Today’s guide is all about implementing the switch statement in Python. Although Python does not support the native switch statement, there exist several approaches that can help you to achieve the mentioned functionality.
So, get ready to explore the dictionary mapping, function mapping, and the new “match
” and “case
” keywords for handling different use cases efficiently.
These approaches will also assist in enhancing the maintainability and readability of your Python code.
Why Use Switch Statement in Python
The Python “switch
” statement can be utilized in scenarios where it is required to evaluate multiple conditions and you have to perform different operations based on the specified conditions.
So, as the number of conditions increases, it becomes difficult for the traditional “if-elif-else
” structure to handle, and ultimately code becomes less readable.
Therefore, in such scenarios, the switch-like behavior offers a more elegant and concise way of handling such situations.
Example: Menu Selection with “if-elif-else” Statement
For instance, one common scenario is menu selection. In this case, the user has to input an option from different available options. Resultantly, different operations or actions are specified based on the selection of the users.
The “if-elif-else
” statement can be utilized in this case. However, with the increased number of options, the code will become less readable.
option = input("Enter any option (1-3): ") if option == "1": print("Option 1 has been selected.") elif option == "2": print("Option 2 has been selected.") elif option == "3": print("Option 3 has been selected.") else: print("You have selected an Invalid option.")
Let’s enter “1
” as the selected option and check out the output.
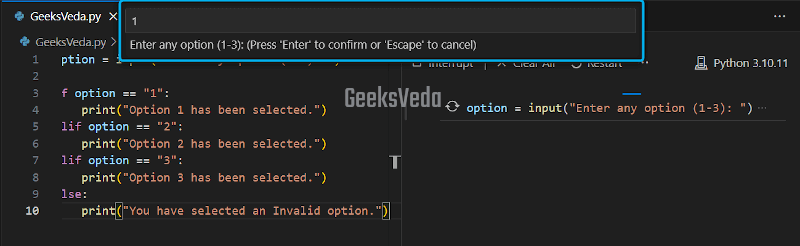
It can be observed that the program is working. However, the switch-like solution will offer a more concise and efficient way of handling such use cases.
How to Use Switch Statements in Python
Python does not support the native “switch
” statement, but there exist alternative approaches that can assist in achieving the same functionality. For example.
- Dictionary Mapping
- Function Mapping
- Use of “
match
” and “case
” Keywords
Head towards the next section to check them out individually.
How to Use Dictionary Mapping in Python
Dictionary mapping refers to the process of utilizing a dictionary for mapping particular conditions or values to respective functions or actions.
In the dictionary, each key-value pair represents a case, where the key indicates the value or condition that needs to be matched and the value is the function or action that is required to perform. More specifically, this approach can be used for achieving the switch-like functionality in Python.
In the given program, we have defined three functions named “option1()
“, “option2()
“, and “option3()
“. These functions represent the actions for each case.
After that, we created an “actions
” dictionary, where the keys are the user input or conditions and the values are the related functions to run.
The “get()
” of the Python dictionary fetches the value associated with the user input. According to the condition evaluation, if the user input matched one of the keys, the specified function will be invoked.
Otherwise, the method will output “None
” and the defined message will be displayed using the “print()” function.
def option1(): print("Option 1 has been selected.") def option2(): print("Option 2 has been selected.") def option3(): print("Option 3 has been selected.") choice = input("Enter your choice (1-3): ") actions = { "1": option1, "2": option2, "3": option3 } selected_action = actions.get(choice) if selected_action: selected_action() else: print("You have selected an Invalid choice.")
As you can see that the dictionary mapping has been performed successfully.
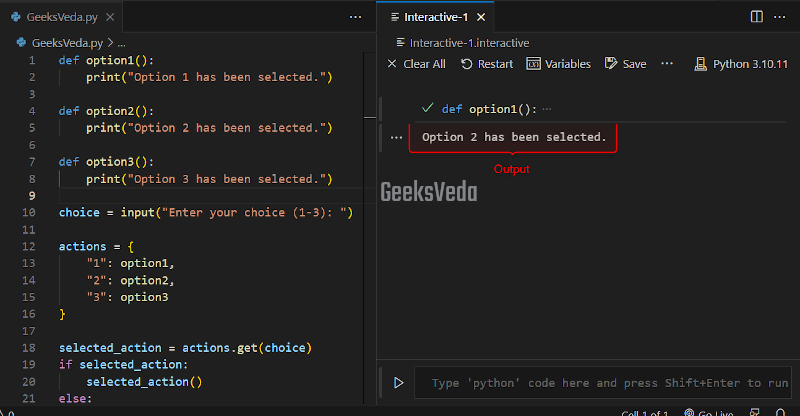
Benefits of Using Dictionary Mapping in Python
Here, we have gathered the major benefits of utilizing the Dictionary mapping technique.
