While programming in Python, you may often feel stuck in a scenario where you want to exchange or arrange data by swapping two variables. This functionality can be achieved by using different Python methods, where each has its own advantages and disadvantages.
In today’s guide, we will explore multiple methods for swapping variables in Python. From traditional to creative approaches, check them out and choose the best one for your program!
What Does it Mean to Swap Variables
Swapping variables refers to the process of interchanging the two variables’ values. This operation is specifically helpful in situations where it is required to manipulate or rearrange the data without the need for extra storage.
The primary objective of swapping variables is to exchange their values, which becomes crucial when you want to reorder or update the data without creating additional variables.
How to Swap Two Variables in Python
In order to swap two variables in Python, you can use:
- A Temporary Variable
- Arithmetic Operations
- Tuple Unpacking (Multiple Assignment or One-Liners)
- XOR operation
Let’s check out each of the mentioned approaches and try to swap variables!
1. Using a Temporary Variable
One of the simplest and traditional methods for swapping variables is to utilize a temporary variable as a placeholder. This method works by storing 1st variable value in the temporary variable, assigning the 2nd variable value to the 1st, and then assigning the value back from the temporary variable to the 2nd variable.
For instance, in the provided code, the value of the variable “a” which is “5” will be stored temporarily in the “temp” variable before swapping it with the value of “b“, which is “10“.
Resultantly, the value of “a” becomes “10” and “b” becomes “5“.
# Swapping variables using a temporary variable a = 5 b = 10 print("Before swapping: a =", a, "b =", b) temp = a a = b b = temp print("After swapping: a =", a, "b =", b)
It can be observed that the values of the specified variables have been swapped and the updated values have been displayed on the console with the print() function.
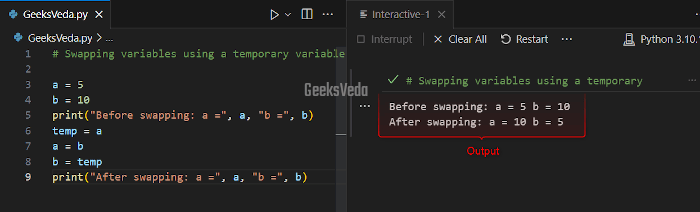
2. Using Arithmetic Operations
This approach is based on addition and subtraction operations for swapping values without requiring an additional variable. More specifically, by adding the values of two variables and then subtracting one from the other, the variables exchange their values.
Here, firstly, the value of “a” will be updated to the sum of “a” and “b“. Then, “b” will be updated to the difference between the new “a” and the original “b” values.
Lastly, the value of “a” will be updated to hold the difference, resulting in swapping the values of “a” and “b“.
# Swapping variables using arithmetic operations a = 9 b = 6 print("Before swapping: a =", a, "b =", b) a = a + b b = a - b a = a - b print("After swapping: a =", a, "b =", b)
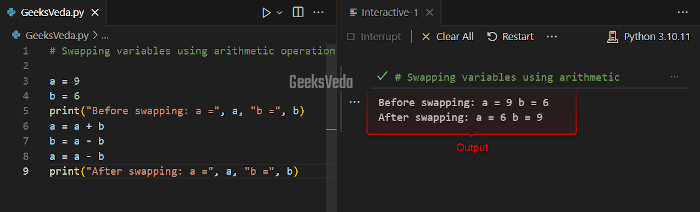
3. Using Tuple Unpacking (Multiple Assignment or One-Liners)
In Python, the tuple unpacking feature offers another elegant way of swapping variables. It is also known as the Multi Assignment or One-Liners approach. This method works by assigning the values of the variables to be swapped into a tuple and then unpacking the tuple into the variables.
According to the given program, the values of the variables “a” and “b” will be assigned to the “(b, a)” tuple, which will be then unpacked into “a” and “b“.
# Swapping variables using tuple unpacking a = 3 b = 8 print("Before swapping: a =", a, "b =", b) a, b = b, a print("After swapping: a =", a, "b =", b)
Consequently, the values of “a” and “b” will be swapped.
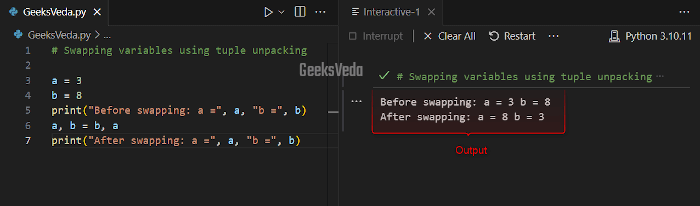
4. Using XOR Operation
The XOR “^” bitwise operator can be utilized for swapping variables. This method uses the XOR properties to interchange the values of defined variables without requiring additional storage.
For instance, in the below-given program, the values of the variables “a” and “b” have been manipulated with the help of the XOR operator. Note that the values of the specified variables will be swapped without using any temporary variable.
# Swapping variables using XOR operation a = 52 b = 14 print("Before swapping: a =", a, "b =", b) a = a ^ b b = a ^ b a = a ^ b print("After swapping: a =", a, "b =", b)
The given output signifies that the values of the “a” and “b” variables have been swapped successfully.
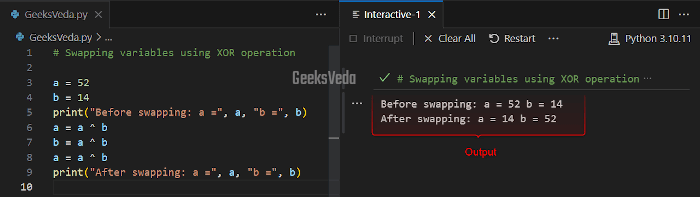
Choosing the Right Method for Variable Swapping
Let’s compare each of the discussed approaches for selecting the right method as per your requirements:
Method | Advantages | Disadvantages | Use Cases |
Temporary Variable |
|
|
Used when readability is a priority. |
Arithmetic Operations |
|
May not work for all data types. |
|
Tuple Unpacking |
|
Limited to swapping two variables. | Ideal when swapping two variables in one statement. |
XOR Operation |
|
May not be intuitive to all developers. | Useful for swapping variables in a unique way. |
That brought us to the end of our guide related to swapping two variables in Python.
Conclusion
For beginners, swapping variables might seem like a complex task. However, Python offers multiple methods for rearranging the values or data without any complication.
From the traditional approach of using a temporary variable, to arithmetic operations, and XOR operations, we have covered all in this guide.
So, understand these techniques and select the right one according to your project preferences.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!