Python strings
are the most crucial data type that can be utilized for representing and manipulating text in programs. They are also referred to as the sequences of characters enclosed in single or double quotes.
In this guide, we will discuss several aspects related to Python strings such as creating and accessing strings, built-in methods, manipulation techniques, formatting, and more.
So, let’s start understanding Python strings right away!
What is String in Python
Python strings
are referred to the sequences of the characters utilized for representing textual data. These characters can be enclosed in single quotes (''
) or double quotes (“”
).
More specifically, strings are immutable, which signifies that they cannot be changed after creation. Strings
in Python also support multiple methods and operations for transformation and manipulation.
Create and Access String in Python
This section will demonstrate the method for creating and accessing strings in Python. We will cover various operations such as checking the length of a string, validating the presence of a substring, accessing characters by index, and utilizing string slicing techniques.
1. Check the Length of a String
In order to determine the length of the defined string, invoke the built-in “len()
” function. This function outputs the Total number of characters a string comprises.
For instance, here, we have first created two strings “name
” and “message
“. Observe that the value of the “name
” string has been embedded in the “message
” string.
Then, we called the “len()
” function for checking the length of the message string. Lastly, the print() function will display the length on the console as output.
# Creating strings name = "GeeksVeda" message = 'Hello, ' + name + 'User!' length = len(message) print(" length:", length)
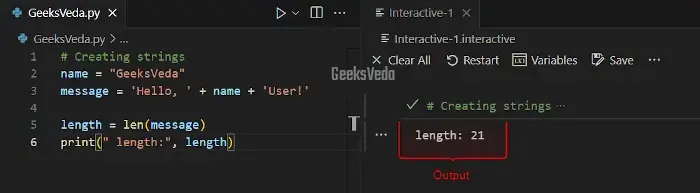
2. Validate the Presence of a Substring
The “in
” operator can be utilized for checking if a substring is present in a string or not. It outputs a boolean value, where “True
” indicates that the substring is present, and “False
” will be returned for the second case.
In this example, we will use the “in
” operator and verify if the “GeeksVeda
” substring is present in the “message
” string.
# Creating strings name = "GeeksVeda" message = 'Hello, ' + name + 'User!' is_present = 'GeeksVeda' in message print("GeeksVeda is present:", is_present)
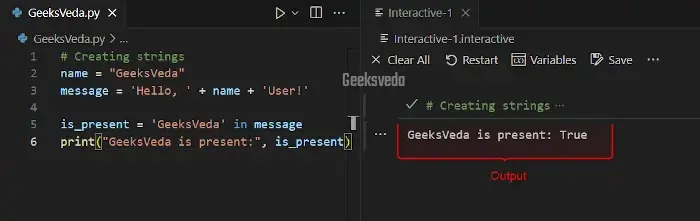
3. Access Characters of a String by Index
Individual characters of a string can be accessed by using the indexing technique. More specifically, Python utilizes zero-based indexing, where the first character is placed at the index 0.
Additionally, characters can be accessed with negative or positive indexing, where positive indexing starts from the string beginning and the negative starts from the ending side.
Here, we will access the first and last character of the “message
” string by specifying the indexes as “0
” and “-1
“, respectively.
# Creating strings name = "GeeksVeda" message = 'Hello, ' + name + 'User!' # Accessing characters first_char = message[0] last_char = message[-1] print("First Character:", first_char) print("Last Character:", last_char)
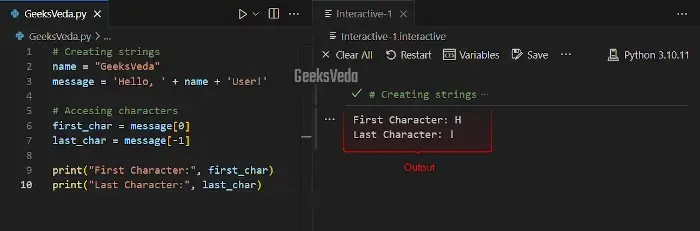
4. Extract a Substring from a String
Python slicing enables you to define a range of indices to access or fetch a portion of a string or substring.
For instance, we will now extract a substring from the “message
” string that starts from “7
” and ends at the “12
” index.
# Creating strings name = "GeeksVeda" message = 'Hello, ' + name + 'User!' # String Slicing substring = message[7:12] print("Substring:", substring)
It can be observed that the “Geeks
” substring has been extracted successfully.
