Python is a versatile programming language that offers a variety of tools to assist developers to write efficient and effective code. More specifically, one of the most essential tools is the for loop
, which allows you to iterate over sequences, perform complex operations, and manipulate data as per your requirements.
In this blog, we will thoroughly discuss the usage of the for loop
in Python, including its syntax, various techniques for iterating over sequences, and how to apply it to different scenarios.
What is a for loop in Python?
In Python, a for loop
is a type of control flow statement that permits you to execute a block of code according to the specified number of iterations. It is primarily used for iterating over a collection of items, such as a tuple or a list, and performing the same operation on each item.
The for loop
works by assigning or allocating each item in the collection to a variable and running the code block for each item. It can be utilized in the case scenarios where it is required to perform a repetitive task, such as generating a sequence of numbers or processing data.
To use the for loop
in Python, follow the provided syntax.
for variable in iterable: # do something
Here:
- The
for
keyword is utilized to start afor loop
, followed by a variable that will hold each value in the iterable. - This iterable represents an items’ collection that the loop will iterate over.
- Finally, the body of the loop contains the instructions or statements that need to be executed for each iteration.
How Does for loop Work in Python?
Below-listed steps will explain the working of the for loop
in Python with respect to the discussed syntax and the given flowchart:
- First of all, the
for loop
will be initialized with a sequence of items for the iteration. This sequence can be a string, tuple, or list. - In the next step, set the
loop
variable according to the first item in the sequence. - Note that the code or the statements added inside the
loop
is executed with theloop
variable set to the current item in the sequence. - After executing the code block, the
loop
variable is updated to the next item in the sequence. - The
for loop
continues to perform the iteration over the sequence until there are no more items left.
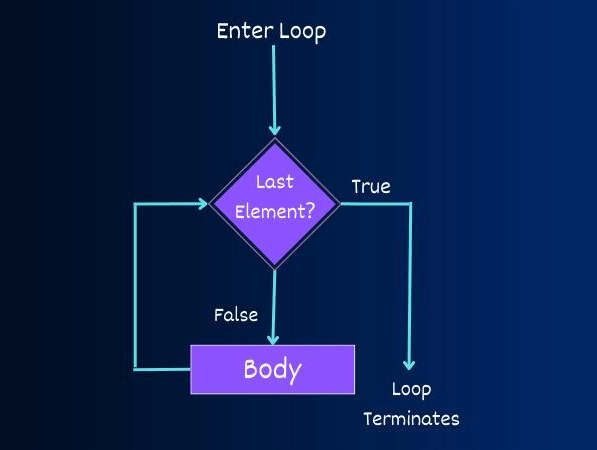
Now, let’s check out some examples to practically understand the usage of the for loop
in Python.
1. Use a for loop in Python with range() Function
Python range()
function creates a sequence of numbers. It accepts three arguments: start, stop, and step, where start refers to the sequence’s starting value (which is 0 by default), stop specifies the sequence’s stopping value (not included in the sequence), and step is the sequence’s step value (the default is 1).
For instance, we will now use a for loop
with the range()
function to print the numbers from 5 to 10.
for i in range(5, 10): print(i)
It can be observed for loop iterated over the given range successfully and the numbers 5, 6, 7, 8, 9, and 10 have been displayed:
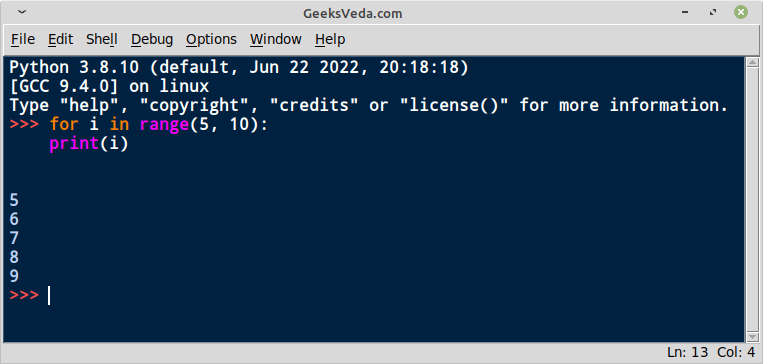
2. Use a for loop in Python to Iterate over Collections
You can also utilize the for loop
to iterate or repeatedly perform execution over a collection, like a dictionary, a tuple, or a list. For each item in the created collection, the for loop
assigns the value to a variable that can be used in the code block.
