We’ve already covered the installation process and created our first Golang project in prior parts of this Golang series.
In this post, we’ll continue our exploration of the Go programming language by looking at variables and constants. Understanding the fundamentals of variables and constants will allow you to write more robust and efficient Go programs.
Variables are an important programming concept that you must understand and grasp since they are symbols that represent the values that you use in your program.
In this article, we will discuss the foundations of variables as well as best practices for dealing with them.
Why Do We Need a Variable
Variables are used in programming to add flexibility and adaptability to programs – a program would be static and predictable if variables were not used, providing the same result for every input.
Variables are used to represent changing values or data during program execution, which enables the program to react to various inputs or conditions, making it more helpful and versatile.
An example of a variable is a company fund that changes in value depending on the amount of money added or removed from it. The value of the fund can only be known at the moment when it needs to be accessed, making it a variable.
In contrast, a constant value, such as a fixed amount of money that needs to be paid each month, is constant because it does not change during the execution of the program.
Variables are important in programming because they enable the development of dynamic and adaptive programs. Programs would be limited to fixed and predictable outcomes if they did not have them.
Variables allow programs to adjust to changing inputs and conditions, increasing their usefulness and power.
Variables in Golang
Consider the following sentence: “Welcome to GeeksVeda, a place where you can learn Linux and programming“. If we need to use this sentence multiple times in our project, it would be cumbersome to rewrite it every time. To avoid this, we can assign the sentence to a variable, like so:
name = "Welcome to GeeksVeda, a place where you can learn Linux and programming"
Then, whenever we need to use the sentence in our project, we can simply call the variable name
.
Go adopts two types of variable writing, namely those with data types and those that are not. Both methods are valid and have the same purpose, the difference is only in the way of writing.
In this section, we will thoroughly examine the various ways of variable declarations.
Declare a Variable with Data Type in Golang
Go has pretty strict rules when it comes to writing variables. When the declaration, the data type used must be written as well. Another term for this concept is manifest typing.
The following is an example of how to create a variable whose data type must be written.
package main import "fmt" func main() { var name string = "Welcome to GeeksVeda, a place where you can learn Linux and programming." var website string website = "geeksveda" fmt.Printf("Hello %s %s", name, website) }

Let’s break down this syntax first. Here we use printf
, which allows us to print formatted output to the screen. And we’re using %s
as a format specifier to indicate that the value of a variable should be treated as a string and inserted into the formatted output.
The var
keyword above is used for variable declarations, examples can be seen in the name and website.
The value of the variable name
is entered immediately during the declaration, in contrast to the website
variable which is assigned the value after the line of code of the declaration, this is allowed in Go.
Declare a Variable without Data Type in Golang
Go’s type system not only supports manifest typing but also includes the concept of type inference. Type inference is a variable declaration method where the data type of a variable is determined by the data type of its assigned value, which is in contrast to manifest typing.
With type inference, the var
keyword and data type are not required to be explicitly written.
package main import "fmt" func main() { var name1 string = "Geeks" name2 := "Veda" fmt.Printf("Welcome To %s %s", name1, name2) }

The name1
variable is declared using type inference, where the data type is not explicitly written at the time of declaration. The :=
operator is used instead of the =
operator. The data type of the name2
variable is determined automatically based on its assigned value, which is the string “Veda
“.
You can continue to use the var
keyword at the time of declaration without specifying the data type, as long as you use the =
operator instead of the :=
operator.
For instance:
var name = "Mead"
is equivalent to:
name := "Mead"
:=
can only be made inside function blocks.Once a variable is declared using type inference, its data type cannot be changed. You can assign a new value to the variable using the =
operator.
For example:
package main import "fmt" func main() { name := "Mead" name = "Ravi" name = "Sharqa" fmt.Printf(name) }
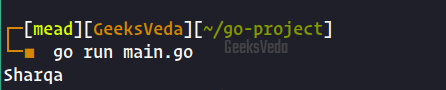
In summary, Go’s type inference system allows for concise and flexible variable declarations, enabling more efficient coding.