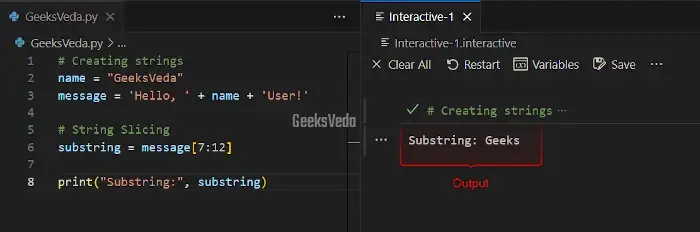
Python String Methods and Operations
Python offers built-in methods for manipulating strings. These methods allow you to perform different operations, such as changing the case to upper or lower case, splitting or joining strings, and formatting output.
Moreover, you can enhance the string manipulation capabilities in Python programs.
The below sub-sections will practically explain some of the majorly utilized methods and their relevant operations.
1. Using uppercase() Method
The “uppercase()
” Python method can be invoked for converting a string to uppercase. It outputs a new string with all characters in uppercase.
For instance, here, the “text
” has been converted into uppercase using the “uppercase()
” method.
text = "hello geeksveda user" uppercase = text.upper() print(uppercase)
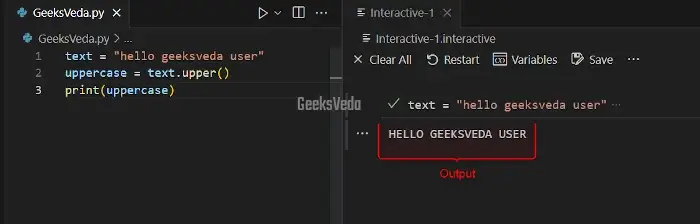
2. Using lowercase() Method
Alternatively, the “lowercase()
” can be called for converting the string with all its characters in the lowercase.
text = "HELLO GEEKSVEDA USER" lowercase = text.lower() print(lowercase)
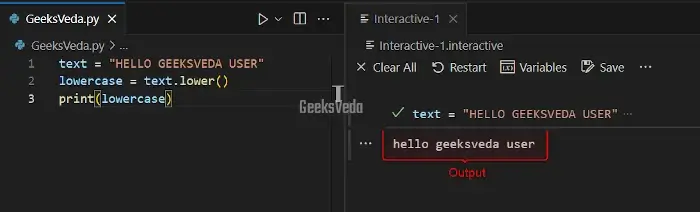
3. Using strip() Method
The “strip()
” method removes or deletes the leading (starting) and trailing (ending) whitespace characters from a string. It outputs a new string with the trimmed whitespaces.
For instance, in the provided code, the “strip()
” method will remove the whitespaces that are present at the start and end of the given string.
text = " Hello GeeksVeda User " trimmed = text.strip() print(trimmed)
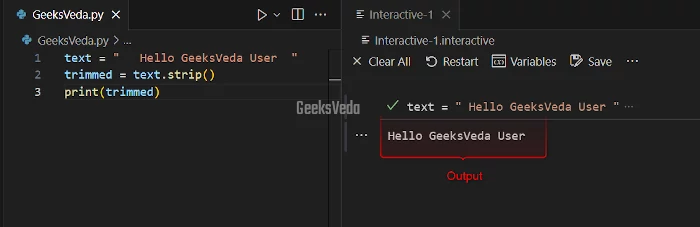
4. Using replace() Method
In order to replace occurrences of any specific existing substring with a new one, invoke the “replace()
” method. It outputs a new string with the new substring that is placed inside it.
Now, we will now replace the “Hello” substring with “Hi” with the “replace()
” method.
text = "Hello GeeksVeda User" replaced = text.replace("Hello", "Hi") print(replaced)
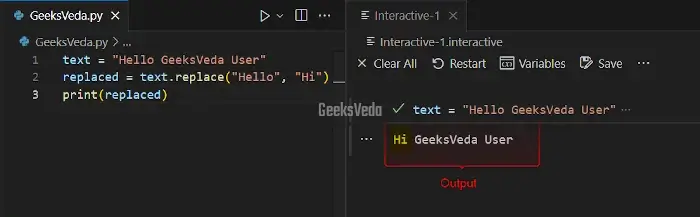
5. Using split() Method
The “split()
” method divides or splits a string into a list of substrings according to the specified separator. It outputs a list of substrings.
