Python sets are essential data structures that offer unique elements collection. These data structures support robust operations like union, intersection, and difference. This ultimately enables you to manipulate data and analyze it efficiently.
Additionally, with their concise syntax and built-in methods and functions, sets are considered valuable tools for handling computational tasks.
In today’s guide, we will explore Python sets, their usage, basic operations, built-in methods and functions, and the approaches to iterate over the set elements.
So, let’s begin this journey of learning something new!
What are sets in Python
Sets
in Python are the unordered collection of unique elements. They are usually represented by curly braces.
Moreover, Python sets support multiple methods for data manipulation, such as finding unions, intersections, differences, and symmetric differences.
You can also utilize sets to eliminate duplicates, solve complex problems, or perform set operations involving unique elements or data filtering.
Create and Initialize Sets in Python
For the purpose of creating a set
in Python, utilizing the curly braces “{}
” or the built-in “set()
” function.
This section will provide examples of creating an empty set and creating and initializing a Python set using a simple approach and with the set()
function.
1. Create an Empty Set
First of all, let’s create an empty Python set.
# Creating an empty set empty_set = set() print("Empty Set:", empty_set)
In this case, the print() function will display nothing on the console.
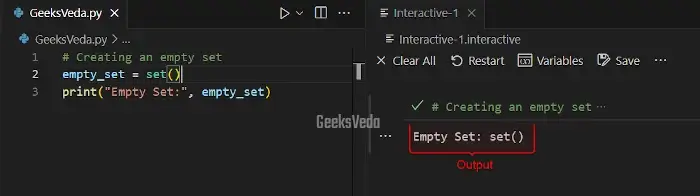
2. Create a Set with Elements
Here, we are creating a “fruits
” set having the following five elements.
# Creating a set with elements fruits = {'apple', 'banana', 'orange', 'apple', 'pear'} print("Fruits Set:", fruits)
As you can see, the print()
function shows the names of the fruits as set elements on the console.
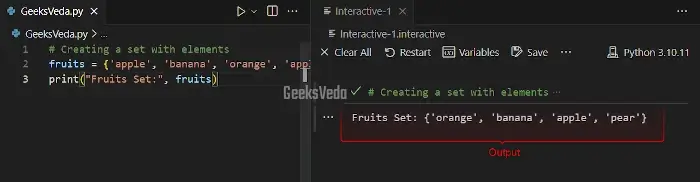
3. Create a Set With set() Function
In this example, we have invoked the “set()
” function for creating and initializing a Python set named “colors
“.
# Using the set() function colors = set(['red', 'blue', 'green', 'blue', 'yellow']) print("Colors Set:", colors)
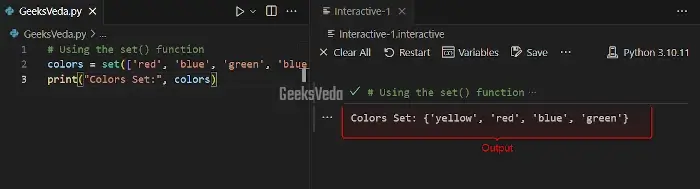
Remember that, While creating a set, all duplicated elements are automatically removed, which makes sure that all set elements are unique.
Basic Operations of Sets in Python
Python sets
offer multiple operations which ultimately makes them highly valued for data analysis and manipulation.
For instance, some of the common set operations are:
Operation | Symbol | Description |
Union | “| “ |
It combines elements from two Python sets without adding duplicates. |
Intersection | “& “ |
It finds the common elements between two defined sets. |
Difference | “- “ |
It fetches elements from one set that are not present in the other set. |
Symmetric Difference | “^ “ |
It fetches the elements that are uncommon in both sets. |
We will now practically observe each operation with separate examples.
1. Union
In this example, the union operation has been performed on “set_A
” and “set_B
” using the “|
” symbol.