- Offers a scalable and flexible solution for implementing switch-like functionality.
- Provides a concise code and clean structure.
- Permits you to easily manage and define the mapping between actions and conditions.
How to Use Dispatch Decorator in Python
In Python, function dispatching can be also used for applying the switch-like functionality. For this purpose, you have to define a separate function for each case and utilize a dispatching approach for selecting and running the appropriate function based on the condition.
First of all, we will define the separate functions “option1()
“, “option2()
“, and “option3()
” for representing the actions for each case.
After that, the “dispatch()
” has been created that accepts the choice of the user as input and utilizes a dictionary for mapping the option to the relevant function.
If the option matches one of the dictionary keys, the corresponding function will run. Otherwise, the given message will be displayed.
def option1(): print("Option 1 has been selected.") def option2(): print("Option 2 has been selected.") def option3(): print("Option 3 has been selected.") def dispatch(choice): actions = { "1": option1, "2": option2, "3": option3 } selected_action = actions.get(choice) if selected_action: selected_action() else: print("Invalid choice.") user_choice = input("Enter your choice (1-3): ") dispatch(user_choice)
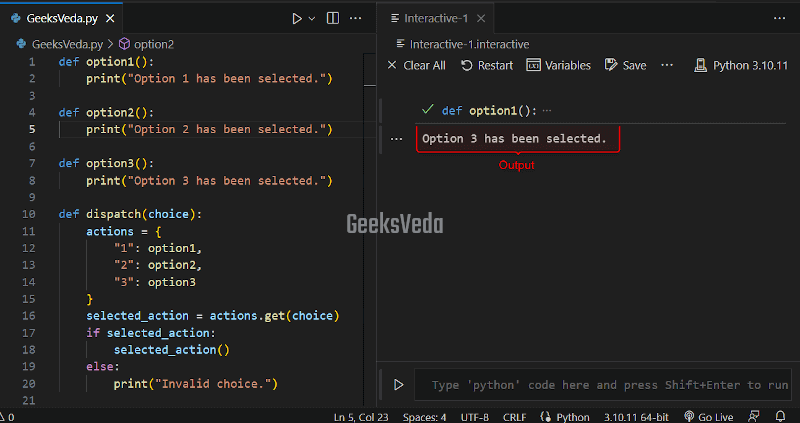
Benefits of Using Function Dispatching in Python
Have a look at the stated benefits of utilizing function dispatching in Python.
- Permits maintainable and modular code by separating the actions into individual functions.
- Enables easy modification or addition of cases without modifying the dispatching logic.
- Provide a clear separation of concerns.
How to Use “match” and “case” Statements
Python 3.10 introduced the “match
” and “case
” keywords as a feature for providing the switch-like functionality, It permits you to match a value according to the defined multiple patterns and run the corresponding code or block based on the evaluation.
In the provided example, we have used the “match
” statement for matching the “option
” value against different cases, Here, the “case
” keyword is followed by a pattern, which is strings “1
“, “2
“, and “3
“.
In case, if the value matched one of the patterns, the respective code block will execute. Note that the “_
” pattern works as a wildcard for matching any value.
def process_option(option): match option: case "1": print("Option 1 has been selected.") case "2": print("Option 2 has been selected.") case "3": print("Option 3 has been selected.") case _: print("Invalid choice.") user_choice = input("Enter your choice (1-3): ") process_option(user_choice)
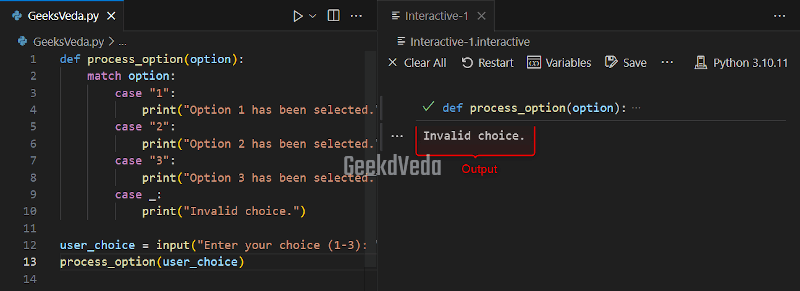
Benefits of Using “match” and “case” Keywords in Python
Some of the major benefits of utilizing the “match
” and “case
” keywords are given below.
- Promotes a more declarative programming style.
- Improves code readability.
- Provide a concise and intuitive way for implementing switch-like functionality.
That’s all from an informative guide related to implementing switch statements in Python.
Conclusion
Python provides several techniques for applying the switch-statement in Python, like dictionary mapping, function dispatching, and the new “match” and “case” statements.
By using these approaches and following the stated best practices, you can efficiently handle multiple cases and make the code more maintainable and readable.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!