Solving quadratic equations in Science and Mathematics is a common task and can be implemented in different languages such as Python. In today’s guide, we will explore several approaches for calculating the quadratic equations.
From using the quadratic
formula to its visual representation, these methods can be used in different scenarios. Moreover, they also provide insights into the roots of the quadratic equation.
So, let’s practical implementation and gain a deeper understanding of the mentioned solutions.
What is Quadratic Equation in Python
A quadratic equation is a second-order polynomial equation in a single variable. It can be expressed as “ax^2 + bx + c= 0“, where “a“, “b“, and “c” are the considered coefficients, and “x” refers to the variables we are solving for.
More specifically, these equations often have two solutions named “root” which can be complex or real.
There exist three types of solutions according to the discriminant which is “b^2 – 4ac“:
- If the discriminant is positive, the equation will have two distinct real roots.
- If the discriminant is negative, the equation will have two complex roots.
- If the discriminant is zero, the equation will have one real root.
How to Solve Quadratic Equations in Python
In order to solve the quadratic equation in Python, you can use:
- The Quadratic Formula
- Factoring Quadratic Equations
- Completing Square Method
- The Graphical Method
Let’s check out each of the listed approaches individually!
1. Using Quadratic Formula
The quadratic
formula is a popular method for solving quadratic equations. It utilized the coefficients “a“, “b“, and “c” for calculating the roots directory with this formula:
x = (-b ± √(b^2 - 4ac)) / 2a
In the following example, we will employ the above-mentioned quadratic formula. This program calculates the discriminant, which decides the nature of the roots.
If the discriminant is negative, the “Complex Roots” message will be returned. In the other case, two real roots will be computed with the math.sqrt() function and the respective formula. These roots will be returned as a tuple.
import math def quadratic_formula(a, b, c): discriminant = b**2 - 4*a*c if discriminant < 0: return "Complex Roots" root1 = (-b + math.sqrt(discriminant)) / (2*a) root2 = (-b - math.sqrt(discriminant)) / (2*a) return root1, root2 a = 1 b = -3 c = 2 roots = quadratic_formula(a, b, c) print("Roots:", roots)
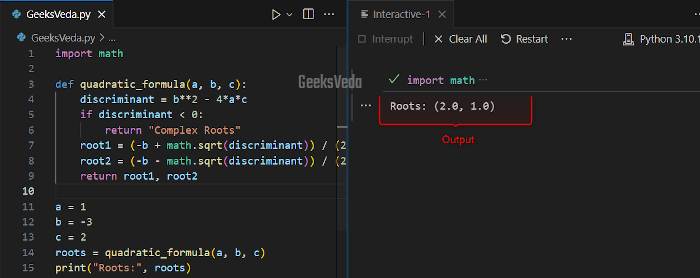
2. Factoring Quadratic Equations
The factoring approach is based on finding two numbers that can be multiplied to give “ac” (as the product of “a” and “c“) and also add up to give “b“. This method is primarily utilized for simple quadratic equations with integer coefficients.
For instance, we will now define a function named “factor_quadratic()
” that finds the integer roots for a quadratic equation by factoring.
Within this function, firstly, we will generate the factors for the product of “a” and “c” coefficients. Then, check the pairs of these factors to verify if their sum equals “b“. If the pair is found, it will return as roots, otherwise, “None” will be displayed on the console.
After invoking this “factor_quadratic()
” function with the respective coefficient value, specify an if-else statement that checks which type of value has been returned and displays the message accordingly.
def factor_quadratic(a, b, c): product = a * c factors = [] for x in range(-abs(product), abs(product) + 1): if x == 0: continue if product % x == 0: factors.append(x) for x in factors: y = product // x if x + y == b: return x, y return None a = 2 b = 7 c = 3 roots = factor_quadratic(a, b, c) if roots: print("Roots:", roots) else: print("No integer roots found.")
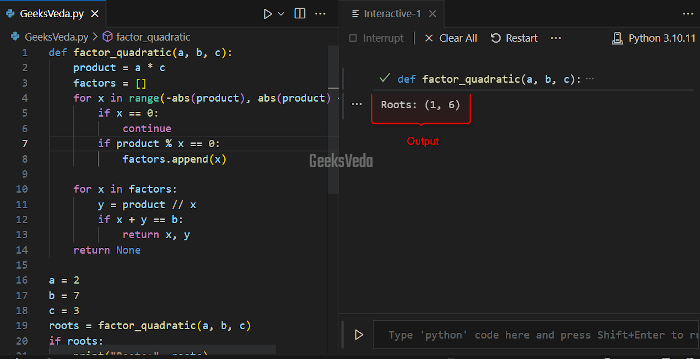
3. Using Completing the Square Method
The completing square method is all about rewriting the quadratic equation in the form “(x – p)^ 2 = q“, where “p” and “q” are constants. This form makes it easier to find the roots.
