In case you are working with Python lists, it is essential to understand the process of counting the occurrences of the specified items.
This functionality assists in performing statistical calculations, processing user input, or analyzing data.
In today’s guide, we will demonstrate different approaches for counting the occurrences of an item of a list from basic methods such as the count()
method, for loop, to more advanced techniques like using collections module or NumPy library.
Count Occurrences of Items in Python List
In Python, you can consider the below enlisted basic methods for counting the occurrences of list items.
count()
Methodfor loop
Method- A dictionary and a for loop
Let’s check out each of the mentioned approaches and count list items.
1. Using Python List count() Method
In Python, the built-in count()
method can be utilized for counting the number of occurrences of a particular or defined item in a list. This method accepts a single argument that represents the value required for counting.
To demonstrate the functionality of the count() method, firstly, we will create a list named list1 having nine items. Then, call the count()
method and pass 3 as an argument.
list1 = [3, 8, 9, 2, 3, 7, 10, 4, 3] count = list1.count(3) print(count)
As a result, the print()
function prints out the number of occurrences of the specified item as 3.
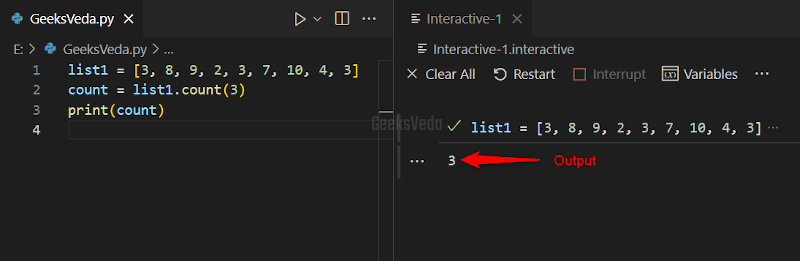
2. Using Python List for loop Method
Utilizing the for loop is another approach that can be used for counting the occurrences of an item. This loop iterates over the newly created list and increments or decrements a counter variable each time the item has been found.
Have a look at the given program.
list1 = [3, 8, 9, 2, 3, 7, 10, 4, 3] count = 0 for num in list1: if num == 3: count += 1 print(count)
According to the above-given code, firstly, we have created a new list1
and initialized a counter variable named count
to the value 0.
Then, a for loop
is added for iterating over the items in the list.
For each item, the for loop
will verify if its value equals 3. If this condition is met, the counter variable’s value will be incremented.
Lastly, the count variable value will be displayed on the console which refers to the number of occurrences of item 3 in the list1
.
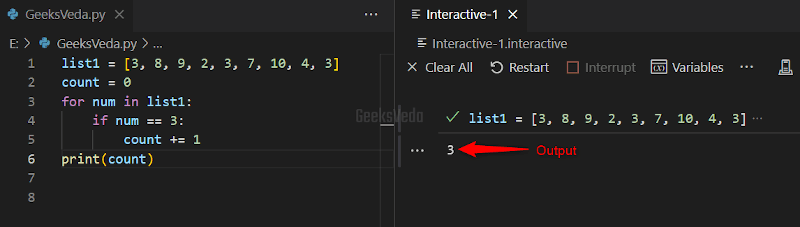
3. Using Python List Through a Dictionary and a for loop
Another basic way for counting the occurrences of items is to create a Python dictionary for storing each item count. Then, utilize for loop
for iterating over the list to update the dictionary counts.
In the provided code, firstly, we have created a list1
. Then, we initialized an empty dictionary named counts
. The next step is to iterate over list1
by utilizing the for loop
.
During each iteration, the added condition will validate if the current item already exists in the counts
dictionary.
If yes, then the associated value of that specific key will be incremented. Otherwise, a new key-value pair will be added with a count 1 value.
In the end, we will display the value of the key 3 from the counts
dictionary.
list1 = [3, 8, 9, 2, 3, 7, 10, 4, 3] counts = {} for num in list1: if num in counts: counts[num] += 1 else: counts[num] = 1 print(counts[3])
The given output signifies that the 3 keys appear three times in the list.
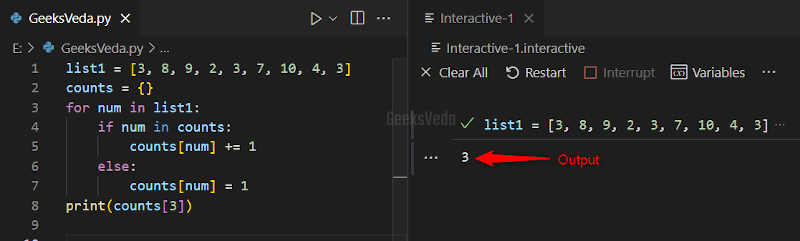
Advanced Methods for Counting List Items in Python
Want to explore some advanced methods for counting the occurrences of list items? Here are the two techniques to your rescue.
- Collections Module
- NumPy library
The given subsections will discuss both of them in detail!
1. How To Use Collections Module in Python
collections is a built-in Python module that offers particular container data types that perform well compared to the built-in containers. This module comprises multiple classes that can be utilized for counting the occurrences of an item in the list.
Using Counter Object
The Counter object can be imported from the collections modules for counting an item in the defined list. To check out its functionality, execute the given program.
from collections import Counter list1 = [3, 8, 9, 2, 3, 7, 10, 4, 3] count = Counter(list1) print(count[3])
Here, first of all, we have imported the Counter class from the collections module. Then, we created a new list named list1
. After that, a new Counter object called count
is created.
