In Python, properly formatted dates are essential for displaying date and time information in a human-readable format.
More specifically, Python offers a robust “datetime” module that can be utilized for formatting dates according to particular requirements.
Today’s blog will explore the “datetime
” module, learn about format codes and check out examples for formatting dates effortlessly.
What is Python “datetime” Module
Python’s “datetime
” module is a robust tool for handling dates, times, and the relevant time intervals in programs. It offers several functionalities for creating, manipulating, and formatting date and time objects.
So understanding and using this module this crucial for managing time-related operations efficiently in your applications.
Working with Dates Using datetime Module in Python
This section will discuss the operations related to working with the datetime module in Python, such as creating date objects and performing arithmetic operations on them.
1. Create Date Objects
The “datetime
” allows you to create date objects with the “datetime()
” class constructor. This constructor accepts arguments for the year, month, and day, enabling you to define a particular date.
For instance, in the provided code, we will create a datetime object named “my_date
” for the July 19, 2023 date.
import datetime # Creating a date object for July 19, 2023 my_date = datetime.datetime(2023, 7, 19) print("Date Object:", my_date)
As you can see, we have successfully created the datetime object.
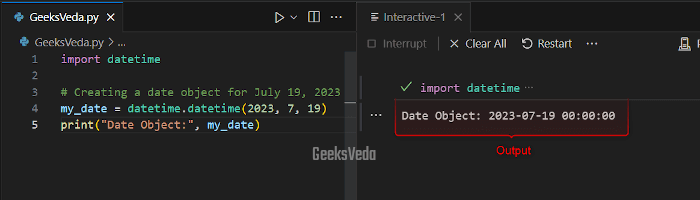
2. Date Arithmetic Operations
The datetime
module permits you to perform arithmetic operations on the date objects.
This can be done with “timedelta
” objects. More specifically, timedelta
represents a time interval or duration that enables you to add or subtract time from a date.
In this example, we will first create two date objects named “date1
” and “date2
“. After that, calculate their “time_difference
” by subtracting “date2
” from “date1
“.
import datetime # Creating date objects for two specific dates date1 = datetime.datetime(2023, 7, 19) date2 = datetime.datetime(2023, 7, 25) # Calculating the time difference between the two dates time_difference = date2 - date1 print("Time Difference:", time_difference)
As you can see, the time difference has been displayed on the console.
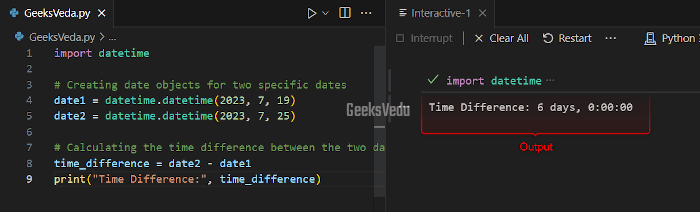
Manage Time with datetime Module in Python
The efficient management of time is essential in several applications. For this use case, Python offers the datetime module having convenient methods for handling specific data related to time.
Let’s check some of the provided crucial functionalities.
1. Create Time Objects
You can create the time object using the “time()
” class constructor of the datetime module. This method accepts the arguments for the specified time’s hour, minute, second, and microsecond components.
Resultantly, it permits you to define a particular time.
Here, we will create a time object by invoking the “time()
” method of the datetime module.
import datetime # Creating a time object for 3:30:45 PM my_time = datetime.time(15, 30, 45) print("Time Object:", my_time)
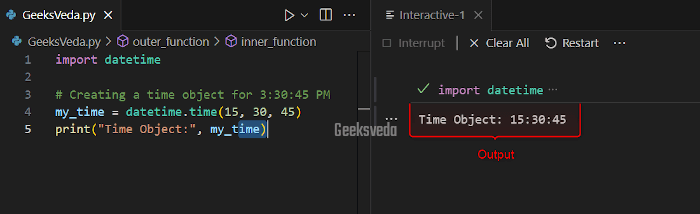
2. Time Arithmetic Operations
Similar to the methodology applied in section 2.2, you can utilize the “timedelta
” objects for performing arithmetic operations on the time objects, such as adding or subtracting time intervals.
