At this stage, we have explored fundamental concepts of Golang, including variable and constant usage, as well as understanding project structure.
In the upcoming lessons, we will delve into more intriguing topics within Golang, expanding our knowledge and skill set. These topics will further enhance our understanding and proficiency in working with the Go programming language.
In programming, there are often three sequential processes involved: Input, Processing, and Output. These processes help us handle specific requirements in a program’s code.
- Input – It involves receiving or obtaining a value that will be used later.
- Processing – This step involves performing operations or calculations on the inputted values.
- Output – It refers to the resulting value obtained from the processing step.
In this lesson, we will explore how to efficiently handle input values and display the output of a Golang program.
1. Take User Input in Golang
Golang offers a convenient function for capturing user input from the keyboard. There are three distinct input methods available in Golang.
Scan()
– Used for obtaining numeric values.Scanf()
– Designed for capturing string values.Scanln()
– Specifically used for reading numeric values at the end of a line.
These three input methods in Golang can be selected based on the data type you are working with, allowing you to tailor the input approach to your specific requirements.
Variables entered in an input can be more than one. Let’s make an example.
package main import ( "fmt" ) func main() { var firstName, lastName string var age int fmt.Println("Your First Name : ") fmt.Scanf("%s", &firstName) fmt.Println("Your Last Name: ") fmt.Scanf("%s", &lastName) fmt.Println("Your age:") fmt.Scanln(&age) }
Please take note of the following code snippet, it includes two variables with string data types and one variable with a numeric (integer) data type.
It’s important to observe that the syntax for capturing input differs between string and integer data types.
For string input, the Scanf()
function is utilized, whereas the Scanln()
function is used for integer input.
Therefore, it is crucial to pay attention to these distinctions when employing these functions in your code.
When executed, it will produce an action against an input.
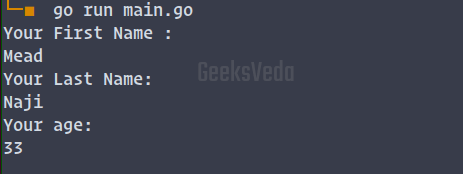
2. Print Output Values in Golang
In Golang, there are multiple ways to print output values using the “fmt
” package. To align with the current curriculum, you can utilize the “println
” function to print standard values in Golang. You can print multiple values by separating them with commas (,
).
Alternatively, the “printf
” function provides additional flexibility by accepting two inputs: a string format specifier and the corresponding variable value(s).
This allows you to print values with specific formatting and include multiple variables if needed.
The following is an example of the code displaying the input.
fmt.Printf("Your Name => %s %s \n", firstName, lastName) fmt.Printf("Your age => %d \n", age)
Symbols %s
and %d
are placeholders for string and integer values, respectively.
The code snippet above displays input based on the code we created earlier. So the complete code is like this.
package main import ( "fmt" ) func main() { var firstName, lastName string var age int fmt.Println("Your First Name : ") fmt.Scanf("%s", &firstName) fmt.Println("Your Last Name: ") fmt.Scanf("%s", &lastName) fmt.Println("Your age:") fmt.Scanln(&age) fmt.Printf("Your Name => %s %s \n", firstName, lastName) fmt.Printf("Your age => %d \n", age) }
Now if it is run it will print the input values for all variables.
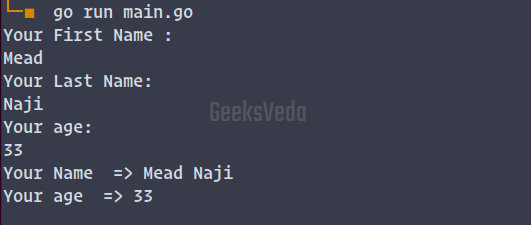
If you take a Look at the code above, there is a symbol for example “%s
“, what is that for?
String Format Specifiers
%v
– Represents any data type and provides a string representation of the value.%t
– Specifically for boolean data type, it displays “TRUE” or “FALSE” accordingly.%b
– Used to display binary data, showing either 0 or 1. For example, when applied to an integer, it prints the binary representation of the integer.%c
– Displays Unicode character data.%d
– Displays integer data with a maximum length of 10.%f
– Displays decimal data when the input data type is float.%s
– Displays string data.%t
– Displays the variable’s type.%%
– Displays the percent character itself.
We use those format specifiers within functions like Printf
to define the expected data type and format of the corresponding values being printed.
3. Write Comments in Golang
Comments serve various purposes in program code, such as adding notes, providing explanations or descriptions for code blocks, and even remarking (deactivating) unused code. When compiling or executing the program, comments are ignored.
In Go, there are two types of comments.
- Go Single-line Comments
- Go Multi-Line Comments
Let’s delve into the application and distinctions between these two comment types in our discussion.
Golang Single Line Comments
Writing this type of comment begins with a double slash sign (//
) and is then followed by the comment message. Single-line comments apply to one line of messages only.
If the comment message is more than one line, then the //
sign must be written again on the next line.
package main func main() { // Hello this a Comment // Another line of comment }
As mentioned earlier, comments do not impact the code’s execution, but allowing us to include comments for documentation purposes.
Additionally, we can use comments to exclude specific lines or blocks of code from being executed let’s do an example here.
package main import "fmt" func main() { // Hello this a Comment // Another line of comment // fmt.println("Hello from comments") fmt.println("hello without comments") }
And the output of this code will be like this.
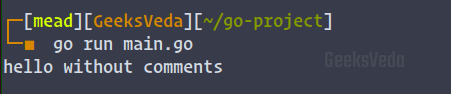
Golang Multi-Line Comments
Comments that are long enough will be neater if written using multiline commenting techniques. The characteristic of this type of comment is that it begins with a /*
sign and ends with */
.
Here is an example of how to use Multi line comments.
package main import "fmt" /* Hello this a multiline Comments fmt.println("Hello from comments") fmt.println("hello without comments") */ func main() { fmt.Println("hello") }
And the output of this code will be like this.

Conclusion
In conclusion, we have covered several fundamental concepts of Golang, including variable and constant usage, understanding project structure, input handling, and displaying output values.
We explored the use of format specifiers to define the expected data types and formats when printing values. Additionally, we discussed the importance of comments in Golang, including Singleline and Multiline comments, and their role in code documentation and remarking unused code.
As we progress in our learning journey, we will continue to explore more interesting topics in GoLang, further enhancing our knowledge and skills in this programming language.