Python Operator Overloading is a robust tool for applying new tricks to the built-in operators. It does not intend to replace their functionality, operator overloading enhances the clarity of code and allows you to perform complex operations.
This blog will discuss the operator overloading functionality, its working with custom objects, and common use cases, and compare it with function calls as well.
What is Python Operator Overloading
Operator Overloading is a concept in Python that enables you to specify the behavior of operators for custom objects. This signifies that you can create your objects with built-in operators, such as “+
“, “*
“, “-
“, and others.
By overloading these operations, you can define custom functionality for your classes.
Understanding Python Built-in Operators
Built-in Operators play a fundamental part in Python syntax and are widely utilized for different operations. Therefore, it is essential to understand these operators first before moving to operator overloading.
Check out our detailed guide to Python Operators for a better understanding.
1. Using Comparison Operators
Comparison operators such as “<
“, “>
“, “==
“, etc can be utilized for evaluating the given conditions and making decisions. Moreover, you can also overload these operators for the custom classes.
For instance, here, we have compared “x
” and “y
” values with the comparison operators “>
” and “==
“, respectively, and displayed the results on the console with the print() function.
x = 50 y = 45 greater = x > y equal = x == y print("x > y:", greater) print("x == y:", equal)
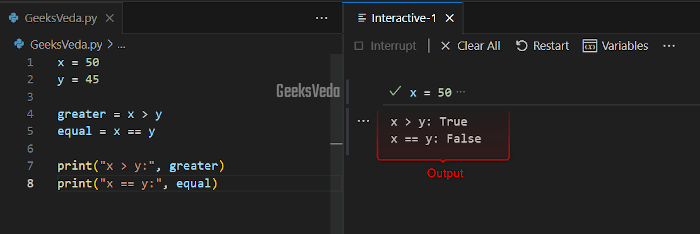
2. Using Arithmetic Operators
Arithmetic operators are commonly utilized for performing different types of mathematical calculations, such as subtraction, multiplication, division, and addition. These operators can be overloaded for performing custom operators on your objects.
Now, we will perform the arithmetic operations “+“, “-
“, “*
“, and “/
” on the values of the variables “a
” and “b
“.
a = 23 b = 13 sum_result = a + b difference_result = a - b product_result = a * b division_result = a / b print("Sum:", sum_result) print("Difference:", difference_result) print("Product:", product_result) print("Division:", division_result)
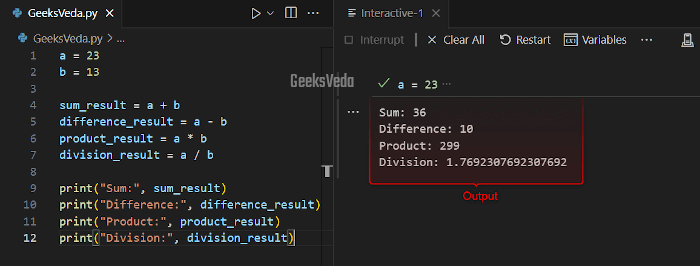
3. Using Logical Operators
The “and
“, “or
“, and “not
” are the Logical operators that are crucial for boolean operations in Python.
For instance, in the given code, we will utilize the mentioned logical operators on “p
” and “q
” boolean values and display their resultant values, respectively.
p = True q = False and_result = p and q or_result = p or q not_result = not p print("p AND q:", and_result) print("p OR q:", or_result) print("NOT p:", not_result)
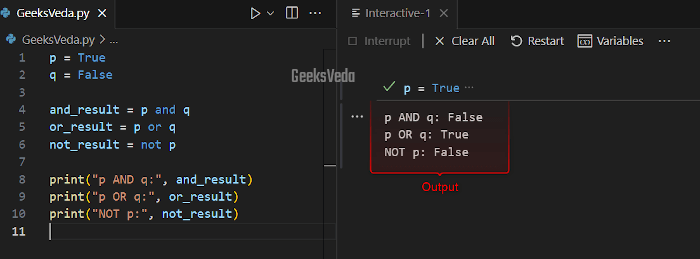
Custom Classes and Operator Overloading
Python custom classes are used for defining custom behaviors for operators. It permits you to create more expressive interactions between objects.