For instance, we will now call the “split()
” method and pass “,
” as the separator to split the text string value at the comma.
text = "Hello, GeeksVeda User" split_list = text.split(",") print(split_list)
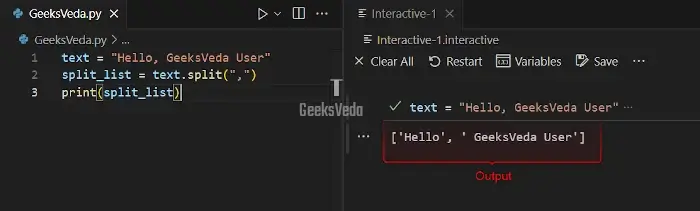
6. Using join() Method
In case, if you want to join a list of strings into a single string with the defined separator, utilize the “join()
” method. It outputs a new string.
Here, we have joined the strings “Hello
” and “GeeksVeda User
” with the hyphen “-
“.
list = "Hello", "GeeksVeda User" joined = "-".join(list) print(joined)
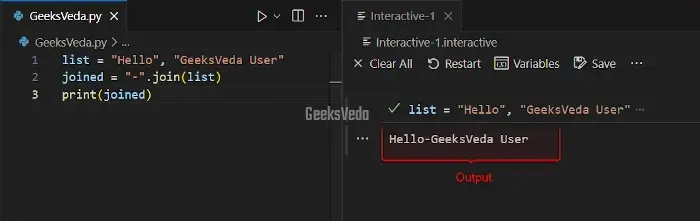
Now, let’s check out some operations that can be performed on Python Strings.
7. Concatenation Operation
In Python, concatenation is the process of combining or concatenating two or more strings to form a single string. More specifically, the “+
” operator can be used for the mentioned purpose.
# Concatenation of two strings str1 = "Hello, " str2 = "GeeksVeda User!" result = str1 + str2 print(result)
In the above example, we will concatenate the value of str1 with str2 using the “+
” operator.
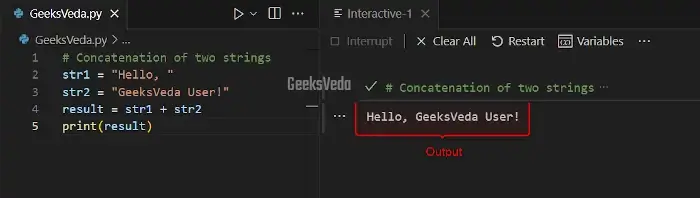
8. Repetition Operation
You can also repeat a string multiple times by utilizing the “*
” operator. For instance, in the provided example, we will repeat the str1 string 3 times.
# Repetition of a string str1 = "Hello! " repeated_str = str1 * 3 print(repeated_str)
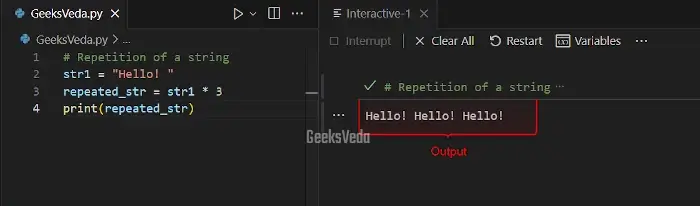
9. Membership Test Operation
Python Membership test can be used for verifying whether a substring is present within the defined string or not. Additionally, the “in
” operator is used for the specified functionality.
# Membership test text = "Hello, GeeksVeda User!" is_present = "GeeksVeda" in text print("GeeksVeda is present:", is_present)
Here, the “GeekVeda
” substring will be checked in the text string.
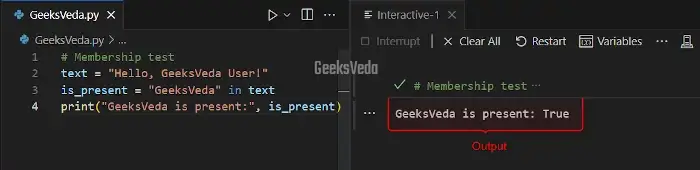
As you can see, the given substring has been found.
10. String Formatting Operation
String formatting approach can be utilized for constructing strings with dynamic content. It also permits incorporating variables and other values into a string.
Moreover, there exist multiple approaches for string formatting in Python including f-strings. For instance, in the given program, we have used f-strings for string formatting.