Example 2.1: Iterating Over a List With for loop
Let’s have a look at the working of the for loop
with the following names list.
names = ['Ravi', 'Kennedy', 'Sharqa'] for name in names: print(name)
According to the given code, the for loop
iterates over the created list and prints out the names one by one on the console.
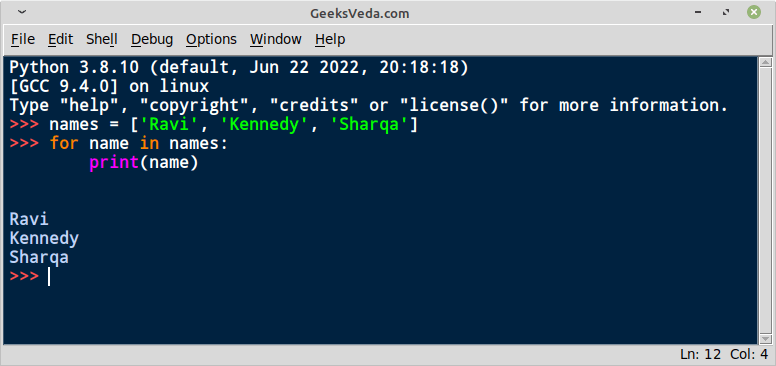
Example 2.2: Iterating over a Tuple With for loop
The for loop
applies the same functionality while iterating over the tuple.
ids = (5, 6, 7, 8, 9, 10) for id in ids: print(id)
According to the given code, the for loop
will iterate through the ids and print out each one by one on the console.
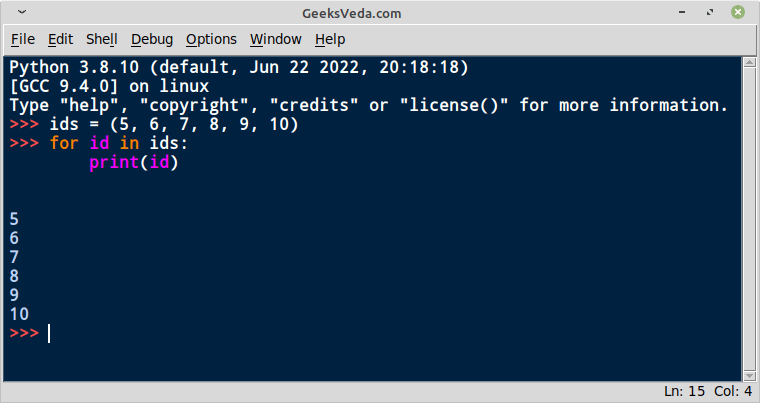
Example 2.3: Iterating Over a Dictionary With for loop
To examine the working of the for loop
with dictionaries, we have defined a dictionary named ids having three key-value pairs. Each key is a string representing a person’s name, and each value is an integer representing their ID. The dictionary contains three entries: Ravi: 5, Kennedy: 6, and Sharqa: 7.
In this example, the for loop
iterates through each item in the ids dictionary by utilizing the items()
method. For each item, the loop assigns the key to the name and the id to the variable. Then, it displays these values by utilizing the print()
function, separated by a space.
ids = {'Ravi': 5, 'Kennedy': 6, 'Sharqa': 7} for name, id in ids.items(): print(name, id)
So, for each iteration of the for loop
, the output will be a string with the person’s name followed by their id. For instance, the first iteration will output Ravi 5, the second iteration will output Kennedy 6, and the third iteration will display Sharqa 7.
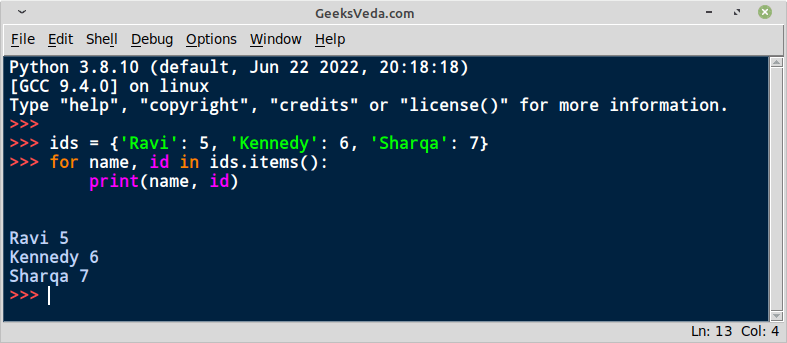
3. Use a for loop in Python With Nested loops
A nested loop is specified inside another loop. It can be used when it is required to iterate over multiple collections simultaneously. More specifically, in a nested loop, the inner loop executes to completion for each outer loop iteration.
