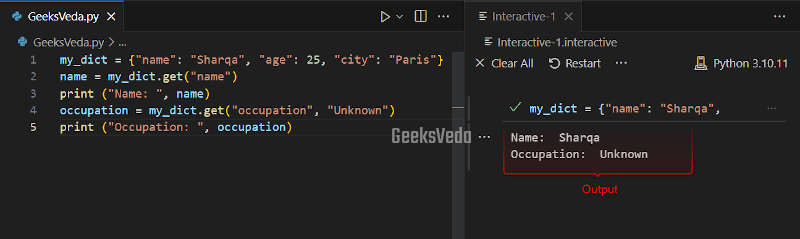
Likewise, to add or modify a key-value pair in a Python dictionary, assign a value to the required key. For instance, in the provided code, we will modify the value of the “age
” key.
print ("Name: ", name) my_dict["age"] = 26 # Modifies the value associated with the "age" key age = my_dict.get("age") print ("Age: ", age)
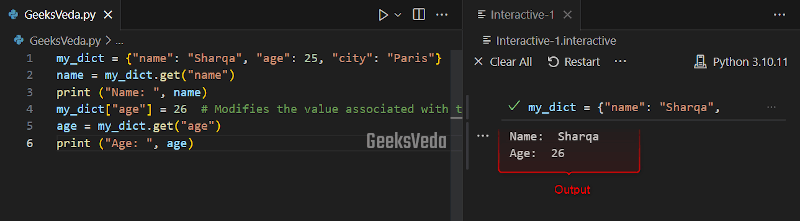
Dictionary Methods in Python
Python dictionary supports several operations and their relevant methods that can be utilized for retrieving or manipulating data. Have a look at the most essential dictionary methods and their operation.
Using clear() Dictionary Method
The “clear()
” method is invoked for clearing all key-value pairs from the created dictionary which ultimately makes it empty. More specifically, it updates the dictionary in place.
Here, we have called the “clear()
” method to empty the created “my_dict
” Python dictionary that has three key-value pairs.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} my_dict.clear() print(my_dict)
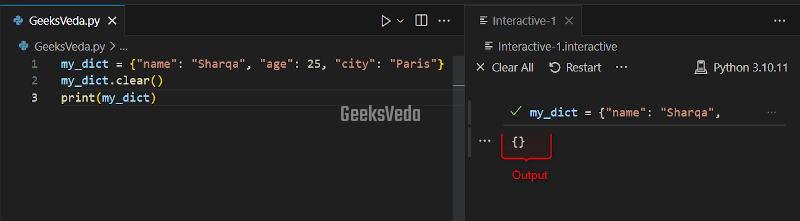
Using get() Dictionary Method
The “get()
” method fetches the value linked with a particular key. If the key is not present in the dictionary, it outputs the optional default value, which is passed as the second argument. However, in case, if no default value is given, this method will return “None
“.
Now, we have the “get()
” method for retrieving the value of the “name
” key.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} name = my_dict.get("name") print("Name: ", name)
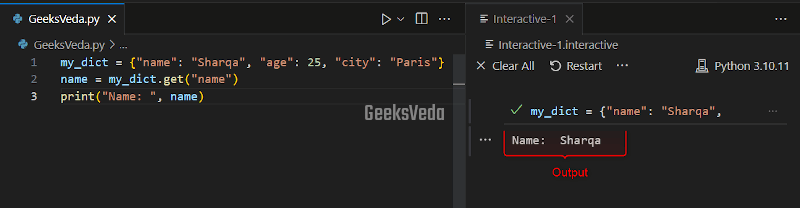
Using items() Dictionary Method
The “items()
” method outputs a new view object that comprises all key-value pairs of the defined dictionary. This view object can be then utilized for iterating over the items, or you can also convert them into a list of tuples.