According to the given program, we have defined the “completing_the_square()
” function that accepts “a“, “b“, and “c” coefficients as inputs. This function then computes the intermediate values “p” and “q” with respect to the specified formula.
After that, the “math.sqrt()
” method has been invoked for calculating the roots, which will be then displayed with the print() function.
import math def completing_the_square(a, b, c): p = b / (2 * a) q = (b**2 - 4*a*c) / (4*a) root1 = p + math.sqrt(q) root2 = p - math.sqrt(q) return root1, root2 a = 1 b = -6 c = 9 roots = completing_the_square(a, b, c) print("Roots:", roots)
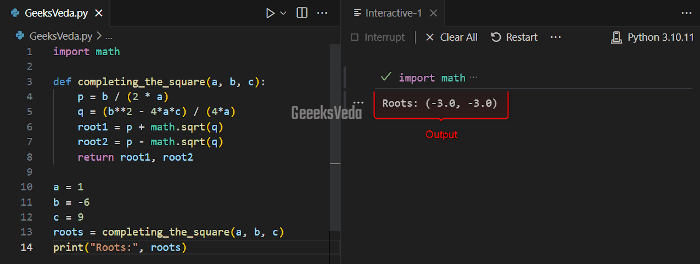
4. Using Graphical Method
In the graphical method, you can visualize the equation on the graph and identify the points where the curves cross the x-axis. This method offers a qualitative understanding of the roots. However, it might not provide accurate roots specifically for complex equations.
You can implement this method with libraries such as “matplotlib
” for visualization.
Here, the below code utilized the “matplotlib
” library for creating the visual representation of a quadratic equation. It defines the equation, then generates the “x” values, and calculates the respective “y” values.
Lastly, it plots the curve with axes, grid lines, and a legend.
import matplotlib.pyplot as plt import numpy as np # Define the quadratic equation def quadratic_equation(x): return x**2 - 4*x + 4 # Generate x values x_values = np.linspace(-2, 6, 400) # Calculate y values using the quadratic equation y_values = quadratic_equation(x_values) # Create the plot plt.plot(x_values, y_values, label='y = x^2 - 4x + 4') plt.axhline(0, color='black', linewidth=0.8) # x-axis plt.axvline(0, color='black', linewidth=0.8) # y-axis plt.title('Graph of Quadratic Equation') plt.xlabel('x') plt.ylabel('y') plt.legend() plt.grid() plt.show()
As you can see this approach offers a clear visual of the quadratic equation’s behavior.
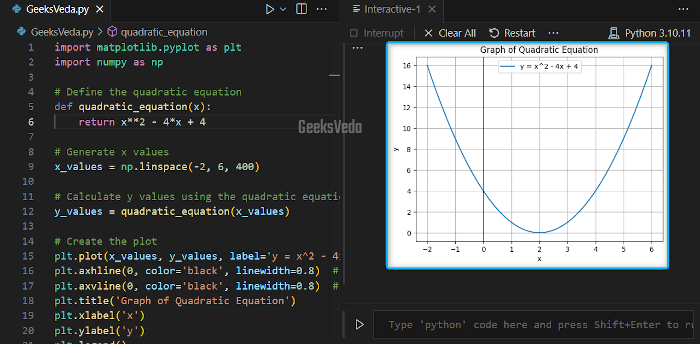
Choose the Right Method to Solve Quadratic Formula
Let’s compare each of the discussed approaches for selecting the right method for solving quadratic formula, as per requirements:
Method | Description | Pros | Cons |
Quadratic Formula | Direct algebraic formula based on coefficients. | Precise solutions. | May involve complex calculations. |
Factoring | Decomposing into factors for integer roots. | Simple for small coefficients. | May not work for all equations |
Completing the Square | Transforming to a perfect square form. | Provides exact solutions. | Requires manipulation and steps. |
Graphical Method | Visualizing intersection points on a graph. | Intuitive understanding. | Less accurate, especially for complex roots. |
That brought us to the end of today’s guide related to solving the quadratic equation in Python.
Conclusion
In Mathematics and other real-world applications, solving quadratics equations is considered a crucial skill. Therefore, in today’s guide, we have explained several approaches to solving quadratics, particularly in Python.
Whether it is factoring, quadratic formula, graphical, or completing the square method, each approach offers a valuable perspective for finding solutions.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!