In Python programming, generators are robust tools that can improve memory usage and also maintain the code flow.
Unlike traditional structures like lists, generators
offer an optimized way of working with infinite sequences and large datasets.
Today’s guide will discuss the working of Python generators
, the method to create custom generators, generator expression, composing and chaining generators, and other concepts.
What are Generators in Python
Python generators
is the more memory-efficient approach for creating iterators. Unlike lists, or any other collection that can be utilized for storing elements in the memory, generators produce values on-the-fly.
Additionally, Python generators are primarily used for dealing with large datasets or infinite sequences.
How Generators Work in Python
Generators
are the types of Python functions that utilize the yield keyword for producing a series of values one at a time. More specifically, when a generator function is invoked, it does not run the function immediately. Instead, it outputs a generator object that can be looped over.
The generator
function runs only when each value is requested. Note that, it also retains its internal states between yields.
For instance, in the provided code, we have a “countdown()
” generator that yields a sequence of numbers.
Here, the for loop is utilized for iteration and each value is generated one by one and displayed on the console, as required.
def countdown(n): while n > 0: yield n n -= 1 counter = countdown(10) for num in counter: print(num)
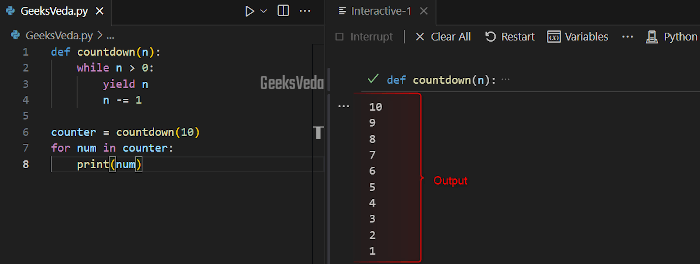
How to Create Custom Generators
For the purpose of creating a custom generator, define a function with the help of the yield keyword for producing values.
During each iteration, the state of the function will be saved. This permits you to resume execution from where it was left off.
This approach is primarily utilized for processing large datasets in your projects.
Now, in the following example, we will create a custom generator named “fibonacci()
” that yields Fibonacci numbers up to the given limit.
Here, the generator function generated each number in the sequence. Moreover, this happens without storing the whole sequence in the memory.
def fibonacci(limit): a, b = 0, 1 while a < limit: yield a a, b = b, a + b fib_sequence = fibonacci(100) for num in fib_sequence: print(num)
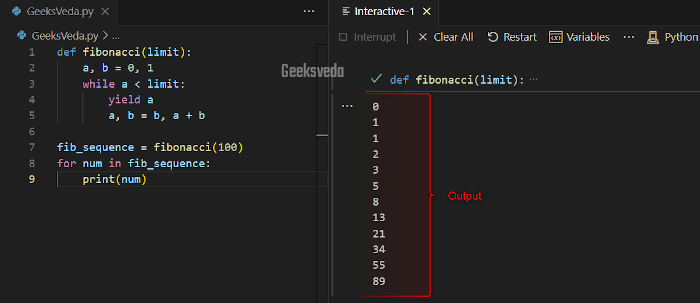
Python’s Generator Expressions
In Python, generator expression offers a concise approach to creating generator functions. Both list comprehensions and generator expressions have this thing in common, they produce value lazily.
This functionality permits you to iterate over large datasets without consuming large memory space.
According to the given code, we have a generator expression that generates even numbers up to 10.
even_num = (x for x in range(10) if x % 2 == 0) for num in even_num: print(num)
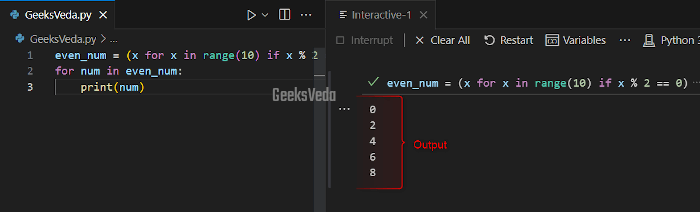
Chaining and Composing Generators Function in Python
You can easily chain and compose generators for creating more complex data processing pipelines. This approach can be utilized for transforming, filtering, or aggregating data.
