In today’s guide, we will explore Python Namespace
and Scope
. These crucial concepts can assist you to master the Python programming language.
We will discuss the boundaries between global and local scope, what enclosing or non-local scope is, the LEGB rule, built-in namespaces, local scope and functions, nested functions and closure, and lastly, compare the namespaces and scopes, respectively.
So, let’s begin this commendable learning journey!
Introduction to Python Namespace and Scope
As discussed in the introductory part, Python Namespace
and Scope
are considered fundamental concepts that can be utilized for evaluating the accessibility and visibility of variables and objects.
More specifically, a namespace
acts as a container that contains the names which are created during the execution of the program. On the other hand, scope
refers to the region where a specific name is accessible.
1. What is Python Namespace in Python
In Python, a namespace
is a process of mapping names to objects. It behaves as a container for functions, variables, classes, and other objects.
Additionally, each Python program comprises several namespaces that assist in organizing and differentiating between different names utilized in the programs. Namespaces
makes sure that the names are self-contained and unique.
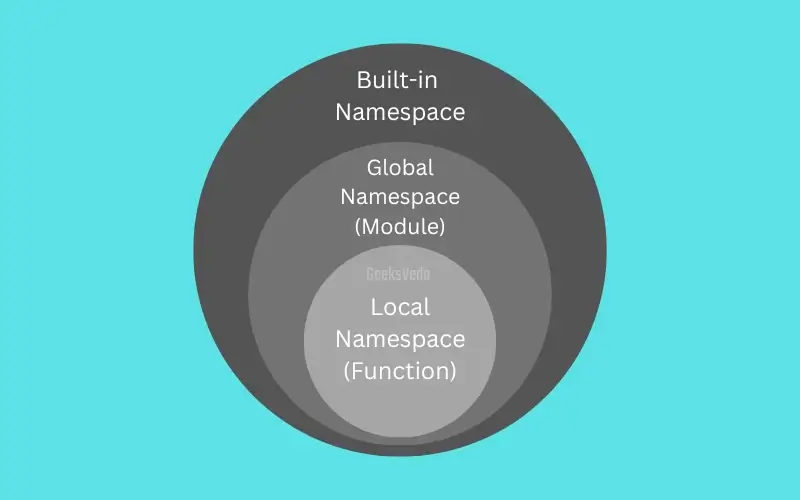
2. What is the scope in Python
Python Scope
specifies the region where the defined name can be accessed. It refers to the name visibility with the given program. Remember that Python has different types of scopes, such as:
- Global
- Local
- Enclosing (Non-Local)
Let’s check them out through practical examples and relevant explanations.
Global vs Local Scope in Python
Global
and local
scope are the two essential aspects of the accessibility of a variable. Therefore, understanding their key differences is important to write bug-free and efficient code.
1. Global Scope
In Python, a variable that has been declared outside of any class or function has global scope. This type of variable can be accessed from anywhere within the program, which comprises classes and functions.
Moreover, global variables retain their values throughout the execution of the program.
For instance, in the provided code, we have declared the “global_var
” global variable outside the “print_global()
” function. However, this function can access and print the value of the mentioned variable on the console.
global_var = 10 def print_global(): print("Global variable:", global_var) print_global()
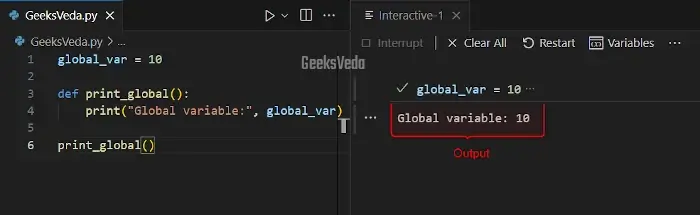
2. Local Scope
A Python variable that is declared inside a block or function has local
scope. This type of variable can be accessed within that particular block of function.
More specifically, the local variables are created when the function is invoked and destroyed when the function completes its execution.