Resultantly, the print()
function will display elements of both sets as “union_set
“.
set_A = {1, 2, 3, 4} set_B = {3, 4, 5, 6} # Union union_set = set_A | set_B print("Union Set:", union_set)
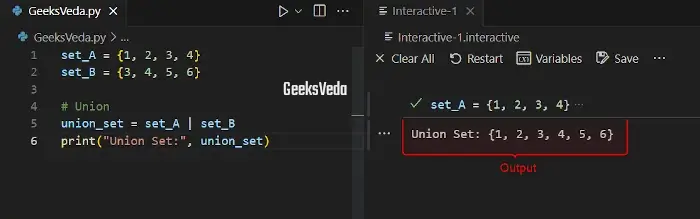
2. Intersection
Now, let’s find the common elements of “set_A
” and “set_B
“.
set_A = {1, 2, 3, 4} set_B = {3, 4, 5, 6} # Intersection intersection_set = set_A & set_B print("Intersection Set", intersection_set)
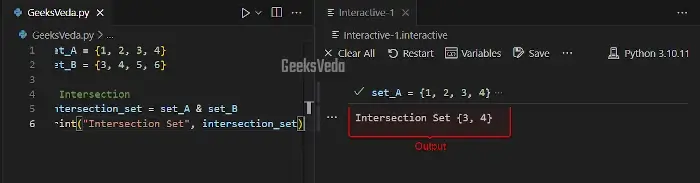
3. Difference
In this example, we will perform the difference set operation to retrieve the elements that are in “set_A
” but not in “set_B
“.
set_A = {1, 2, 3, 4} set_B = {3, 4, 5, 6} # Difference difference_set = set_A - set_B print("Difference Set:", difference_set)
It can be observed that 1 and 2 have not been found in the “set_B
“.
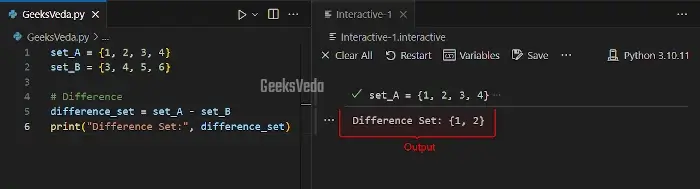
4. Symmetric Difference
In this example, we will find the uncommon elements from both “set_A
” and “set_B
“.
set_A = {1, 2, 3, 4} set_B = {3, 4, 5, 6} # Symmetric Difference symmetric_diff_set = set_A ^ set_B print("Symmetric Difference Set:", symmetric_diff_set)
Check out the elements that are in either of the sets.
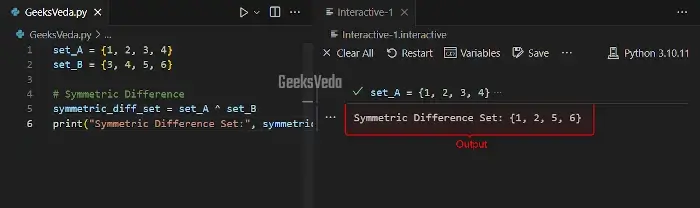
Modify Sets in Python
Modifying sets refers to the concept of adding or removing elements from the set. To perform such kinds of operations, you can use any of the given methods.
Add Elements to Python Sets | |
add() | It can be utilized for adding a single element to the set. |
update() | It can be utilized for adding multiple elements to the set. |
Remove Element from Python Sets | |
remove() | It removes a certain element from the set. Else, raise a “KeyError ” in case the element is not present. |
discard() | It removes a particular element from the set. However, it does not raise “KeyError ” if the element does not exist. |
pop() | It removes an arbitrary element from the defined set. |
1. Add a Single Element Using add() Method
In the following example, firstly, we have initialized a set named “programming_languages
” having three elements. After that, the “add()
” method has been invoked for adding a “JavaScript
” element to the defined set.
# Initializing a set programming_languages = {'Python', 'Java', 'C++'} # Adding an element using add() programming_languages.add('JavaScript') print(programming_languages)
As you can see, the specified element has been added successfully.
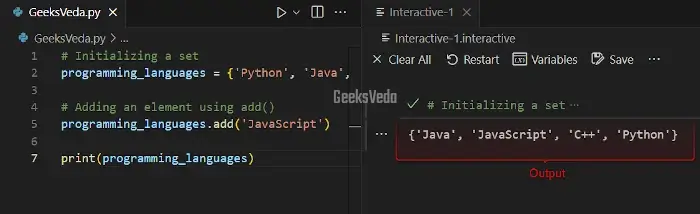
2. Add Multiple Elements Using update() Method
Now, we will try to add two elements in the same set using the “update()” method as follows.