For instance, in the below code, we have a generator function named “squares()
” that will produce squared values.
Then, the “negatives()
” generator negates them. By composing these generators, we will compute the negative squares of a list of numbers.
def squares(nums): for num in nums: yield num ** 2 def negatives(nums): for num in nums: yield -num numbers = [1, 2, 3, 4, 5] result = negatives(squares(numbers)) for num in result: print(num)
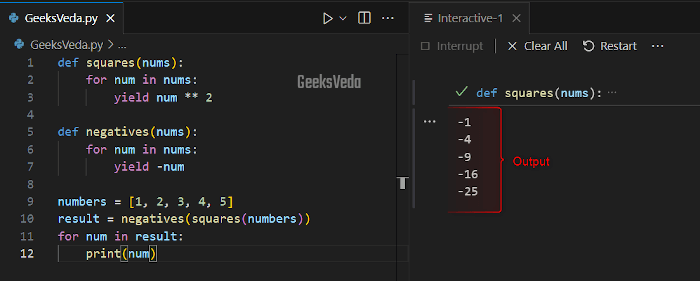
Infinite Sequences with Generators Function
In case you want to create infinite sequences, like infinite sequences of numbers, generators can be utilized. These sequences will be generated on-the-fly as needed. This approach enables you to work efficiently with potentially limitless data.
Now, let’s define a generator named “count_up()
” that produces an infinite sequence of numbers in incremental order. Then, we will use a while loop and yield numbers.
In this way, the generator function will permit you to work with sequences without worrying about memory limits.
def count_up(): num = 1 while True: yield num num += 1 counter = count_up() for num in counter: print(num) if num >= 10: break
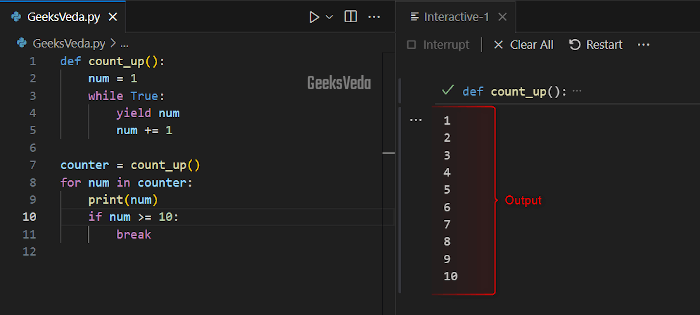
Generators vs Lists Function in Python
Let’s compare Python generators and lists with respect to the listed aspects:
Aspect | Generators Function | Lists Function |
Memory Efficiency | Memory-efficient generates items on the fly. | Eagerly store all items in memory. |
Evaluation | Lazily evaluated, one item at a time. | Eagerly evaluated, all items computed at once. |
Syntax | Created using functions and the yield keyword. | Created using square brackets and commas. |
Iteration | Efficient for large datasets. | Suitable for small to medium datasets. |
Comprehensions | Generator expressions are available. | List comprehensions are available. |
Length | Cannot use len() directly. | len() provides the number of items. |
Performance | Can be faster due to on-demand computation. | Slightly slower due to upfront computation. |
Use Cases | Streaming data, infinite sequences, and memory conservation. | Storing and processing small datasets. |
That’s all from this informative guide regarding Python Generators.
Conclusion
Python generators
offer an efficient solution for improving the code and optimizing the code usage. Moreover, they can be utilized for performing on-the-fly computation.
So, it is important for you to understand how generators work, the method to create custom generators, and other advanced techniques such as generator expressions and chaining. All of these concepts have been explained in our today’s guide.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!