In the given program, we have called the “items()
” method with a “for loop” for iterating over the key-value pairs of the “my_dict
” dictionary.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} for key, value in my_dict.items(): print(key, value)
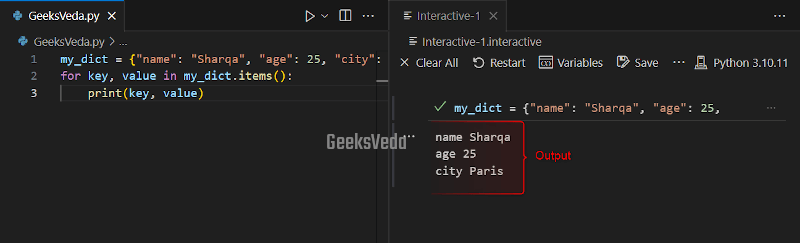
Using keys() Dictionary Method
This method outputs a view object that comprises all the dictionary keys. These keys can be then converted into a list or used for iteration.
For instance, here, the “key()
” method will output the list of keys present in the “my_dict
” dictionary.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} keys = my_dict.keys() for key in keys: print(key)
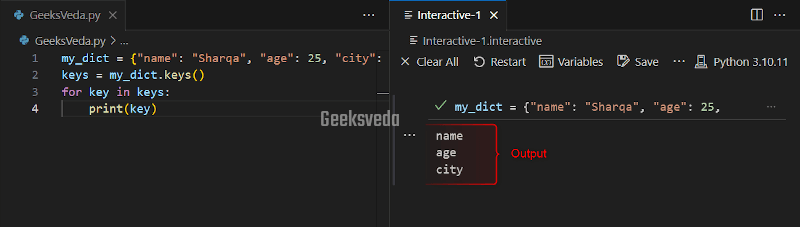
Using values() Dictionary Method
Similarly, you can utilize the “values()
” method for iteration over the values of the defined dictionary. This method outputs a view object that contains all dictionary values.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} values = my_dict.values() for value in values: print(value)
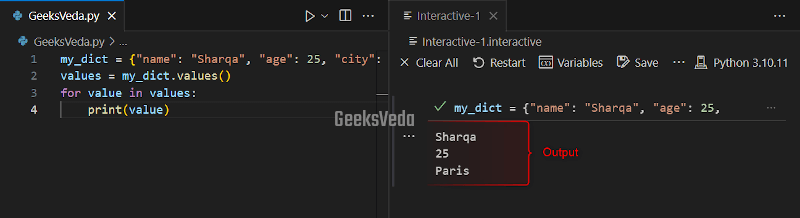
Using pop() Dictionary Method
The “pop()
” method is utilized for removing and returning the value associated with a particular key from the dictionary. If the key is not present, it outputs the optional default value which has been passed as the second argument. However, if no second argument is defined, the “KeyError
” will be raised.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} age = my_dict.pop("age") # Removes and returns 25 print("Age: ", age) occupation = my_dict.pop("occupation", "Unknown") # Returns "Unknown" as the default value print("Occupation: ", occupation)
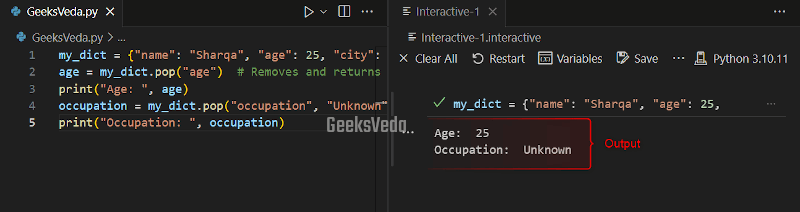
Using popitem() Dictionary Method
The “popitem()
” method of the Python dictionary removes an arbitrary key-value pair from the dictionary. This method can be used in scenarios, where it is required to delete or remove an item without mentioning a specific key.
Here, the “popitem()
” will remove the last key-value pair from the “my_dict
” dictionary.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} key, value = my_dict.popitem() print(my_dict)
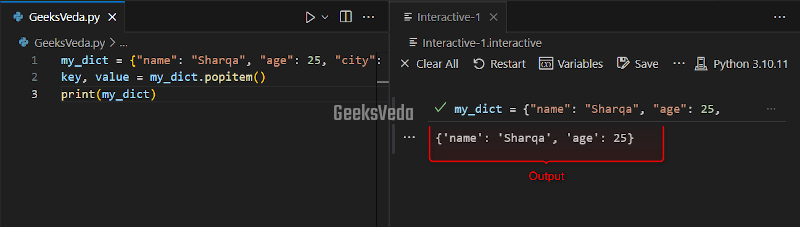
Using update() Dictionary Method
In order to update the dictionary with any other iterable object or key-value pairs, the “update()
” method can be used. If the key already exists, its value will be modified. Otherwise, a new key-value pair will be added to the dictionary.