Go Multiple Variable Declaration
Go supports the method of declaring multiple variables at the same time, you do this by delimiting the variables with a comma (,
). It is also allowed to fill in the value at the same time.
var name1, name2 string name1, name2 = "geeks", "veda"
Filling in value can also be done simultaneously at the time of declaration. You do this by writing the value of each variable sequentially according to the variable with a comma delimiter (,
).
var firstName, lastName, language string = "geeks", "veda", "golang"
If you want to be more concise:
firstName, lastName, language := "geeks", "veda", "golang"
Using type inference techniques, the multi-variable can be done for variables whose data type is different from each other.
id, isAdmin, message := 1, true, "Hello Admin"
Variable Declaration Using Underscore
In Go, every declared variable must be used, unlike many other languages. If a variable is declared but not used, a compilation error will occur, and the program will not run.
To handle situations where a value is assigned to a variable but not used, the underscore (_
) variable is reserved as a placeholder to store unused values. It can be thought of as a wastebasket for values that are not needed.
For instance:
package main import "fmt" func main() { _ = "Geeks Veda" _ = "Learn Go Programming" name, _ := "website", "language" fmt.Println(name) }

In the example above, the variable name contains the string “website
“, while the value “language
” is assigned to the underscore variable, indicating that this value is not used.
The underscore variable is predefined, so you can directly assign values to it using the =
operator, without requiring the :=
operator.
However, when using type inference to declare multiple variables, you can include an underscore variable to indicate that a specific value will not be used.
The underscore variable is commonly used to hold the return value of a function that is not used in the code.
Please note that the contents of an underscore variable cannot be displayed. Any data assigned to an underscore variable will be lost and cannot be retrieved.
It’s similar to a black hole, where any object that enters it will be trapped forever in the singularity and cannot escape.
Variable Declaration Using Keyword New in Golang
The new
keyword in Go is used to allocate memory and create a pointer variable of a specific data type. By default, the value of the pointer variable will be nil. Here’s an example:
package main import "fmt" func main() { age := new(int) fmt.Println(age) }
In the example above, age is a pointer variable of type *int
. When we print age, it displays the memory address of the value that the name points to in hexadecimal notation. To display the actual value, we need to dereference the pointer variable using the *
operator.
Pointers can be confusing at first, but they are an important concept in Go and many other programming languages. We will explain pointers in more detail later on.
Variable Declaration Using Keyword Make in Golang
The make
keyword can only be used to create several types of variables, namely:
- channels
- slice
- folder
And again, perhaps many will be confused. When you have entered into the discussion of each of these points later in this series, you will see what the use of this make keyword is.
Global and Local Variables in Golang
In Go, it’s essential to understand variable scope to ensure that variables are accessible where and when they are needed. Variables can have global or local scope.
Global variables are declared outside functions and can be accessed from anywhere in the program. On the other hand, local variables are defined inside functions and can only be accessed within that function.
Consider the following example:
package main import "fmt" var year = 2023 func getDate() { var month = "May" fmt.Println(month) fmt.Println(year) } func main() { getDate() fmt.Println(year) }
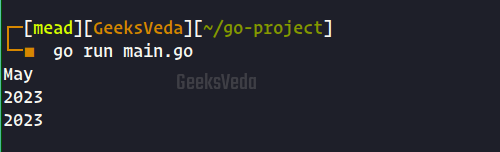
In this example, year
is a global variable, and month
a local variable. When getDate()
is called, it prints the value of month
(“local”) and then returns.
Then main()
prints the value of the year
(“global”). Since g is global, it can be accessed from within getDate()
.
If we try to access a local variable outside of its scope, the program will throw an error. Consider this example:
package main import "fmt" var year = 2023 func getDate() { month := "may" fmt.Println(month) } func main() { fmt.Println(month) }
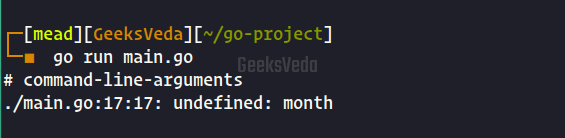
This program will throw an error, stating that month
is undefined in the scope of main()
. This is because the month is only defined within the scope of getDate()
, and cannot be accessed outside of that function.
Sometimes, we may want to use the same variable name for both a global and local variable. Consider this example:
package main import "fmt" var name = "Mead" func getNames() { name := "Ravi" name2 := "Sharqa" fmt.Println(name) fmt.Println(name2) } func main() { getNames() fmt.Println(name) }
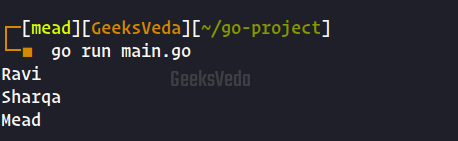
Note that here we use a new concept, which is functions, just to demonstrate how local and global variables work. To make it simple, think of a function like a house that contains some stuff.