For instance, in this program, we will calculate the difference between the given time using the time.delta()
method and display using print() function.
import datetime # Creating two time objects time1 = datetime.time(12, 30, 15) time2 = datetime.time(8, 45, 20) # Calculating the time difference between the two times time_difference = datetime.timedelta(hours=12, minutes=30, seconds=15) - datetime.timedelta(hours=8, minutes=45, seconds=20) print("Time Difference:", time_difference)
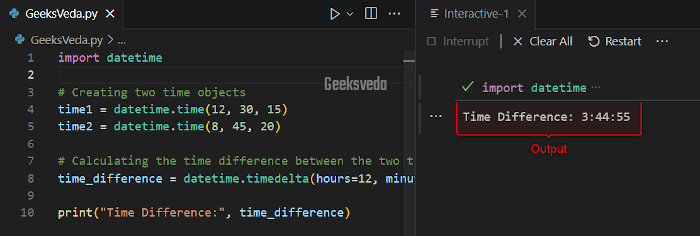
Combine Dates and Times in Python
The Python “datetime()
” method enables you to create objects that can combine both date and time as given below.
import datetime # Creating a datetime object for July 19, 2023, at 3:30:45 PM my_datetime = datetime.datetime(2023, 7, 19, 15, 30, 45) print("Datetime Object:", my_datetime)
It can be observed that the defined date and time have been combined successfully.
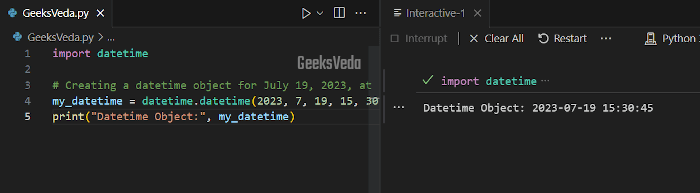
Format and Parse Dates and Times
Working with date and time often involves the operations related to conversion between string representation and datetime objects.
In this section, we will specifically check out the method for formatting datetime objects and parsing dates and times.
1. Format datetime Objects
The strftime() method can be utilized for formatting datetime objects as strings. This method accepts a format string as an argument that comprises different format codes that refer to the different datetime object parts.
Using these codes, you can format the output string as per requirements.
import datetime # Create a datetime object date_time_obj = datetime.datetime(2023, 7, 19, 15, 30, 45) # Format the datetime object using format codes formatted_date = date_time_obj.strftime("%Y-%m-%d") formatted_time = date_time_obj.strftime("%H:%M:%S") # Output the formatted strings print(formatted_date) print(formatted_time)
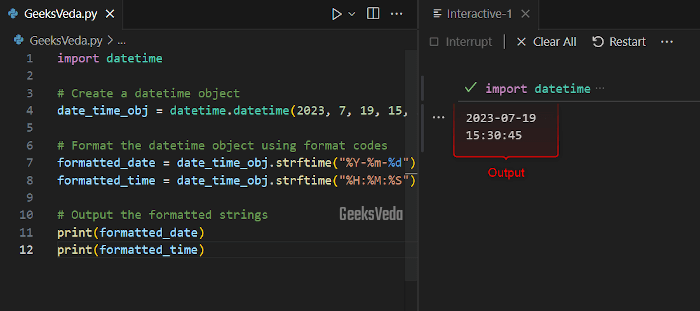
2. Parse Strings to datetime objects
Python datetime
module also enables you to convert strings representing dates and times into their corresponding datetime objects with the strptime() method.