For instance, in the below-given example, the outer for loop calls the range()
function to iterate from 1 up to 3, but not including, 3. This means that the loop will execute twice, with i
taking the values of 1 and 2.
The inner for loop utilized the range()
function to iterate from 1 up to, but not including, 5. This signifies that the loop will execute four times for each iteration of the outer loop, with j
taking the values of 1, 2, 3, and 4.
for i in range(1, 3): for j in range(1, 5): print(i, j)
During each iteration of the inner loop, the values of i
and j
are printed to the console with the help of the print()
function. This results in the following output.
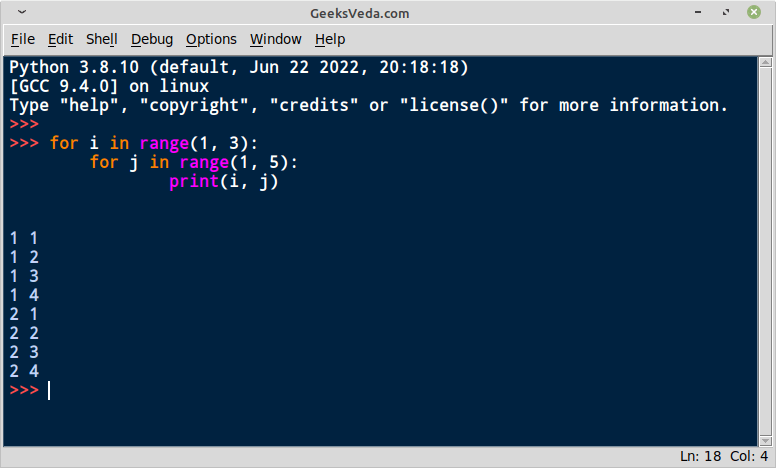
4. Use a for loop in Python With break and continue Statements
The break and continue statements can be used with a for loop
for controlling the flow. The break statement exits the loop completely. On the other hand, the continue statement skips to the next iteration.
Example 4.1: Using break Statement With for loop
In the given example, the for loop
iterates over each element in the names list using the keyword. During each iteration, the variable name accepts the current element value.
Inside the for loop, the added if
statement checks if the name variable value is equal to the string Sharqa. If this condition is true, the break statement will run, which immediately exits the loop and continues to the code specified after that.
names = ['Ravi', 'Kennedy', 'Sharqa'] for name in names: if name == 'Sharqa': break print(name)
If the specified condition is evaluated as false, the code inside the if
block is skipped, and the print()
function is invoked, which then prints the value of the name to the console.
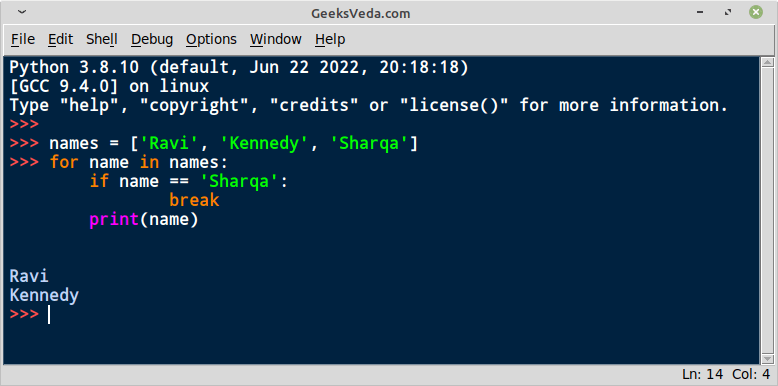
Example 4.2: Using continue Statement With for loop
Now, we will initialize a list of integer ids with six elements. The for loop
then performs the same iteration functionality as discussed earlier.
