While programming in Python, you may want to use the “time
” module.
This module equips you with different functionalities to work with time, introduce delays, measure execution time, and perform other operations.
So, whether you are a beginner or an experienced Python programmer, utilizing the time module will improve your ability for managing time-related efficiently.
Let’s start discovering the Python “time
” module right away!
What is time Module in Python
Python “time
” module is a built-in module that supports several functions for working with operations related to time. It permits you to handle time-related tasks, such as introducing time delays, measuring code execution time, and formatting dates and times.
How to Use time Module in Python
In order to use the “time
” module in the program, import it first by adding the below-given statement.
import time
After doing so, you can use the supported methods of the “time
” module as per your requirements. For example, here we have enlisted its most common functions:
Function | Description |
time() | Outputs the current time in seconds since the epoch. |
sleep() | Adds a delay in the program execution. |
ctime() | Converts a time in seconds according to the epoch to a human-readable string. |
gmtime() | Outputs the time in UTC based on the seconds according to the epoch. |
localtime() | Outputs the time in the local time zone based on the seconds according to the epoch. |
strftime() | Formats the time object into the corresponding user-readable string. |
strptime() | Parses a string and then converts it into a time object. |
Let’s check out the practical usage of each of the mentioned methods.
1. Using Python time() Method
In the first example, let’s get the current time in the form of seconds using the “time()
” method.
import time current_time = time.time() print("Current time:", current_time)
The output displayed the current time in seconds since the epoch.
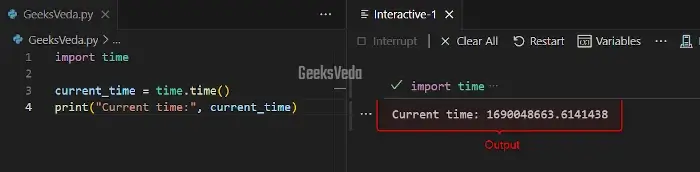
2. Using Python sleep() Method
Now, we will introduce a delay of two seconds with the help of the sleep() method.
import time print("Waiting for 2 seconds...") time.sleep(2) print("Delay complete!")
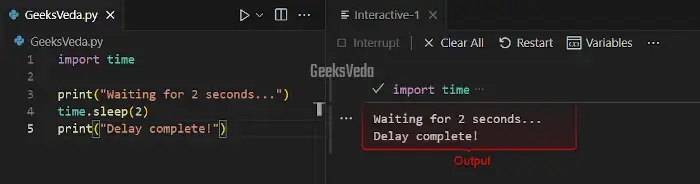
3. Using Python ctime() Method
Here, we have invoked the “ctime()
” method for converting the given epoch time in seconds to human-readable format.
import time epoch_time = 1229098400 human_readable_time = time.ctime(epoch_time) print("Human-readable time:", human_readable_time)
It can be observed that Fri Dec 12 21:13:20 2008
has been shown as the representation of the defined epoch time.
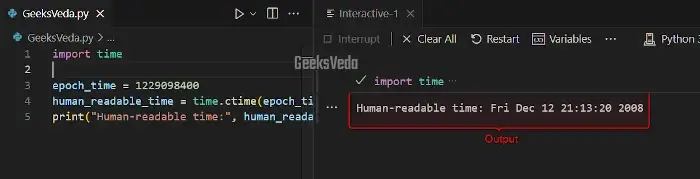
4. Using Python gmtime() Method
In the provided program, we have utilized the “gmtime()
” for getting the current time in UTC as a “time.struct_time
” object.
import time current_time = time.gmtime() print("Current UTC time:", current_time)
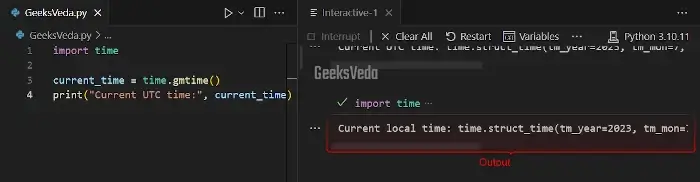
5. Using the localtime() Method
Here, the “localtime()
” function outputs the current time in the local timezone as a “time.struct_time
” object.