Now, we will add a new key-value pair and update the “my_dict
” dictionary.
my_dict = {"name": "Sharqa", "age": 25, "city": "Paris"} new_data = {"city": "Paris", "occupation": "Author"} my_dict.update(new_data) print(my_dict)
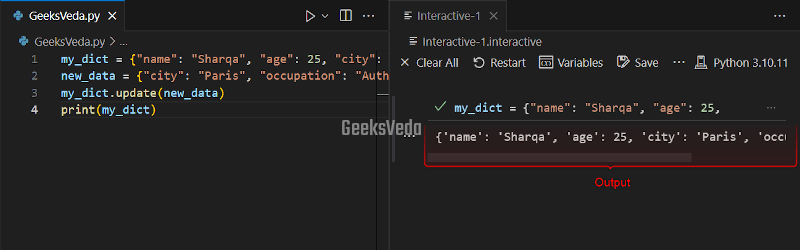
Python Dictionary Comprehension
In Python, dictionary comprehension offers an efficient and concise way of creating dictionaries. They follow the same syntax to list comprehensions, however, generate or produce key-value pairs instead.
Additionally, this approach permits you to create dictionaries in a single line of code.
The syntax of dictionary comprehension involves mentioning the key-value pairs separated by the colon and embedding them within curly braces “{}
” as follows
my_dict = {key: value for key, value in iterable}
Let’s explore this concept more with some examples.
Filter Data with Dictionary Comprehension
In this example, the dictionary comprehension approach is utilized for filtering out the even numbers from the “numbers
” list. It then creates a dictionary where the keys represent the even number and the corresponding values indicate their squares.
numbers = [1, 2, 3, 4, 5, 6, 7] even_squares = {num: num**2 for num in numbers if num % 2 == 0} print(even_squares)
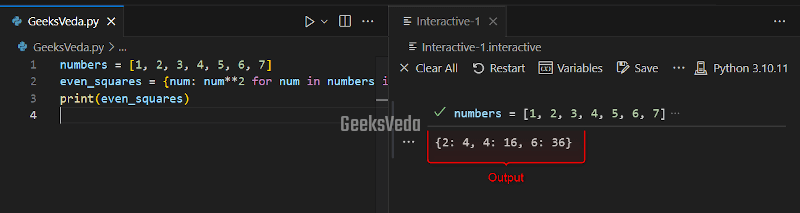
Transform Data with Dictionary Comprehension
In this scenario, dictionary comprehension creates a dictionary where the keys are the same as the “names
” list, and the respective values represent the length of the “names
” keys.
names = ["Sharqa", "Ravi", "Anusha"] name_lengths = {name: len(name) for name in names} print(name_lengths)
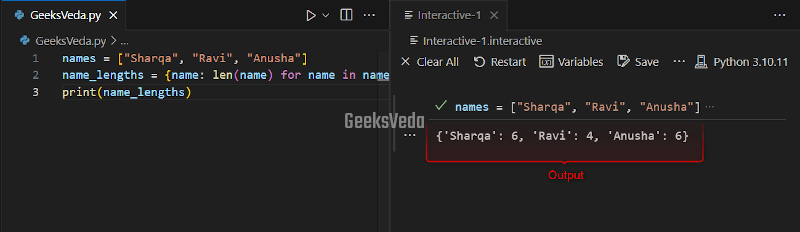
That’s all from this effective guide related to Python dictionaries.
Conclusion
Python dictionaries are a robust data structure that permits the efficient storage, fetching, and manipulation of data with key-value pairs. They provide multiple methods and operations for accessing, modifying, and iterating over the dictionary elements.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!