During each iteration, the variable id takes the current element value. Inside the for loop
, an if
statement is added to check if the value of id is an even number.
If this condition is true (i.e., if id is divisible by 2 with no remainder), the continue statement will get executed. This statement skips the remaining code in the loop and moves to the next iteration.
However, in case the if
condition is evaluated as false (i.e. if id is an odd number), the code inside the if
block is skipped, and the print()
function is invoked, which prints the value of id to the console.
ids = [5, 6, 7, 8, 9, 10] for id in ids: if id % 2 == 0: continue print(id)
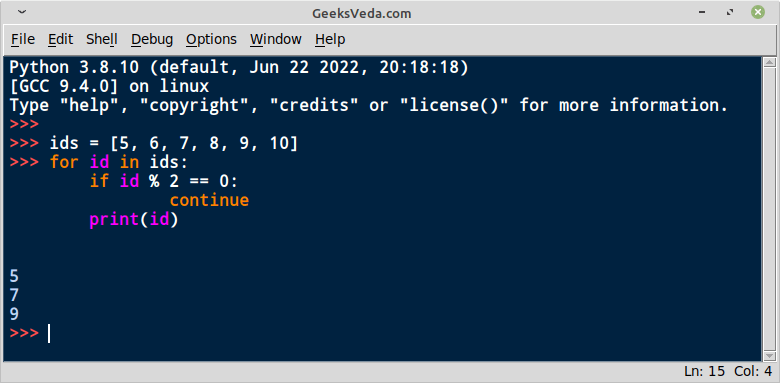
5. Use a for loop in Python With else Statement
The else statement in Python can be used with a for loop
for executing a block of code when the loop completes normally. If the loop is exited prematurely with a break statement, the else block will not run.
According to the provided code example, the for loop iterates over each element in the ids list using the in keyword. During each iteration, the variable takes the value likewise. Then, inside the for loop, the print()
function is called, which prints the value of id to the console.
After the loop completes all its iterations, the else block is executed, and the print()
function is invoked again, which displays the added message on the console.
ids = [5, 6, 7, 8, 9, 10] for id in ids: print(id) else: print("For loop has been completed.")
6. Use a for loop in Python With pass Statement
Sometimes, you may need to include a for loop
in your program, but not have any code to execute inside the loop. In such a scenario, utilize the pass statement to tell Python to do nothing and move on to the next iteration.
In this example, the for loop
starts iterating over the created list of names. For each iteration, it verifies if the current name matches the specified value, then do nothing. This functionality is achieved by adding the pass statement.
In the other case, if the added if
condition is evaluated as false, then the else
code block will execute, which prints the names on the console.
names = ['Ravi', 'Kennedy', 'Sharqa'] for name in names: if name == 'Sharqa': pass else: print(name)
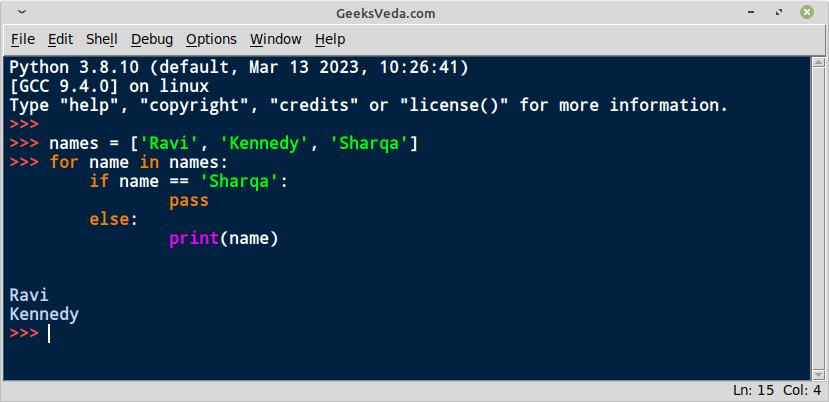
That was all about different use-case scenarios of for loop
in Python.
Conclusion
In Python, the for loop
can help you perform several tasks, from simple data manipulation to complex operations that involve multiple iterations.
Whether you’re a beginner or a professional developer, learning how to use the for loop
effectively can enhance your productivity and efficiency, and assist you in coding as well.
So keep experimenting, keep practicing, and do not be afraid to push the limits of what is possible with the for loop in Python!