Are you a Python programmer who does not know about converting timestamps to readable date and time formats?
No worries! In today’s guide, we will check out multiple approaches for transforming timestamps into datetime objects.
More specifically, these methods include using the built-in “datetime
” module, and external libraries, such as “dateutil
” and “pytz
“.
So, let’s master the function of handling time-related data and convert timestamps to datetime objects!
What are Timestamps in Python
In Python, timestamps
refers to the way of representing dates and times in a readable and concise format. These timestamps are primarily utilized for recording and comparing time-related events in several applications, like event recording, data logging, and scheduling tasks.
Additionally, a timestamp
indicates the number of seconds or milliseconds that have elapsed according to a specific reference point, like an epoch.
This epoch has been defined as January 1, 1970, at 00:00:00 UTC.
What are Python datetime Objects
A “datetime
” object signifies a particular date and time. It comprises multiple attributes, like year, month, day, hour, minutes, second, and millisecond, which ultimately enable you to manipulate the time-related data in an efficient manner.
Moreover, the “datetime
object supports multiple functionalities, such as arithmetic operations, comparison, formatting, and time-zone handling.
How to Convert Timestamp to datetime Object in Python
Python provides different methods and libraries to perform the timestamp to datetime object conversion. In the upcoming sub-sections, we will explore the two majorly used approaches, which are using the “datetime
” built-in module and some external libraries, such as “pytz
” and “dateutil
“.
1. Using the “datetime” Module
Python’s “datetime
” module offers built-in functionalities to work with the date and time. It provides the “fromtimestamp()
” method that can be specifically used for converting the timestamps to datetime objects.
Convert Unix Timestamp to datetime
As discussed previously, the “datetime.fromtimestamp()
” method can be invoked for converting Unix timestamps to the datetime objects. This method accepts a timestamp as an argument and outputs the respective datetime object.
For instance, in the provided program, we have converted the Unix timestamp which represents August 10, 2021, at 13:51:40 to its corresponding datetime object with the “fromtimestamp()
” method.
import datetime timestamp = 1628585500 datetime_object = datetime.datetime.fromtimestamp(timestamp) print("Datetime object:", datetime_object)
It can be observed that the datetime object has been displayed successfully.
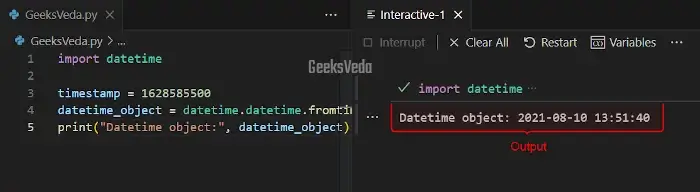
Handle Timestamps with Time Zones
The Python “datetime
” module can also have the functionality of handling the timestamps having different time zones.
Moreover, you can also localize datetime objects into particular time zones and convert them to the desired time zone.
Here, firstly, we will convert the timestamp to a datetime object with the “fromtimestamp()
” method. Then, we will manually handle the timezone offset by calling the “timedelta()
” method.
Then, subtract it from the datetime object. In this way, the datetime object has been adjusted to the desired time zone without using any external library.
import datetime timestamp_with_offset = 1628585500 # Creating a datetime object from the timestamp datetime_object = datetime.datetime.fromtimestamp(timestamp_with_offset) # Defining the time zone offset as 5 hours 30 minutes time_zone_offset = datetime.timedelta(hours=5, minutes=30) # Adjusting the datetime object to the desired time zone adjusted_datetime = datetime_object - time_zone_offset print("Adjusted Datetime:", adjusted_datetime)
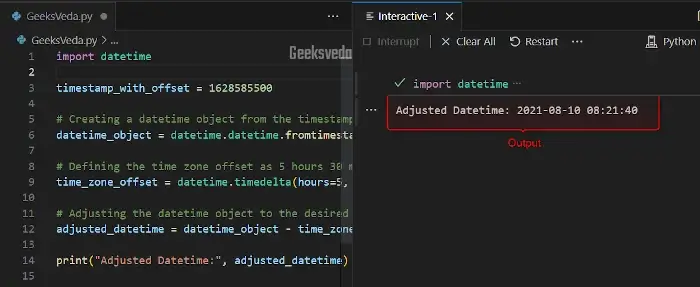
2. Using External Libraries
To jump into more advanced timestamp conversion in Python, utilize external libraries such as “pytz
” and “dateutil
“.
