Knowing about reading inputs as numbers is crucial in programming for permitting accurate calculations and data manipulation. More specifically, mastering this skill in Python enables you to process integers, floats, complex numbers, decimals, and fractions efficiently.
With precise numerical data handling, you can unleash the potential for scientific analysis, and financial modeling.
Today’s guide will discuss different methods that can be utilized for reading inputs as numbers such as int()
, float()
, complex()
, Decimals()
, and Fractions()
.
Moreover, we will also enlist the best practices for reading inputs as numbers in Python.
Read Inputs as Integers in Python
In Python, the built-in int()
function can be utilized for converting the user input into an integer. This function takes a number or string as an argument and resultantly outputs an integer.
More specifically, this function can be combined with the input()
function where it is required to read user input as integers.
For instance, in the provided example, firstly, we will call the input()
function for reading the user input as a string. Then, for converting the string input into an integer, the int()
function will be used.
For example, what if the user submits a non-integer value? To handle such a situation, wrap the int()
function in the try-except block and catch the ValueError exception.
The added catch block can then print an error message and prompt the user for entering a valid integer in the prompt.
# Reading integer input from user user_input = input("Enter any integer: ") # Converting input to integer with int() function try: integer_input = int(user_input) print("Integer input is:", integer_input) except ValueError: print("Invalid input. Kindly enter any integer.")
After executing the code, we will enter 54 as the input value. It can be observed that the input has been converted to a number successfully.
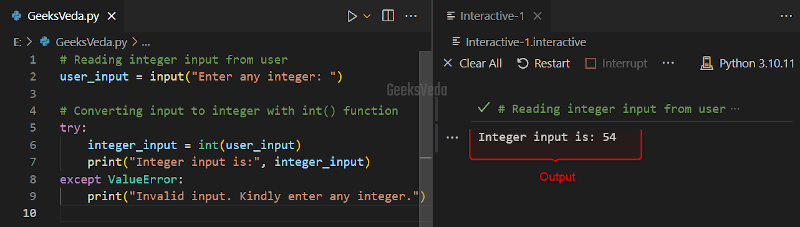
However, in case of entering an invalid input, the following message will be shown on the terminal.
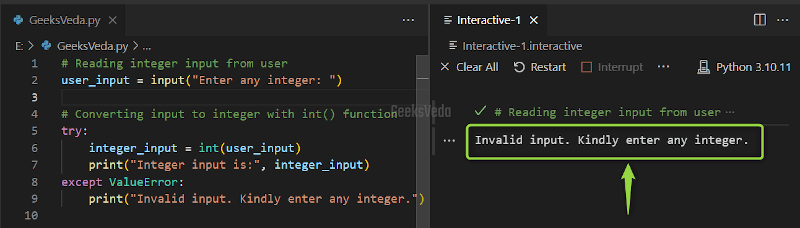
Read Inputs as Float in Python
The built-in Python function named float()
can convert the user input into a floating number. This function accepts a number or string as an argument.
It then converts the passed value to the floating point and outputs the value in combination with the input()
function.
Here, in the provided code, the program will first accept the user input by utilizing the input()
function Then it passes this value to the float()
function that is wrapped in the try block. More specifically, the try-except block is added to handle the non-floating point value.
# Reading floating-point input from user user_input = input("Enter a floating-point number: ") # Converting input to float with float() function try: float_input = float(user_input) print("Floating-point input is:", float_input) except ValueError: print("Invalid input. Kindly enter a floating-point number!")
Enter value after executing the program. It can be observed that the user input has been converted to a floating point successfully.
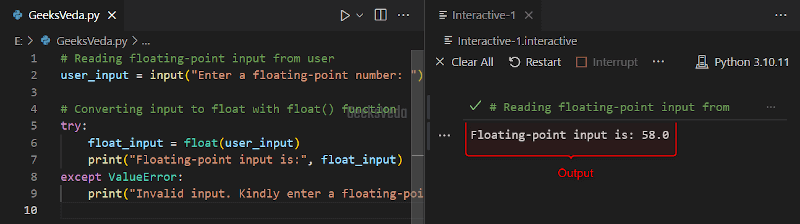
Read Input as Complex Numbers in Python
Want to create a complex number from an imaginary or real value as a+bj or a-bj
? You can utilize the complex()
function in such a scenario. This built-in method accepts two arguments, the real and imaginary parts, and resultantly outputs the corresponding complex number.
Now, let’s first invoke the input()
function to get the user input. After that, we will add a try-except block to handle invalid complex numbers, non-numeric characters, or strings having incorrect formats.
# Reading complex number input from user user_input = input("Enter a complex number in a+bj form: ") # Converting input to complex number using complex() function try: complex_input = complex(user_input) print("Complex number input is:", complex_input) except ValueError: print("Invalid input. Kindly enter a valid complex number!")
Let’s enter a complex number in a+bj format as 2+3j.