Here, we have created the “local_var
” variable inside the “print_local()
” function. This local variable can be only accessed with the mentioned function and not outside.
def print_local(): local_var = 5 print("Local variable:", local_var) print_local()
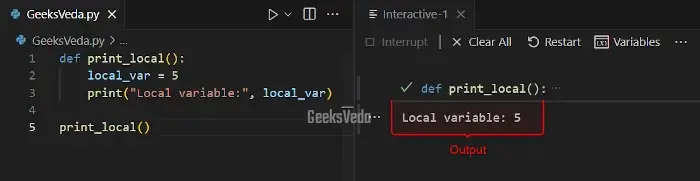
3. Comparison Between Global and Local Scope
The given comparison table demonstrates the key differences between global and local scope:
Scope | Visibility | Lifetime |
Global | Throughout the code | Until program ends |
Local | Within a function | Until function ends |
Enclosing Scope (Non-local Scope) in Python
Non-local
or Enclosing Scope
is a specified kind of scope that can be applied to nested functions. It permits the inner function to access the variable from the containing of enclosing functions.
1. How to Access Variables in the Enclosing Scope
In order to access variables from the enclosing scope, utilize the “nonlocal
” keyword. This keyword enables you to modify the variable value in enclosing scope from within the inner function.
In the given example, the “inner_function()
” uses the “nonlocal
” keyword for accessing and updating the value of the “outer_var
” from the enclosing scope which is “outer_function
” in this case.
def outer_function(): outer_var = 10 def inner_function(): nonlocal outer_var outer_var = 20 print("Modified outer_var inside inner_function:", outer_var) inner_function() print("outer_var outside inner_function:", outer_var) outer_function()
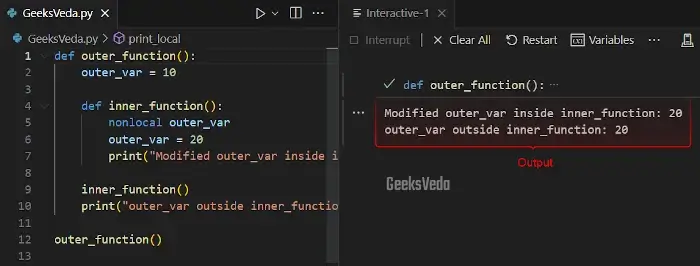
2. LEGB Rule in Python
LEGB is an abbreviation for Local
, Enclosing
, Global
, and Built-in
. It signifies the order in which Python searches for a variable name.
More specifically, Python follows the LEGB rule looking at the variable names in different scopes in the following order:
- Local: Python first looks for the variable inside the current function or local scope.
- Enclosing: If it is not located in the local scope, Python looks in the enclosing scope. For instance, if the function is nested inside another function.
- Global: Then, Python looks in the global scope, if not found in the enclosing or local scope.
- Built-in: Lastly, if the variable is still not found, Python will look in the built-scope, which comprises all the built-in variables and functions.
Here, the “inner()
” function contains a local variable “x
“. Python will find the value of those variables using the LEGB rule and in the local scope of the “inner()
” function.
x = "global" def outer(): x = "enclosing" def inner(): x = "local" print("Inner:", x) inner() print("Enclosing:", x) outer() print("Global:", x)
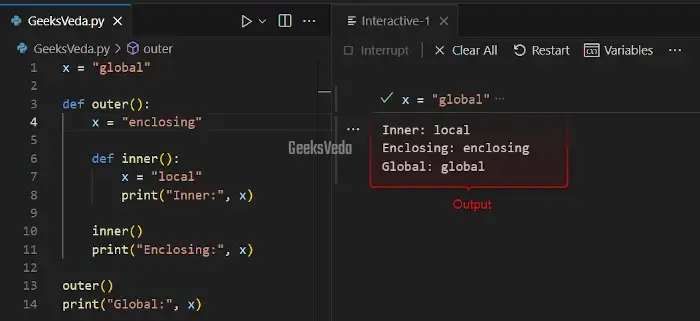
Built-in Namespaces in Python
Python built-in namespaces comprise built-in functions and variables that can be utilized without importing any module. Moreover, these built-in variables and functions can be accessed from any part of the code.