import time current_time = time.localtime() print("Current local time:", current_time)
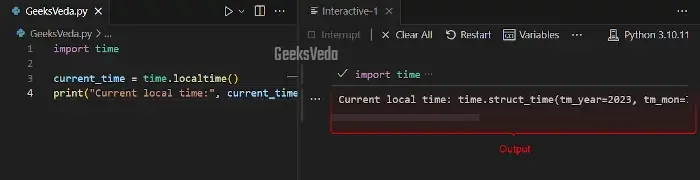
6. Using Python strftime() Method
Firstly, get the current time with the “time.localtime()
” method and then format the time as a string with strftime(). This method formatted the given object according to the format code, passed as its first argument.
import time # Getting the current time current_time = time.localtime() # Formatting the time as a string formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", current_time) print("Formatted time:", formatted_time)
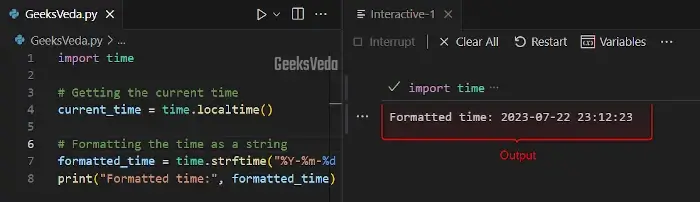
7. Using Python strptime() Method
The strptime() function can parse a string that refers to date and convert it into a “time.struct_time
” object.
import time # A string representing a date and time time_string = "2023-07-30 10:30:00" # Converting the string to a time object time_object = time.strptime(time_string, "%Y-%m-%d %H:%M:%S") print("Time object:", time_object)
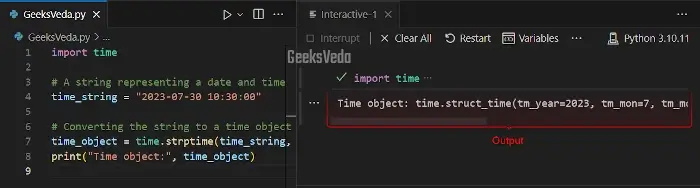
What is the epoch Time in Python
Epoch time
is referred to as a system for measuring time as the number of seconds that have elapsed since January 1, 1970, at 00:00:00 UTC. It is a common format for representing multiple computer systems.
1. How to Convert Epoch Time to Human Readable Format
In order to convert epoch time to a human-readable format, you can utilize functions such as “ctime()
” or “strftime()
“. For instance, in the below-given program, firstly we have defined the “epoch_time
” and then invoked the “time.ctime()
” function for converting it into the human-readable format.
import time epoch_time = 1629098400 human_readable_time = time.ctime(epoch_time) print("Human-readable time:", human_readable_time)
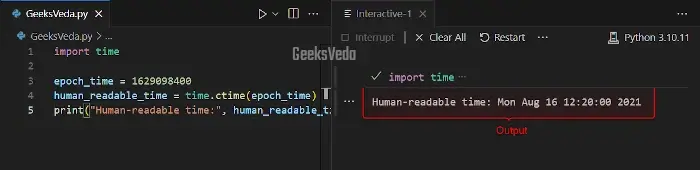
2. How to Calculate Time Differences with Epoch
In most cases, the epoch time is quite useful when you want to calculate the time difference between two events. More specifically, you can determine the time elapsed between two epoch times by subtracting them.
In the provided code, we will first calculate the sum of numbers from 1 to 1000000 and then measure the time it takes to complete this task. This can be done with the “time.time()” method. Note that, the elapsed time will be calculated by subtracting the start time from the end time.
import time start_time = time.time() # Task: Calculate the sum of numbers from 1 to 1000000 total_sum = 0 for i in range(1, 1000001): total_sum += i end_time = time.time() elapsed_time = end_time - start_time print("Elapsed time:", elapsed_time, "seconds")
As you can see, the elapsed time has been displayed on the console.
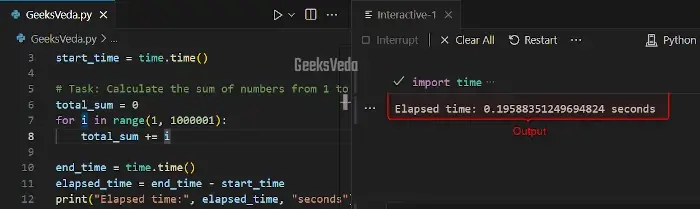
Measure Elapsed Time in Python
While doing a Python project, you may need to measure the execution time of the code for identifying the performance bottleneck. It also optimized the overall efficiency. For the corresponding purpose, Python offers different methods for measuring execution time based on the respective use cases.