# String formatting using f-strings name = "Sharqa Hameed" age = 25 formatted_str = f"My name is {name} and I am {age} years old." print(formatted_str)
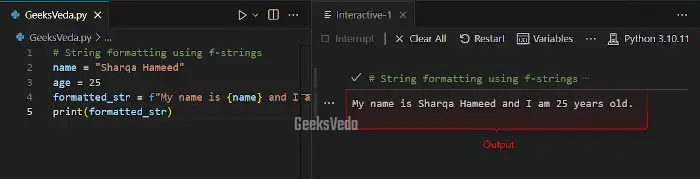
String Manipulation in Python
Searching and manipulating Python strings involves operations such as finding substrings, splitting strings, and replacing strings. These techniques permit efficient handling of the strings in Python.
1. Search Substring Using find() Method
The “find()
” method looks for a substring in the given string. It outputs the index of the first occurrence of the substring. Otherwise, it returns “-1
“, in case the substring is not found.
In the below-provided example, we will check the existence of the “GeeksVeda
” substring in the “text
” string.
text = "Hello, GeeksVeda User!" is_present = text.find("GeeksVeda") != -1 print("GeeksVeda is present:", is_present)
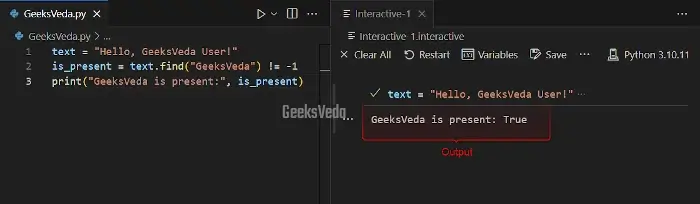
2. Check if a String Starts with a Specified Prefix
The “startsWith()
” method validates if the given string starts with the specified prefix or not and outputs a boolean value.
Here, we will use the “startsWith()
” method for checking if the “text
” string starts with the “Hello
” substring.
text = "Hello, GeeksVeda User!" starts_with_hello = text.startswith("Hello") print(starts_with_hello)
The returned “True
” value indicates that the value is present at the start of the given string.
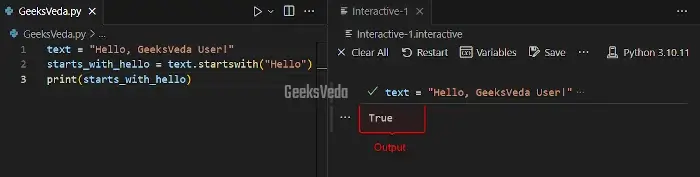
3. Find String Character Using Index
The “index()
” method can be utilized for getting the index of a single character or a complete substring. Let’s find the index of the “U
” character in the “text
” string.
text = "Hello, GeeksVeda User!" index = text.index("U") print(index)
The output signifies that the “U
” character has been found at index 17.
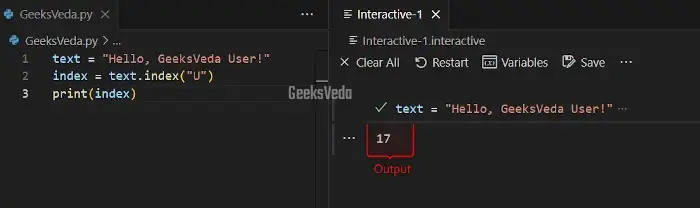
4. Reverse a String
“[::-1]
” slicing technique can be used for reversing a string. It outputs a new string with the characters in the reverse order. Here, we have specified the code for reversing the “text
” string.
text = "Hello, GeeksVeda User!" reversed_string = text[::-1] print(reversed_string)
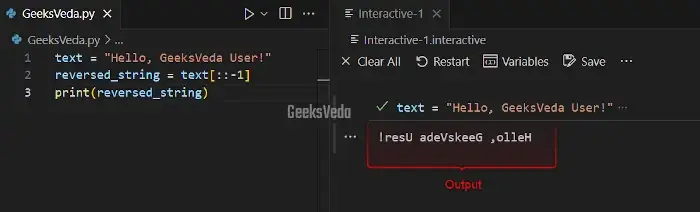
5. Pad a String with Whitespaces
For padding a string with whitespace characters and aligning it to the left, the “ljust()
” method can be invoked. It outputs a new string with the given length.
