While creating the weather project app in Python, you may need to convert temperatures between Celsius and Fahrenheit. This can be done using different Python approaches such as formula-based conversion, conversion function, Object-oriented approach, lambda function, or user-defined conversion precision.
More specifically, whether you are working on scientific simulations, weather applications, or everyday calculations, understanding the techniques of converting temperatures between the mentioned two units is essential.
So, let’s start the practical demonstration!
What are Celsius and Fahrenheit in Python
Celsius and Fahrenheit are the two temperature units, where Celsius is denoted as °C, and Fahrenheit as °F.
Celsius is a metric temperature scale commonly utilized worldwide. On the other hand, Fahrenheit is used in the United States, and some other countries.
Additionally, from the creation of weather forecasting to scientific calculations, it is considered crucial to convert the temperature between these two units.
How to Convert Celsius to Fahrenheit in Python
In order to convert Celsius to Fahrenheit in Python, you can use:
- Formula-based Conversion
- Conversion Function
- Object-oriented Approach
- Lambda Function
- User-defined Conversion Precision
1. Using Formula-based Conversion
Formula-based conversion is one of the simplest methods for converting Celsius to Fahrenheit. The formula for this conversion is:
Fahrenheit = (Celsius * 9/5) + 32
Now, we will implement the above-given formula in our program.
def celsius_to_fahrenheit(celsius): fahrenheit = (celsius * 9/5) + 32 return fahrenheit celsius_temperature = 25 fahrenheit_temperature = celsius_to_fahrenheit(celsius_temperature) print(f"{celsius_temperature}°C is equal to {fahrenheit_temperature}°F")
Here, we have defined a function named “celsius_to_fahrenheit()
” that accepts a Celsius temperature as an argument and then calculates the respective Fahrenheit temperature, which is then displayed on the console with print() function.
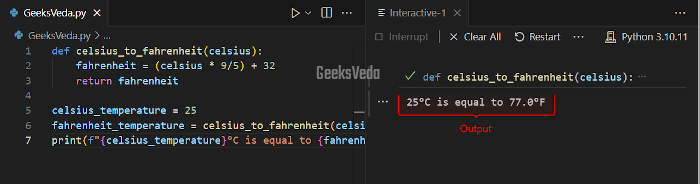
2. Using a Conversion Function
There also exist Python built-in functions that can be invoked for temperature conversion, For example, the “round()
” function is called for rounding off the decimal values to the given precision.
In the following program, we will utilize the round()
function and pass the conversion formula as its argument to round off the precision of the converted value.
def celsius_to_fahrenheit(celsius): return round((celsius * 9/5) + 32, 2) # Round to 2 decimal places celsius_temperature = 30.5 fahrenheit_temperature = celsius_to_fahrenheit(celsius_temperature) print(f"{celsius_temperature}°C is approximately {fahrenheit_temperature}°F")
Resultantly, the Fahrenheit value will be shown with two decimal points.
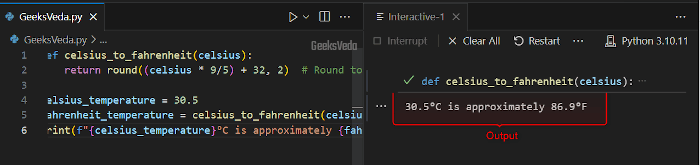
3. Using an Object-oriented Approach
Python’s object-oriented paradigm permits the creation of reusable and organized code structures. By implementing an object-oriented approach, you can encapsulate the conversion logic within a class. This promotes maintainability and modularity.
