Whether you are working with lists, strings, tuples, or another iterable object in Python, the “enumerate()
” function offers an efficient way for enhancing the iteration process. It is a robust tool that simplifies the task of iterating over elements in the sequence.
Moreover, it also keeps track of the relevant indices. So let’s dive in and check out how Python enumerate() function works and discuss its use cases.
What is Enumerate () Function in Python
The enumerate()
python function is a built-in function that adds counter values to the defined iterable object and outputs an enumerate object. More specifically, it enables you to iterate over the iterable elements while keeping track of the position or index of each element.
Here is the syntax to utilize the enumerate() function in Python
enumerate(iterable, start=0)
According to the given syntax:
- The
enumerate()
function accepts an “iterable
” object as the first argument. - “
start
” is an optional parameter that has “0
” as its default value. - The
enumerate()
function returns an enumerate object that comprises tuples with two elements, the index and its corresponding value in the iterable.
Now, let’s head toward some practical examples.
Using Python enumerate() With Strings
A string is referred to as the sequence of characters that are embedded in single or double quotes. Using the “enumerate()
” function with strings permits you to iterate over each character along with its respective index.
Check out an example of the discussed scenario. To do so, we will create a string with the value “GeeksVeda
“. Next, the enumerate()
function will iterate over each character in the newly created string “using the for loop“.
Within the for loop, the print()function will display the index and the relevant character on each iteration.
string = "GeeksVeda" for index, char in enumerate(string): print("Character:", char, "Index:", index)
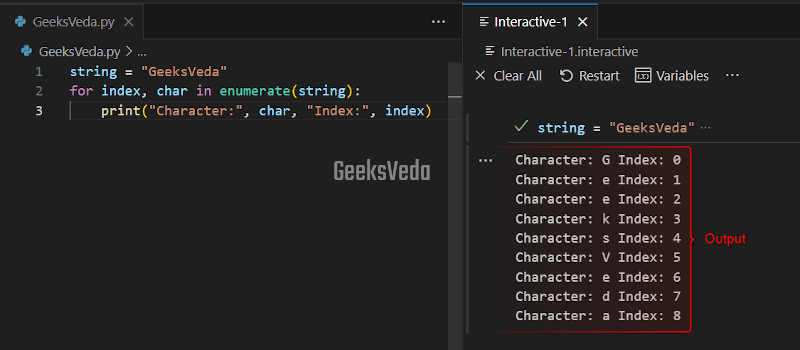
Using Python enumerate() With Tuple
A Python tuple is known as an immutable sequence of elements that are enclosed in parentheses. When the “enumerate()
” function is invoked with tuples, it enables you to access both the index and the value of each element.
Now, let’s create a tuple named “tuple1
” and add three elements to it. After that, the enumerate()
function is called for iterating over the tuple elements. The added print()
function will then show the resultant value on the terminal.
tuple1 = ("blue", "black", "yellow") for index, element in enumerate(tuple1): print("Element:", element, "Index:", index)
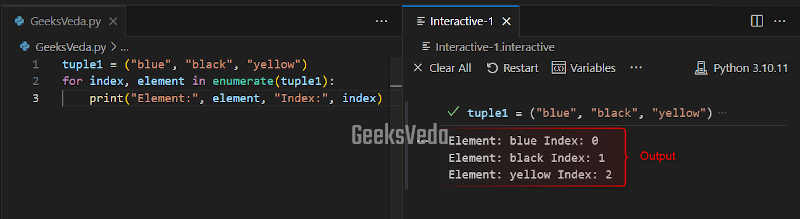
Using Python enumerate() With a List
In Python, a List is a mutable sequence of elements enclosed in square brackets. More specifically, when the “enumerate()
” function is utilized with the list, you can access both the index and the corresponding value of each element.
For instance, we have created a list named “list1
” and added 5 items to it. After that, enumerate()
function is invoked with the for loop for iterating over the elements in the created list. Then, the index and the value for each iteration are displayed using the “print()
” function.
list1 = [11, 33, 55, 77, 99] for index, value in enumerate(list1): print("Value:", value, "Index:", index)
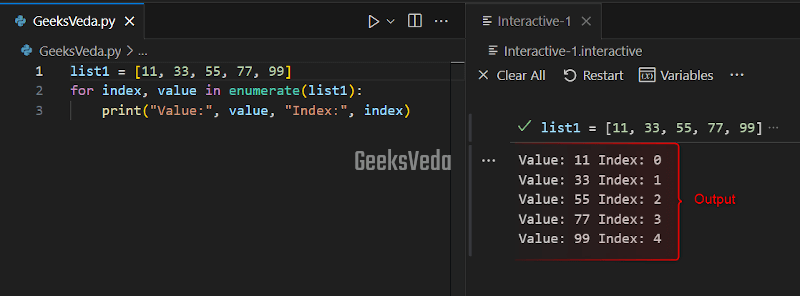
Using Python enumerate() With Dictionary
A Python dictionary is an unordered collection of key pairs that are placed in curly braces. So, when the “enumerate()
” function is invoked with dictionaries, you can iterate over the keys along with their relative values.