In the provided example, the “text
” string will be passed with whitespace with the width of “30
“.
text = "Hello, GeeksVeda User!" padded_string = text.ljust(30) print(padded_string)
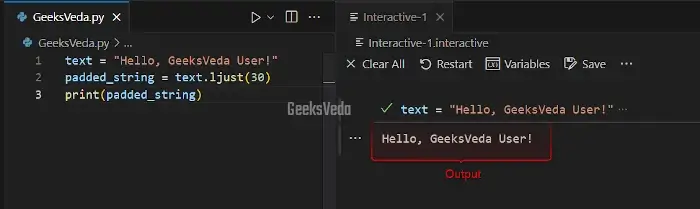
6. Truncate a String to Specified Length
You can also truncate a string to the given length with the slicing technique. It outputs a new string with the defined number of characters.
For instance, here, the “text
” string will be truncated to the first five characters.
text = "Hello, GeeksVeda User!" truncated_string = text[:5] print(truncated_string)
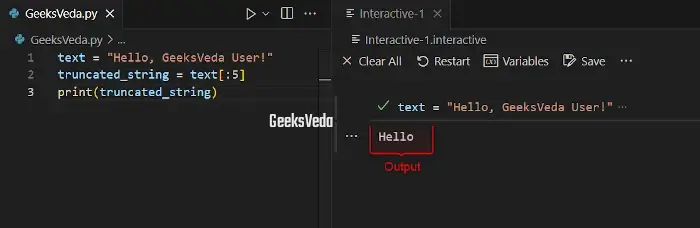
String Formatting and Templates in Python
Navigate to our “Formatting Strings
” guide in which we have thoroughly discussed old style and new style string formatting.
Moreover, we also demonstrated the common string formatting techniques like formatting numbers, dates, and time, handling precision and rounding, alignment and padding string, and other advanced string formatting approaches, such as using formatting specifiers and conversion.
Python String Encode and Decode Methods
In Python, strings can be encoded into different byte representations and decoded back into strings using the encode()
and decode()
methods. These methods are useful when working with string encodings, such as Unicode, ASCII, UTF-8, and others.
1. Encoding String With encode()
The encode()
method is used to convert a string into its corresponding encoded byte representation. More specifically, this method is utilized for encoding the “text
” string with “UTF-8
” encoding.
text = "Hello, GeeksVeda User!" encoded = text.encode("UTF-8") print("Encoded text:", encoded)
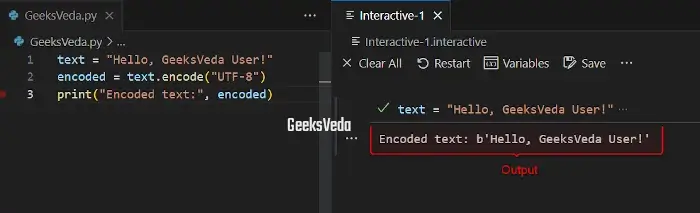
2. Decoding String With decode()
Likewise, decoding involves converting a string from a certain encoding format back to the original format. Here, the “decode()
” method is used for decoding the encoded string.
text = "Hello, GeeksVeda User!" encoded = text.encode("UTF-8") decoded = encoded.decode("UTF-8") print("Decoded Text:", decoded)
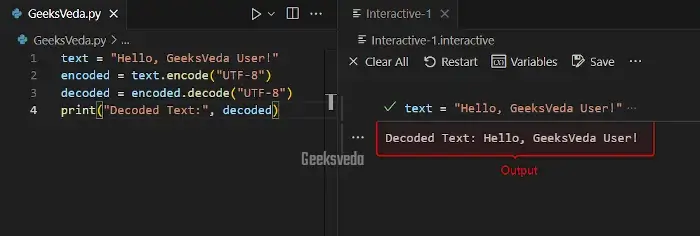
String Compare and Search Substring in Python
String comparison and searching in Python is based on comparing strings for equality and searching for substrings. These operations enable efficient manipulation and data retrieval within strings.
1. Compare String With the “==” Operator
You can easily compare strings with the “==
” operator. It outputs a boolean value that justifies whether strings are equal or not.
In the provided example, the “text
” string has been compared with the “Hello, GeeksVeda User!