The example defines a function “TemperatureConverter
” class that accepts a Celsius temperature as its parameter. Inside this class, the “to_fahrenheit()
” method calculates the equivalent Fahrenheit temperature using the same conversion formula.
class TemperatureConverter: def __init__(self, celsius): self.celsius = celsius def to_fahrenheit(self): return (self.celsius * 9/5) + 32 celsius_temperature = 20 converter = TemperatureConverter(celsius_temperature) fahrenheit_temperature = converter.to_fahrenheit() print(f"{celsius_temperature}°C is equal to {fahrenheit_temperature}°F")
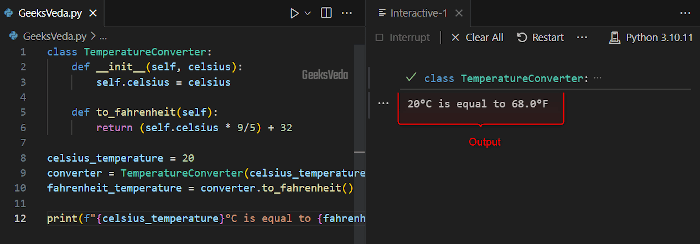
4. Using Lambda Function
Lambda or Anonymous function also supports a method for performing quick computations. In the discussed functionality, a lambda function can be utilized for temperature conversion on the fly.
Here, the “celsius_to_fahrenheit()
” function takes a Celsius temperature as its argument and immediately calculates the corresponding Fahrenheit temperature.
celsius_to_fahrenheit = lambda celsius: (celsius * 9/5) + 32 celsius_temperature = 15 fahrenheit_temperature = celsius_to_fahrenheit(celsius_temperature) print(f"{celsius_temperature}°C is approximately {fahrenheit_temperature}°F")
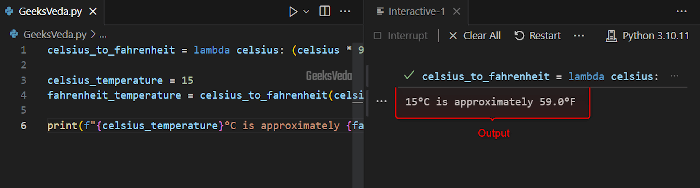
5. Using User-defined Conversion Precision
Python allows you to modify the function according to your requirements. For instance, you can control the precision of the Celsius to Fahrenheit conversion results.
According to the given program, the “celsius_to_fahrenheit()
” function accepts an additional “precision” parameter with the default value “2“.
This function invoked the round() function for controlling the precision of the result according to the specified value.
def celsius_to_fahrenheit(celsius, precision=2): return round((celsius * 9/5) + 32, precision) celsius_temperature = 28.75 fahrenheit_temperature = celsius_to_fahrenheit(celsius_temperature, precision=1) print(f"{celsius_temperature}°C is approximately {fahrenheit_temperature}°F")
It can be observed that “28.75” Celsius has been successfully converted to Fahrenheit “83.8“.
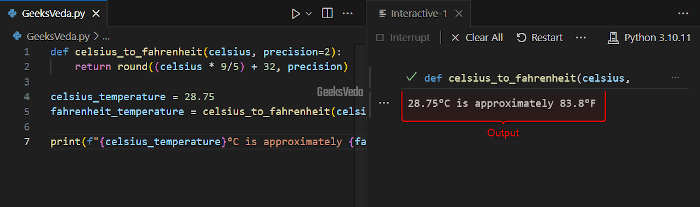
Choose the Right Method for Celsius to Fahrenheit Conversion
Here is the comparison table for selecting the right method for the Celsius to Fahrenheit conversion:
Method | Advantages | Disadvantages | Use Cases |
Formula-Based |
|
|
Quick conversions where precision is not critical. |
Conversion Function |
|
|
Basic temperature conversions with specified precision. |
Object-Oriented |
|
|
Projects requiring multiple conversions and extensibility. |
Lambda Function |
|
|
Quick conversions in small-scale scripts or functions. |
User-Defined Precision |
|
|
Scenarios demanding specific precision levels. |
That brought us to the end of this Celsius to Fahrenheit conversion in Python.
Conclusion
In today’s guide, we have explored different techniques related to mastering temperature conversion in Python. Whether you prefer the simplicity of formula-based conversion, the modularity of object-oriented programming, or the precision of user-defined conversion, Python allows this flexibility to meet the requirements of your project.
With the help of the techniques given in this guide, you can confidently convert the temperature and enhance your programming skills.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!