1. Built-in Functions and Variables
Python also offers several built-in functions and variables that can serve multiple purposes. For example, you can invoke the “len()
” function to check the length of the given argument, “range()
” to define a range, or the print() function for displaying the output on the console.
# Using the built-in functions print("Hello GeeksVeda User!") print(len([1, 2, 3])) print(range(5))
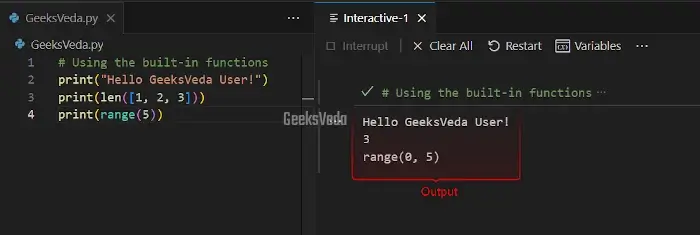
Likewise, the variables, such as “True
“, “None
“, and “False
” are also considered a part of the built-in namespace.
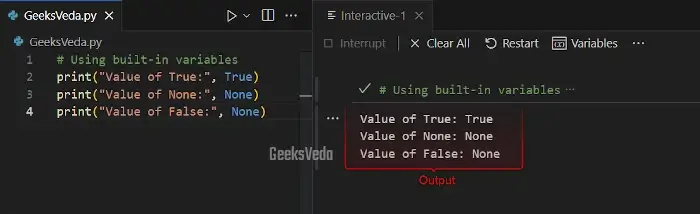
Local Scope and Functions
Local Scope
can be created when a function is called or invoked. It comprises the objects and variables defined within that function.
1. How to Create New Local Scope
When you invoke or call a function, a new local scope is created for it. Remember, any variables defined inside the function are local to that function and can be only accessed within it.
Here, we have defined the “local_var
” inside the “example_function()
“, which makes it a local variable and it can be only accessed within the mentioned function.
def example_function(): local_var = 10 print("Local variable inside the function:", local_var) example_function()
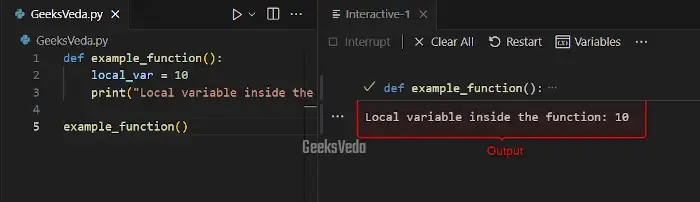
2. Variables and Functions Parameters
In Python, function parameters are also referred to as local variables within the defined function. Any variable that has been accepted as a function argument becomes a part of the function’s local scope.
For instance, here, the “a
” and “b
” variables are function parameters. Then, the “result
” variable is created inside the function. All these variables are local to the “multiply_numbers()
” function.
def multiply_numbers(a, b): result = a * b return result product = multiply_numbers(5, 3) print("Product:", product)
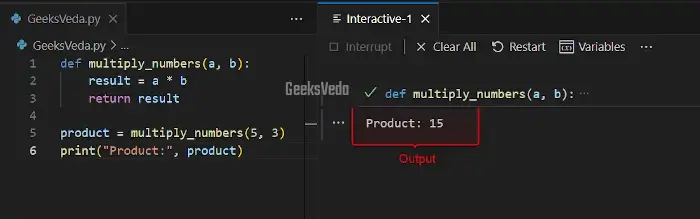
3. Nested Functions and Closure
Python enables the programmer to define functions inside other functions (called nested functions). These nested functions then have access to variables from the enclosing scope of the function, even after the execution of the enclosing function has been finished. This behavior is called Closure.