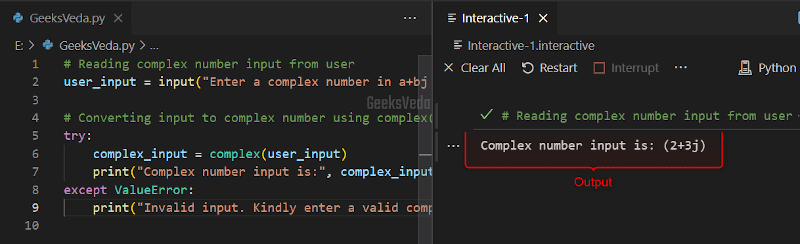
In case when any non-numeric character is entered, the ValueError exception will be displayed and the prompt will ask to enter a valid complex number.
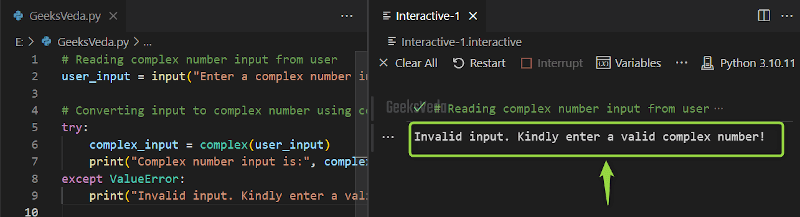
Read Inputs as Fraction and Decimal Numbers in Python
Python also supports the option to read other number types such as decimals and fractions, in addition to complex numbers, floating-point, and integers.
Fractions numbers represent rational numbers. On the other hand, the decimals type indicates the numbers having high precision.
Now, let’s write out a Python program that will read fraction input. To do so, firstly, import the fractions module in your Python code.
Then, take user input in a/b form and convert the input to a fraction by utilizing the Fraction()
function.
# Reading fraction input from user from fractions import Fraction user_input = input("Enter a fraction in a/b form: ") # Converting input to Fraction using Fraction class try: fraction_input = Fraction(user_input) print("Fraction input:", fraction_input) except ValueError: print("Invalid input. Kindly enter a valid fraction!")
Here, we will input the fraction as 5/11. Resultantly, it will be converted to a fraction and the value will be displayed on the console as follow.
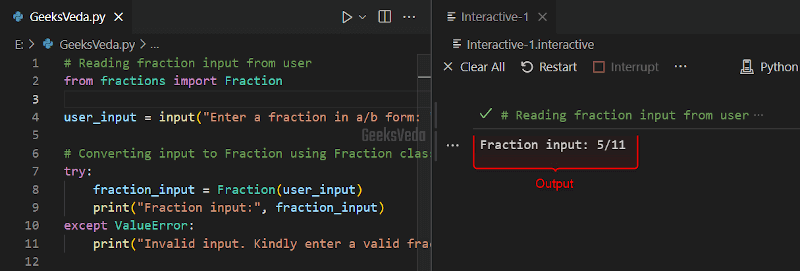
Likewise, we will import the decimal module to convert the input to decimal using the Decimal()
function.
# Reading decimal input from user from decimal import Fraction user_input = input("Enter a decimal number: ") # Converting input to Decimal using Decimal class try: decimal_input = Decimal(user_input) print("Decimal input:", decimal_input) except ValueError: print("Invalid input. Kindly enter a valid decimal number!")
We have entered 0.02 as the input value. Finally, the specified value has been converted into decimal number.
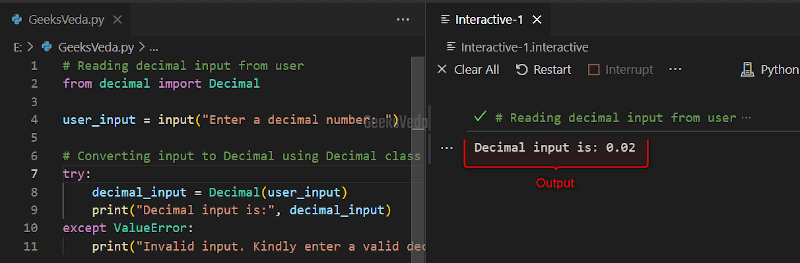
Best Practices for Reading Inputs as Numbers
Have a look at the given list of best practices for reading inputs as numbers in Python.
- Make sure that the input meets the expected range and format.
- Utilize the most suitable function like
int()
,float()
,complex()
,Fraction()
, andDecimal()
, according to the desired number type. - Use try-except blocks for catching the error during the conversion.
- Provide clear instructions regarding the entering number in the expected format.
- Prompt the user until the valid input is entered.
- Validate the code and test thoroughly.
That’s all from this information guide regarding reading inputs as numbers in Python.
Conclusion
As a Python programmer, you must master the skill of reading inputs as numbers if you want to write effective code. You can ensure correct numerical conversion by utilizing the most appropriate functions like int()
, float()
, complex()
, Decimal()
, and Fractions()
, error handling through the try-except block, and input validation.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!