This section will specifically discuss the custom classes and operator overloading relationship.
1. Vector Addition
Here, the “Vector
” class demonstrates how operator loading has been done for vector additions. Note that, by defining the “__add__()
” method, you can utilize the “+
” for adding two Vector objects.
class Vector: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): return Vector(self.x + other.x, self.y + other.y) v1 = Vector(1, 2) v2 = Vector(3, 4) result = v1 + v2 print(f"Resultant Vector: ({result.x}, {result.y})")
As a result, a new Vector object will be created with combined components.
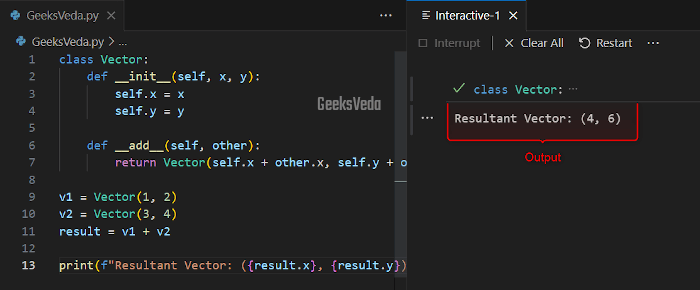
2. Complex Number Arithmetic
In this example, the “ComplexNumber
” class showcases the functionality of operator overloading for complex number arithmetic.
More specifically, with the “__add__()
” method, the “+
” operator will perform addition on the specified complex numbers.
class ComplexNumber: def __init__(self, real, imag): self.real = real self.imag = imag def __add__(self, other): real_sum = self.real + other.real imag_sum = self.imag + other.imag return ComplexNumber(real_sum, imag_sum) num1 = ComplexNumber(3, 4) num2 = ComplexNumber(1, 2) result = num1 + num2 print("Sum of Complex Numbers:", result.real, "+", result.imag, "I")
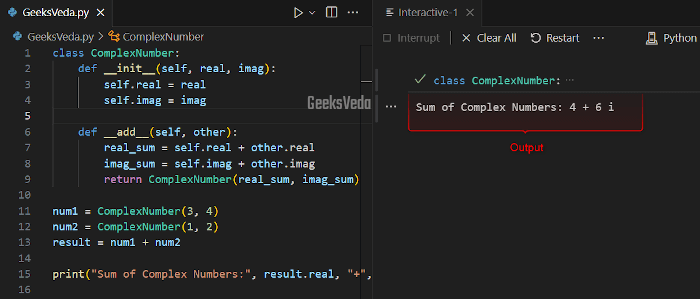
3. Matrix Addition
In the provided program, the “Matrix
” class illustrated how operator overloading can be done for matrix addition. For the corresponding purpose, the “__add__()
” method and the “+
” operator is utilized for adding the elements of two matrices.
class Matrix: def __init__(self, data): self.data = data def __add__(self, other): result = [] for row1, row2 in zip(self.data, other.data): sum_row = [x + y for x, y in zip(row1, row2)] result.append(sum_row) return Matrix(result) mat1 = Matrix([[1, 2], [3, 4]]) mat2 = Matrix([[5, 6], [7, 8]]) result = mat1 + mat2 print("Matrix Addition:") for row in result.data: print(row)
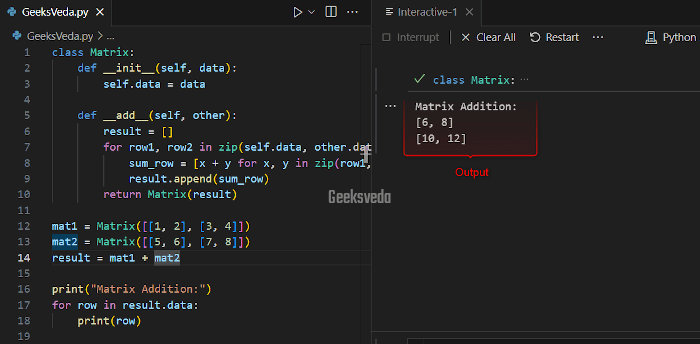
Operator Overloading Examples
Operator overloading allows custom classes to act like built-in types. This also improves the expressiveness and overall usability of your code.