According to the given program, the “inner_function()
” has been nested inside the “outer_function()
“. This “outer_function()
” outputs or returns “inner_function
” which ultimately created a closure.
Then, the “add_five
” variable holds the closure with “x=5
“, which resultantly adds five in three and outputs “8
“.
def outer_function(x): def inner_function(y): return x + y return inner_function add_five = outer_function(5) result = add_five(3) print("Result:", result)
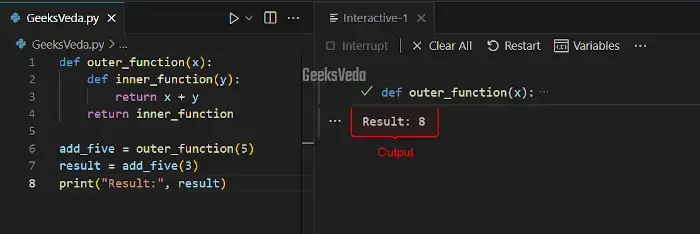
Global Scope and Variables in Python
In a Python program, global scope indicates the outermost level where variables are defined, such as outside any class of function. These types of variables are accessible from anywhere in your program.
1. How to Modify Global Variables
You can modify the global variables from within a function with the help of the “global
” keyword. This assists you to modify or change the value of the global variable from inside a function.
Here, the “modify_global()
” function utilized the “global
” keyword for accessing and modifying the “global_var
” variable. After invoking the function, the value of the “global_var
” variable will be changed to 15.
global_var = 10 def modify_global(): global global_var global_var += 5 modify_global() print("Modified Global Variable:", global_var)
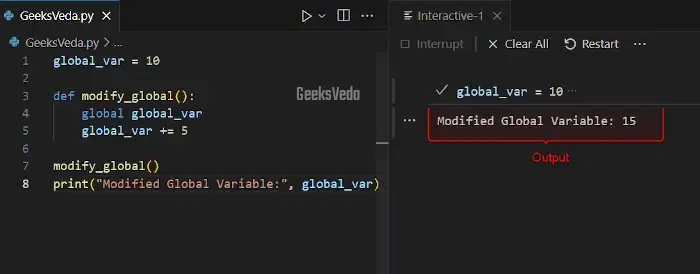
2. Global Keyword and its Usage
While writing a Python program, the “global
” keyword can be utilized for declaring a variable as global inside the function. It tells Python to use the created global variable rather than creating a new local variable having the same name.
In the following code, the “user_global()
” function utilized the “global
” keyword for the “global_var
” variable. Next, the function defined a local variable named “local_var
“.
Now, when the “use_global()
” function has been invoked, it outputs the sum of the created local and global variable as “15
“.
global_var = 10 def use_global(): global global_var local_var = 5 return global_var + local_var result = use_global() print("Result:", result)
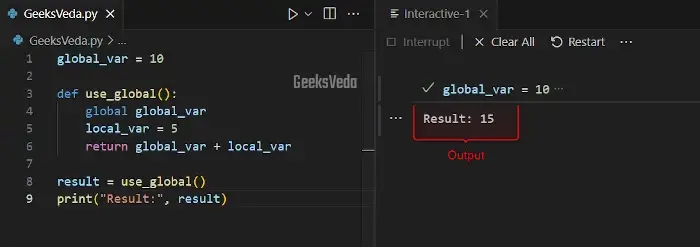
Understanding Python nonlocal Keyword
The “nonlocal
” keyword can be used for accessing and updating the variables in the non-local or enclosing scope of the nested function. It is different from the “global
” keywords, which handle the variables with the global scope.
1. Modify Variables in Enclosing Scope
The “nonlocal
” keyword for modifying the variables in the enclosing scope of the nested function. It can be used in scenarios where it is needed to have a nested function and you want to update or modify the variables in the outer scope of the function.
