Welcome to our guide on the key differences between the __str__()
and __repr__()
methods in Python. If you are a Python developer, it is crucial to grasp how these methods affect the object representation in your code.
In today’s blog, we will explore the purposes, syntax, and usage of __str__()
and __repr__()
methods. Moreover, we will also highlight their differences based on different aspects to help you create more readable and accurate object representations.
So, let’s delve into the world of __str__()
and __repr__()
in Python and unlock their potential!
What is __str__() in Python
In Python, the “__str__()
” method offers a human-readable representation of an object. It can be utilized for converting an object into a string, which makes it more user-friendly and understandable. More specifically, this method is primarily utilized for presenting information about an object in a format that can be easily interpreted by humans.
1. Syntax of __str__() Method in Python
Follow the provided syntax for using the __str__()
method in Python.
def __str__(self): # Return the string representation of the object
Note that the __str__()
method should be defined within a class. This method must output an object representation. It can access the methods and attributes of the object by utilizing the “self
” keyword.
Now, let’s check out some examples related to the usage of the __str__()
method in Python.
2. How to Implement __str__() For a Python Class
In the following example, the __str__
method is defined within the “Author
” class. This method resultantly outputs a string representation of an Author object, including the author’s name and age.
class Author: def __init__(self, name, age): self.name = name self.age = age def __str__(self): return f"Author: {self.name}, Age: {self.age}" author = Author("Sharqa Hameed", 25) print(str(author))
It can be observed that the __str__()
method successfully returned the string representation of the newly created “author
” object.
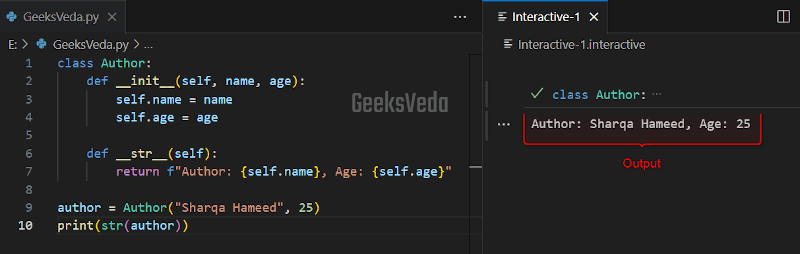
3. How to Use __str__() to Represent a Complex Number
Here, we will use the __str__
method for offering a string representation of a ComplexNumber object, which will display both real and imaginary parts.
class ComplexNumber: def __init__(self, real, imaginary): self.real = real self.imaginary = imaginary def __str__(self): return f"{self.real} + {self.imaginary}i" num = ComplexNumber(4, 7) print(str(num))
As you can see, we have created a “num
” object of the ComplexNumber class and passed 4 as real and 7 as its imaginary part which has been returned by the __str__()
method.
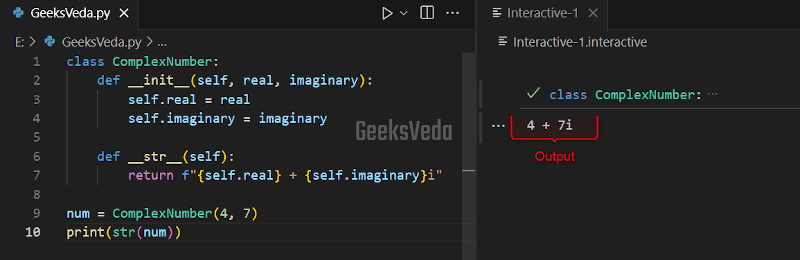
4. Advantages of Using __str__() in Python
Check out the given advantages of __str__()
method in Python.
- Customizable formatting options.
- Improved readability for users.
- Simplifies printing and display.
- Enhances debugging capabilities.
- User-friendly output.
What is __repr__() in Python
The “__repr__()
” method supports an unambiguous representation of a Python object. The main objective of using this method is to return a string that can be further utilized for recreating the object entirely. Unlike __str__()
, the __repr__()
method provides a precise and more detailed object representation for development and debugging purposes.
1. Syntax of __repr__() Method in Python
Have a look at the given syntax for using the __repr__()
method in Python.
def __repr__(self): # Return the unambiguous representation of the object
Similar to __str__()
, the __repr__()
method should be also defined within a Python class and must output a string that represents the object. This method typically includes the class name and other relevant information for the object recreation.