In our example here, variables indicate that if you are inside the house, you have access to everything inside (local), and if you are outside the house, you don’t.
In this example, name
is declared as a global variable with a value of “Mead
“. In getNames()
, a new local variable named name1 is declared and assigned a value of “Ravi
“.
When getNames()
is called, it prints the value of the name “Ravi
” and name2 “Sharqa
“. Then main()
prints the value of the global variable name “Mead
“.
When working with variables, it’s important to choose between global and local variables depending on the use case. Local variables are usually preferred, but Global variables can be useful when the same variable needs to be accessed in multiple functions.
Understanding Data Types in Golang
The data type is a type of data stored in a variable. Data types have different forms and uses. A data type will later be assigned a value.
The following data types are used in the Golang programming language:
Integers
Integers are used for numbers, for example, 1, 2.
But basically, integers can be broken down into integer-covered data types. The following are integer data types.
- uint8 : 0 to 255
- uint16 : 0 to 65535
- uint32 : 0 to 4294967295
- uint64 : 0 to 18446744073709551615
- int8: -128 to 127
- int16 : -32768 to 32767
- int32 : -2147483648 to 2147483647
- int64 : -9223372036854775808 to 9223372036854775807
An example using the Integer data type:
var age integer = 33
Floats
Float is used for data in the form of fractional data / decimal numbers. For example 1,2.
Following are the different types of float data types:
- float32
- float64
- complex64
- complex128
An example using the float data type:
var float width = 3.2
Usually, the float data type is used for the use of mathematical operators, such as multiplication, division, and others.
String
The String data type is used for data in the form of text usually, for example “welcome to geeksveda
“. For Instance.
var name string = "GeeksVeda"
In the Golang programming language, the value of a text must be enclosed in double quotation marks (“…”
). The string data type is usually used to write text descriptions.
Boolean
The Boolean data type can be used to provide only 2 values, namely TRUE and FALSE.
It should be noted that writing TRUE and FALSE must start with an uppercase letter so that it does not cause errors. For Example.
var isAdmin boolean = True
Have you ever heard an analogy that states that a positive value is added to a negative and the result is negative?
The Boolean data type can also be done, as follows. The result of an expression between two meeting values between TRUE and FALSE.
Conditions or expressions are usually marked with && (and)
and || (or)
.
Expression && (and)
Expression && (and)
returns true if both operands are true, and false otherwise.
- true && true : true
- true && false : false
- false && true : false
- false && false : false
Expression || (or)
Expression || (or)
returns true if at least one operand is true.
- true || true : true
- true || false : true
- false || true : true
- false || false : false
Constants
Constants are like variables, but with a value that cannot be changed after it is declared. They are useful when you want to use a specific value in your program that should not be modified, such as the value of pi in math or the tax rate in a shopping cart system. Using a constant instead of a variable ensures that the value remains the same throughout your program, even if you accidentally attempt to change it.
You can declare a constant in Go using the following syntax:
package main import "fmt" func main() { const salary = 5000 fmt.Println("Your Salary is", salary) }
Of course, we don’t want the salary
to be constant, but if a project owner sets a constant, it will make the salary constant.
Attempting to modify the value of a constant will result in a compile-time error.
Constants can be of a specific type or untyped. If a constant is untyped, it can be used with any other type. If a constant is of a specific type, it can only be used with values of the same type.
You can specify the type of a constant using the following syntax.
package main</pre> import "fmt" func main() { const name = "Mead" const age int = 33 fmt.Println("Your age is", age) }
In the example above, the name is an untyped constant, so it can be used with any other data type. age, on the other hand, age is a typed constant with a type of int, so it can only be used with other int values.
Conclusion
In this tutorial, we covered the fundamentals of variables and constants in the Go programming language. We explore the different ways to declare variables in Go, as well as the distinctions between local and global variables.
Additionally, we also delve into the concept of constants, how they are defined and used, and how they differ from variables. At the end of this tutorial, you should now have a solid understanding of how to declare and utilize variables and constants in Go.
In the upcoming lessons, we will delve deeper into the Go language and explore more concepts.