1. Custom Container Class
According to the given code, the “CustomList
” class justifies the use of operator overloading for enabling custom indexing for a container. More specifically, the “__getitem__
” method permits you to utilize the indexing operator “[]
” with the CustomList objects.
class CustomList: def __init__(self, items): self.items = items def __getitem__(self, index): return self.items[index] my_list = CustomList([1, 2, 3, 4, 5]) element = my_list[2] print("Element at index 2:", element)
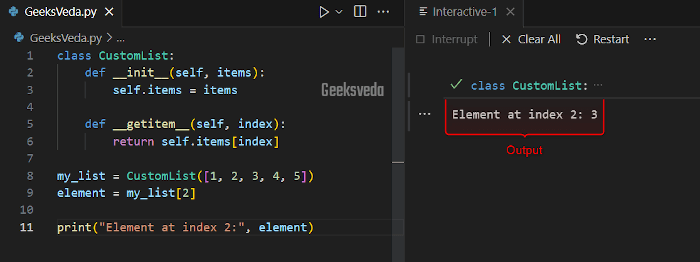
2. Custom Numeric Type
Here, the “CustomNumber
” class demonstrates the operator overloading for custom numeric types. Note that, with the “__mul__()
” method, the “*
” operator performed the multiplication operation on the CustomNumber objects.
class CustomNumber: def __init__(self, value): self.value = value def __mul__(self, other): return CustomNumber(self.value * other.value) num1 = CustomNumber(5) num2 = CustomNumber(3) result = num1 * num2 print("Product of Custom Numbers:", result.value)
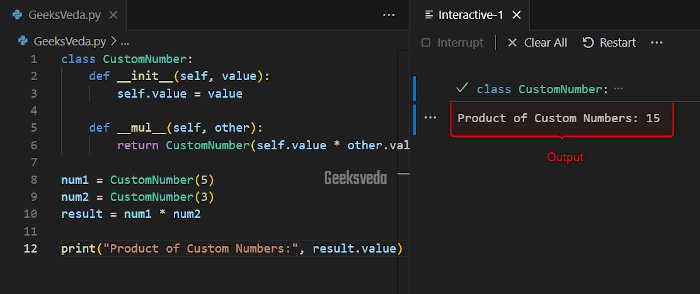
3. Complex Number Comparison
In this example, we will compare the equality of two ComplexNumber objects by implementing the “__eq__()
” method and the “==
” operator.
class ComplexNumber: def __init__(self, real, imag): self.real = real self.imag = imag def __eq__(self, other): return self.real == other.real and self.imag == other.imag num1 = ComplexNumber(3, 4) num2 = ComplexNumber(3, 4) result = num1 == num2 print("Are the complex numbers equal?", result)
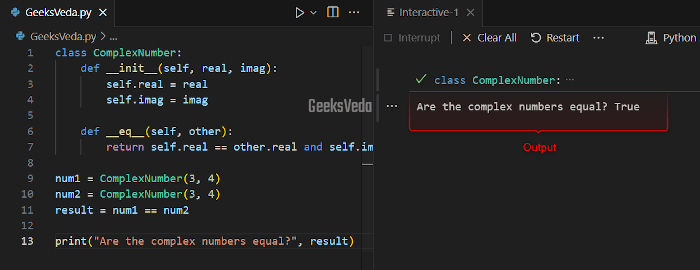
Advanced Operator Overloading Techniques
Let’s dig into operator overloading a little deeper and check out its advanced approaches for offering fine control in different scenarios, for instance, performing in-place subtraction or in-place division, etc.