According to the given program, firstly the “outer_function()
” defines the “outer_var”. Inside this function, we will add a nested function named “inner_function()
” which uses the “nonlocal
” keyword for accessing and modifying the “outer_var
“.
After invoking the “inner_function()
“, the value of the “outer_variable
” will be updated to “15
“.
def outer_function(): outer_var = 10 def inner_function(): nonlocal outer_var outer_var += 5 inner_function() print("Modified Enclosing Variable:", outer_var) outer_function()
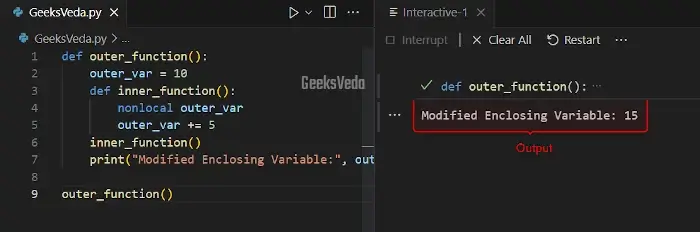
2. Difference Between “global” and “nonlocal”
The primary difference between “global
” and “nonlocal
” is that global keywords modify the variables in the global scope. On the other hand, “nonlocal
” updates the variables in the enclosing scope of the nested function.
In the following example, the “outer_function()
” defines “global_var
” and “outer_var
“. After that, the nested function “inner_function()
” utilized both “global
” and “nonlocal
” keywords, where “global_var
” has been updated with the global keyword and the “nonlocal
” keyword updates the “outer_var
“.
The resultant output will display the updated values for both of the mentioned variables.
global_var = 10 def outer_function(): outer_var = 5 def inner_function(): global global_var nonlocal outer_var global_var += 2 outer_var += 3 print("Inner Function Variables:", global_var, outer_var) inner_function() print("Outer Function Variables:", global_var, outer_var) outer_function()
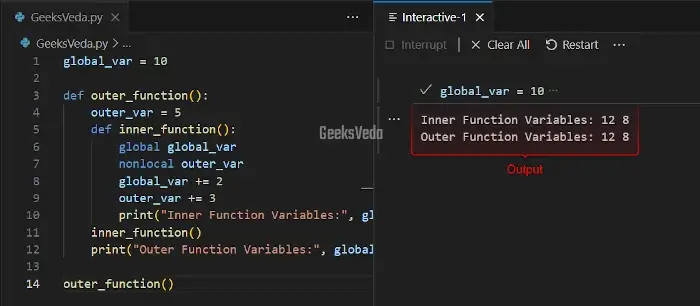
Difference Between Built-in, Global, and Local Namespace in Python
The given table compares the built-in, global, and local namespace based on enlisted features:
Features | Built-in Namespace | Global Namespace | Local Namespace |
Scope | Global scope. | Global scope. | Local scope. |
Accessibility | Accessible throughout the program. | Accessible throughout the program. | Accessible within specific code blocks. |
Declaration | Automatically available in any context. | Explicitly declared outside functions. | Explicitly declared inside functions. |
Examples | print() , len() , range() , True . |
Variables defined outside functions. | Variables defined inside functions. |
Difference Between Global, Local, and Enclosing Scope in Python
Now, we will compare all three types of scopes:
Features | Global Scope | Local Scope | Enclosing Scope (Non-local Scope) |
Scope | Global scope. | Local scope. | Inner function scope within outer scope. |
Accessibility | Accessible throughout the program. | Accessible within specific code blocks. | Accessible within nested functions. |
Examples | Variables defined outside functions. | Variables defined inside functions. | Variables are defined in the outer function. |
That’s all from today’s effective guides regarding Python Namespace and Scope.
Conclusion
Having knowledge related to Python namespace and scope is essential to do bug-free programming. Therefore, in today’s guide, we have discussed multiple concepts, such as global and local scope, built-in namespaces, and enclosing scope.
Moreover, the usage of global and nonlocal keywords, dynamic vs static scope, and the LEGB rule are also practically demonstrated.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!