1. Using Python time.time()
The “time.time()
” method of the time module is primarily utilized for measuring the execution time. It outputs the current time in seconds according to the epoch.
More specifically, you can also determine the elapsed time by capturing the start and end times of code execution and calculating the relevant difference as discussed in the section “How to Calculate Time Differences with Epoch”.
2. Profiling Code with Python timeit Module
The “timeit
” module of Python is specifically deployed for profiling code and comparing the execution of small code snippets. Moreover, it provides a simple way for creating and running timing experiments.
Here, we will use the “timeit()
” method of the “timeit
” module for calculating the elapsed time of the given for loop.
import timeit # Code to measure execution time code_to_measure = ''' for i in range(1000000): pass ''' elapsed_time = timeit.timeit(code_to_measure, number=1) print("Elapsed time:", elapsed_time, "seconds")
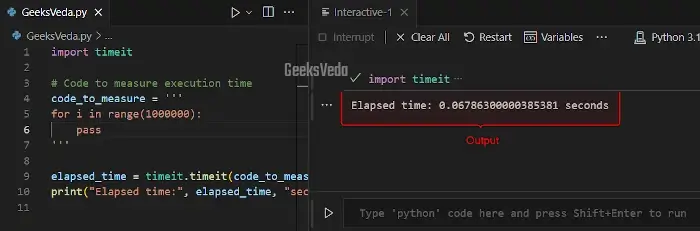
3. Measure Function Execution Time with Python timeit
Additionally, the “timeit
” module can be also utilized for measuring the execution time of a particular function. It automatically handles multiple repetitions and also outputs more accurate timing.
In the provided example, we have invoked the “timeit.timeit()
” method for calculating the execution time “my_function()
“.
import timeit # Function to measure execution time def my_function(): for i in range(1000000): pass elapsed_time = timeit.timeit(my_function, number=1) print("Elapsed time:", elapsed_time, "seconds")
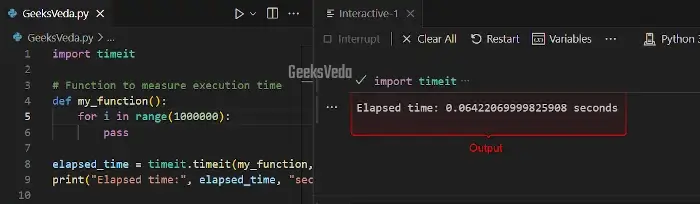
How to Create Time Delays in Python
Sometimes, you may require adding delays in programs for simulating real-time interactions, adding pauses between operations, or implementing time-sensitive tasks. For this purpose, the “time.sleep()
” function can be specifically used.
1. Time Delays with time.sleep() Module
In this section, we will demonstrate how to use the “time.sleep()
” method for suspending the program execution for the specified duration. It also permits controlling the flow of the code and creating time delays.
For instance, in the given code, we have introduced a time delay of 5 seconds using the “time.sleep()
” method.
import time print("Start") time.sleep(5) print("End")
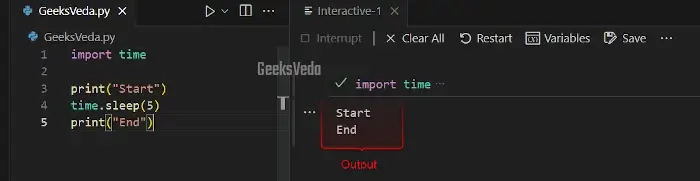
2. Time Delays with signal Module
Python “signal
” module can be utilized for setting a signal handler for handling and catching the interruptions gracefully.
Here, when we execute the below-given program, pressing the “CTRL+C
” will invoke the “interrupt_handler()
” function and print the “Delay interrupted!
” message.
import time import signal def interrupt_handler(signum, frame): print("Delay interrupted!") # Setting the interrupt handler for SIGINT (Ctrl+C) signal.signal(signal.SIGINT, interrupt_handler) print("Start") time.sleep(5) # Introducing a 5-second delay print("End")
However, in our case, we have not pressed the mentioned keys combination. So, the program will run normally.