This method accepts a string and a format string as input and outputs the respective datetime object as follows.
import datetime # A string representing a date and time date_string = "2023-07-19 15:30:45" # Parse the string into a datetime object using the format parsed_datetime = datetime.datetime.strptime(date_string, "%Y-%m-%d %H:%M:%S") # Output the parsed datetime object print(parsed_datetime)
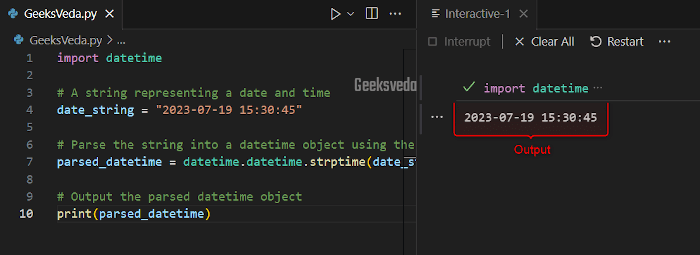
Working with Timezones
While working with global applications or handling time-sensitive data, it is essential to deal with timezones. Moreover, using the datetime module, you can understand these operations and convert timezones.
1. Understanding Timezones
Timezones refer to the regional time offset from the Coordinated Universal Time (UTC). They are important for accurately handling the time data across different locations.
Here, we will first get a timezone object for the “New_York
” time. Then, utilize the created object for fetching the current datetime in the defined timezone.
This is done by invoking the “datetime.now()
” method. Lastly, we will display the current datetime on the console.
import datetime import pytz # Get a timezone object for New York ny_timezone = pytz.timezone('America/New_York') # Get the current datetime in New York timezone ny_datetime = datetime.datetime.now(ny_timezone) # Output the current datetime in New York print(ny_datetime)
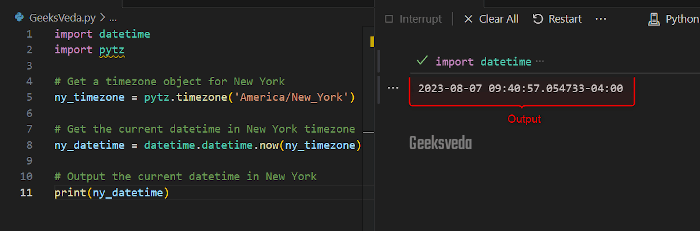
2. Convert Timezones
Invoke the “astimezone()
” method for converting datetime objects from one timezone to a specified one. This method adjusts the datetime object to the desired timezone while preserving the actual time values.
import datetime import pytz # Create a datetime object in UTC utc_datetime = datetime.datetime(2023, 7, 19, 15, 30, 45, tzinfo=pytz.utc) # Convert the UTC datetime to New York timezone ny_timezone = pytz.timezone('America/New_York') ny_datetime = utc_datetime.astimezone(ny_timezone) # Output the converted datetime objects print(utc_datetime) print(ny_datetime)
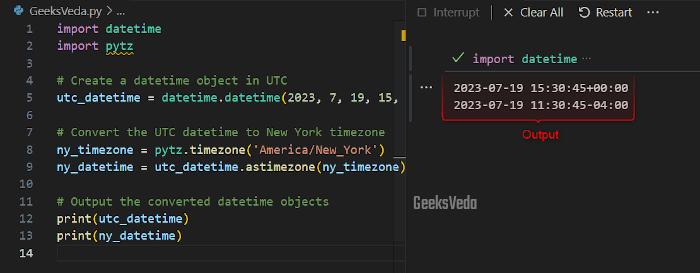
Working with Timestamps
Python timestamps represent the time as the number of seconds elapsed since January 1, 1970 (also called Unix epoch). The datetime module supports multiple methods for working with timestamps and converting them to datetime objects.
1. Introduction to Unix Timestamps
Unix timestamps are primarily used in several applications, specifically when it is required to deal with time-sensitive data or perform time-related calculations. They offer a standard way of representing time independent of daylight or timezones saving changes.