In the given code, the enumerate()
function is called for iterating over the keys in the dictionary named “dict1
“. The “for
” loop is defined to perform the iteration. Within this loop, the added print()
function will show the key, value, and the corresponding index of the key-value pairs on the console.
dict1 = {"x": 7, "y": 8, "z": 9} for index, key in enumerate(dict1): print("Key:", key, "Value:", dict1[key], "Index:", index)
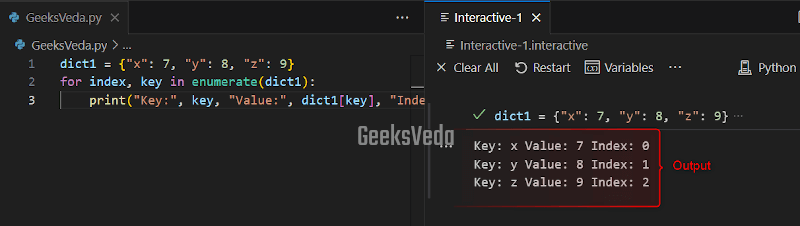
Using Python enumerate() With Nested Data Structures
In Python, Nested data structures comprise other data structures within them. Using these structures with the enumerate()
function enables you to access elements at multiple levels and perform multiple operations on them based on their positions.
For instance, here the enumerate()
function is utilized for over the list of dictionaries named “data
“. With the help of the added “for
” loop and the “print()
” function, the index, and the author (dictionary) are displayed in each iteration.
data = [{"name": "Sharqa", "age": 25}, {"name": "Ravi", "age": 30}] for index, person in enumerate(data): print("Author:", person, "Index:", index)
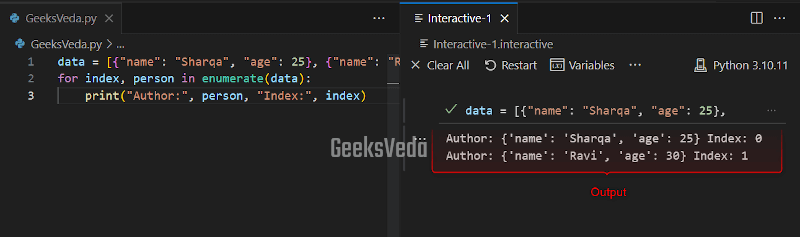
How to Loop Over an Enumerate Object in Python
You can also create an enumerate object in Python with the help of the enumerate()
function. After doing so, iterate through its item using the “for
” loop and fetch the index and the corresponding value in the sequence.
Now, we will call the enumerate()
function utilized for creating an enumerated object from the “colors
” list. Then, the for loops iterate over the enumerated object. In each iteration, the index and the related color value will be displayed, which provides the position and value of each color in the list.
colors = ["black", "red", "pink"] enum_colors = enumerate(colors) for index, color in enum_colors: print("Index:", index, "Color:", color)
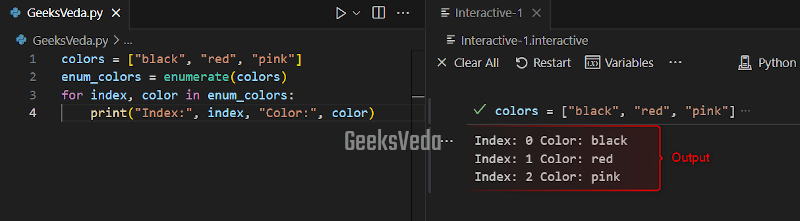
Advantages of Using Python enumerate() Function
Here is the list of advantages of utilizing enumerate() function in Python.
- Eliminates the need for manual index tracking.
- Simplifies iteration by offering index-value pairs in a single step.
- Offers easy access to positional information.
- Enhances productivity and reduces bugs.
- Supports more expressive coding patterns.
That’s all from this essential guide regarding using Python enumerate()
function.
Conclusion
Python enumerate()
function is a useful tool for iterating over objects with an index. This method improves readability and simplifies the code, which enables the developers to write more elegant and efficient solutions. Moreover, by using enumerate()
function, you can reduce errors and enhance productivity in several programming tasks.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!