2. How to Implement __repr__() For a Python Class
In the given program, the __repr__()
method returns a string that represents a “Point
” object. It also comprises the values of the relevant “x
” and “y
” coordinates that can be utilized for recreating the same object.
class Point: def __init__(self, x, y): self.x = x self.y = y def __repr__(self): return f"Point({self.x}, {self.y})" point = Point(3, 5) print(repr(point))
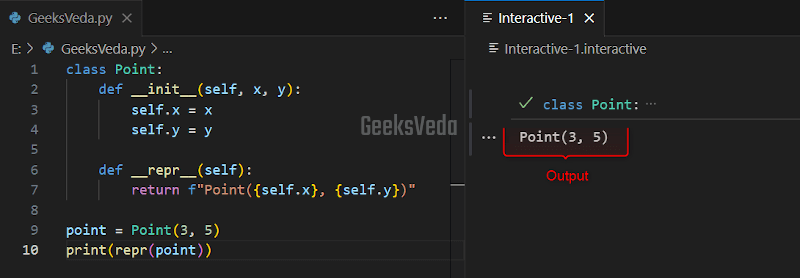
3. How to Use __repr__() to Represent a Custom Python Class
In the given code, the __repr__()
method displays a string representation of the CustomClass object, which also contains the value of its data attribute. This representation will be unambiguous and can be utilized for recreating the object.
class CustomClass: def __init__(self, data): self.data = data def __repr__(self): return f"CustomClass({self.data})" obj = CustomClass("Hello GeeksVeda User!") print(repr(obj))
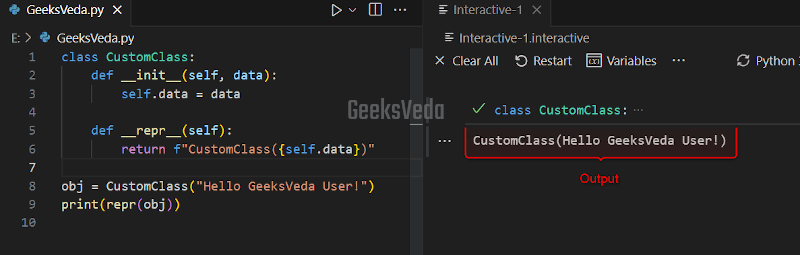
4. Advantages of Using __repr__() in Python
Have a look at the enlisted advantages of using __repr__()
in Python.
- Unambiguous representation.
- Offers detailed information for object recreation.
- Ensure accuracy in object representation.
- Facilities development and debugging.
- Useful for developers and internal systems.
Differences Between __str__() and __repr__() in Python
Now, check out the differences between __str__()
and __repr__()
methods in Python.
__str__() | __repr__() | |
Purpose | Offers a human-readable representation of an object. | Offers an unambiguous and detailed representation of an object. |
Intended Use | Readability and user-friendly output. | Development, debugging, and internal use. |
Format | Provides flexible formatting options. | Machine-readable format. |
Clarity | Focuses on offering information in an understandable format. | Make sure that the format includes uniqueness and more technical details. |
Output | Optimized for human consumption. | Emphasizes precision. |
Use cases | Generating reports, presentation data to users, and user interfaces. | Understanding object composition, debugging, and internal systems. |
Best Practices for Using __str__() and __repr__() in Python
Here are some of the best practices for using __str__()
and __repr__()
in Python.
- Keep
__str__()
concise and__repr__()
ambiguous and complete. - Implement both methods for detailed and user-friendly representations.
- Ensure consistency and test thoroughly.
- Use formatting options to improve output readability.
- Document the usage and purpose of both methods.
That’s all regarding the major differences between __str__() and __repr__() from this guide.
Conclusion
Understanding the differences between __str__()
and __repr__()
methods in Python is essential for effective object representation. More specifically, __str__()
focuses on user-friendly output. On the other hand, __repr__()
offers unambiguous details for debugging.
Implementing both methods improves the user experience, and code readability, and facilitates accurate object representation. By taking advantage of these methods, you can create robust Python applications that meet the requirements of both users and developers.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!