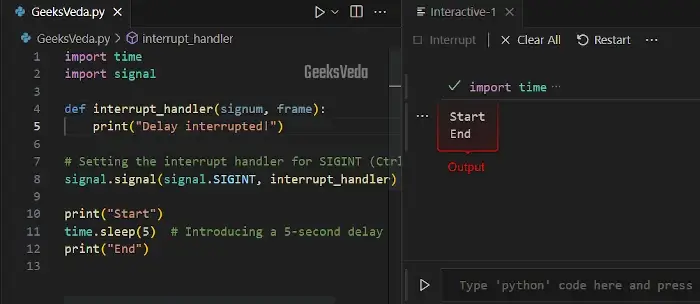
Format Dates and Times in Python
In terms of maintaining the readability of the output, it is important to format the dates and times. More specifically, the Python “time
” module offers different functions for formatting and parsing time objects.
1. Format Time Objects with time.strftime()
The “time.strftime()
” function can be invoked for converting the time object into the respective formatted string representation. It accepts a format string as an argument, which specifies the desired format for displaying the date and time components as given below.
import time current_time = time.localtime() formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", current_time) print("Formatted Time:", formatted_time)
It can be observed that the local time in seconds has been now converted into the defined format of date and time components.
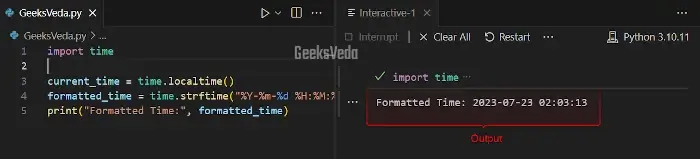
2. Customize Date and Time Format
Additionally, the “time.strftime()
” method also enables you to customize the format for displaying the date and time as per needs. In this process, the format codes act as placeholders for representing different date and time components.
Here, we will define a customized date and format and pass it to the “strftime()
” method.
import time current_time = time.localtime() formatted_time = time.strftime("Today is %A, %d %B %Y. The time is %I:%M %p.", current_time) print(formatted_time)
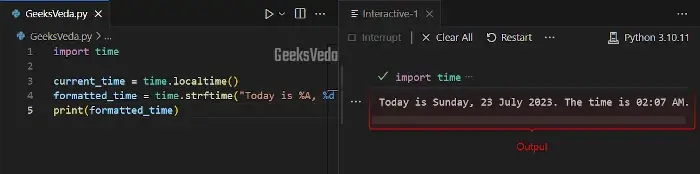
3. Parse Strings to Time Objects with time.strptime()
You can also pass an input string to the “strptime()
” method as an argument. Resultantly, the mentioned will parse the formatted string and convert it into a date object as follows.
import time input_time = "2023-07-20 10:30:00" time_object = time.strptime(input_time, "%Y-%m-%d %H:%M:%S") print("Time object:", time_object)
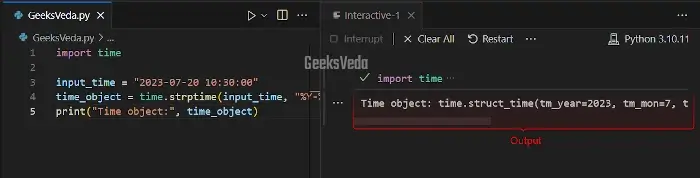
Comparing time With datetime Module
In the last section, we will provide a table of comparison “time
” vs “datetime
” module, based on different aspects:
Aspect | “time” Module | “datetime” Module |
Functionality | Limited | Extensive |
Time Representation | Unix timestamp | datetime objects |
Time Formatting | Limited options | Extensive options |
Time Zone Support | External libraries | Built-in support |
Precision | Less precise | High precision |
Ease of Use | Simpler functions | Steeper learning |
That’s all from the effective guide related to the usage of the Python time module.
Conclusion
The Python “time
” module is a crucial tool for handling time-related tasks in Python programs. It also offers functions for working with time in multiple formats, or for creating time delays, calculating execution time, and more.
However, for more extensive date and time operations, go for the “datetime” module that provides more enhanced capabilities, such as precise date formatting and advanced time zone handling.
Want to explore and learn more related to Python, do check out our dedicated Python Tutorial Series!