1. In-Place Subtraction
Here, the “CustomerCounter
” class demonstrates the in-place subtraction operation with the “__isub__()
” method. Moreover, by utilizing the “-=
” operator, we will modify the value of the CustomCounter object.
class CustomCounter: def __init__(self, value): self.value = value def __isub__(self, decrement): self.value -= decrement return self counter = CustomCounter(15) counter -= 3 print("Updated Counter:", counter.value)
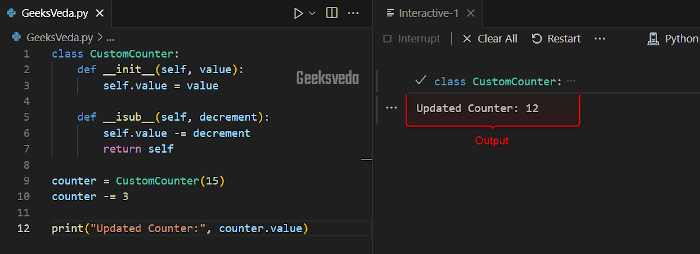
2. In-Place Division
According to the given program, the “CustomFraction
” class shows how the in-place division operator can be performed. For this purpose, the “__itruediv__()
” is used with the “/=
” operator.
This enables the direct division of the numerator and denominator of the “CustomFraction
” object.
You can use this approach for fractional calculations.
class CustomFraction: def __init__(self, numerator, denominator): self.numerator = numerator self.denominator = denominator def __itruediv__(self, divisor): self.numerator /= divisor self.denominator /= divisor return self fraction = CustomFraction(6, 8) fraction /= 2 print("Updated Fraction:", fraction.numerator, "/", fraction.denominator)
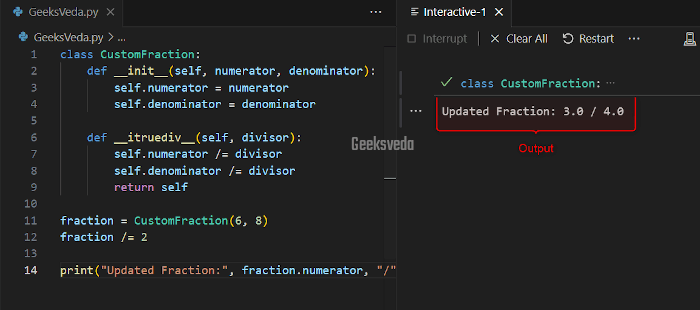
Operator Overloading vs Function Calls
Here, we will compare Operator Overloading with Function Calls based on the listed aspects:
Aspect | Operator Overloading | Function Calls |
Syntax | Uses special dunder methods. | Requires regular functions. |
Readability | Often more concise and intuitive. | May be more verbose. |
Compatibility | Works with various types. | Typically user-defined types. |
Commutativity | Supports commutative operations. | Needs separate functions. |
Polymorphism | Achieves polymorphism easily. | Requires extra method calls. |
Code Clarity | Enhances clarity and elegance. | Offers clear separation. |
Performance | May introduce slight overhead. | Can optimize certain cases. |
Flexibility | Provides custom behavior. | Limited for built-ins. |
Ease of Use | Offers elegant syntax. | Requires explicit functions. |
That’s all essential information related to operator overloading in Python.
Conclusion
Python operator overloading permits you to redefine the behavior of built-in operators when applied to custom objects. Using this technique, you can enhance data manipulations and other computations. Moreover, it also improves the clarity and expressiveness of your program.
Today’s guide covered the operator overloading approach, and its use cases, and also compared it with traditional function calls.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!