” string.
text = "Hello, GeeksVeda User!" is_equal = text == "Hello, GeeksVeda User!" print(is_equal)
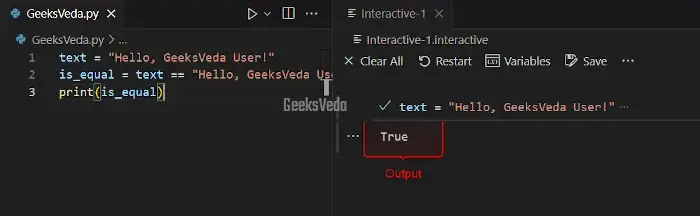
2. Find Substring With find() Method
Now, we will invoke the “find()
” method for finding the index of the “GeekVeda
” substring within the “text
” substring. This method will output the index of the first occurrence of the specified substring.
text = "Hello, GeeksVeda User!" index = text.find("GeeksVeda") print(index)
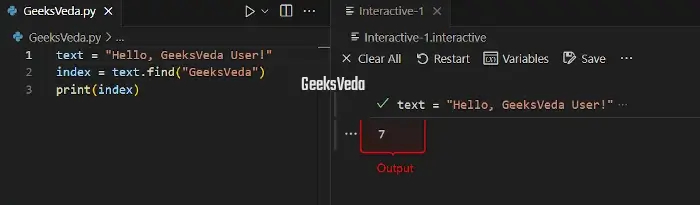
Iterate and Enumerate String in Python
In Python, strings are iterable objects, which means you can iterate over each character or element in a string.
Additionally, the enumerate() function provides a convenient way to iterate over both the characters and their corresponding indices in a string. This guide will explain how to iterate and enumerate strings in Python.
1. Iterate String with for loop
To iterate over a string, you can use a for loop or a list comprehension to access each character in the string individually. Python for loop permits you to iterate the string and access each character of it.
For instance, in the given example, we have added the for loop for getting each character of the “text
” string and printing it on the console.
text = "Hello, GeeksVeda User!" for char in text: print(char)
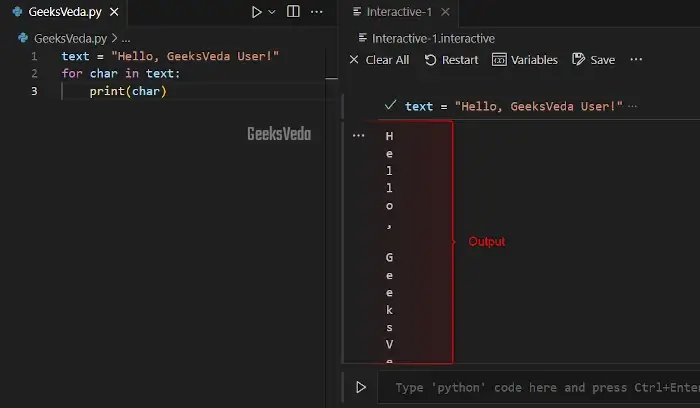
2. Enumerate String with enumerate() Function
The “enumerate()
” function can be also called for iterating over a string and fetching both the character and its relevant index.
For instance, here the characters of the “text
” string have been displayed along with their corresponding indexes.
text = "Hello, GeeksVeda User!" for index, char in enumerate(text): print(f"Character '{char}' at position {index}")
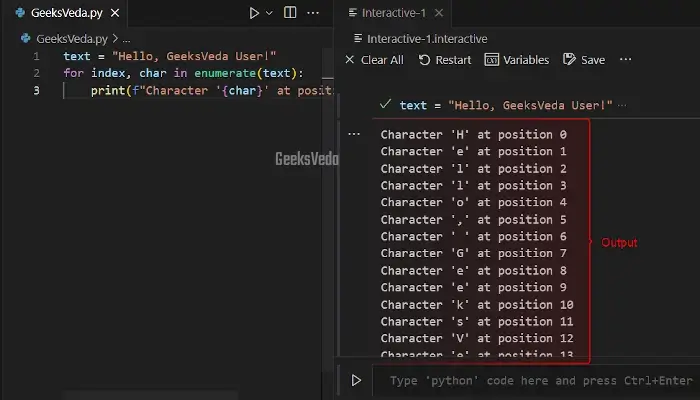
That was all from this effective guide related to Python strings.
Conclusion
Python strings provide a wide range of functionalities for working with text data. From creating and manipulating strings to comparing and searching substrings, checking the various methods and operations available permits effective text processing.
So, master the usage of Python strings and handle the programming challenges effectively.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!