import time # Get the current timestamp current_timestamp = int(time.time()) # Output the current timestamp print(current_timestamp)
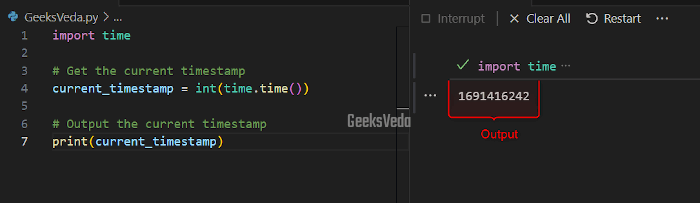
2. Convert Timestamps to datetime Objects
The “fromtimestamp()
” method of the datetime objects permits you to convert Unix timestamps into datetime objects. This method accepts the timestamp as an argument and outputs the respective datetime object as follows.
import datetime # Unix timestamp to convert timestamp = 1679474575 # Convert the timestamp to a datetime object datetime_obj = datetime.datetime.fromtimestamp(timestamp) # Output the datetime object print(datetime_obj)
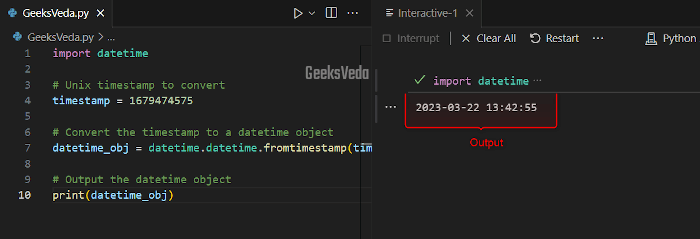
Handling Time Intervals
Time intervals are defined as the period between two datetime points. The “datetime
” module supports working with time intervals with the help of timedelta objects.
Additionally, timedelta can be used for performing arithmetic operations with datetime objects.
In the following example, firstly we created two datetime objects for the given dates. After that, we calculated the time difference between them and displayed them on the console.
import datetime # Create datetime objects for two specific dates date1 = datetime.datetime(2023, 7, 19) date2 = datetime.datetime(2023, 7, 25) # Calculate the time difference between the two dates time_difference = date2 - date1 # Output the time difference print(time_difference)
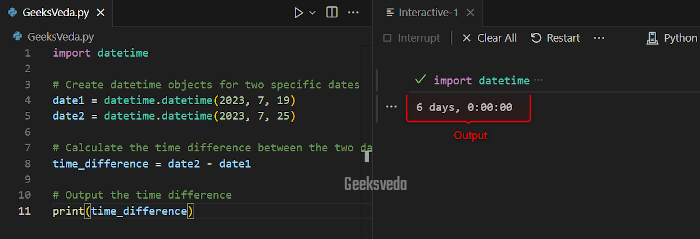
Time and Date Formatting Directives
Using format codes, you can format the datetime objects into human-readable formats. These format codes behave as placeholders for various components of the datetime objects, like a year, month, day, etc.
The below example utilizes the format codes “%Y-%m-%d
” and “%H:%M:%S
” for formatting the datetime object into a string representing the date and time, accordingly.
import datetime # Create a datetime object date_time_obj = datetime.datetime(2023, 7, 19, 15, 30, 45) # Format the datetime object using format codes formatted_date = date_time_obj.strftime("%Y-%m-%d") formatted_time = date_time_obj.strftime("%H:%M:%S") # Output the formatted strings print(formatted_date) print(formatted_time)
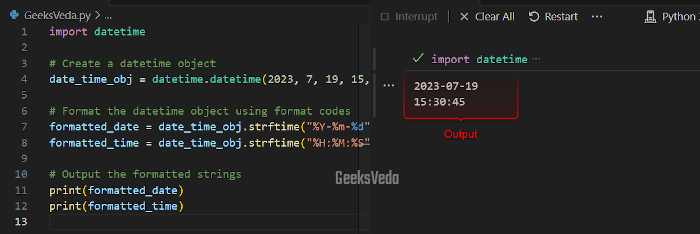
That’s all from this effective guide regarding the use of the “datetime” module.
Conclusion
Python’s “datetime
” module can be utilized for presenting or formatting dates and times in a user-friendly manner. Its format codes permit you to customize date presentations, which makes sure that your application shows dates exactly as required.
It is important for you to understand the date formatting principles for improving user experience, data visualization, and overall functionality of your Python application.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!