# Initializing a set programming_languages = {'Python', 'Java', 'C++'} # Adding multiple elements using update() programming_languages.update(['Ruby', 'Swift']) print(programming_languages)
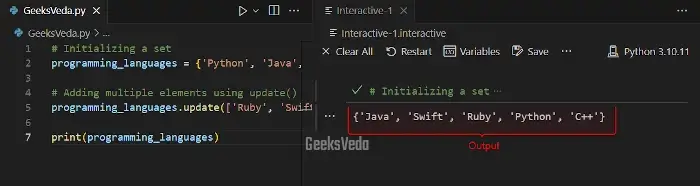
3. Remove an Element Using remove() Method
In order to remove an element from the “programming_languages” Python set, we will invoke the “remove()
” method and pass “Java
” as its argument.
# Initializing a set programming_languages = {'Python', 'Java', 'C++'} # Removing an element using remove() programming_languages.remove('Java') print(programming_languages)
Resultantly, “Java
” will be removed from the given set.
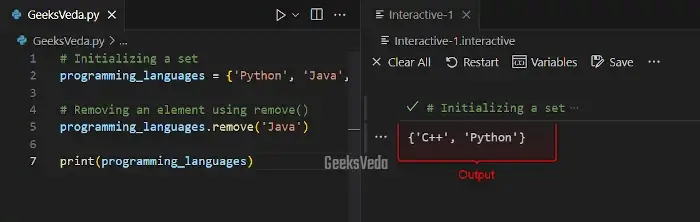
4. Remove an Element Using discard() Method
Now, we will attempt to remove “C#
” from the “programming_languages
“.
# Initializing a set programming_languages = {'Python', 'Java', 'C++'} # Removing an element using discard() programming_languages.discard('C#') print(programming_languages)
This code will do nothing since “C#
” is not present in the “programming_languages
“.
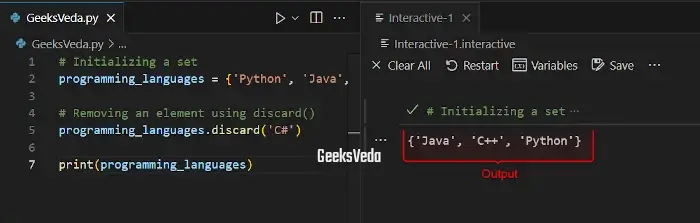
5. Remove Arbitrary Element Using pop() Method
The last method is to utilize the “pop()
” method for removing an arbitrary element which will be then displayed on the console.
# Initializing a set programming_languages = {'Python', 'Java', 'C++'} # Removing an arbitrary element using pop() removed_element = programming_languages.pop() print(programming_languages)
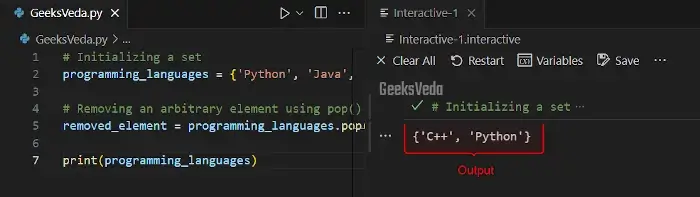
Built-in Functions and Methods of Sets in Python
Here is the table comprising the primarily utilized Python methods and functions.
Method/Function | Description |
copy() | Creates a shallow copy of the set. |
union() | Outputs the union of two or more given sets. |
intersection() | Outputs the intersection of two or more given sets. |
difference() | Outputs the difference between two defined sets. |
symmetric_difference() | Outputs the symmetric difference of two sets. |
issubset() | Verifies if a set is a subset of another set. |
issuperset() | Validates if a set is a superset of another set. |
clear() | Removes or deletes all elements in the set. |
len() | Outputs the total number of elements in the given set. |
max() | Outputs the maximum value in the set. |
min() | Outputs the minimum value in the set. |
sum() | Outputs the sum of all elements in the given set. |
1. Using copy() Method
As the first example, we will create a shallow copy of the "set_a
” with the copy()
method and save it in “set_c
“. Then, the print() function will display the copied set as output.