The next step is to retrieve the count of key 3 from the count dictionary and display it on the console.
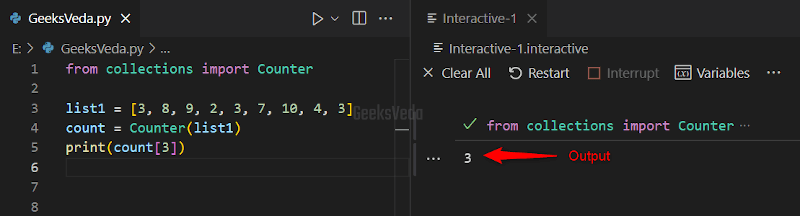
It can be observed that the specified key occurred 3 times in the list.
Using defaultdict Object
defaultdict is another object that can be used for counting the occurrences of an item in the list. The primary difference between defaultdict and Counter is that the defaultdict object can be initialized with the default key value that is not present in the dictionary.
So, to use this object, first of all, we imported defaultdict
from the collections module. Then, we created a new defaultdict object named count
having 0 as its initial value.
After that, the for loop
is used for iterating over the list1
items. On each iteration, the value of the count is incremented.
Lastly, the value associated with key 3 has been displayed on the console.
from collections import defaultdict list1 = [3, 8, 9, 2, 3, 7, 10, 4, 3] count = defaultdict(int) for num in list1: count[num] += 1 print(count[3])
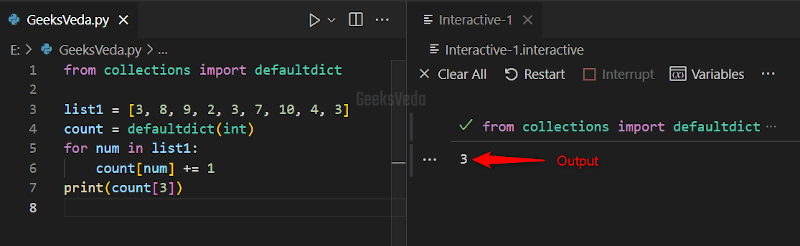
2. How to Use NumPy Library in Python
In Python, NumPy is a library that offers support for multi-dimensional arrays, and large matrices. This library provided multiple functions for manipulating these arrays.
More specifically, you can use this library for counting the occurrences of a list item by using.
numpy.unique()
methodnumpy.bincount()
method
Using numpy.unique() Method
This method is called for finding the unique items in a list with their corresponding count.
To use the numpy.unique()
method, firstly, you have to import the numpy module. Then, likewise, create a list.
After that, invoke the np.unique()
method for finding the unique items in the created list1 along with their counts.
More specifically, the second argument return_counts=True
tells the method to output an array of the count.
import numpy as np list1 = [3, 8, 9, 2, 3, 7, 10, 4, 3] unique, counts = np.unique(list1, return_counts=True) print(counts[3])
unique()
method will display its number of occurrences as 1.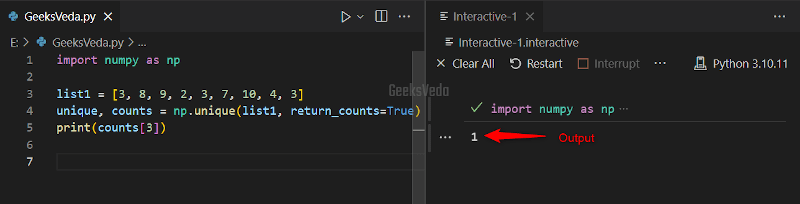
Using numpy.bincount() Method
The functionality of numpy.bincount()
is the same as that of the Counter object. However, it also works with non-negative integers. This method created a counts array in which each item index indicates an integer value.
This index value refers to the number of times the specific integer appeared in the list. Have a look at the given example.
import numpy as np list1 = [3, 8, 9, 2, 3, 7, 10, 4, 3] count = np.bincount(list1) print(count[3])
According to the given code, the bincount()
method returns the value associated with the integer 3 in the count array.
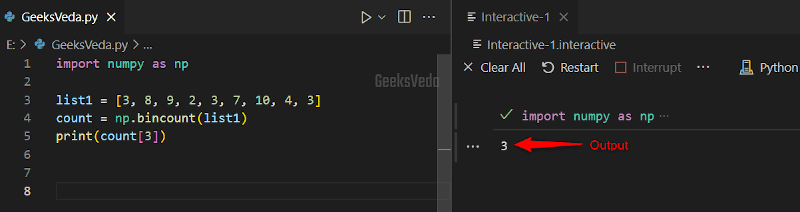
Advantages and Disadvantages of All Methods
In this last section, we will compare all of the advantages and disadvantages of the discussed methods.
Method | Advantages | Disadvantages |
count() method | Easy to use and simple. | Limited to only counting occurrences of a single item. |
for loop | Flexible for counting any item | Needs manual iteration and more coding |
dictionary and for loop | Count any item efficiently | Needs additional memory and manual iteration |
collections.Counter | Optimized for counting occurrences and supports any item | Needs to import the collections module |
collections.defaultdict | Optimized for counting, offers default value | Needs to import the collections module |
numpy.unique() | Provides unique counts, efficient for larger lists | Needs to import the numpy library |
numpy.bincount() | Works efficiently for non-negative integers also | Needs to import the numpy library |
Conclusion
Python supports several ways of counting the occurrences of a list item. More specifically, the count()
method and for loop
are the simplest options, while dictionaries and the collections module provide efficiency and additional features.
Advanced methods, such as unique()
and bincount()
of the NumPy library offer optimized solutions.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!