These libraries offer more functionalities that are not supported by the built-in “datetime
” module.
Convert timestamp With “pytz” Library
The “pytz
” Python library supports advanced time zone handling and also offers access to an extensive database of time zones.
For example, we will now first convert the given timestamp to a datetime object with its timezone as “america/New_York
”. This can be done by invoking the “pytz.timezone()
” method.
Lastly, we will display the datetime object with the print() function.
import datetime import pytz timestamp = 1628585500 # Converting the timestamp to a datetime object with time zone information timezone = pytz.timezone('America/New_York') datetime_object_with_tz = datetime.datetime.fromtimestamp(timestamp, tz=timezone) # Displaying the datetime object with time zone information print("Datetime object with time zone:", datetime_object_with_tz)
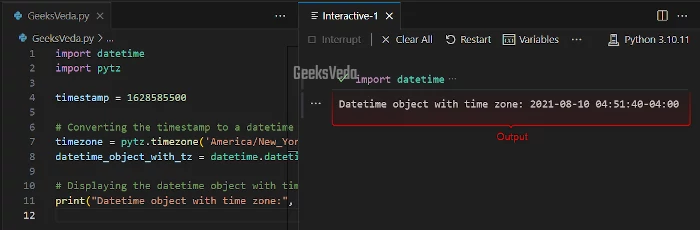
Working with “dateutil” Library
The “dateutil
” library also provides robust methods related to the manipulation and parsing of the datetime strings. This makes it useful in scenarios where you are handling multiple date and time formats.
In the following example, we have utilized the “parse()
” function of the “dateutil.parser
” module for converting the defined datetime string to a datetime object.
import datetime from dateutil.parser import parse datetime_string = '2021-08-10 12:25:00' # Parsing the datetime string and convert it to a datetime object datetime_object = parse(datetime_string) # Displaying the datetime object print("Parsed Datetime object:", datetime_object)
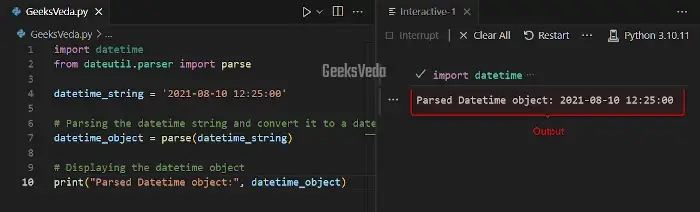
How to Format datetime Objects?
Formatting datetime objects is crucial for presenting the information related to date and time in a human-readable manner.
Moreover, the “datetime
” module offers multiple methods for customizing the format of the datetime object with respect to the requirements.
1. Display datetime in Custom Formats
In order to format datetime objects into custom string representations, the strftime() method can be invoked. It permits you to define a format string with particular format codes for representing different components of the datetime.
For instance, in the given program, first, we fetched the current date and time using the “datetime.now()
” method. After that, we called the “strftime()
” method with the desired format code.
Resultantly, we will see the current datetime in a custom format, representing the year, month, day, hour, minutes, and second.
import datetime # Current datetime object current_datetime = datetime.datetime.now() # Formatting the datetime object into a custom format formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S") # Displaying the formatted datetime print("Formatted Datetime:", formatted_datetime)
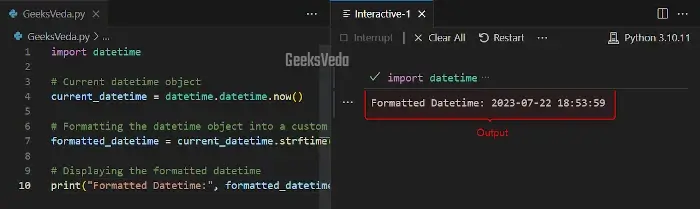
2. Extract Specific Components from datetime
You can also extract or fetch specific components like year month, day, hour, minute, and second from the given datetime object as follows.