# Initializing a set set_a = {1, 2, 3, 4} # Using set method: copy() set_c = set_a.copy() print(set_c)
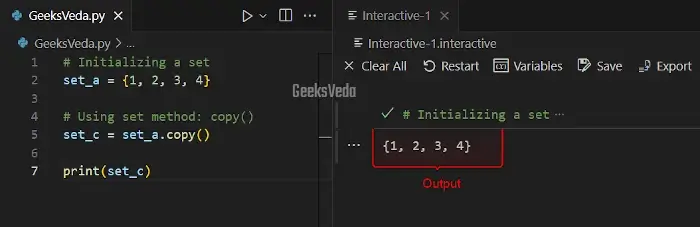
2. Using union() Method
Now, let’s combine ‘set_a
” and “set_b
” using the “union()
” method for creating a new set “set_d
” that comprises all unique elements.
# Initializing sets set_a = {1, 2, 3, 4} set_b = {3, 4, 5, 6} # Using set method: union() set_d = set_a.union(set_b) print(set_d)
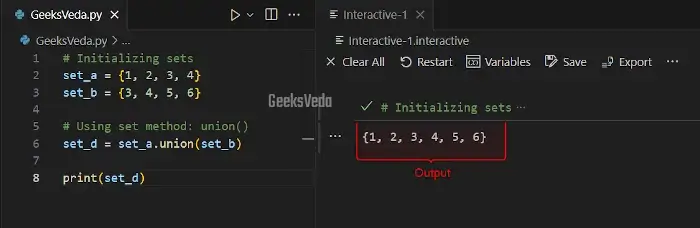
3. Using intersection() Method
In order to find the common elements between “set_a
” and “set_b
“, invoke the intersection() method and store the resultant set in “set_g
“.
# Initializing sets set_a = {1, 2, 3, 4} set_b = {3, 4, 5, 6} # Using set method: intersection() set_g = set_a.intersection(set_b) print(set_g)
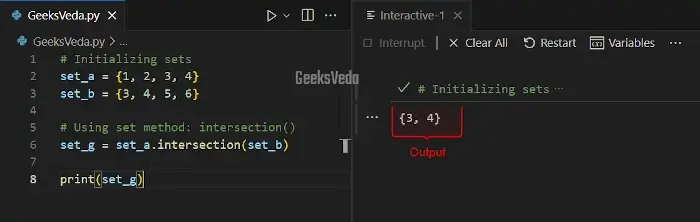
4. Using difference() Method
Here, we are using the difference()
method for finding the elements that are in “set_a
” but not in “set_b
“.
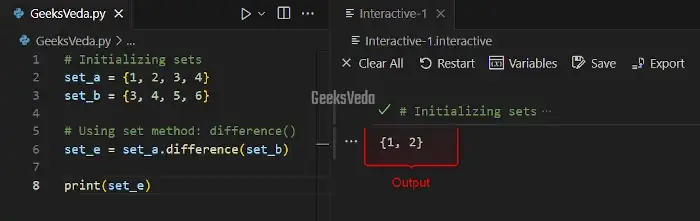
5. Using symmetric_difference() Method
Utilize the "symmetric_difference()
” method for finding the elements that are in either “set_a
” or “set_b
“, but not in both.
# Initializing sets set_a = {1, 2, 3} set_b = {3, 4, 5} # Using set method: symmetric_difference() set_i = set_a.symmetric_difference(set_b) print(set_i)
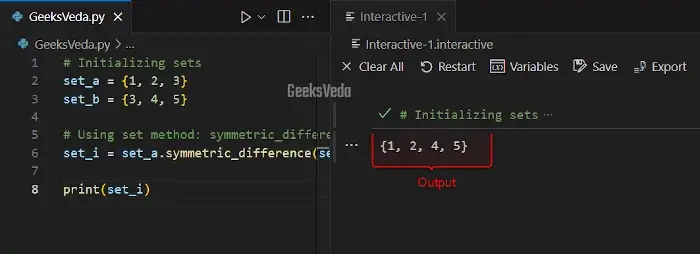
6. Using issubset() Method
The issubset()
method can be invoked for verifying if the “set_a
” is the subset of “set_b
“. This method will output a boolean value based on the evaluation.
# Initializing sets set_a = {1, 2, 3} set_b = {1, 2, 3, 4, 5} # Using set method: issubset() is_subset = set_a.issubset(set_b) print(is_subset)
It can be observed that “set_a
” is the subset of “set_b
“.
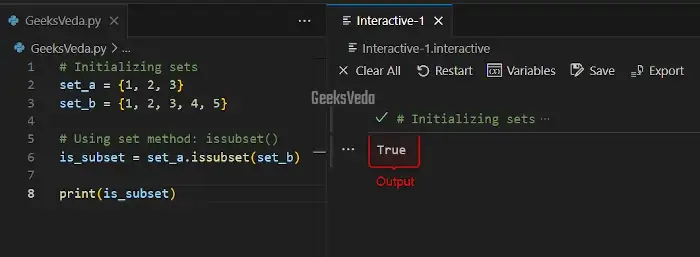
7. Using issuperset() Method
Here, the "issuperset()
” is called for checking if the “set_a
” is the superset of “set_b
“.
# Initializing sets set_a = {1, 2, 3, 4, 5} set_b = {1, 2, 3} # Using set method: issuperset() is_superset = set_a.issuperset(set_b) print(is_superset)
the “True
” value indicates that “set_a
” is the superset of “set_b
“.
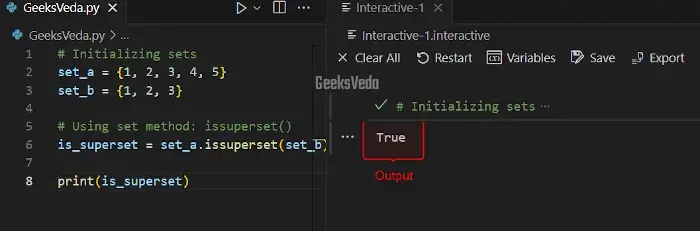
8. Using clear() Method
In order to clear all elements from the “set_a
“, we will use the “clear()
” method as follows.
# Initializing a set set_a = {1, 2, 3, 4} # Using set method: clear() set_a.clear() print(set_a)
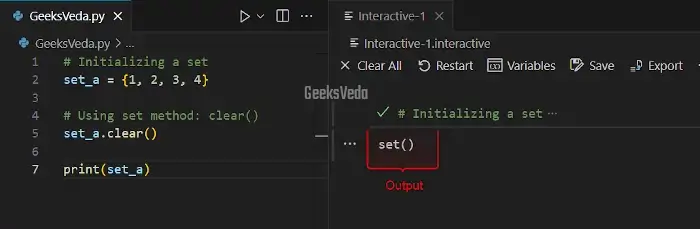
9. Using len() Function
Want to find the total number of elements in “set_a
“? Call the “len()
” function and store it in the relevant variable as we stored here in “num_elements_in_set_c
“.
# Initializing a set set_a = {1, 2, 3, 4} # Using built-in function: len() num_elements_in_set_c = len(set_a) print(num_elements_in_set_c)
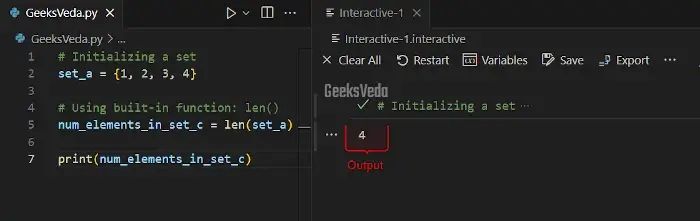
10. Using max() Function
The “max()
” function is used in the below-provided code for finding the maximum value in the “set_a
“.