import datetime # Current datetime object current_datetime = datetime.datetime.now() # Extracting specific components year = current_datetime.year month = current_datetime.month day = current_datetime.day hour = current_datetime.hour minute = current_datetime.minute second = current_datetime.second # Displaying the extracted components print("Year:", year) print("Month:", month) print("Day:", day) print("Hour:", hour) print("Minute:", minute) print("Second:", second)
According to the given program, we extracted the values of the “year
“, “month
“, “day
“, “hour
“, “minute
“, and “second
” attributes.
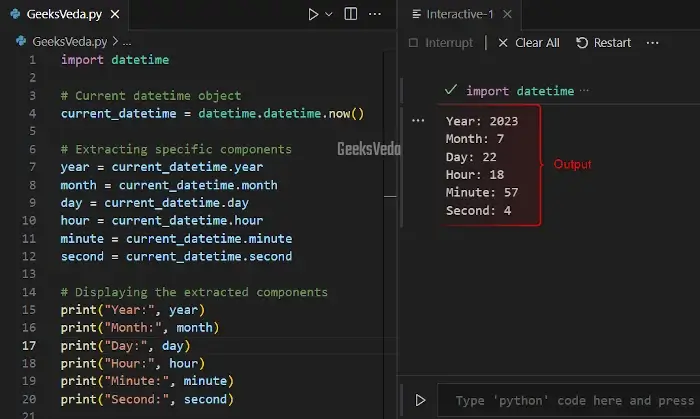
Handle Invalid or Unrecognized Timestamps
In the case of dealing with timestamps from user inputs or external sources, it is crucial to handle the unrecognized and invalid timestamps gracefully for preventing potential errors in the code.
According to the given program, the “convert_timestamp_to_datetime()
” function handles both the “ValueError
” and the “TypeError
” exceptions.
The ValueError will be raised when the input cannot be converted to a float and the TypeError will be raised when the input is None. This provides a suitable response for invalid or unrecognized timestamps.
import datetime def convert_timestamp_to_datetime(timestamp): try: # Converting the timestamp to a datetime object datetime_object = datetime.datetime.fromtimestamp(float(timestamp)) return datetime_object except (ValueError, TypeError): # Handling invalid or unrecognized timestamps return None # Invalid timestamp invalid_timestamp = "invalid_timestamp" # Converting the invalid timestamp to a datetime object result = convert_timestamp_to_datetime(invalid_timestamp) if result is None: print("Invalid or Unrecognized Timestamp") else: print("Datetime object:", result)
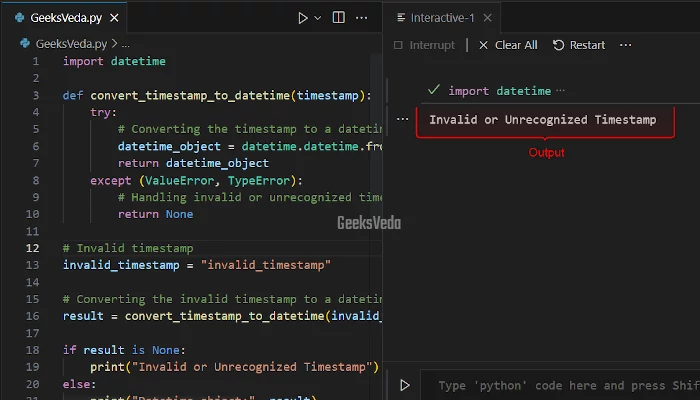
That’s all from this effective guide for converting timestamps to datetime objects.
Conclusion
Python offers robust tools for converting timestamps into datetime objects. This allows flexible presentation and manipulation of the date and time information.
More specifically, by utilizing the “datetime
” module and the external libraries, such as “pytz
” and the “dateutil
“, you can handle time zone information and also customize the datetime formats as per requirements.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!