# Initializing a set set_a = {1, 2, 3, 4} # Using built-in functions: max() max_value_in_set_d = max(set_a) print(max_value_in_set_d)
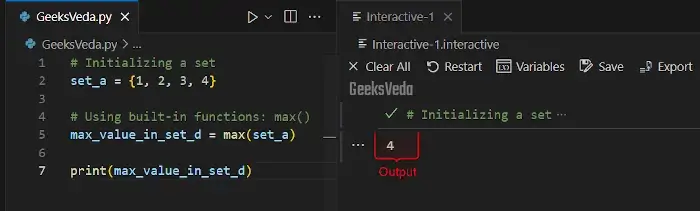
11. Using min() Function
Likewise, invoke the “min()
” function for finding the minimum value from the “set_a
“.
# Initializing a set set_a = {1, 2, 3, 4} # Using built-in functions: min() min_value_in_set_d = min(set_a) print(min_value_in_set_d)
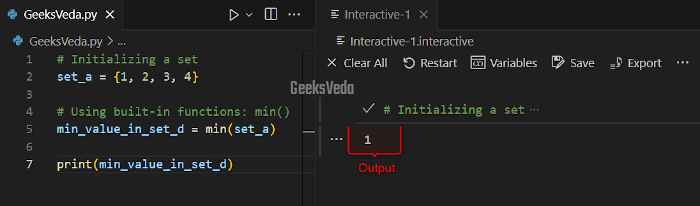
12. Using sum() Function
Last, but not the least, you can also calculate the sum of all “set_a
” elements with the “sum()
” function.
# Initializing a set set_a = {1, 2, 3, 4} # Using built-in function: sum() sum_of_set_a = sum(set_a) print(sum_of_set_a)
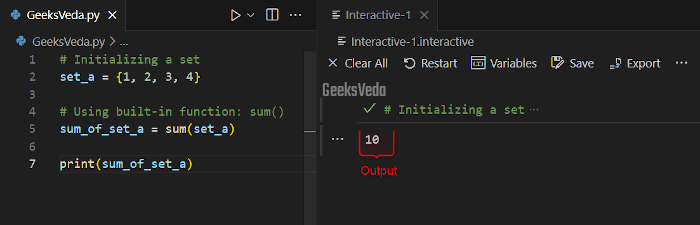
Iterate Over Python Sets
In Python, the operation of iterating over the Python sets can be done with for loops or set comprehensions.
1. Using for loop
In the first example, we will utilize the “for
” loop for iterating through the “languages
” and display each language on the console with the print() function.
# Initializing a set languages = {'Python', 'Java', 'JavaScript'} # Using a for loop to iterate over the set for lang in languages: print(lang)
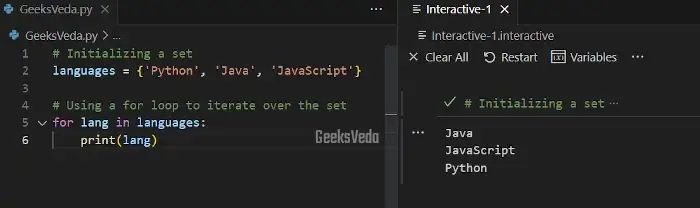
2. Using Set Comprehension
Here, set
comprehension technique has been deployed for creating a new set named “upper_case_languages
“, where each language name will be converted to uppercase.
# Initializing a set languages = {'Python', 'Java', 'JavaScript'} # Using set comprehension upper_case_languages = {lang.upper() for lang in languages} print(upper_case_languages)
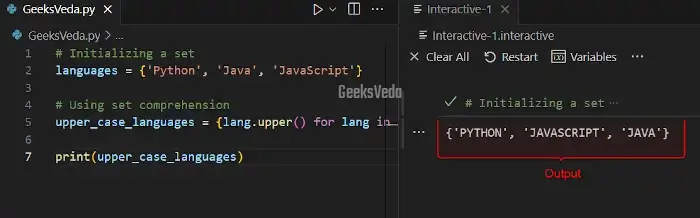
That’s all from today’s guide related to Python Sets and their usage.
Conclusion
Sets in Python provide a unique collection of elements with no duplicates. They can be used for performing operations like union, intersection, and difference between two sets.
This assists in problem-solving and enables seamless data manipulation. So, use these data structures for enhancing performance in your Python projects.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!